1. Problems arise
- When we used the map in Vue, we referenced Baidu's api on the home page but still reported an error. This problem is actually very easy to solve.
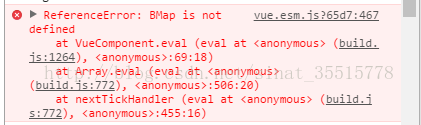
2. Solutions
2.1. Formal methods
- Introduce Baidu's API in the index.html page
/*
* index.html
**/
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>逛吃管理系统</title>
<meta name="viewport"
content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<meta name="description" content="逛吃管理系统">
<meta name="keyword" content="餐饮,娱乐">
<link rel="icon" href="/asserts/images/favicon.ico" />
</head>
<body>
<div id="app"></div>
<script src="bundle.js"></script>
<!--百度地图-秘钥-->
<script type="text/javascript" src="https://api.map.baidu.com/api?v=2.0&ak=mEBI4fumOXV3HdfdfdNnl903dfdfdfdfrPwI6wtX&s=1"></script>
<!--百度地图-加载鼠标绘制工具-->
<script type="text/javascript" src="https://api.map.baidu.com/library/DrawingManager/1.4/src/DrawingManager_min.js"></script>
<link rel="stylesheet" href="https://api.map.baidu.com/library/DrawingManager/1.4/src/DrawingManager_min.css"/>
<!--百度地图-加载检索信息窗口-->
<script type="text/javascript" src="https://api.map.baidu.com/library/SearchInfoWindow/1.4/src/SearchInfoWindow_min.js"></script>
<link rel="stylesheet" href="https://api.map.baidu.com/library/SearchInfoWindow/1.4/src/SearchInfoWindow_min.css"/>
<!--百度UEditor-->
<script type="text/javascript" src="/asserts/js/UEditor/UE/ueditor.config.js"></script>
<script type="text/javascript" src="/asserts/js/UEditor/UE/ueditor.all.min.js"></script>
<script type="text/javascript" src="/asserts/js/UEditor/UE/lang/zh-cn/zh-cn.js"></script>
<script type="text/javascript" src="/asserts/js/UEditor/UE/ueditor.parse.min.js"></script>
</body>
</html>
- Modify the configuration file of webpack
/*
* 增加externals属性,并把百度用到的对象都导出去
**/
externals: {
"BMap": "BMap",
"BMapLib": "BMapLib"
},
- When used in the vue component, you can directly import the BMap object and use it casually.
<template>
<div>
<div id="allmap" style="width: 100%;height:575px;"></div>
<div class="block">
<div class="s_block">
<p>{{title}}</p>
<h3>{{plase}}</h3>
<button @click="btn_add">{{btn}}</button>
</div>
</div>
</div>
</template>
<script>
import BMap from 'BMap'
import BMapLib from 'BMapLib'
export default{
data(){
return {
title: '目前您并没有区块数据',
plase: '请添加区块',
btn: '添加区块',
pointArr:[]
}
},
mounted(){
this.$nextTick(function () {
let _this = this
//在此调用api
let map = new BMap.Map("allmap") // 创建Map实例
map.centerAndZoom(new BMap.Point(116.404, 39.915), 11)
let local = new BMap.LocalSearch(map, {
renderOptions: {map: map, autoViewport: true}
})
//回调获得覆盖物信息
let overlaycomplete = function(e){
let item=[]
let path=e.overlay.getPath()//返回多边形的点数组
for(var i=0;i<path.length;i++){
console.log("lng:"+path[i].lng+"lat:"+path[i].lat)
let obj={}
obj.lng=path[i].lng
obj.lat=path[i].lat
item.push(obj)
}
_this.pointArr.push(item)
console.log(_this.pointArr)
localStorage['mapPoint']=JSON.stringify(_this.pointArr)
}
let BMapPoint1=[]
var polygon = new BMap.Polygon(BMapPoint1, {strokeColor:"#438bd2", strokeWeight:3, strokeOpacity:0.8,strokeStyle: 'dashed'}) //创建多边形
map.addOverlay(polygon) //增加多边形
let styleOptions = {
strokeColor: "#438bd2", //边线颜色。
strokeWeight: 3, //边线的宽度,以像素为单位。
strokeOpacity: 0.8, //边线透明度,取值范围0 - 1。
fillOpacity: 0.6, //填充的透明度,取值范围0 - 1。
strokeStyle: 'dashed' //边线的样式,solid或dashed。
}
//实例化鼠标绘制工具
let drawingManager = new BMapLib.DrawingManager(map, {
isOpen: false, //是否开启绘制模式
