Preface
When writing automated test cases, we often encounter scenarios where we need to write procedural test cases. Generally, procedural test cases have more test steps. Adding detailed steps to the test cases will improve the readability of the test cases.
The decorator @allure.step() provided by allure is a very useful function of the allure test reporting framework. It can help us describe the test steps in detail in the test case.
Usage example of @allure.step:
Realize a shopping scenario: 1. Log in; 2. Browse products; 3. Add products to the shopping cart; 4. Place an order; 5. Pay the order;
# file_name: test_allure_step.py
import pytest
import allure
@allure.step
def login():
"""
执行登录逻辑
:return:
"""
print("执行登录逻辑")
@allure.step
def scan_good():
"""
执行浏览商品逻辑
:return:
"""
print("执行浏览商品逻辑")
@allure.step
def add_good_to_shopping_car():
"""
将商品添加到购物车
:return:
"""
print("将商品添加到购物车")
@allure.step
def generator_order():
"""
生成订单
:return:
"""
print("生成订单")
@allure.step
def pay():
"""
支付订单
:return:
"""
print("支付订单")
def test_buy_good():
"""
测试购买商品:
步骤1:登录
步骤2:浏览商品
步骤3:将商品加入到购物车中
步骤4:下单
步骤5:支付
:return:
"""
login()
scan_good()
add_good_to_shopping_car()
generator_order()
pay()
with allure.step("断言"):
assert 1
if __name__ == '__main__':
pytest.main(['-s', 'test_allure_step.py'])
Excuting an order:
> pytest test_allure_step.py --alluredir=./report/result_data
> allure serve ./report/result_data
View the test report display effect:
As you can see from the report, the steps we defined in advance through @allure.step() are displayed under the test case test_buy_good().
@allure.step supports nesting, calling step in step
# file_name: steps.py
import allure
@allure.step
def passing_step_02():
print("执行步骤02")
pass
Test case:
# file_name: test_allure_step_nested.py
import pytest
import allure
from .steps import passing_step_02 # 从外部模块中导入
@allure.step
def passing_step_01():
print("执行步骤01")
pass
@allure.step
def step_with_nested_steps():
"""
这个步骤中调用nested_step()
:return:
"""
nested_step()
@allure.step
def nested_step_with_arguments(arg1, arg2):
pass
@allure.step
def nested_step():
"""
这个步骤中调用nested_step_with_arguments(),并且传递参数
:return:
"""
nested_step_with_arguments(1, 'abc')
def test_with_imported_step():
"""
测试@allure.step()支持调用从外部模块导入的step
:return:
"""
passing_step_01()
passing_step_02()
def test_with_nested_steps():
"""
测试@allure.step()支持嵌套调用step
:return:
"""
passing_step_01()
step_with_nested_steps()
passing_step_02()
if __name__ == '__main__':
pytest.main(['-s', 'test_allure_step_nested.py'])
Excuting an order:
pytest test_allure_step_nested.py --alluredir=./report/result_data
allure serve ./report/result_data
View the test report display effect:
As you can see from the above results:
- @step can be saved to other modules first, and then imported when needed in test cases;
- @step also supports nested calls to other steps within a step; the nested form is displayed in a tree shape in the test report;
@allure.step supports adding descriptions and passing parameters through placeholders
# file_name: test_allure_step_with_placeholder.py
import pytest
import allure
@allure.step('这是一个带描述语的step,并且通过占位符传递参数:positional = "{0}",keyword = "{key}"')
def step_title_with_placeholder(arg1, key=None):
pass
def test_step_with_placeholder():
step_title_with_placeholder(1, key="something")
step_title_with_placeholder(2)
step_title_with_placeholder(3, key="anything")
if __name__ == '__main__':
pytest.main(['-s', 'test_allure_step_with_placeholder.py'])
Excuting an order:
pytest test_allure_step_with_placeholder.py --alluredir=./report/result_data
allure serve ./report/result_data
View the test report display effect:
As can be seen from the above execution results, @allure.step() supports input descriptions and supports passing parameters to the description through placeholders.
Define @allure.step in the conftest.py file
conftest.py file:
# file_name: conftest.py
import pytest
import allure
@pytest.fixture()
def fixture_with_conftest_step():
conftest_step()
@allure.step("这是一个在conftest.py文件中的step")
def conftest_step():
pass
Test case:
# file_name: test_allure_step_in_fixture_from_conftest.py
import pytest
import allure
@allure.step
def passed_step():
pass
def test_with_step_in_fixture_from_conftest(fixture_with_conftest_step):
passed_step()
if __name__ == '__main__':
pytest.main(['-s', 'test_allure_step_in_fixture_from_conftest.py'])
Excuting an order:
pytest test_allure_step_in_fixture_from_conftest.py --alluredir=./report/result_data
allure serve ./report/result_data
View the test report display effect:
As you can see from the running results, the steps defined in the fixture will be displayed in separate tree structures in setup and teardown.
