topic
判断字符数组中是否所有的字符都只出现过一次
java code
package com.lizhouwei.chapter5;
/**
* @Description: 判断字符数组中是否所有的字符都只出现过一次
* @Author: lizhouwei
* @CreateDate: 2018/4/24 21:18
* @Modify by:
* @ModifyDate:
*/
public class Chapter5_8 {
public boolean isUnique(char[] chars) {
//堆初始化
for (int i = 0; i < chars.length; i++) {
heapInsert(chars, i);
}
//堆初调整
for (int i = chars.length - 1; i != 0; i--) {
swap(chars, 0, i);
heapify(chars, 0, i);
}
for (int i = 1; i < chars.length; i++) {
if (chars[i] == chars[i - 1]) {
return false;
}
}
return true;
}
public void heapInsert(char[] arr, int index) {
int parent = 0;
while (index > 0) {
parent = (index - 1) / 2;
if (arr[parent] < arr[index]) {
swap(arr, parent, index);
index = parent;
} else {
break;
}
}
}
public void heapify(char[] arr, int index, int heapSize) {
int left = 2 * index + 1;
int right = 2 * index + 2;
int largest = index;
while (left < heapSize) {
if (arr[left] < arr[index]) {
largest = left;
}
if (right < heapSize && arr[right] < arr[left]) {
largest = right;
}
if (largest == index) {
break;
}
swap(arr, index, largest);
index = largest;
left = 2 * index + 1;
right = 2 * index + 2;
}
}
public void swap(char[] arr, int a, int b) {
char temp = arr[b];
arr[b] = arr[a];
arr[a] = temp;
}
//测试
public static void main(String[] args) {
Chapter5_8 chapter = new Chapter5_8();
char[] chars1 = {'a','b','c'};
boolean result1 = chapter.isUnique(chars1);
System.out.println("{'a','b','c'}:中是否有重复字符:" + result1);
char[] chars2= {'1','2','1'};
boolean result2 = chapter.isUnique(chars2);
System.out.println("{'1','2','1'}:中是否有重复字符:" + result2);
}
}
result
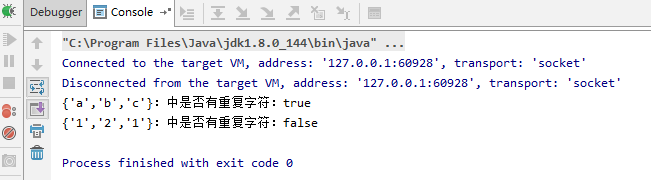