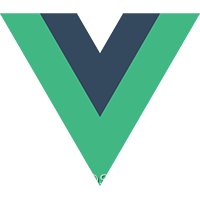
COVID-19 Self-Detection System Web Design and Development Documents
Sylvan Ding's first project based on Vue.js. The information provided in this project is for reference only, and the accuracy, validity, timeliness and completeness of the information are not guaranteed. For more information, please see the National Health and Health Commission Commission website!
Explore the docs »
View Demo · Report Bug · Request Feature
Vue project encapsulates Axios
During the development process, Axios needs to be further encapsulated to facilitate its use in the project, which tends to reduce code duplication.
development environment
node | 14.16.1 |
---|---|
npm | 8.18.0 |
view-cli | 2.9.6 |
vue | 2.5.2 |
solution
In order to avoid polluting the global Axios and affect other requests when one request modified, we use src/utils/requests.js
to create a new instance of axios with a custom config. You can switch different base URLs according to different environments. And all JSON mocks used by Axios in development environment have been put in static/mock/*.json
for the purpose of keeping web’s original file structure, which allows you to access them by http://localhost:8080/static/mock/*.json
.
When using Axios to Get data and load it in Element UI components, we import request.js
and create function loadData()
in Vue methods, then call this method in the hook function mounted()
:
<script>
import request from '@/utils/request'
export default {
data() {
return {
tableData: [],
}
},
mounted() {
this.loadData()
},
methods: {
loadData() {
request.get('/to/mock/file.json').then((response) => {
this.tableData = response.data
})
},
},
}
</script>
src/utils/requests.js
:
import {
errorMsg } from '@/utils/msgsettings.js'
const axios = require('axios')
const qs = require('qs')
const prodBaseURL = 'http://localhost:5000'
let axiosConfig = {
timeout: 3000,
// `transformRequest` allows changes to the request data before it is sent to the server
// This is only applicable for request methods 'PUT', 'POST', 'PATCH' and 'DELETE'
// The last function in the array must return a string or an instance of Buffer, ArrayBuffer,
// FormData or Stream
// You may modify the headers object.
transformRequest: [
function(data, headers) {
// Do whatever you want to transform the data
headers['content-type'] = 'application/x-www-form-urlencoded'
data = qs.stringify(data)
return data
},
],
}
// set baseURL under production environment
if (process.env.NODE_ENV === 'production') {
axiosConfig.baseURL = prodBaseURL
}
const instance = axios.create(axiosConfig)
// Add a request interceptor
instance.interceptors.request.use(
function(config) {
// Do something before request is sent
return config
},
function(error) {
// Do something with request error
errorMsg(error)
return Promise.reject(error)
}
)
// Add a response interceptor
instance.interceptors.response.use(
function(response) {
// Any status code that lie within the range of 2xx cause this function to trigger
// Do something with response data
console.log(response)
return response
},
function(error) {
// Any status codes that falls outside the range of 2xx cause this function to trigger
// Do something with response error
errorMsg(error)
console.log(error)
return Promise.reject(error)
}
)
export default instance
Please indicate the source when reprinting: ©️ Sylvan Ding 2022