本博客展示的仅仅只是tensorboard画出网络结构图,使得画图更加清晰,其他操作将在下一篇中展示,作为画图功能,只要用到以下两个操作
1. 命名空间
2. 将图结构利用语句tf.summary.FileWriter(‘logs/’, sess.graph)写入
import tensorflow as tf
from tensorflow.examples.tutorials.mnist import input_data
mnist = input_data.read_data_sets('MNIST_data',one_hot = True)
iteration_num = 1
learning_rate = 0.2
batch_size = 64
n_batch = mnist.train.num_examples // batch_size
with tf.name_scope('input'):
x = tf.placeholder(tf.float32, [None, 784],name = 'x_input')
y = tf.placeholder(tf.float32, [None, 10],name = 'y_input')
with tf.name_scope('weight'):
with tf.name_scope('w_weight'):
w = tf.Variable(tf.zeros([784,10]))
with tf.name_scope('b_weight'):
b = tf.Variable(tf.zeros([10]))
with tf.name_scope('wx'):
wx = tf.matmul(x,w)
with tf.name_scope('softmax'):
prd = tf.nn.softmax(wx + b)
with tf.name_scope('loss'):
loss = tf.reduce_mean(tf.nn.softmax_cross_entropy_with_logits(labels=y, logits=prd))
with tf.name_scope('train'):
train = tf.train.GradientDescentOptimizer(learning_rate).minimize(loss)
with tf.name_scope('accuracy_part'):
with tf.name_scope('correct_prd'):
correct_prd = tf.equal(tf.argmax(y,1), tf.argmax(prd,1))
with tf.name_scope('accuracy'):
accuracy = tf.reduce_mean(tf.cast(correct_prd,tf.float32))
init = tf.global_variables_initializer()
with tf.Session() as sess:
sess.run(init)
writer = tf.summary.FileWriter('logs/', sess.graph)
for epoch in range(iteration_num):
for batch in range(n_batch):
batch_xs,batch_ys = mnist.train.next_batch(batch_size)
sess.run(train, feed_dict={x:batch_xs, y:batch_ys})
acc = sess.run(accuracy,feed_dict = {x:mnist.test.images, y :mnist.test.labels})
print('Iteration_num' + str(epoch)+',Testing_acc is '+str(acc))
结果分析
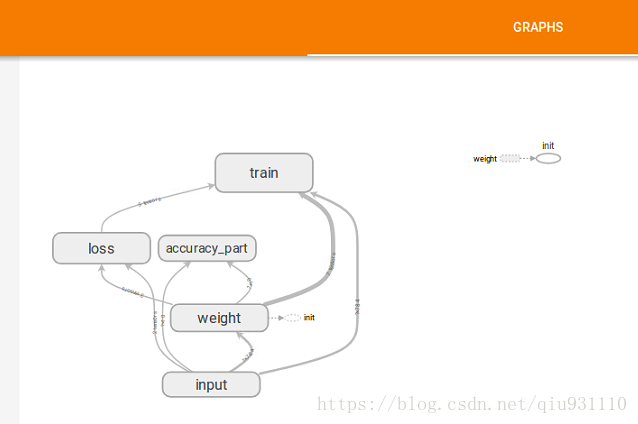