1. Basic introduction
1. SVG 指可伸缩矢量图形 (Scalable Vector Graphics)
2. SVG 用于定义用于网络的基于矢量的图形
3. SVG 使用 XML 格式定义图形
4. SVG 图像在放大或改变尺寸的情况下其图形质量不会有损失
5. SVG 是万维网联盟的标准
- Advantages of SVG, compared to other image formats such as JPEG and GIF, the advantages of using SVG are:
1. SVG 图像可通过文本编辑器来创建和修改
2. SVG 图像可被搜索、索引、脚本化或压缩
3. SVG 是可伸缩的
4. SVG 图像可在任何的分辨率下被高质量地打印
5. SVG 可在图像质量不下降的情况下被放大
Attributes |
describe |
xmlns |
Define the SVG namespace |
version |
Defines the SVG version used |
width |
Sets the width of this SVG document |
height |
Sets the height of this SVG document |
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
</style>
</head>
<body>
<svg xmlns="http://www.csnd.org/2000/svg" version="1.1" height="200" width="200">
<polygon points="100,10 40,180 190,60 10,60 160,180"
style="fill:lime;stroke:purple;stroke-width:5;fill-rule:evenodd;" />
</svg>
</body>
<script>
</script>
</html>
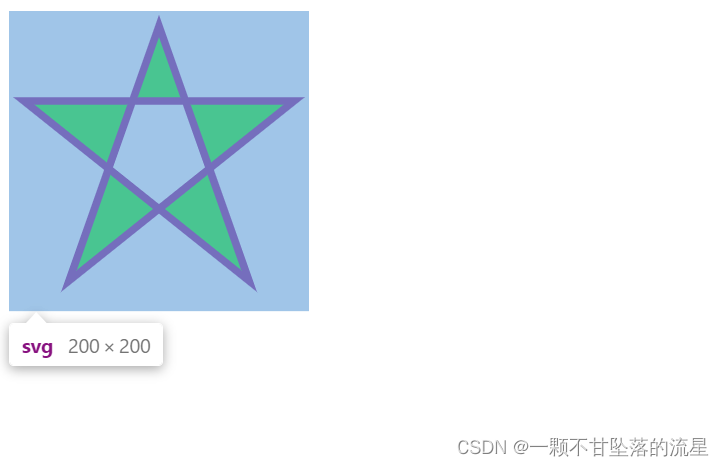
2. Detailed usage
- The style attribute of the graphic
Attributes |
describe |
fill |
Define the fill color of the shape |
stroke-width |
Defines the width of the graphics border |
stroke |
Define the color of the graph border |
fill-opacity |
Define fill color transparency (legal range: 0 - 1) |
stroke-opacity |
Defines the transparency of the stroke color (legal range: 0 - 1) |
opacity |
Defines the transparency value for the entire element |
2.1, rectangle (rect)
<rect>
Labels can be used to create rectangles, and variants of rectangles.
