1. The basic principle of key scanning
The schematic diagram of the general independent button:
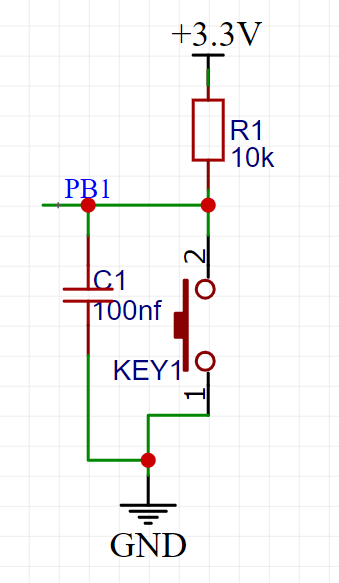
Recognition of button signals:
Generally speaking, one end of the two pins of the button is pulled up to a high level through a resistor, and the other end is grounded. When no key is pressed, the input pin is high level, and when a key is pressed, the input pin is low level. By repeatedly reading the signal of the key input pin, and then identifying the high and low levels to determine whether there is a key trigger.
The necessity of key debounce:
When a key is pressed, it may be an interference signal. Therefore, it is necessary to filter these interference signals through de-jitter processing, so as to obtain a real key trigger signal.
How to debounce:
After the low level of the key input pin is detected for the first time, after a short delay, the pin is read again. If it is still low, it is confirmed as a key trigger signal; otherwise, it is judged as an interference signal and will not be processed.
2. Commonly used functions about buttons in the HAL library
Level output function
void HAL_GPIO_WritePin(GPIO_TypeDef *GPIOx, uint16_t GPIO_Pin, GPIO_PinState PinState);
level flip function
void HAL_GPIO_TogglePin(GPIO_TypeDef *GPIOx, uint16_t GPIO_Pin);
Level input function
GPIO_PinState HAL_GPIO_ReadPin(GPIO_TypeDef *GPIOx, uint16_t GPIO_Pin);
example:
Judging the input signal of PB1 pin, if it is high level, switch the switch state of the LED light controlled by PB10 pin.
if(HAL_GPIO_ReadPin(GPIOB, GPIO_PIN_1) == GPIO_PIN_SET)
{
HAL_GPIO_TogglePin(GPIOB,GPIO_PIN_10);
}
3. Training case:
On the STM32F103C8T6 development board, STM32CubeMX and Keil5 are used for collaborative development to complete the following functions: Press the button (PB1) to switch the level of PB10. (See the beginning of the article for the schematic diagram of the independent button)
(1) CubeMX configuration
Configure PB1 as pull-up input mode, and change the username to KEY.
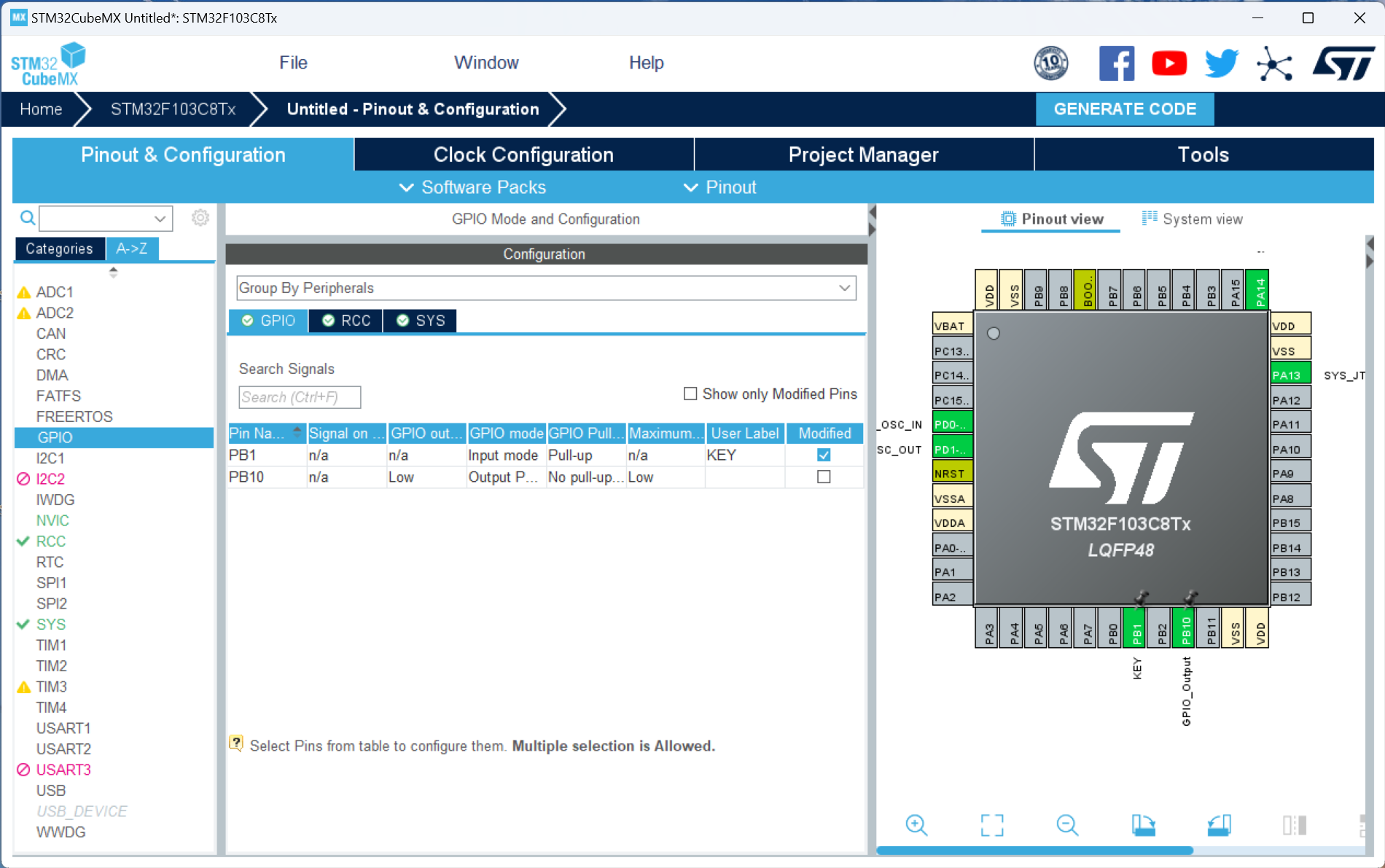
(2) Keil5 write code
/* Private user code ---------------------------------------------------------*/
/* USER CODE BEGIN 0 */
#define KEY HAL_GPIO_ReadPin(GPIOB,GPIO_PIN_1)
void Scan_Key()
{
if(KEY == GPIO_PIN_RESET)
{
HAL_Delay(5);
if(KEY == GPIO_PIN_RESET)
{
HAL_GPIO_TogglePin(GPIOB,GPIO_PIN_10);
}
while(KEY == GPIO_PIN_RESET);
}
}
/* USER CODE END 0 */
/* Infinite loop */
/* USER CODE BEGIN WHILE */
while (1)
{
Scan_Keys();
/* USER CODE END WHILE */
/* USER CODE BEGIN 3 */
}
/* USER CODE END 3 */