Table of contents
1. Basic components
Blank: fill control
Button: button
ButtonType enumeration description
Text: text display
QRCode
2. Common layout
Linear layout (Row and Column)
cascade layout
Elastic Layout (Flex)
1. Basic components
Blank: fill control
This is a new component added by Hongmeng that does not exist in Android development. Its main function is to fill in the blank. In the direction of the main axis of the container, the blank fill component has the ability to automatically fill the empty part of the container. Only takes effect when the parent component is Row/Column.
1. How to use
Blank(min?: number | string).color(color:ResourceColor) |
min: The minimum size of the blank padding component on the main axis of the container. Default value: 0. When the parent component arranges the child components horizontally, if the width of the parent component is not set and the min is not set, the Blank filling display will not be visible. At this time, the min parameter will play a role.
color: fill color
2. Examples
@Entry @Component struct Index {
@State message: string = 'Hello World' build() {
Column() {
Row() {
Text( 'Bluetooth' ).fontSize( 18 ) //最小值 Blank().color(Color.White) Toggle({ type: ToggleType.Switch }) }.width( '100%' ).backgroundColor( 0xFFFFFF ) .borderRadius( 15 ).padding({ left: 12 }) .margin({bottom: 50 }) Row() {
Text( 'Bluetooth' ).fontSize( 18 ) //在父组件没有设置宽度时设置最小值 Blank( 100 ).color(Color.Black) Toggle({ type: ToggleType.Switch }) }.backgroundColor( 0xFFFFFF ).borderRadius( 15 ).padding({ left: 12 }) }.backgroundColor( 0xEFEFEF ).padding( 20 ) } } |
3. Display content
Portrait state
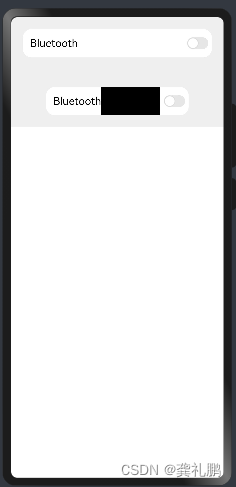
Landscape state
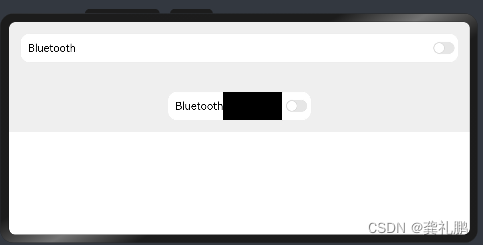
Button component, which can quickly create buttons with different styles.
1. How to use
There are two ways to use it as follows:
Button(options?: {type?: ButtonType, stateEffect?: boolean }) |
type: Describes the display style of the button. Default: ButtonType.Capsule
stateEffect: Whether to enable the press state display effect when the button is pressed. When set to false, the press effect is disabled. Default: true
Note: When the pressed state display effect is turned on, when the developer sets the state style, the color will be superimposed based on the background color after the state style is set .
Button(label?: ResourceStr, options?: { type?: ButtonType, stateEffect?: boolean }) |
label: button text content. Cannot set child component in Button if label is set
Attributes
type: Same as above
stateEffect: Same as above
Capsule: Capsule button (rounded corners default to half the height).
Circle: A circular button.
Normal: Normal buttons (without rounded corners by default).