enableDrawingTool: true, //是否显示工具栏
drawingToolOptions: {
anchor: BMAP_ANCHOR_TOP_RIGHT, //位置
offset: new BMap.Size(5, 5), //偏离值
},
polygonOptions: styleOptions, //多边形的样式
})
//添加鼠标绘制工具监听事件,用于获取绘制结果
drawingManager.addEventListener('overlaycomplete', overlaycomplete)
})
},
methods: {
btn_add(){
if (this.btn == '添加区块') {
this.title = ''
this.plase = "请保存添加区块"
this.btn = "保存"
} else {
this.title = '目前您并没有区块数据',
this.plase = '请添加区块',
this.btn = '添加区块'
}
}
}
}
</script>
<style>
.BMapLib_marker,.BMapLib_circle,.BMapLib_polyline,.BMapLib_rectangle {
display: none;
}
</style>
<style lang="scss" scoped>
.block {
width: 318px;
height: 100%;
background: #f2f2f2;
position: absolute;
left: 0;
top: 0;
box-shadow: 3px 3px 3px #ccc;
color: #ccc;
.s_block {
width: 318px;
position: absolute;
left: 50%;
top: 50%;
transform: translate3d(-50%, -50%, 0);
text-align: center;
line-height: 30px;
button {
background: #328ff7;
color: #fff;
border: none;
border-radius: 5px;
height: 25px;
cursor: pointer;
}
}
}
</style>
2.2. Informal methods
export function MP(ak) {
return new Promise(function (resolve, reject) {
window.onload = function () {
resolve(BMap)
}
var script = document.createElement("script")
script.type = "text/javascript"
script.src = "http://api.map.baidu.com/api?v=2.0&ak="+ak+"&callback=init"
script.onerror = reject
document.head.appendChild(script)
})
}
在你的百度地图页面中调用(ak 就是你的密钥)
<template>
<div class="welcomeContainer">
欢迎使用B2B后台管理系统,如果有bug,请反馈到技术组。
<div id="allmap"></div>
</div>
</template>
<style scoped lang="scss">
#allmap {
height: 600px;
width: 100%;
}
/*不要嵌套太深*/
/*定义内容区域常量*/
$contentWidth: 1200px;
.homeContainer {
overflow-y: visible;
position: static;
> div {
width: 100%;
}
}
.header {
background-color: limegreen;
height: 60px;
}
.main {
position: absolute;
top: 60px;
bottom: 0;
display: flex;
}
.menu {
width: 200px;
background-color: purple;
float: left;
}
.content {
float: left;
flex: 1;
background-color: yellowgreen;
}
</style>
<script>
import {MP} from './map.js'
export default{
data(){
return {
ak: 'xxxxxxI4fumOXV3HW5xUjNnl903rPwI6wtX'
}
},
mounted(){
this.$nextTick(function () {
var _this = this
MP(_this.ak).then(BMap => {
//在此调用api
var map = new BMap.Map("allmap") // 创建Map实例
map.centerAndZoom(new BMap.Point(116.404, 39.915), 11)
var local = new BMap.LocalSearch(map, {
renderOptions: {map: map, autoViewport: true}
})
local.searchNearby("小吃", "前门")
})
})
}
}
</script>
3. References and citations
4. Special thanks
5. Disclaimer
- Some of the content in this document is taken from many blogs on the Internet, and is only used as a supplement and arrangement of my own knowledge, and is shared with other coders in need, and will not be used for commercial use.
- Because the addresses of many blogs are not saved in time after reading, many of them will not indicate the source here. Thank you very much for your sharing, and I hope everyone understands.
- If the original author feels uncomfortable, you can contact me in time at [email protected], and I will delete the content of the dispute in time
6. Statement of Accountability
- If there is a large paragraph that quotes more than 50% of the full text, please indicate the source of the original text at the end of the document: Longma Xingkong - Shi Guoqing - Jupiter - https://my.oschina.net/u/1416844/blog , otherwise it will be regarded as For plagiarism, legal investigation, please respect your personal intellectual property rights.