堆、常量池、方法区栈与队列
在Java 中,下面关于String 类和StringBuffer 类的描述正确的是哪一个
a. StringBuffer 类的对象调用toString()方法将转化为String 类型
b. 两个类都有append()方法
c. 可以直接将字符串”test” 赋值给声明的String 类和StringBuffer 类的变量
d. 两个类的实例的值都能够改变public void demo01() { StringBuffer bf = new StringBuffer("AAA"); //不可以bf = "AAA" bf.append("BBB");//String对象没有append方法 String s = bf.toString(); System.out.println(s); }
String对象的创建
“`
// new对象, 与字符串直接赋值的区别
public void demo02() {
String s1 = new String(“AAA”);//直接new对象会存储在内存的堆里边
String s2 = new String(“AAA”);
// 比较地址
System.out.println(s1 == s2);//false
// 比较对象的值
System.out.println(s1.equals(s2));//true
System.out.println();
// 不用new的字符串 会存储到常量池中
String s3 = “AAA”;
String s4 = “AAA”;
System.out.println(s3 == s4);//true
System.out.println(s3.equals(s4));//true
}
```
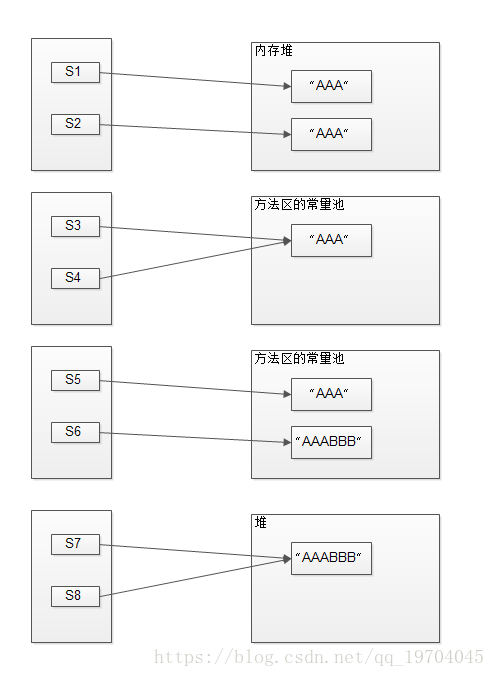
// 测试StringBuffer与Stirng的不同
public void demo03() {
String s5 = "AAA";
String s6 = s5;
s6 = s6 + "BBB";
// 字符串是不可变的,每次的修改本质上都是创建了一个副本,在副本基础上进行处理和操作
System.out.println(s5 == s6);//false
System.out.println(s5.equals(s6));//false
System.out.println();
// 如果遇到字符串的频繁相加如何处理,StringBuffer来处理,StringBuffer是可变的
StringBuffer s7 = new StringBuffer("AAA");
StringBuffer s8 = s7;
s8.append("BBB");
System.out.println(s7 == s8);//true
System.out.println(s7.equals(s8));//true
}
- 下列”栈的概念”叙述中正确的是
a. 在栈中, 栈中元素随栈底指针与栈顶指针的变化而动态变化
b. 在栈中, 栈顶指针不变, 栈中的元素对峙栈底指针的变化而动态变化
c. 在栈中, 栈底指针不变, 栈中的元素随栈顶指针的变化而动态变化
d. 上述三种说法都不对
栈和队列
栈:后进先出的结构
队列:先进先出的结构
方法的调用的过程就是入栈和出栈的过程
消息机制和线程的执行 –队列
自定义栈
// 采用数组自定义栈结构
public class MyStack<T> {
// 设置栈的初始大小
private final int DEFAULT_SIZE = 3;
// 当前栈中的元素
private int size = 0;
// 每次需要的扩展大小
private int capacity = 0;
// 永远指向能够添加元素的位置,默认是0
private int index = 0;
// 声明用来存储数据的容器(数组)
private Object[] array;
public static void main(String[] args) {
MyStack<String> stack=new MyStack<String>();
System.out.println("判断数组是否为空:" + stack.isEmpty()); // true
// 压栈操作
stack.push("A");
stack.push("B");
stack.push("C");
System.out.println("判断数组的元素数量:" + stack.size); // 3
System.out.println("查看栈顶的元素:" + stack.peek()); // C
System.out.println("判断数组的元素数量:" + stack.size); // 3
stack.push("D"); // System.out.println("enlarge..........");
System.out.println("判断数组的元素数量:" + stack.size); // 4
System.out.println("出栈操作:" + stack.pop()); // D
System.out.println("出栈操作:" + stack.pop()); // C
System.out.println("出栈操作:" + stack.pop()); // B
