Introduction to Software Engineering - Personal Project
Topic requirements
user:
Elementary, middle and high school mathematics teachers.
Function:
1. Enter the user name and password on the command line, separated by a space (the program presets three accounts for elementary school, junior high school and high school, see the attached table for details), if the user name and password are correct, it will display " The current choice is XX, where XX is one of the three options of primary school, junior high school and high school. Otherwise, it prompts "Please enter the correct user name and password", and re-enter the user name and password; while loop
2. After logging in, the system prompts "Ready to generate XX math questions, please enter the number of generated questions (entering -1 will log out of the current user and log in again):", XX is one of the three options of primary school, junior high school and high school, and the user enters The number of questions required for the paper will be prepared according to the account type by default. The number of operands for each question is between 1-5, and the value range of operands is 1-100;
3. The effective input range of the number of questions is "10-30" (including 10, 30, or -1 to log out), and the program will generate papers with questions that meet the difficulty of elementary school, junior high school and high school according to the number of questions entered (see the attached surface). The questions in the papers of the same teacher cannot be repeated with the questions in the previous papers (subject to the files existing in the specified folder, see 5);
4. In the login state, if the user needs to switch the type option, enter "switch to XX" in the command line, XX is one of the three options of elementary school, junior high school and high school. When the input item does not meet the requirements, the program console will prompt "Please Enter one of the three options of primary school, junior high school and high school"; after the input is correct, it will display "" and the system prompts "Ready to generate XX math questions, please enter the number of questions to be generated", the user inputs the number of questions for the required paper, and the system will update Set the type of question;
5. The generated questions will be saved in the form of "year-month-day-hour-minute-second.txt", one folder for each account. Each question has a question number, and there is a blank line between each question;
6. Individual projects should be submitted to the innovative course management system before 10:00 pm on September 11. Submission method: The project file is packaged, and the compressed package is named "Several Classes + Name.rar". Points will be deducted for those who are late for 2 days or less, and 20% will be deducted for each day. 0 points for late submission of 2 days or more.
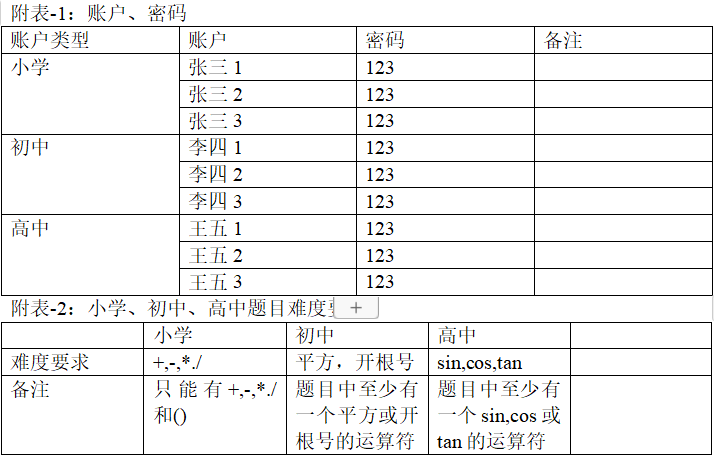
code analysis
1. User class-User
According to the requirements of the topic, the user class should mainly have three attributes: user name, password, and the type of school to which the user belongs. Set up the constructor, getter, and setter of the User class to facilitate subsequent addition and modification operations.
Define the makePaper method to generate corresponding types of test papers.
Note: Use the equals() method when judging that the characters are the same
public class User {
private String username;
private String password;
private String type;
public User(String username, String password, String type) {
this.username = username;
this.password = password;
this.type = type;
}
public String getUsername() {
return username;
}
public String getPassword() {
return password;
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
//开始制作试卷
public void makePaper(User user, int num) {
PaperMaker paperMaker = new PaperMaker();
if (this.type.equals("小学")) {
paperMaker.makePrimary(user, num);
} else if (this.type.equals("初中")) {
paperMaker.makeJunior(user, num);
} else if (this.type.equals("高中")) {
paperMaker.makeSenior(user, num);
}
}
}
2. Test paper generation class-PaperMaker
Because the topic is divided into three levels of difficulty, three linked lists are created to store the symbols corresponding to the level of difficulty, and the data is initialized in the constructor.
