登录数据
mysql -uroot -proot
退出数据库
exit
quit
ctrl + d
查看数据库版本
select version();
显示时间
select now();
查看当前使用的数据库
select databases();
查看所有数据库
show databases;
创建数据库
create database python charset=utf8;
使用数据库
use python;
删除数据库
drop database python;
查看当前数据库中的所有表
show tables;
创建表
create table students(
id int unsigned primary key auto_increment,
name varchar(20) not null,
age tinyint(1),
high decimal(3,2),
dender enum('M','W'),
cls_id int unsigned
);
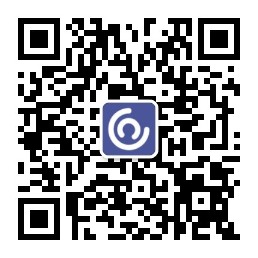
查看数据表结构
desc classes;
查看创建表结构
show create table students \G;
添加字段名
alter table students add birthday datetime;
alter table students add is_delete bit default 0; //bit 取值 0,1
重命名字段名称
alter table students change birthday birth datetime;
修改字段类型
alter table students modify birth date not null;
删除字段
alter table students drop birth;
删除表
drop table students;
查询数据
select from classes;
select from students where name='sima3';
select id,name from students;
给字段取别名
select id as '编号', name as '姓名' from students;
select age,name from students;
插入数据
insert into classes values(1, 'python20', 70);
insert into classes values(null, 'python19', 60);
单条插入
insert into students values(null, 'sima2', 18, 1.74, 'M', 1);
insert into students(id, name) values(null, 'sima3');
多条插入
insert into students values(null, 'oyang', 18, 1.74, 'M', 1),(null, 'oyang1', 18, 1.74, 'M', 1);
修改数据
update students set age=38;
update students set age=88 where name='sima3';
update students set high=1.2,gender='M' where name='sima3';
删除数据
delete from students where id=2;
数据备份
mysqldump -uroot -proot python > python.sql
mysql -uroot -proot python < python.sql
给表取别名
select s.id,s.name from students as s;
select students.id,students.name from students as s;(报错)
去重
select distinct gender from students;
比较运算符
select from students where age>18;
select from students where age=<18;
select * from students where age!=18;
逻辑运算
select from students where age>18 and age<20;
select from students where age<18 or age>20;
select * from students where not age>18;
模糊查询
select from students where name like 'wang%'; (开头)
select from students where name like '%wang%';(包含)
select from students where name like '__'; (两个字的名字)
select from students where name like '__%'; (大于 两个字的名字)
范围查询
select from students where id in (1,3);
select from students where id between 1 and 3; (包含1 3)
select * from students where id not between 1 and 3; (包含1 3)
空值判断
select from students where height is null;
select from students where height is not null;
排序 where 之后
select from students order by height;
select from students order by height desc;
select * from students order by age desc,height desc;
聚合函数
select count(*) as count from students; (记录总记录数)
select max(age) from students; (最大年龄)
select min(age) from students; (最小年龄)
select sum(age) from students; (总和)
select avg(age) from students; (平均值)
select round(avg(age),2) from students; (四舍五入, round不是聚合函数)
group分组
select gender from students group by gender; (查询年龄类别)
select gender,count() from students group by gender;
select gender,max(age) from students where gender='M' or gender='W' group by gender;
select gender,group_concat(name) from students group by gender; (group_concat 对应的名字连接)
select gender,avg(age) from students group by gender;
select gender,avg(age) as avg from students group by gender having avg>19;(分组后的年龄大于19岁)
select gender,avg(age),group_concat(name) from students group by gender;
select gender,count() as c,group_concat(name) from students group by gender having c>2;
select gender,count(*) from students group by gender with rollup;(总计)
limit限制记录 (写在sql最后)
select from students limit 0,2; (起始0,后2条记录)
select from students limit 2;
SQL标准:
select 字段 from 表 [where] [group by] [order by] [limit]
分页
select from students limit 0,2;
select from students limit (n-1)*m,m; (分页查询,n为第几页,m每页的数量)
连接 (on 关联表的条件 where 查询条件)
