程序代码
#include<stdio.h>
#include<stdlib.h>
#include<string.h>
#define MaxSize 100
typedef struct BTN {
char data;
struct BTN *lchild,*rchild;
}BTN,*BiTNode;
typedef struct {
BiTNode *base;
int top;
int stacksize;
}SqStack;
typedef struct{
int front,rear;
BiTNode *base;
}SqQueue;
void InitStack(SqStack &S){
S.base = (BiTNode*)malloc(MaxSize*sizeof(BiTNode));
if(!S.base) return ;
S.top = 0;
S.stacksize = MaxSize;
}
int StackEmpty(SqStack &S){
if(S.top == 0)
return 1;
return 0;
}
void Push (SqStack &S,BiTNode e){
if(S.top >=S.stacksize){
S.base = (BiTNode*)realloc(S.base,(S.stacksize+10)*sizeof(BiTNode));
if(!S.base) exit(0);
S.stacksize += 10;
}
S.base[S.top++] = e;
}
void Pop(SqStack &S,BiTNode &e){
if(S.top == 0)
exit(0);
else
e = S.base[--S.top];
}
int GetTop(SqStack &S,BiTNode &e){
if(S.top == 0)
return 0;
e = S.base[S.top-1];
return 1;
}
void InitQueue(SqQueue *fq){
fq->front = fq->rear = 0;
fq->base = (BiTNode *)malloc(MaxSize*sizeof(BiTNode));
}
void EnQueue(SqQueue *fq,BiTNode e){
fq->base[fq->rear] = e;
fq->rear = (fq->rear +1)%MaxSize;
}
void DeQueue(SqQueue *fq,BiTNode &p){
if(fq->front == fq->rear)
return ;
p = fq->base[fq->front];
fq->front = (fq->front +1)%MaxSize;
}
int QueueEmpty(SqQueue *fq){
if(fq->front == fq->rear)
return 1;
else
return 0;
}
void visit(BiTNode bt){
printf("%3c",bt->data);
}
void CreatBiTree(BiTNode &bt){
char e;
scanf("%c",&e);
if(e == '#'){
bt = NULL;
}
else{
bt = (BiTNode)malloc(sizeof(BiTNode));
if(!bt) return ;
bt->data = e;
CreatBiTree(bt->lchild);
CreatBiTree(bt->rchild);
}
}
void PreOrderTraverse(BiTNode bt){
if(bt){
visit(bt);
PreOrderTraverse(bt->lchild);
PreOrderTraverse(bt->rchild);
}
}
void InOrderTraverse(BiTNode bt){
if(bt){
InOrderTraverse(bt->lchild);
visit(bt);
InOrderTraverse(bt->rchild);
}
}
void PostOrderTraverse(BiTNode bt){
if(bt){
PostOrderTraverse(bt->lchild);
PostOrderTraverse(bt->rchild);
visit(bt);
}
}
void fPreOrderTraverse(BiTNode bt){
if(bt){
BiTNode p;
SqStack S;
InitStack(S);
Push(S,bt);
while(!StackEmpty(S)){
while(GetTop(S,p)&&p){
visit(p);
Push(S,p->lchild);
}
Pop(S,p);
if(!StackEmpty(S)){
Pop(S,p);
Push(S,p->rchild);
}
}
}
}
void fInOrderTraverse(BiTNode bt){
if(bt){
BiTNode p;
SqStack S;
InitStack(S);
Push(S,bt);
while(!StackEmpty(S)){
while(GetTop(S,p)&&p){
Push(S,p->lchild);
}
Pop(S,p);
if(!StackEmpty(S)){
Pop(S,p);
visit(p);
Push(S,p->rchild);
}
}
}
}
void fPostOrderTraverse(BiTNode bt){
SqStack S;
BiTNode p,q;
if(bt){
InitStack(S);
Push(S,bt);
while(!StackEmpty(S)){
while(GetTop(S,p) && p)
Push(S,p->lchild);
Pop(S,p);
if(!StackEmpty(S)){
GetTop(S,p);
if(p->rchild)
Push(S,p->rchild);
else{
Pop(S,p);
visit(p);
while(!StackEmpty(S) && GetTop(S,q) && q->rchild==p){
Pop(S,p);
visit(p);
}
if(!StackEmpty(S)){
GetTop(S,p);
Push(S,p->rchild);
}
}
}
}
}
}
void LevelOrderTraverse(BiTNode bt){
if(bt){
SqQueue fq;
BiTNode p;
InitQueue(&fq);
EnQueue(&fq,bt);
while(!QueueEmpty(&fq)){
DeQueue(&fq,p);
visit(p);
if(p->lchild) EnQueue(&fq,bt->lchild);
if(p->rchild) EnQueue(&fq,bt->rchild);
}
}
}
static int count = 0;
void LeafNode(BiTNode bt){
if(bt){
LeafNode(bt->lchild);
if(!bt->lchild && !bt->rchild)
count++;
LeafNode(bt->rchild);
}
}
int BiTreeDepth(BiTNode bt){
int depthL,depthR;
if(bt==NULL)
return 0;
else{
depthL=BiTreeDepth(bt->lchild);
depthR=BiTreeDepth(bt->rchild);
if(depthL>=depthR)
return depthL+1;
else return depthR+1;
}
}
int main(){
BiTNode bt;
printf("请输入想要遍历的二叉树(空节点用'#’代替):\n");
CreatBiTree(bt);
printf("递归先序遍历结果:\n");
PreOrderTraverse(bt);
printf("\n递归中遍历结果:\n");
InOrderTraverse(bt);
printf("\n递归后序遍历结果:\n");
PostOrderTraverse(bt);
printf("\n非递归先序遍历结果:\n");
fPreOrderTraverse(bt);
printf("\n非递归中序遍历结果:\n");
fInOrderTraverse(bt);
printf("\n非递归后序遍历结果:\n");
fPostOrderTraverse(bt);
LeafNode(bt);
printf("\n该二叉树叶子节点数为:%d",count);
printf("\n二叉树的深度:%d",BiTreeDepth(bt));
return 0;
}
运行结果
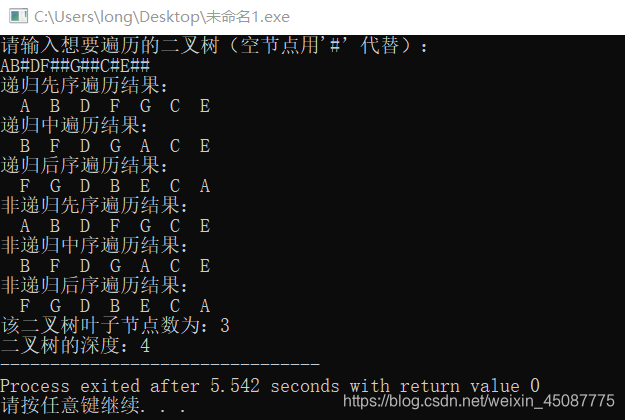