Attributes |
describe |
width |
Define the width of the rectangle |
height |
Define the height of the rectangle |
style |
Define CSS properties |
x |
Define the left position of the rectangle (for example, x="0" defines the distance from the rectangle to the left side of the browser window is 0px) |
y |
Define the top position of the rectangle (for example, y="0" defines that the distance from the rectangle to the top of the browser window is 0px) |
rx and ry |
rounds the corners of a rectangle |
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
svg {
border: 1px greenyellow solid;
}
</style>
</head>
<body>
<svg xmlns="http://www.csnd.org/2000/svg" version="1.1" height="200" width="200">
<rect x="20" y="20" width="100" height="100" rx="20" ry="20"/>
</svg>
</body>
<script>
</script>
</html>
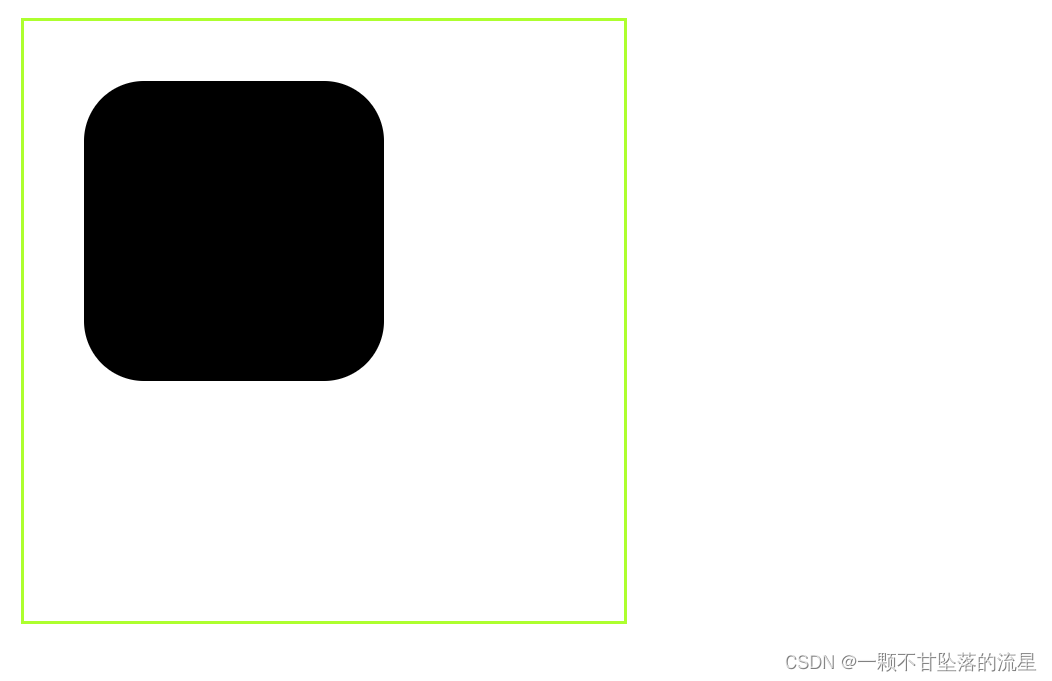
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
svg {
border: 1px greenyellow solid;
}
</style>
</head>
<body>