select from students inner join classes;(笛卡尔积)
select from students inner join classes where students.cls_id=classes.id;
select students.name,classes.name from students inner join classes where students.cls_id=classes.id;
select s.name,c.name from students as s inner join classes as c where s.cls_id=c.id;
select s.name,c.name from students as s,classes as c where s.cls_id=c.id;
select s.,c.name from students as s inner join classes as c on s.cls_id=c.id;
select from students inner join classes where students.cls_id=classes.id order by classes.id;
select i.code,i.price,f.note_info from infos i , focus f where i.id=f.id;
外连接:主表在从表没有找到数据,这从表对应给NULL
左外连接(左边表为主表,右边表为从表)
select * from students left join classes on students.cls_id=classes.id where classes.name is null;
右外连接(右边表为主表,左边表为从表)
select * from students right join classes on students.cls_id=classes.id;
自连接
select * from areas as city inner join areas province on city.pid=province.aid where province.title='广西省';
子查询
1、子查询只返回一个数据,称为标量子查询
select from students where height > (select avg(height) from students);
2、返回结果是一列,即一列多行,称为列子查询
select from students where id in (select id from classes);
去重
select cate_name from goods group by cate_name;
select distinct cate_name from goods;
select * from goods where price > (select avg(price) from goods) order by price desc;
select * from goods
inner join
(select cate_name,max(price) as max_price from goods group by cate_name ) as max_price_goods
on goods.cate_name = max_price_goods.cate_name and goods.price = max_price_goods.max_price;
分组插入
insert into goods_cates(name) (select cate_name from goods group by cate_name);
update goods inner join goods_cates on goods.cate_name=goods_cates.name set goods.cate_name=goods_cates.id;
alter table goods change cate_name cate_id int unsigned not null;
外键约束:一个表的主键在另外一个表出现,主键是另外一个表的外键;不能存在无外键的数据
alter table goods add foreign key (cate_id) references goods_cates(id);
alter table goods add foreign key (brand_id) references goods_brands(id);
create table goods_test (
id int unsigned primary key auto_increment,
name varchar(150) not null,
cate_id int unsigned not null,
brand_id int unsigned not null,
foreign key (cate_id) references goods_cates(id),
foreign key (brand_id) references goods_brands(id)
);
alter table goods_test drop foreign key 外键名称(show create table goods_test);
视图:只查询语句执行结果的字段类型和约束,把复杂的Sql封装一个虚拟表
select goods.name gname,goods_cates.name gcname,goods_brands.name gbname from goods inner join goods_cates on goods.cate_id=goods_cates.id inner join goods_brands on goods.brand_id=goods_brands.id;
创建
create view v_goods_info as
select goods.name gname,goods_cates.name gcname,goods_brands.name gbname from goods
inner join goods_cates on goods.cate_id=goods_cates.id
inner join goods_brands on goods.brand_id=goods_brands.id;
查看
show tables;
select * from v_goods_info;
删除
drop view v_goods_info;
索引:(BTREE)
前提1:对于一个经常需要更新和插入的表,就没有必要为了一个很少使用的where语句单独建立索引
查看 show index from goods;
创建 create index idx_1 on goods(name(150));
删除索引 drop index idx_1 on goods;
set profiling=1 (时间监测)
show profiles; 查看使用时间
用户管理:
查看所有用户:select host,user from user;
创建用户:create user 'wufj1'@'%' identified by 'wufj1';
授权:grant select on jing_dong.* to 'wufj1'@'%'; (多个数据库,执行多条语句)
权限:select alert drop insert update delete select =>all privileges
指定ip授权:grant all privileges on . to 'root'@'10.3.32.61' identified by 'root' WITH GRANT OPTION;;
所以权限:grant all on wufj.* to 'wufj'@'%' identified by 'wufj' WITH GRANT OPTION;;
刷新权限:flush privileges;
查看用户权限:show grants for 'wufj1'@'%';
修改权限:grant select,update on jing_dong.* to 'wufj1'@'%' with grant option;
修改密码:alter user 'wufj1'@'%' identified by '123';
删除用户:
drop user 'wufj1'@'%';
delete from user where host='%' and user='wufj1'
不同数据库之间表数据拷贝
INSERT INTO sw_setname SELECT * FROM wangsunew.sw_setname;