[The following is the most comprehensive software testing engineer learning knowledge architecture system diagram that I have compiled in 2023]
1. Python programming from entry to proficiency
2. Practical implementation of interface automation projects
3. Web automation project actual combat
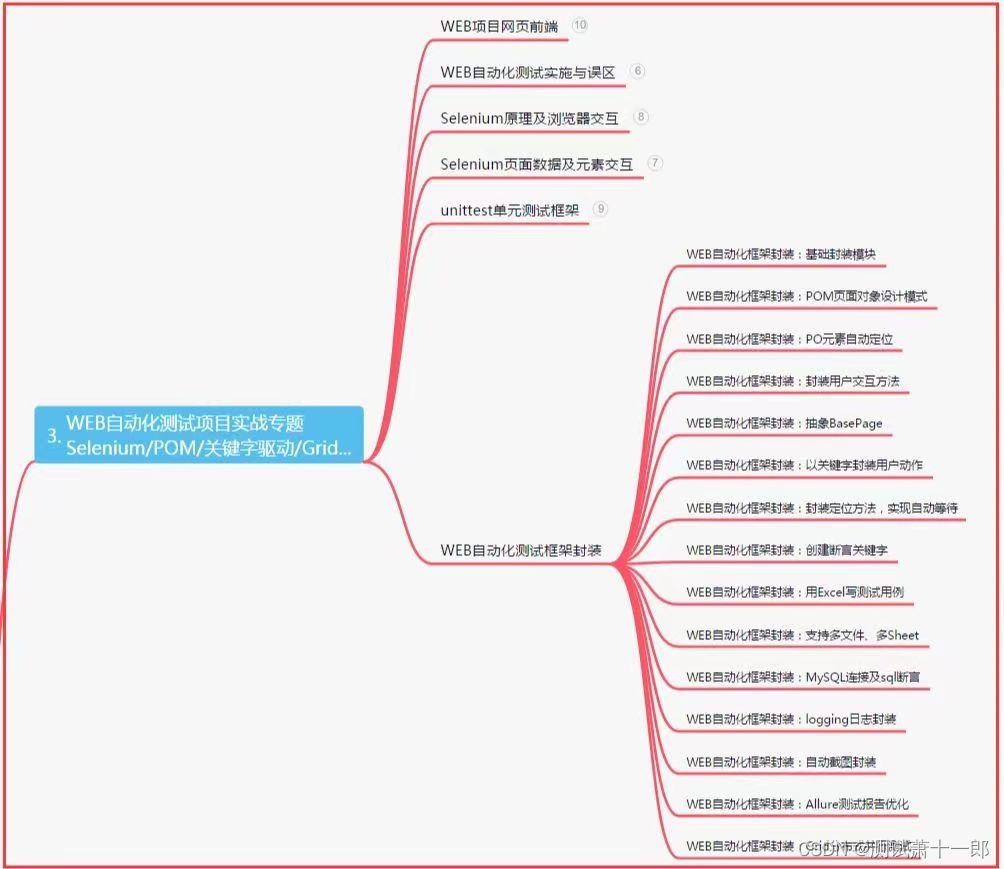
4. Practical implementation of App automation project
5. Resumes of first-tier manufacturers
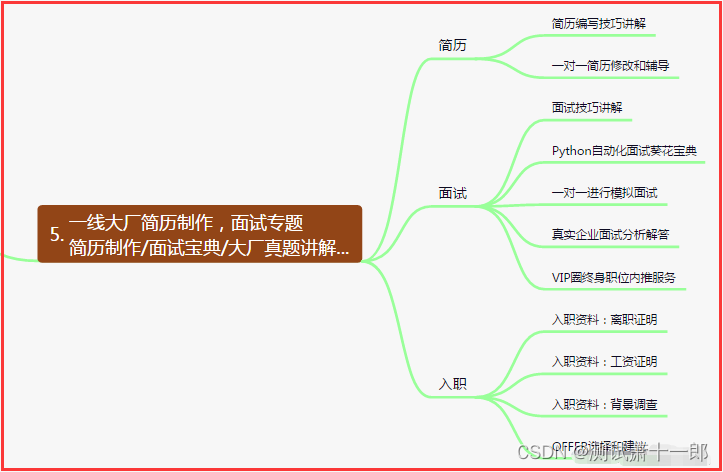
6. Test and develop DevOps system
7. Commonly used automated testing tools
8. JMeter performance test
9. Summary (little surprise at the end of the article)
life is long so add oil. Every effort will not be disappointed, as long as you persevere, you will eventually be rewarded. Cherish your time and pursue your dreams. Don’t forget your original intention and forge ahead. Your future is in your control!
Life is short and time is precious. We cannot predict what will happen in the future, but we can control the present. Cherish every day, work hard, and make yourself stronger and better. With firm belief and persistent pursuit, success will eventually belong to you!
Only by constantly challenging yourself can you constantly surpass yourself. Keep pursuing your dreams and move forward bravely, and you will find that the process of struggle is so beautiful and worthwhile. Believe in yourself, you can do it!
Finally, I would like to thank everyone who reads my article carefully. Reciprocity is always necessary. Although it is not a very valuable thing, if you can use it, you can take it directly:
This information should be the most comprehensive and complete preparation warehouse for [software testing] friends. This warehouse has also accompanied tens of thousands of test engineers through the most difficult journey. I hope it can also help you!