<svg xmlns="http://www.csnd.org/2000/svg" version="1.1" height="200" width="200">
<rect x="20" y="20" width="100" height="100" rx="20" ry="20"
style="fill:red; stroke:black; stroke-width:5; opacity:0.5"/>
</svg>
</body>
<script>
</script>
</html>
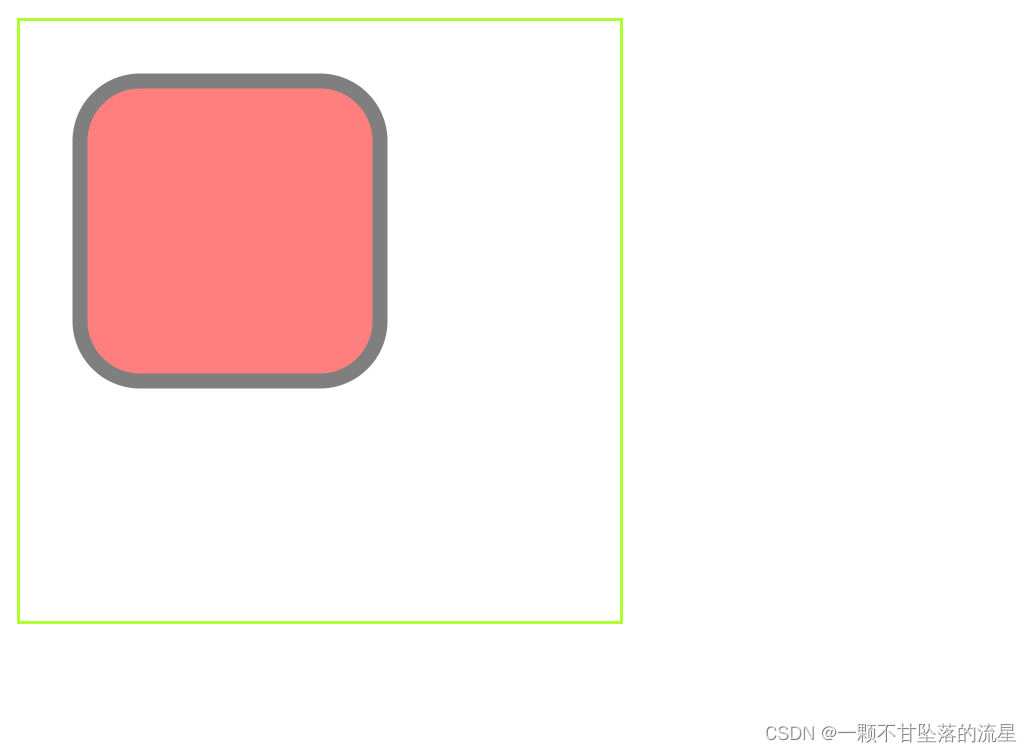
2.2, circle (circle)
<circle>
The label can be used to create a circle (if cx and cy are omitted, the center of the circle will be set to (0, 0) )
Attributes |
describe |
r |
Define the radius of the circle |
cx |
the x-coordinate of the dot |
cy |
the y-coordinate of the dot |
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
svg {
border: 1px greenyellow solid;
}
</style>
</head>
<body>
<svg xmlns="http://www.csnd.org/2000/svg" version="1.1" height="150" width="150">
<circle cx="100" cy="50" r="40"/>
</svg>
</body>
<script>
</script>
</html>
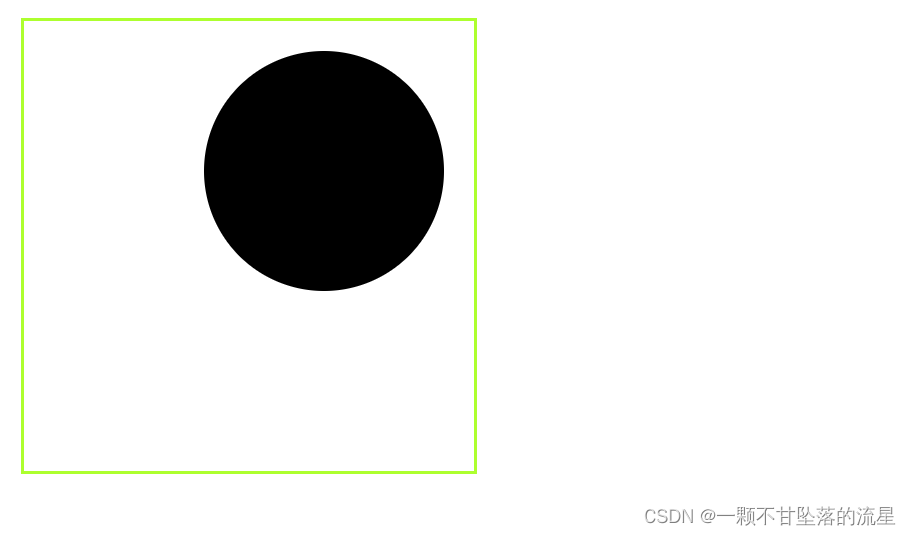
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
svg {
border: 1px greenyellow solid;