//小学专用符号
private LinkedList<String> primary = new LinkedList<>();
//初中专用符号
private LinkedList<String> junior = new LinkedList<>();
//高中专用符号
private LinkedList<String> senior = new LinkedList<>();
public PaperMaker() {
primary.add("+");
primary.add("-");
primary.add("×");
primary.add("÷");
junior.add("²");
junior.add("√");
senior.add("");
senior.add("sin");
senior.add("cos");
senior.add("tan");
}
makePrimary is the method to generate elementary school questions: pass in the object user and the number of questions to be generated, and then generate.
To achieve random operands, set the cnt counter, and fix the maximum number to 5; set the breakOrNot parameter, which is randomly updated after each data addition to determine whether to exit, so as to realize the function of random operands.
Realize the function of random brackets, set the bracket parameter to record the number of unclosed brackets, set the bracketOrNot parameter to take a random number every round to decide whether to add a left bracket, set the rightBracket parameter to take a random number every round to decide whether to add a right bracket, after the calculation is generated, Complete the right bracket at the end of the expression according to the value of bracket.
//生成小学难度题目 生成数量是number
public void makePrimary(User user, int number) {
//生成多少道题目就执行多少次循环
for (int i = 0; i < number; i++) {
Random random = new Random();
StringBuffer stringBuffer = new StringBuffer();
//判断括号配对
int bracket = 0;
//判断退出
int breakOrNot = 0;
//操作数最多个数
int cnt = 5;
//保证操作数不超过5个
while (cnt-- > 0) {
//判断是否本轮退出
breakOrNot = random.nextInt(2);
//随机加入 (
int bracketOrNot = random.nextInt(3);
if (bracketOrNot == 1 && breakOrNot != 1 && cnt != 0) {
bracket++;
stringBuffer.append("(");
}
//随机生成1-100之间的操作数
int ran = random.nextInt(100) + 1;
stringBuffer.append(ran);
//判断 加入 )
int rightBracket = random.nextInt(2);
if (bracketOrNot != 1 && bracket > 0 && rightBracket == 1) {
stringBuffer.append(")");
bracket--;
}
//随机结束算式
if (cnt != 4) {
if (breakOrNot == 1 || cnt == 0) {
break;
}
}
//随机生成符号
int symbolSelect = random.nextInt(4);
String symbol = primary.get(symbolSelect);
stringBuffer.append(symbol);
}
//补全 )
for (int j = 0; j < bracket; j++) {
stringBuffer.append(")");
}
//输出 =
stringBuffer.append("=");
//生成试卷
IOController ioController = new IOController();
//生成试卷文件
File newFile = ioController.makeFile(user);
//存储题目
String title = stringBuffer.toString();
//题目查重
if (ioController.check(newFile, title) == true) {
i--;
continue;
}
//写入试卷
ioController.writeIn(newFile, i + 1, title);
}
}
makeJunior is a method for generating junior high school questions. The logic is basically similar to the above, and a method of randomly adding root signs and squares is added. Since it is necessary to ensure that each expression has at least one square root or square, define squareOrNot = 1 outside the while loop to ensure that each expression has a square.
int squareOrNot = 1;
//随机加根号
int evolutionOrNot = random.nextInt(2);
if (evolutionOrNot == 1) {
stringBuffer.append(junior.get(1));
}
//随机加平方
if (squareOrNot == 1) {
stringBuffer.append(junior.get(0));
}
squareOrNot = random.nextInt(2);
makeSenior is a method for generating high school questions. The logic is basically similar to the above. The method of adding trigonometric functions is added and will not be explained in detail.
int tFunction = random.nextInt(3) + 1;
//随机生成三角函数
stringBuffer.append(senior.get(tFunction));
tFunction = random.nextInt(4);
3. File output class - IOController
The file output is mainly divided into three parts:
Find or generate the corresponding folder and txt file
First determine the path of the folder. If the folder does not exist before, create a new folder. Then get the current time, generate the title of the txt file, and create this txt file.
//生成专用文件夹 和 txt文件
public File makeFile(User user) {
String mkPath = "D:\\JavaProject\\AutomationPaper\\paper\\" + user.getUsername();
File file = new File(mkPath);
Date date = new Date();
//时间处理 用于txt文件的名称
SimpleDateFormat simpleDateFormat = new SimpleDateFormat("yyyy-MM-dd-HH-mm-ss");
String title = simpleDateFormat.format(date) + ".txt";
if (!file.exists()) {
//单例模式 如果没有对应文件夹则生成
file.mkdirs();
}
File paper = new File(mkPath, title);
try {
paper.createNewFile();
} catch (IOException e) {
e.printStackTrace();
}
return paper;
}
file writing
Nothing to say, just look at the code.
Remember to call the flush() function to refresh the file after writing, otherwise writing may fail.
