一、遇到问题:
今天写代码的是遇到想对vector进行排序的问题,隐约记得std::sort函数是可以对vector进行排序的,但是这次需要排序的vector中压的是自己定义的结构体(元素大于等于2),想以其中某一个元素进行正序或逆序排序,则不能直接使用sort函数。
二、解决方案:
1.C++中当 vector 中的数据类型为基本类型时,我们调用std::sort函数很容易实现 vector中数据成员的升序和降序排序,代码如下(摘自http://www.cplusplus.com/reference/algorithm/sort/):
- // sort algorithm example
- #include <iostream> // std::cout
- #include <algorithm> // std::sort
- #include <vector> // std::vector
- bool myfunction (int i,int j) { return (i<j); }
- struct myclass {
- bool operator() (int i,int j) { return (i<j);}
- } myobject;
- int main () {
- int myints[] = {32,71,12,45,26,80,53,33};
- std::vector<int> myvector (myints, myints+8); // 32 71 12 45 26 80 53 33
- // using default comparison (operator <):
- std::sort (myvector.begin(), myvector.begin()+4); //(12 32 45 71)26 80 53 33
- // using function as comp
- std::sort (myvector.begin()+4, myvector.end(), myfunction); // 12 32 45 71(26 33 53 80)
- // using object as comp
- std::sort (myvector.begin(), myvector.end(), myobject); //(12 26 32 33 45 53 71 80)
- // print out content:
- std::cout << "myvector contains:";
- for (std::vector<int>::iterator it=myvector.begin(); it!=myvector.end(); ++it)
- std::cout << ' ' << *it;
- std::cout << '\n';
- return 0;
- }
- myvector contains: 12 26 32 33 45 53 71 80
2.然而当vector中的数据类型为自定义结构体类型时,我们该怎样实现排序?
其实就是对上面代码中std::sort函数的第三个参数comp调用的函数或object进行修改即可。在这里我们使用函数作为comp作为例子,代码如下:
- #include <vector>
- #include <iostream>
- #include <algorithm>
- using namespace std;
- struct Point2
- {
- int x;
- int y;
- };
- bool GreaterSort (Point2 a,Point2 b) { return (a.x>b.x); }
- bool LessSort (Point2 a,Point2 b) { return (a.x<b.x); }
- int main()
- {
- vector<Point2> aaa;
- Point2 temp;
- temp.x=1;
- temp.y=1;
- aaa.push_back(temp);
- temp.x=2;
- temp.y=2;
- aaa.push_back(temp);
- temp.x=3;
- temp.y=3;
- aaa.push_back(temp);
- sort(aaa.begin(),aaa.end(),GreaterSort);//降序排列
- cout<<"Greater Sort:"<<endl;
- for (int i =0;i<aaa.size();i++)
- {
- cout<<aaa[i].x<<" "<<aaa[i].y<<endl;
- }
- sort(aaa.begin(),aaa.end(),LessSort);//升序排列
- cout<<"Less Sort:"<<endl;
- for (int i =0;i<aaa.size();i++)
- {
- cout<<aaa[i].x<<" "<<aaa[i].y<<endl;
- }
- return 1;
- }
- 运行结果如下:
- Greater Sort:
- 3 3
- 2 2
- 1 1
- Less Sort:
- 1 1
- 2 2
- 3 3
扫描二维码关注公众号,回复:
100996 查看本文章
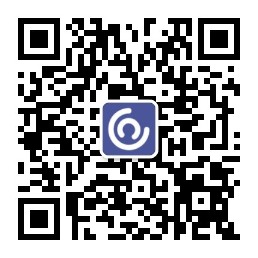
以上代码在visual stdio 2012环境下编译通过,也是自己在实践过程中的总结,如有不妥的地方,欢迎您指出。
三、参考文献:
http://www.cplusplus.com/reference/algorithm/sort/
http://blog.csdn.net/aguisy/article/details/5787257
http://blog.csdn.net/aguisy/article/details/5787257