}
</style>
</head>
<body>
<svg xmlns="http://www.csnd.org/2000/svg" version="1.1" height="150" width="150">
<circle cx="100" cy="50" r="40" style="fill:red; stroke:black; stroke-width:5; opacity:0.5"/>
</svg>
</body>
<script>
</script>
</html>
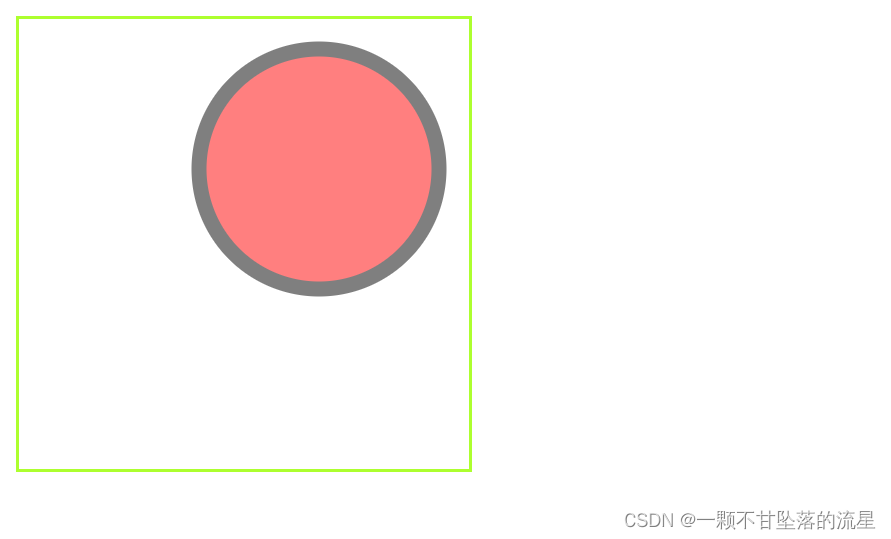
2.3, ellipse (ellipse)
<ellipse>
Labels can be used to create ellipses.
Attributes |
describe |
rx |
Define the horizontal radius |
ry |
define vertical radius |
cx |
the x-coordinate of the dot |
cy |
the y-coordinate of the dot |
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
svg {
border: 1px greenyellow solid;
}
</style>
</head>
<body>
<svg xmlns="http://www.csnd.org/2000/svg" version="1.1" height="150" width="150">
<ellipse cx="75" cy="75" rx="60" ry="30"/>
</svg>
</body>
<script>
</script>
</html>
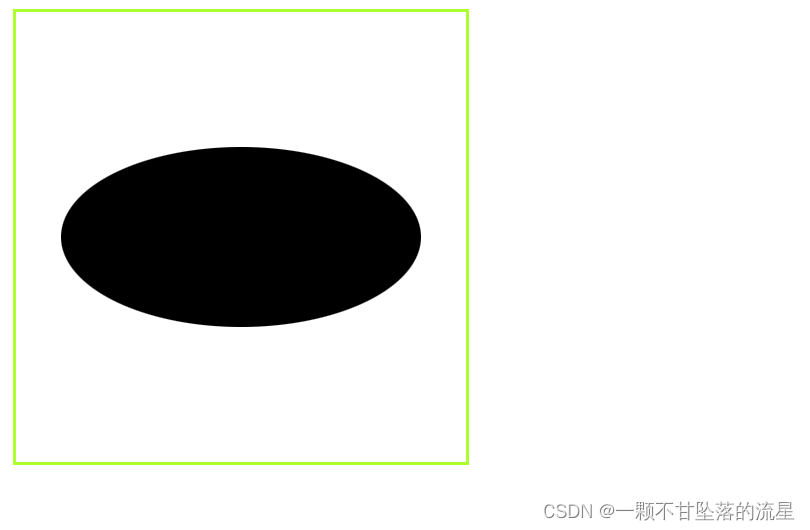
- Use the style attribute: use the same method as above
2.4, line (line)
<line>
Labels are used to create lines.
