topic
去掉字符串中连续出现k 个0 的子串
java code
package com.lizhouwei.chapter5;
/**
* @Description: 去掉字符串中连续出现k 个0 的子串
* @Author: lizhouwei
* @CreateDate: 2018/4/23 21:34
* @Modify by:
* @ModifyDate:
*/
public class Chapter5_3 {
public String removeKZero(String str, int k) {
if (str == null) {
return null;
}
char[] chars = str.toCharArray();
int count = 0;
int start = -1;
for (int i = 0; i < chars.length; i++) {
if (chars[i] == '0') {
count++;
start = start == -1 ? i : start;
} else {
if (count == k) {
while (count-- != 0) {
chars[start++] = ' ';
}
}
count = 0;
start = -1;
}
}
if (count == k) {
while (count-- != 0) {
chars[start++] = ' ';
}
}
return String.valueOf(chars);
}
//测试
public static void main(String[] args) {
Chapter5_3 chapter = new Chapter5_3();
String str = "A0000B000";
System.out.println("A0000B000 去除连续3个0:" + chapter.removeKZero(str, 3));
}
}
result
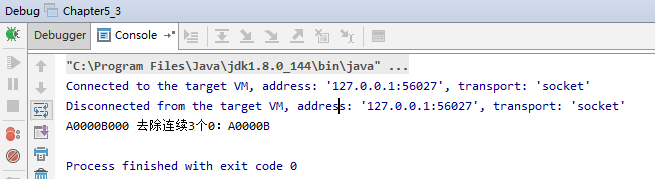