/************************************************************************
* *
* 发送消息进程 *
* *
************************************************************************/
#include <stdio.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
struct msgt
{
long msgtype;
char msgtext[1024];
};
int main()
{
int msqid;
int msg_type;
char str[256];
struct msgt msgs;
/* 创建消息队列 */
msqid = msgget((key_t) 1024, IPC_CREAT);
while(1)
{
printf("please input message type, 0 for quit!\n");
/* 获取消息类型 */
scanf("%d",&msg_type);
/* 如果用户输入的消息类型为0, 退出该循环 */
if(msg_type == 0)
break;
/* 获取消息数据 */
printf("please input message content !\n");
scanf("%s", str);
msgs.msgtype = msg_type;
strncpy(msgs.msgtext, str, 256);
/* 发送消息 */
msgsnd(msqid, &msgs, sizeof(struct msgt), 0);
}
/* 删除消息队列 */
msgctl(msqid, IPC_RMID, 0);
return 0;
}
/************************************************************************
* *
* 接收消息进程 *
* *
扫描二维码关注公众号,回复:
1020274 查看本文章
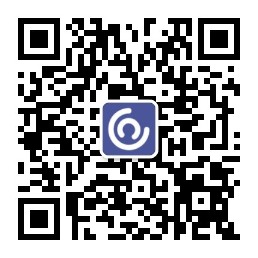
************************************************************************/
#include <stdio.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#include <unistd.h>
struct msgt
{
long msgtype;
char msgtext[1024];
};
int msqid = 0; // 消息队列的id
void childprocess()
{
struct msgt msgs;
while(1)
{
// 接收消息队列
msgrcv(msqid, &msgs, sizeof(struct msgt), 0, 0);
// 打印消息队列的数据
printf("msg text : %s\n", msgs.msgtext);
}
return;
}
int main()
{
int i;
int cpid;
/* 打开消息队列 */
msqid = msgget((key_t)1024, IPC_CREAT);
/* 创建3个子进程 */
for(i=0; i<3; i++)
{
cpid = fork();
if(cpid < 0)
printf("creat child process error!\n");
else if(cpid == 0)
childprocess();
}
return 0;
}