Attributes |
describe |
x1 |
Defines the start of the line on the x-axis |
y1 |
Defines the start of the line on the y-axis |
x2 |
Defines the end of the line on the x-axis |
y2 |
Defines the end of the line on the y-axis |
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
svg {
border: 1px greenyellow solid;
}
</style>
</head>
<body>
<svg xmlns="http://www.csnd.org/2000/svg" version="1.1" height="150" width="150">
<line x1="0" y1="10" x2="100" y2="150" style="stroke:red;"/>
</svg>
</body>
<script>
</script>
</html>
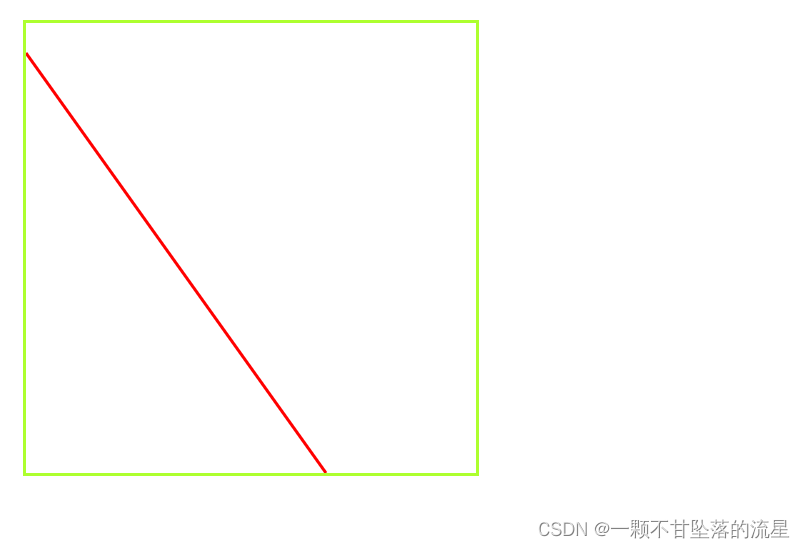
2.5, polyline (polyline)
<polyline>
Labels are used to create shapes that contain only straight lines.
Attributes |
describe |
points |
Defines the x and y coordinates of each vertex of the polyline |
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
svg {
border: 1px greenyellow solid;
}
</style>
</head>
<body>
<svg xmlns="http://www.csnd.org/2000/svg" version="1.1" height="150" width="150">
<polyline points="0,0 0,20 20,20 20,40 40,40 40,60"/>
</svg>
</body>
<script>
</script>
</html>
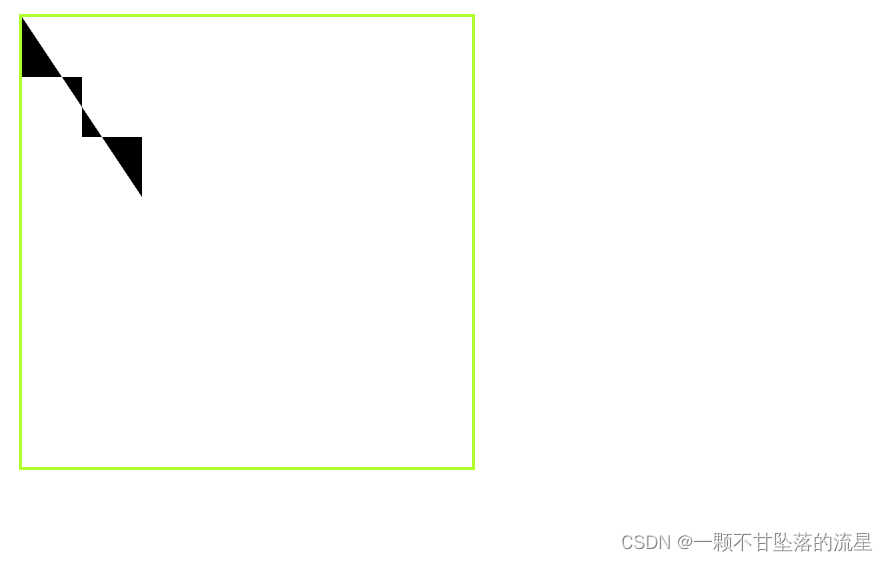
2.6, polygon (polygon)
<polygon>
Labels are used to create graphs with no fewer than three sides.