System.out.println("出栈操作:" + stack.pop()); // A
System.out.println("判断数组是否为空:" + stack.isEmpty()); // true
stack.clear();
System.out.println(stack.size + "," + stack.capacity); // 0 + "," + DEFAULT_SIZE
}
public MyStack() {
this.capacity = this.DEFAULT_SIZE;
this.array = new Object[this.capacity];
}
// 在创建栈的时候自定义大小
public MyStack(int capacity) {
this.capacity = capacity;
this.array = new Object[this.capacity];
}
// 判断栈是否为空
public boolean isEmpty() {
return size == 0;
}
// 查看栈顶的元素(元素并不出栈)
@SuppressWarnings("unchecked")
public T peek() {
return (T) this.array[this.index - 1];
}
// 出栈(元素会被删除)
@SuppressWarnings("unchecked")
public T pop() {
index--;
T t = (T) this.array[this.index];
this.array[index] = null;
this.size--;
return t;
}
// 压栈操作(如果栈满,则需要扩展栈空间在添加元素)
public void push(T t) {
// 首先判断栈是否已满
if (this.size < this.capacity) {
this.array[index] = t;
// 永远指向能够添加元素的位置
index++;
this.size++;
} else {
// 扩充容量,然后在添加数据
enlarge();
push(t);
}
}
// 首先创建一个新数组,然后把原始数据复制到新数组中. 然后在赋回来
public void enlarge() {
System.out.println("enlarge..........");
// 设置扩展的大小
this.capacity = this.capacity + this.DEFAULT_SIZE;
Object[] temp = new Object[capacity];
// 把原始数组的数据赋值给目标数组
System.arraycopy(this.array, 0, temp, 0, array.length);
// 把原始数组的数据清空
Arrays.fill(array, null);
// 吧新的目标数组,赋值给原始数组
this.array = temp;
}
// 清空数组,让数组回到原始状态
public void clear() {
Arrays.fill(array, null);
this.index = this.size = 0;
this.capacity = this.DEFAULT_SIZE;
// 重新创建一个数组对象
this.array = new Object[this.CAPACITY];
}
}
测试类:
public static void main(String[] args) {
Stack<Object> stack = new Stack<Object>(); // 创建堆栈对象
stack.push(new Integer(1)); // 向栈中压入
stack.push("2"); // 向 栈中 压入
stack.push(new Double(3.00)); // 向 栈中 压入
printStack(stack); // 显示栈中的所有元素
System.out.println("3.00 的位置" + stack.search(3.00));
System.out.println("元素" + stack.pop() + "出栈");
printStack(stack); // 显示栈中的所有元素
System.out.println("元素" + stack.pop() + "出栈");
printStack(stack); // 显示栈中的所有元素
System.out.println("元素" + stack.pop() + "出栈");
printStack(stack); // 显示栈中的所有元素
}
private static void printStack(Stack<Object> stack) {
if (stack.empty())
System.out.println("堆栈是空的,没有元素");
else {
System.out.print("堆栈中的元素:");
Enumeration<Object> items = stack.elements(); // 得到 stack 中的枚举对象
while (items.hasMoreElements())
// 显示枚举(stack ) 中的所有元素
System.out.print(items.nextElement() + " ");
}
System.out.println(); // 换行
}
}