Example:
// xxx.ets @Entry @Component struct ButtonExample {
build() {
Flex({ direction: FlexDirection.Column, alignItems: ItemAlign.Start, justifyContent: FlexAlign.SpaceBetween }) {
Text( 'Normal button' ).fontSize( 9 ).fontColor( 0xCCCCCC ) Flex({ alignItems: ItemAlign.Center, justifyContent: FlexAlign.SpaceBetween }) {
Button( 'OK' , { type: ButtonType.Normal, stateEffect: true }) .borderRadius( 8 ) .backgroundColor( 0x317aff ) .width( 90 ) .onClick(() => {
console.log( 'ButtonType.Normal' ) }) Button({ type: ButtonType.Normal, stateEffect: true }) {
Row() {
LoadingProgress().width( 20 ).height( 20 ).margin({ left: 12 }).color( 0xFFFFFF ) Text( 'loading' ).fontSize( 12 ).fontColor( 0xffffff ).margin({ left: 5 , right: 12 }) }.alignItems(VerticalAlign.Center) }.borderRadius( 8 ).backgroundColor( 0x317aff ).width( 90 ).height( 40 ) Button( 'Disable' , { type: ButtonType.Normal, stateEffect: false }).opacity( 0.4 ) .borderRadius( 8 ).backgroundColor( 0x317aff ).width( 90 ) } Text( 'Capsule button' ).fontSize( 9 ).fontColor( 0xCCCCCC ) Flex({ alignItems: ItemAlign.Center, justifyContent: FlexAlign.SpaceBetween }) {
Button( 'OK' , { type: ButtonType.Capsule, stateEffect: true }).backgroundColor( 0x317aff ).width( 90 ) Button({ type: ButtonType.Capsule, stateEffect: true }) {
Row() {
LoadingProgress().width( 20 ).height( 20 ).margin({ left: 12 }).color( 0xFFFFFF ) Text( 'loading' ).fontSize( 12 ).fontColor( 0xffffff ).margin({ left: 5 , right: 12 }) }.alignItems(VerticalAlign.Center).width( 90 ).height( 40 ) }.backgroundColor( 0x317aff ) Button( 'Disable' , { type: ButtonType.Capsule, stateEffect: false }).opacity( 0.4 ) .backgroundColor( 0x317aff ).width( 90 ) } Text( 'Circle button' ).fontSize( 9 ).fontColor( 0xCCCCCC ) Flex({ alignItems: ItemAlign.Center, wrap: FlexWrap.Wrap }) {
Button({ type: ButtonType.Circle, stateEffect: true }) {
LoadingProgress().width( 20 ).height( 20 ).color( 0xFFFFFF ) }.width( 55 ).height( 55 ).backgroundColor( 0x317aff ) Button({ type: ButtonType.Circle, stateEffect: true }) {
LoadingProgress().width( 20 ).height( 20 ).color( 0xFFFFFF ) }.width( 55 ).height( 55 ).margin({ left: 20 }).backgroundColor( 0xF55A42 ) } }.height( 400 ).padding({ left: 35 , right: 35 , top: 35 }) } } |
Display style:
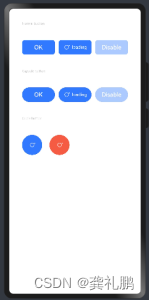
Text: text display
A component that displays a piece of text.
usage
Text(content?: string | Resource)
content: text content. It does not take effect when the subcomponent Span is included, and the content of the Span is displayed, and the style of the text component does not take effect at this time. Defaults:' '
Attributes
name |
Parameter Type |
describe |
