1022. Sum of Root To Leaf Binary Numbers*
https://leetcode.com/problems/sum-of-root-to-leaf-binary-numbers/
题目描述
Given a binary tree, each node has value 0
or 1
. Each root-to-leaf path represents a binary number starting with the most significant bit. For example, if the path is 0 -> 1 -> 1 -> 0 -> 1
, then this could represent 01101
in binary, which is 13
.
For all leaves in the tree, consider the numbers represented by the path from the root to that leaf.
Return the sum of these numbers.
Example 1:
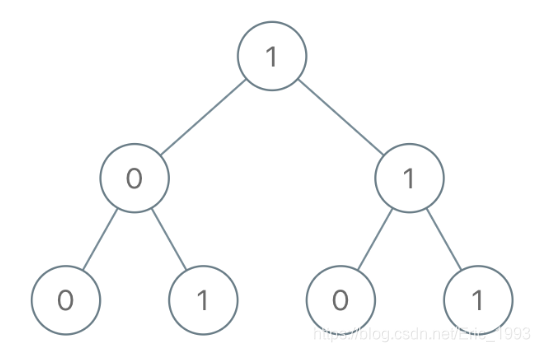
Input: [1,0,1,0,1,0,1]
Output: 22
Explanation: (100) + (101) + (110) + (111) = 4 + 5 + 6 + 7 = 22
Note:
- The number of nodes in the tree is between 1 and 1000.
node.val
is 0 or 1.- The answer will not exceed
2^31 - 1
.
C++ 实现 1
采用 DFS + Backtracing 的思路. 注意在 cur.pop_back()
时, 需要判断左右节点是否为空. 另外, 为了将二进制字符串转换为整数, 可以采用 std::stoi
方法: (参考 C++ STL stoi() function)
int stoi (const string& str, [size_t* idx], [int base]);
中间这个参数设置为 0 即可. 只有当当前节点为叶子节点时, 才需要将当前节点的值加入到 sum
中.
class Solution {
private:
void dfs(TreeNode *root, string &cur, int &sum) {
if (!root) return;
cur += std::to_string(root->val);
if (!root->left && !root->right) {
sum += std::stoi(cur, 0, 2);
return;
}
dfs(root->left, cur, sum);
if (root->left) cur.pop_back();
dfs(root->right, cur, sum);
if (root->right) cur.pop_back();
}
public:
int sumRootToLeaf(TreeNode* root) {
int sum = 0;
string cur;
dfs(root, cur, sum);
return sum;
}
};
C++ 实现 2
来自 LeetCode Submission, 代码比我的简洁. 只有当当前节点为叶子节点时, 才需要将当前节点的值加入到 summation
中.
/**
* Definition for a binary tree node.
* struct TreeNode {
* int val;
* TreeNode *left;
* TreeNode *right;
* TreeNode(int x) : val(x), left(NULL), right(NULL) {}
* };
*/
class Solution {
public:
int sumRootToLeaf(TreeNode* root) {
int cnt = 0;
dfs(root, cnt);
return summation;
}
private:
int summation = 0;
void dfs(TreeNode* root, int cnt)
{
if(!root)
return;
cnt = 2*cnt+root->val;
if(!root->left && !root->right)
summation += cnt;
dfs(root->left, cnt);
dfs(root->right, cnt);
}
};