Attributes |
describe |
points |
define the x and y coordinates of each corner of the polygon |
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
svg {
border: 1px greenyellow solid;
}
</style>
</head>
<body>
<svg xmlns="http://www.csnd.org/2000/svg" version="1.1" height="150" width="150">
<polygon points="22,10 30,21 17,25"/>
</svg>
</body>
<script>
</script>
</html>
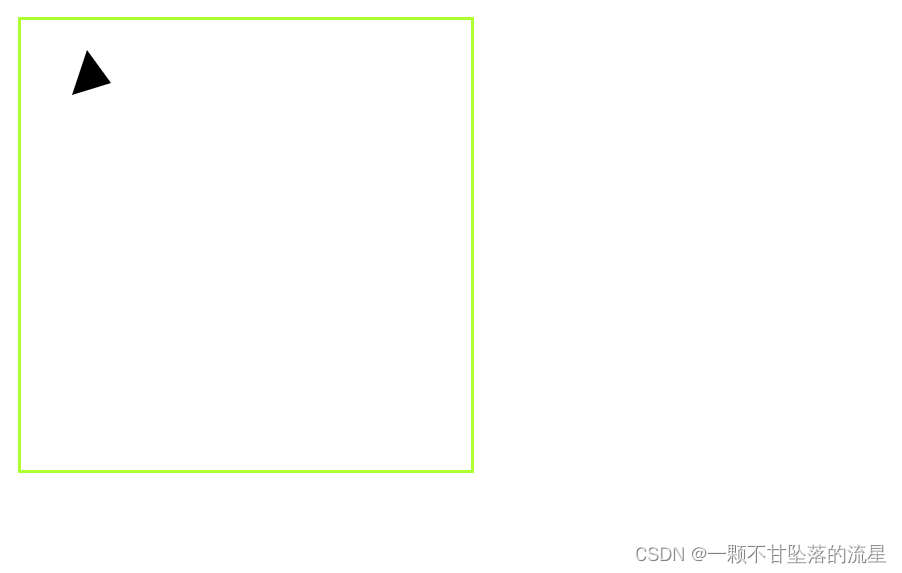
2.7, path (path)
<path>
Labels are used to define paths.
- All the following commands allow lowercase letters. Uppercase means absolute positioning, lowercase means relative positioning.
Order |
describe |
Chinese |
M |
moveto |
move |
L |
lineto |
draw a line |
H |
horizontal lineto |
horizontal line |
V |
vertical lineto |
vertical line |
C |
curveto |
curve |
S |
smooth curveto |
smooth curve |
Q |
quadratic Belzier curve |
quadratic Bezier curve |
T |
smooth quadratic Belzier curveto |
Quadratic Bézier smooth curve |
A |
elliptical Arc |
elliptical arc |
Z |
closepath |
end drawing path |
