993. Cousins in Binary Tree*
https://leetcode.com/problems/cousins-in-binary-tree/
题目描述
In a binary tree, the root node is at depth 0
, and children of each depth k
node are at depth k+1
.
Two nodes of a binary tree are cousins if they have the same depth, but have different parents.
We are given the root
of a binary tree with unique values, and the values x
and y
of two different nodes in the tree.
Return true
if and only if the nodes corresponding to the values x
and y
are cousins.
Example 1:
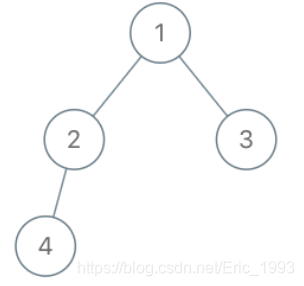
Input: root = [1,2,3,4], x = 4, y = 3
Output: false
Example 2:
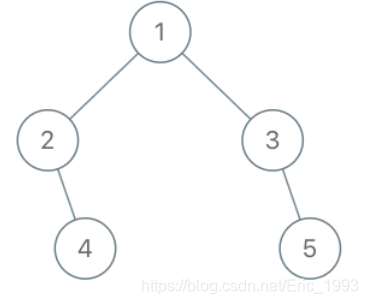
Input: root = [1,2,3,null,4,null,5], x = 5, y = 4
Output: true
Example 3:
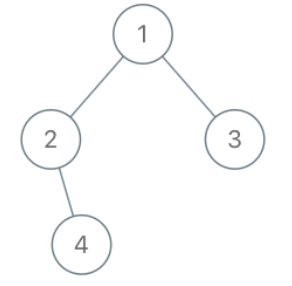
Input: root = [1,2,3,null,4], x = 2, y = 3
Output: false
Note:
- The number of nodes in the tree will be between
2
and100
. - Each node has a unique integer value from
1
to100
.
C++ 实现 1
这道题感觉用层序遍历更为方便. 访问每一层的时候, 需要做如下判断:
- 如果该层只有一个节点, 而且还满足条件, 那么返回 false. 我这里的 “满足条件” 指的是该节点的值刚好等于
x
或者y
. 以下描述中的满足条件都是这个意思; - 如果当前层有多个节点, 对于某个具体的节点
r
如果它的左右孩子满足条件, 当然不是 cousins, 需要返回 false; 那么如何表示某个节点左右孩子满足条件呢? 下面代码中我用num
来判断某个节点r
的两个孩子是否同时满足条件, 如果同时满足, 那么返回 false. 另外使用count
来判断这一层的所有节点是否满足条件, 如果满足, 则返回 true (if (count == 2) return true;
). - 如果当前层的所有节点遍历完, 发现
count == 1
, 此时说明, 就算下一层存在节点满足条件, 也不可能是 cousins 了, 因为两个节点不在同一层, 所以要返回 false.
/**
* Definition for a binary tree node.
* struct TreeNode {
* int val;
* TreeNode *left;
* TreeNode *right;
* TreeNode(int x) : val(x), left(NULL), right(NULL) {}
* };
*/
class Solution {
public:
bool isCousins(TreeNode* root, int x, int y) {
unordered_set<int> record{x, y};
queue<TreeNode*> q;
q.push(root);
while (!q.empty()) {
auto size = q.size();
if (size == 1 && record.count(q.front()->val)) return false;
int count = 0;
while (size --) {
int num = 0; // num 用来记录当前节点的左右孩子是否满足条件
auto r = q.front();
q.pop();
if (r->left) {
q.push(r->left);
if (record.count(r->left->val)) {
count ++;
num ++;
}
}
if (r->right) {
q.push(r->right);
// 同一个节点的左右孩子如果满足条件, 那么就不是 cousins
if (record.count(r->right->val)) {
if (num == 1) return false;
count ++;
}
}
}
// 当前层如果只有一个节点满足条件, 那么当然不是 cousins
if (count == 1) return false;
if (count == 2) return true;
}
return false;
}
};