textAlign |
TextAlign |
Sets the alignment of text paragraphs in the horizontal direction Default: TextAlign.Start illustrate: 文本段落宽度占满Text组件宽度;可通过align属性控制文本段落在垂直方向上的位置。 从API version 9开始,该接口支持在ArkTS卡片中使用。 |
textOverflow |
{overflow: TextOverflow} |
设置文本超长时的显示方式。 默认值:{overflow: TextOverflow.Clip} 说明: 文本截断是按字截断。例如,英文以单词为最小单位进行截断,若需要以字母为单位进行截断,可在字母间添加零宽空格:\u200B。 需配合maxLines使用,单独设置不生效。 从API version 9开始,该接口支持在ArkTS卡片中使用。 |
maxLines |
number |
设置文本的最大行数。 默认值:Infinity 说明: 默认情况下,文本是自动折行的,如果指定此参数,则文本最多不会超过指定的行。如果有多余的文本,可以通过 textOverflow来指定截断方式。 从API version 9开始,该接口支持在ArkTS卡片中使用。 |
lineHeight |
string | number | Resource |
设置文本的文本行高,设置值不大于0时,不限制文本行高,自适应字体大小,Length为number类型时单位为fp。 从API version 9开始,该接口支持在ArkTS卡片中使用。 |
decoration |
{
type: TextDecorationType, color?: ResourceColor } |
设置文本装饰线样式及其颜色。 默认值:{
type: TextDecorationType.None, color:Color.Black } 从API version 9开始,该接口支持在ArkTS卡片中使用。 |
baselineOffset |
number | string |
设置文本基线的偏移量,默认值0。 从API version 9开始,该接口支持在ArkTS卡片中使用。 说明: 设置该值为百分比时,按默认值显示。 |
letterSpacing |
number | string |
设置文本字符间距。 从API version 9开始,该接口支持在ArkTS卡片中使用。 说明: 设置该值为百分比时,按默认值显示。 |
minFontSize |
number | string | Resource |
设置文本最小显示字号。 需配合maxFontSize以及maxline或布局大小限制使用,单独设置不生效。 从API version 9开始,该接口支持在ArkTS卡片中使用。 |
maxFontSize |
number | string | Resource |
设置文本最大显示字号。 需配合minFontSize以及maxline或布局大小限制使用,单独设置不生效。 从API version 9开始,该接口支持在ArkTS卡片中使用。 |
textCase |
TextCase |
设置文本大小写。 默认值:TextCase.Normal 从API version 9开始,该接口支持在ArkTS卡片中使用。 |
copyOption9+ |
CopyOptions |
组件支持设置文本是否可复制粘贴。 默认值:CopyOptions.None 该接口支持在ArkTS卡片中使用。 |
示例1
textAlign,textOverflow,maxLines,lineHeight使用示例。
// xxx.ets @Entry @Component struct TextExample1 {
build() {
Flex({ direction: FlexDirection.Column, alignItems: ItemAlign.Start, justifyContent: FlexAlign.SpaceBetween }) {
// 文本水平方向对齐方式设置 // 单行文本 Text( 'textAlign' ).fontSize( 9 ).fontColor( 0xCCCCCC ) Text( 'TextAlign set to Center.' ) .textAlign(TextAlign.Center) .fontSize( 12 ) .border({ width: 1 }) .padding( 10 ) .width( '100%' ) Text( 'TextAlign set to Start.' ) .textAlign(TextAlign.Start) .fontSize( 12 ) .border({ width: 1 }) .padding( 10 ) .width( '100%' ) Text( 'TextAlign set to End.' ) .textAlign(TextAlign.End) .fontSize( 12 ) .border({ width: 1 }) .padding( 10 ) .width( '100%' ) // 多行文本 Text( 'This is the text content with textAlign set to Center.' ) .textAlign(TextAlign.Center) .fontSize( 12 ) .border({ width: 1 }) .padding( 10 ) .width( '100%' ) Text( 'This is the text content with textAlign set to Start.' ) .textAlign(TextAlign.Start) .fontSize( 12 ) .border({ width: 1 }) .padding( 10 ) .width( '100%' ) Text( 'This is the text content with textAlign set to End.' ) .textAlign(TextAlign.End) .fontSize( 