常用的遍历查找算法find、binary_search、adjacent_find、find_if、count、count_if、transform、for_each
一、遍历算法
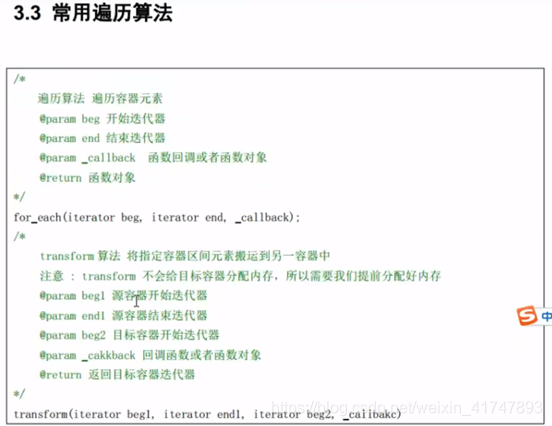
二、查找算法
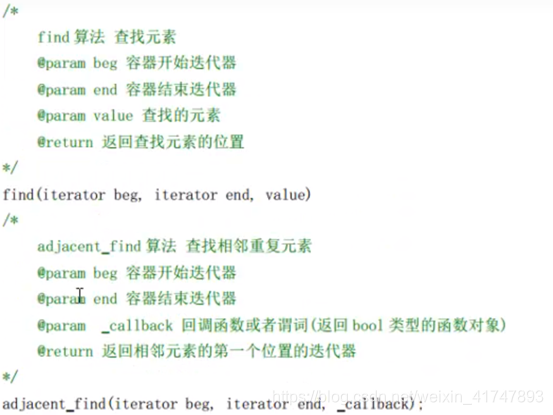
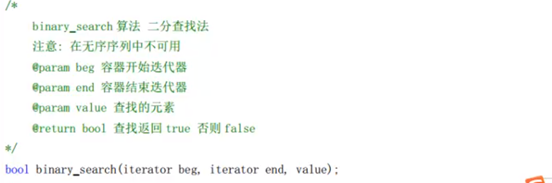

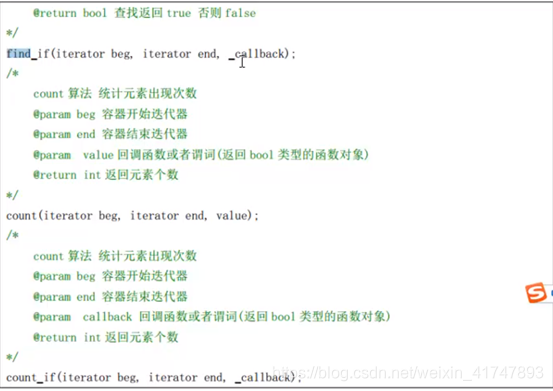
三、案例使用举例:
#include<iostream>
#include<vector>
#include<algorithm>
using namespace std;
struct myplus {
int operator()(int v){
return v;
}
};
void myprint(int v) {
cout << v+100 << " ";
}
void test01() {
vector<int>v1;
vector<int>v2;
for (int i = 0; i < 10; ++i) {
v1.push_back(i);
}
v2.resize(v2.size());
transform(v1.begin(), v1.end(), v2.begin(), myplus());
for_each(v2.begin(), v2.end(), myprint);
}
void test02() {
vector<int>v1;
for (int i = 0; i < 10; ++i) {
v1.push_back(i);
}
vector<int>::iterator ret=find(v1.begin(), v1.end(), 5);
if (ret != v1.end()) {
cout << *ret << endl;
}
else {
cout << "未找到" << endl;
}
}
class Person {
public:
Person(int id,int age):id(id),age(age){}
bool operator==(const Person& p)const {
return p.id == this->id && p.age == this->age;
}
public:
int age;
int id;
};
void test03() {
vector<Person>v1;
Person p1(10, 20), p2(20, 30);
v1.push_back(p1);
v1.push_back(p2);
vector<Person>::iterator ret = find(v1.begin(), v1.end(), p1);
if (ret == v1.end()) {
cout << "未找到" << endl;
}
else {
cout << "找到" << endl;
}
}
bool Mysearch2(int val) {
return val > 5;
}
bool Mysearch(int val) {
return val > 5;
}
void test04() {
vector<int>v1;
for (int i = 0; i < 10; i++) {
v1.push_back(i);
}
bool ret=binary_search(v1.begin(), v1.end(), 5);
if (ret) {
cout << "存在" << endl;
}
else {
cout << "不存在" << endl;
}
vector<int>::iterator it=adjacent_find(v1.begin(), v1.end());
if (it != v1.end()) {
cout << "找到相邻重复元素" <<*it<< endl;
}
else {
cout << "没有找到相邻重复元素" << endl;
}
it=find_if(v1.begin(), v1.end(), Mysearch);
if (it != v1.end()) {
cout << "找到相邻重复元素" << *it << endl;
}
else {
cout << "没有找到相邻重复元素" << endl;
}
int num=count(v1.begin(), v1.end(), 9);
cout << "9出现的个数" << num << endl;
int num = count_if(v1.begin(), v1.end(), Mysearch2);
}
int main(void) {
test04();
return 0;
}