//写入文件
public void writeIn(File file, Integer n, String title) {
try {
//传入文件 确定类型为续写
FileWriter fileWriter = new FileWriter(file, true);
//序号
String num = n.toString() + ".";
fileWriter.append(num + " " + title);
//空一行
fileWriter.append("\r\n");
fileWriter.append("\r\n");
//刷新文件,关闭writer
fileWriter.flush();
fileWriter.close();
} catch (IOException e) {
e.printStackTrace();
}
}
Mathematical check
Traverse the upper-level directory of the txt file to obtain all the test questions obtained by the user, and then traverse and compare each set of test questions, return true if repeated, otherwise return false.
public boolean check(File file, String title) {
//定义变量 是否重复
boolean repetition = false;
//获取txt文件上一级目录的路径
String parentPath = file.getParent();
File parent = new File(parentPath);
//遍历上一级目录,获得其下的所有文件
File[] files = parent.listFiles();
//一个一个文件进行查重对比
for (int i = 0; i < files.length; i++) {
try {
FileReader fileReader = new FileReader(files[i]);
BufferedReader bufferedReader = new BufferedReader(fileReader);
while (true) {
//读取一行数据
String usedTitle = bufferedReader.readLine();
//如果是空行就跳过
if (usedTitle == null) {
break;
}
//以" "为分隔符," "后面的就是算式
String[] uT = usedTitle.split(" ");
if (uT.length > 1) {
if (uT[1].equals(title)) {
System.out.println("重复");
//如果重复改变变量的值
repetition = true;
}
}
}
} catch (Exception e) {
e.printStackTrace();
}
}
return repetition;
}
4. Login class - Login
The login class needs to store the information of the current existing users, and is responsible for the user's login judgment, operation judgment and changing the user type.
information initialization
HashMap<String, User> userMap = new HashMap<>();
public Login() {
userMap.put("张三1", new User("张三1", "123", "小学"));
userMap.put("张三2", new User("张三2", "123", "小学"));
userMap.put("张三3", new User("张三3", "123", "小学"));
userMap.put("李四1", new User("李四1", "123", "初中"));
userMap.put("李四2", new User("李四2", "123", "初中"));
userMap.put("李四3", new User("李四3", "123", "初中"));
userMap.put("王五1", new User("王五1", "123", "高中"));
userMap.put("王五2", new User("王五2", "123", "高中"));
userMap.put("王五3", new User("王五3", "123", "高中"));
}
User login and operation judgment
According to the information in the table, the password obtained by the user name is compared with the entered password to judge that the login is successful. After successful login, judge whether the user wants to switch, generate questions, or exit according to the length of the input data.
//用户登录
public void userLogin(String username, String password) {
User user = userMap.get(username);
System.out.println("当前选择为" + user.getType() + "出题");
//判断密码是否正确
if (user.getPassword().equals(password)) {
while (true) {
System.out.println("准备生成" + user.getType()
+ "数学题目,请输入生成题目数量(输入-1将退出当前用户,重新登录):");
String inStr = new Scanner(System.in).next();
//当输入的是 切换为XX 时
if (inStr.length() > 4) {
String sub = inStr.substring(0, 3);
if (sub.equals("切换为")) {
setUserType(username, inStr.substring(3, 5));
}
} else {//输入的是数字时
int num = Integer.parseInt(inStr);
if (num == -1) {
break;
} else if (num >= 10 && num <= 30) {
user.makePaper(user, num);
} else {
System.out.println("请输入10-30之间的自然数 或 输入-1退出");
}
}
}
} else {
System.out.println("请输入正确的用户名、密码");
}
}
change user type
There is nothing to say, just pass in the username and the type you want to become.
//用于改变用户的类型
public void setUserType(String username, String type) {
if (type.equals("小学")) {
userMap.get(username).setType(type);
} else if (type.equals("初中")) {
userMap.get(username).setType(type);
} else if (type.equals("高中")) {
userMap.get(username).setType(type);
} else {
System.out.println("请输入小学、初中和高中三个选项中的一个");
}
}
5. Main class-Main
The program will not end after logging out through an infinite loop, but let the user enter the user name and password again. The user name and password are separated by spaces, so the passwords are trimmed.
public class Main {
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
Login login = new Login();
while (true) {
//识别用户名和密码
System.out.println("请输入用户名、密码");
login.userLogin(in.next(), in.nextLine().trim());
}
}
}
To be honest, the overall program logic is still a bit messy, and I want to optimize and adjust it in the future.