4. Event binding
Use on+event name to bind an event. Note that this is different from native events. Native events are all lowercase onclick, and events in React are hump onClick. React events are not native events, but synthetic events.
Above code:
import React, {
Component } from 'react'
export default class Header extends Component {
render() {
return (
<div>
<div>我是头部</div>
<input type="text" />
{
/* 如果处理逻辑过多,不推荐这种写法 */}
<button onClick={
() => {
console.log(this, "1111") }}>按钮一</button>
{
/* 需要考虑this的指向可以用bind改变this的指向,第一参数必须写this,后面的参数可以进行传参,不推荐这种写法 */}
<button onClick={
this.button1.bind(this,11111)}>按钮二</button>
{
/* 不需要考虑this指向,但是不能进行传参,推荐这种写法 */}
<button onClick={
this.button2}>按钮三</button>
{
/* 这样不用考虑this的指向 也可以进行传参,很推荐这种写法 */}
<button onClick={
() => this.button3(11111)}>按钮四</button>
</div>
)
}
button1(num) {
console.log(this, "22222",num);
}
button2 = () => {
console.log(this, "333");
}
button3(num) {
console.log(this, "44444");
console.log(num);
}
}
Supplement 1: Three ways to change the point of this
call Change this to automatically execute the function
apply Change this to automatically execute the function
bind Change this to manually execute the function Example: handle().bind(this)() will be executed in this way
Supplement 2:
React does not really bind events to any specific
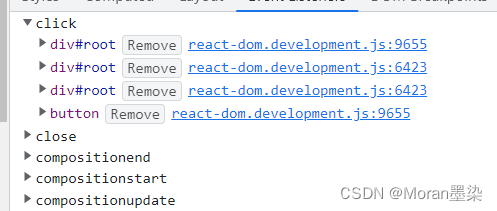
When we remove the event binding on the button, we find that the event will not disappear.
Only after we remove all the events on the div#root will it disappear.
Like common browsers, event handlers will be automatically passed an event object, which contains basically the same methods and properties as common browser event objects. The difference is that the event object in React is not provided by the browser, but built internally by itself. It also has common methods such as event.stopPropagation and event.preventDefault
Five, the use of Ref
1. Set ref="username" to the label, and get this.refs.username through this. The ref can get the real dom of the application.
import React, {
Component } from 'react'
export default class App extends Component {
render() {
return (
<div>
<input type="text" ref="mytext" />
<button onClick={
() => {
console.log(111, this.refs.mytext.value);
}}>button</button>
</div>
)
}
}
But when we use VScode, we will find that there will be a horizontal line on refs, (it can be found that it has been deprecated), but the console does not give us an error.
So add here:
root.render(
<React.StrictMode>
<App />
</React.StrictMode>
)
Turn on the strict mode and you can see the error in the console
Correct way of writing:
import React, {
Component } from 'react'
export default class App extends Component {
myRef = React.createRef()
render() {
return (
<div>
<input type="text" ref={
this.myRef} />
<button onClick={
() => {
console.log(111, this.myRef.current.value);
}}>button</button>
</div>
)
}
}
Not only can you get the dom node, but the console will no longer report errors in strict mode.
6. Application of State
The state is the data that the component describes a certain display situation. It is set and changed by the component itself, that is to say, it is maintained by the component itself. The purpose of using the state is to make the display of the component different in different states (self-management)
import React, {
Component } from 'react'
export default class App extends Component {
render() {
let text = "收藏" //临时变量
return (
<div>
<h1>欢迎来到react开发</h1>
<button onClick={
() => {
console.log(text);
text = "取消收藏"
console.log(text);
}}>{
text}</button>
</div>
)
}
}
//这里两种写法是一样的结果
import React, {
Component } from 'react'
export default class App extends Component {
text = "收藏" //全局变量
render() {
// let text = "收藏"
return (
<div>
<h1>欢迎来到react开发</h1>
<button onClick={
() => {
console.log(this.text);
this.text = "取消收藏"
console.log(this.text);
}}>{
this.text}</button>
</div>
)
}
}
We can see that the text has actually changed but not updated on the dom.
Reason:
this.state is a pure js object. In vue, the data attribute is processed by Object.defineProperty. When the data of data is changed, the data will be triggered getter and setter, but there is no such processing in React. If you change it directly, react will not know it. Therefore, you need to use the special method setState to change the state.
import React, {
Component } from 'react'
export default class App extends Component {
state = {
text: "收藏"
}
render() {
return (
<div>
<h1>欢迎来到react开发</h1>
<button onClick={
() => {
this.setState({
text: '取消收藏'
})
}}>{
this.state.text}</button>
</div>
)
}
}
Here I found that it can only be changed once. If I want to change it every time I click, I need to add a status flag to control it.
The first
import React, {
Component } from 'react'
export default class App extends Component {
state = {
flag: false
}
render() {
return (
<div>
<h1>欢迎来到react开发</h1>
<button onClick={
() => {
this.setState({
flag: !this.state.flag
})
}}>{
this.state.flag ? "取消收藏" : "收藏"}</button>
</div>
)
}
}
the second
import React, {
Component } from 'react'
export default class App extends Component {
constructor() {
super()
this.state = {
text: "收藏",
flag: false
}
}
render() {
return (
<div>
<h1>欢迎来到react开发 --- {
this.state.text}</h1>
<button onClick={
() => {
this.setState({
flag: !this.state.flag,
text: this.state.flag ? "收藏" : "取消收藏"
})
}}>{
this.state.flag ? "取消收藏" : "收藏"}</button>
</div>
)
}
}
Here we need to pay attention to the method of sending the constructor, we need to write super(), we need to inherit from the Component component class, and take the previous properties.
extra
We can use the map method for dom loop rendering when rendering an array list
import React, {
Component } from 'react'
export default class App extends Component {
state = {
list: [111, 2222, 3333, 44444]
}
render() {
return (
<div>
{
this.state.list.map((item, index) =>
<li key={
index}>{
item} --- {
index}</li>
)
}
</div>
)
}
}
//第二种
import React, {
Component } from 'react'
export default class App extends Component {
state = {
list: [111, 2222, 3333, 44444]
}
render() {
let arrlist = this.state.list.map((item, index) =>
<li key={
index}>{
item} --- {
index}</li>
)
return (
<div>
{
arrlist
}
</div>
)
}
}
Note that the key value can be added. If there is no operation such as adding, deleting, and updating the state, the index value can be used, otherwise it must be a unique identifier (id)