- A path is defined which starts at position 25 15, goes to position 15 35, then starts from there to 35 35, and finally closes the path at 25 15.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
svg {
border: 1px greenyellow solid;
}
</style>
</head>
<body>
<svg xmlns="http://www.csnd.org/2000/svg" version="1.1" height="150" width="150">
<path d="M25 15 L15 35 L35 35 Z"/>
</svg>
</body>
<script>
</script>
</html>
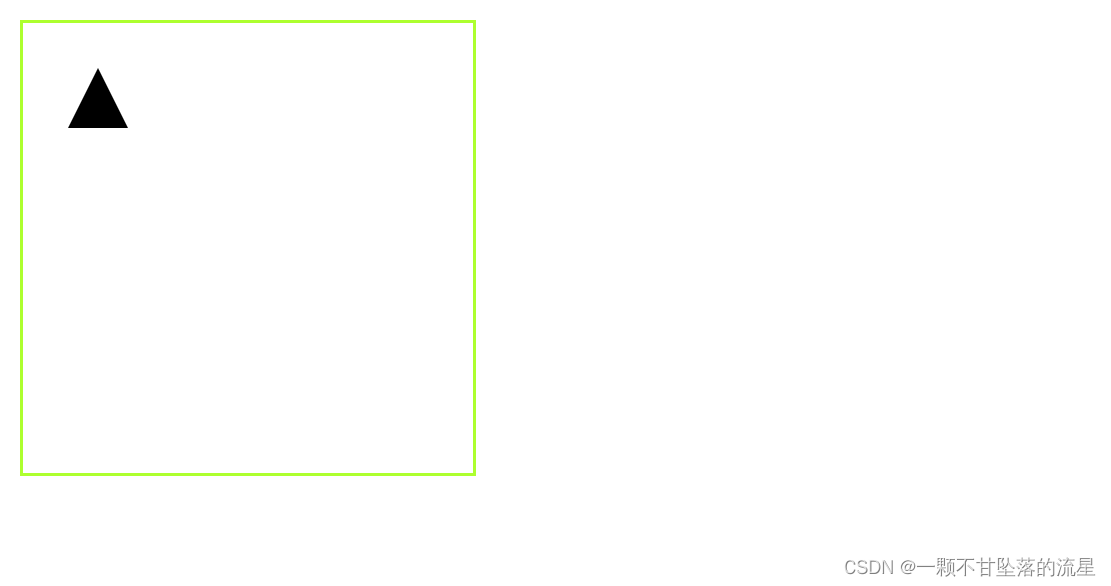
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
svg {
border: 1px greenyellow solid;
}
</style>
</head>
<body>
<svg xmlns="http://www.csnd.org/2000/svg" version="1.1" height="500" width="100%">
<path d="M153 334
C153 334 151 334 151 334
C151 339 153 344 156 344
C164 344 171 339 171 334
C171 322 164 314 156 314
C142 314 131 322 131 334
C131 350 142 364 156 364
C175 364 191 350 191 334
C191 311 175 294 156 294
C131 294 111 311 111 334
C111 361 131 384 156 384
C186 384 211 361 211 334
C211 300 186 274 156 274"/>
</svg>
</body>
<script>
</script>
</html>
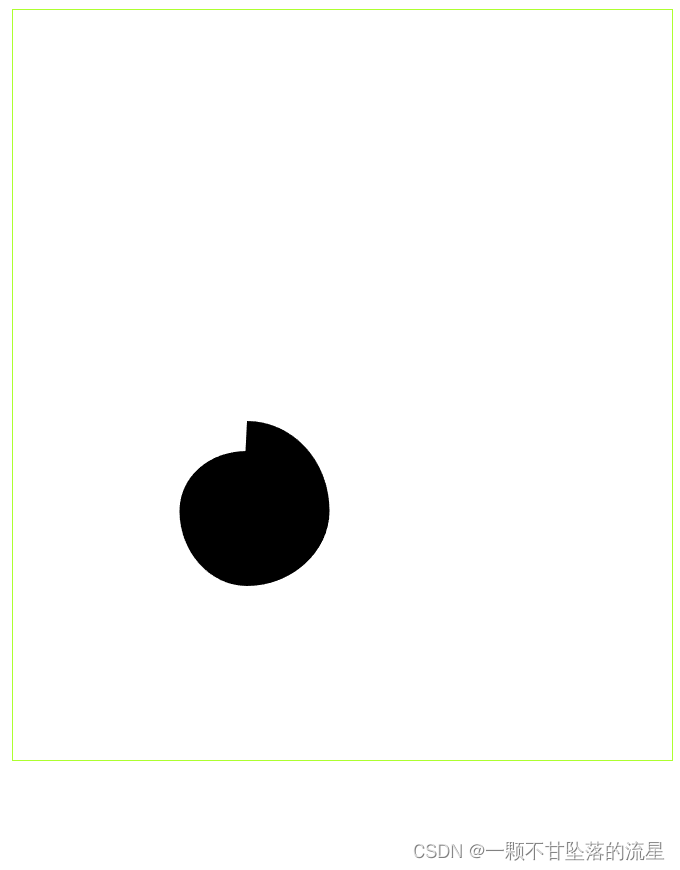