12 ) .border({ width: 1 }) .padding( 10 ) .width( '100%' ) // 文本超长时显示方式 Text( 'TextOverflow+maxLines' ).fontSize( 9 ).fontColor( 0xCCCCCC ) // 超出maxLines截断内容展示 Text( 'This is the setting of textOverflow to Clip text content This is the setting of textOverflow to None text content. This is the setting of textOverflow to Clip text content This is the setting of textOverflow to None text content.' ) .textOverflow({ overflow: TextOverflow.None }) .maxLines( 1 ) .fontSize( 12 ) .border({ width: 1 }) .padding( 10 ) // 超出maxLines展示省略号 Text( 'This is set textOverflow to Ellipsis text content This is set textOverflow to Ellipsis text content.' .split( '' ) .join( '\u200B' )) .textOverflow({ overflow: TextOverflow.Ellipsis }) .maxLines( 1 ) .fontSize( 12 ) .border({ width: 1 }) .padding( 10 ) Text( 'lineHeight' ).fontSize( 9 ).fontColor( 0xCCCCCC ) Text( 'This is the text with the line height set. This is the text with the line height set.' ) .fontSize( 12 ).border({ width: 1 }).padding( 10 ) Text( 'This is the text with the line height set. This is the text with the line height set.' ) .fontSize( 12 ).border({ width: 1 }).padding( 10 ) .lineHeight( 20 ) }.height( 600 ).width( 350 ).padding({ left: 35 , right: 35 , top: 35 }) } } |
显示内容如下:
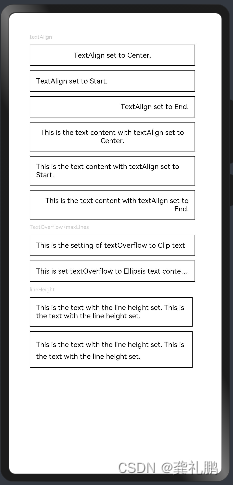
示例2
decoration,baselineOffset,letterSpacing,textCase使用示例:
@Entry @Component struct TextExample2 {
build() {
Flex({ direction: FlexDirection.Column, alignItems: ItemAlign.Start, justifyContent: FlexAlign.SpaceBetween }) {
Text( 'decoration' ).fontSize( 9 ).fontColor( 0xCCCCCC ) Text( 'This is the text content with the decoration set to LineThrough and the color set to Red.' ) .decoration({
type: TextDecorationType.LineThrough, color: Color.Red }) .fontSize( 12 ) .border({ width: 1 }) .padding( 10 ) .width( '100%' ) Text( 'This is the text content with the decoration set to Overline and the color set to Red.' ) .decoration({
type: TextDecorationType.Overline, color: Color.Red }) .fontSize( 12 ) .border({ width: 1 }) .padding( 10 ) .width( '100%' ) Text( 'This is the text content with the decoration set to Underline and the color set to Red.' ) .decoration({
type: TextDecorationType.Underline, color: Color.Red }) .fontSize( 12 ) .border({ width: 1 }) .padding( 10 ) .width( '100%' ) // 文本基线偏移 Text( 'baselineOffset' ).fontSize( 9 ).fontColor( 0xCCCCCC ) Text( 'This is the text content with baselineOffset 0.' ) .baselineOffset( 0 ) .fontSize( 12 ) .border({ width: 1 }) .padding( 10 ) .width( '100%' ) Text( 'This is the text content with baselineOffset 30.' ) .baselineOffset( 30 ) .fontSize( 12 ) .border({ width: 1 }) .padding( 10 ) .width( '100%' ) Text( 'This is the text content with baselineOffset -20.' ) .baselineOffset(- 20 ) .fontSize( 12 ) .border({ width: 1 }) .padding( 10 ) .width( '100%' ) // 文本字符间距 Text( 'letterSpacing' ).fontSize( 9 ).fontColor( 0xCCCCCC ) Text( 'This is the text content with letterSpacing 0.' ) .letterSpacing( 0 ) .fontSize( 12 ) .border({ width: 1 }) .padding( 10 ) .width( '100%' ) Text( 'This is the text content with letterSpacing 3.' ) .letterSpacing( 3 ) .fontSize( 12 ) .border({ width: 1 }) .padding( 10 ) .width( '100%' ) Text( 'This is the text content with letterSpacing -1.' ) .letterSpacing(- 1 ) .fontSize( 12 ) .border({ width: 1 }) .padding( 10 ) .width( '100%' ) Text( 'textCase' ).fontSize( 9 ).fontColor( 0xCCCCCC ) Text( 'This is the text content with textCase set to Normal.' ) .textCase(TextCase.Normal) .fontSize( 12 ) .border({ width: 1 }) .padding( 10 ) .width( '100%' ) // 文本全小写展示 Text( 'This is the text content with textCase set to LowerCase.' ) .textCase(TextCase.LowerCase) .fontSize( 12 ) .border({ width: 1 }) .padding( 10 ) .width( '100%' ) // 文本全大写展示 Text( 'This is the text content with textCase set to UpperCase.' ) .textCase(TextCase.UpperCase) .fontSize( 12 ).border({ width: 1 }).padding( 10 ) }.height( 700 ).width( 350 ).padding({ left: 35 , right: 35 , top: 35 }) } } |
显示内容如下:
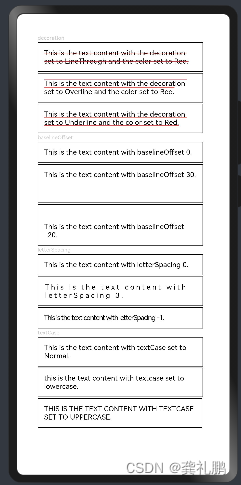
QRCode
用于显示单个二维码的组件。
用法:
QRCode(value: string)
value:传入用于生成二维码的字符串
属性:
color:设置二维码颜色
backgroundColor:设置二维码背景颜色
示例:
// xxx.ets @Entry @Component struct QRCodeExample {
private value: string = 'hello world' build() {
Column({ space: 5 }) {
Text( 'normal' ).fontSize( 9 ).width( '90%' ).fontColor( 0xCCCCCC ).fontSize( 30 ) QRCode( this .value).width( 200 ).height( 200 ) // 设置二维码颜色 Text( 'color' ).fontSize( 9 ).width( '90%' ).fontColor( 0xCCCCCC ).fontSize( 30 ) QRCode( this .value).color( 0xF7CE00 ).width( 200 ).height( 200 ) // 设置二维码背景色 Text( 'backgroundColor' ).fontSize( 9 ).width( '90%' ).fontColor( 0xCCCCCC ).fontSize( 30 ) QRCode( this .value).width( 200 ).height( 200 ).backgroundColor(Color.Orange) }.width( '100%' ).margin({ top: 5 }) } } |
显示如下:
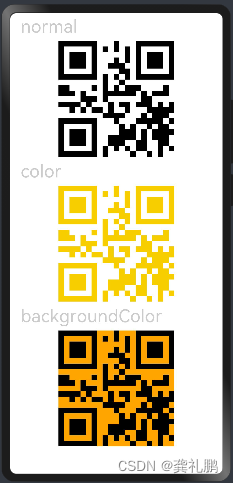
二.常用布局
线性布局(Row和Column)
Row和Colum的线性布局,类似于android的LinearLayout,其中Row是横向的线性布局,Column是竖向的线性布局
参数:
{ space?: string | number; } |
space是item中间的间距
属性:
alignItems(value: HorizontalAlign) justifyContent(value: FlexAlign) |
alignItems:这个是Column中子组件的左中右布局方向,Row同理是子组件的上中下方向
justifyContent:这个是Column中子组件的竖向方向布局,Row中同理是子组件中横向的布局,不过值较多,需要结合图片理解,如下:
示例:
// xxx.ets @Entry @Component struct RowAndColumnTest {
build() {
Column({}) {
Column() {
}.width( '80%' ).height( 50 ).backgroundColor( 0xF5DEB3 ) Column() {
}.width( '80%' ).height( 50 ).backgroundColor( 0xD2B48C ) Column() {
}.width( '80%' ).height( 50 ).backgroundColor( 0xF5DEB3 ) }.width( '100%' ).height( 300 ).backgroundColor( 'rgb(242,242,242)' ).justifyContent(FlexAlign.SpaceEvenly) } } |
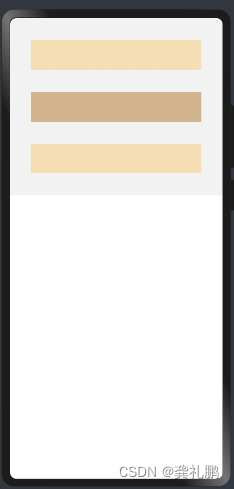
// xxx.ets @Entry @Component struct QRCodeExample {
private value: string = 'hello world' build() {
Column({ space: 5 }) {
Text( 'normal' ).fontSize( 9 ).width( '90%' ).fontColor( 0xCCCCCC ).fontSize( 30 ) QRCode( this .value).width( 200 ).height( 200 ) // 设置二维码颜色 Text( 'color' ).fontSize( 9 ).width( '90%' ).fontColor( 0xCCCCCC ).fontSize( 30 ) QRCode( this .value).color( 0xF7CE00 ).width( 200 ).height( 200 ) // 设置二维码背景色 Text( 'backgroundColor' ).fontSize( 9 ).width( '90%' ).fontColor( 0xCCCCCC ).fontSize( 30 ) QRCode( this .value).width( 200 ).height( 200 ).backgroundColor(Color.Orange) }.width( '100%' ).margin({ top: 5 }).alignItems(HorizontalAlign.End) } } |
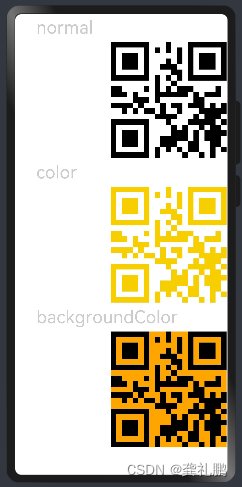
层叠布局
类似于android的帧布局,子组件可以叠加上去,并且可以控制叠加的顺序。
主要参数:
alignContent:对其方式,子控件对齐父控件的位置
zIndex:子组件的叠层排序,zIndex值大的组件会覆盖在zIndex值小的组件上方。
示例:
@Entry @Component struct StackTest {
build() {
Stack({ alignContent: Alignment.BottomStart }) {
Column() {
Text( 'Stack子元素1' ).fontSize( 20 ) }.width( 100 ).height( 100 ).backgroundColor( 0xffd306 ).zIndex( 2 ) Column() {
Text( 'Stack子元素2' ).fontSize( 20 ) }.width( 150 ).height( 150 ).backgroundColor(Color.Pink).zIndex( 1 ) Column() {
Text( 'Stack子元素3' ).fontSize( 20 ) }.width( 200 ).height( 200 ).backgroundColor(Color.Grey) }.margin({ top: 100 }).width( 350 ).height( 350 ).backgroundColor( 0xe0e0e0 ) } } |
显示如下:
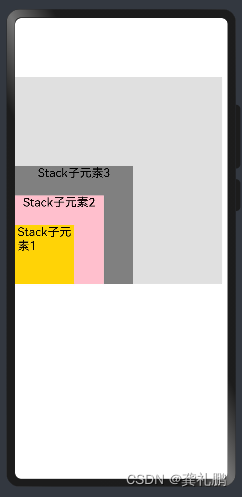
弹性布局(Flex)
Android中没有对应的布局,简单来说就是更加人性化的将子组件放到合适的位置,在设置可以换行的情况下子组件比较多宽度不足的情况下自动将子组件换行到下一行显示
使用方式:
参数名 |
参数类型 |
必填 |
默认值 |
参数描述 |
direction |
FlexDirection |
否 |
FlexDirection.Row |
子组件在Flex容器上排列的方向,即主轴的方向。 |
wrap |
FlexWrap |
否 |
FlexWrap.NoWrap |
Flex容器是单行/列还是多行/列排列。 说明: 在多行布局时,通过交叉轴方向,确认新行堆叠方向。 |
justifyContent |
FlexAlign |
否 |
FlexAlign.Start |
子组件在Flex容器主轴上的对齐格式。 |
alignItems |
ItemAlign |
否 |
ItemAlign.Start |
子组件在Flex容器交叉轴上的对齐格式。 |
alignContent |
FlexAlign |
否 |
FlexAlign.Start |
交叉轴中有额外的空间时,多行内容的对齐方式。仅在wrap为Wrap或WrapReverse下生效。 |
示例:
@Entry @Component struct FlexTest{
build(){
Flex({ justifyContent: FlexAlign.End,wrap :FlexWrap.Wrap }) {
Text( '1' ).width( '40%' ).height( 50 ).backgroundColor( 0xF5DEB3 ) Text( '2' ).width( '40%' ).height( 50 ).backgroundColor( 0xD2B48C ) Text( '3' ).width( '40%' ).height( 50 ).backgroundColor( 0xF5DEB3 ) } .width( '90%' ) .padding({ top: 10 , bottom: 10 }) .backgroundColor( 0xAFEEEE ) } } |
显示如下:
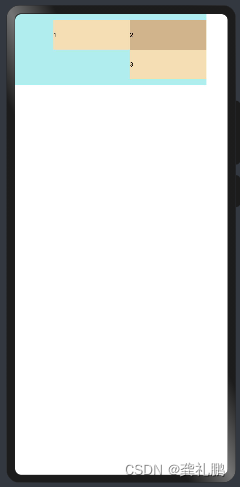