分析图示:
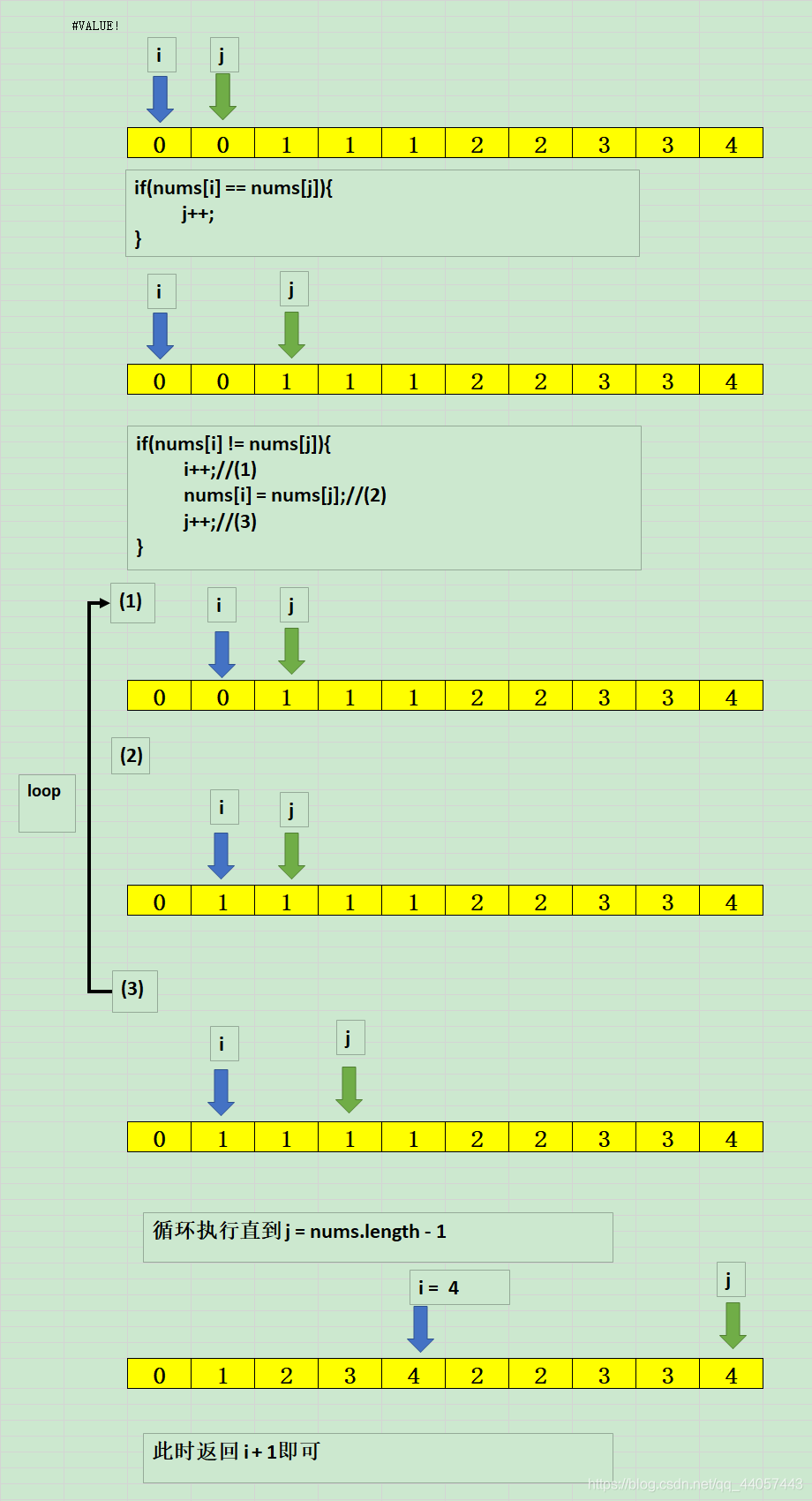
/**
* 给定一个排序数组,你需要在原地删除重复出现的元素,使得每个元素只出现一次,返回移除后数组的新长度。
* 不要使用额外的数组空间,你必须在原地修改输入数组并在使用 O(1) 额外空间的条件下完成。
* @author mtreellen
* @create 2019-10-16-22:48
*/
public class RemoveDuplicates {
public static void main(String[] args) {
int[] arr = new int[]{0,0,1,1,1,2,2,3,3,4};
System.out.println(removeDuplicates(arr));
}
public static int removeDuplicates(int[] nums) {
if(nums == null || nums.length == 1){
return nums.length;
}
int i = 0;
int j = 1;
for(j = 1; j < nums.length;){
if(nums[i] == nums[j]){
j++;
}else{
i++;
nums[i] = nums[j];
j++;
}
}
return i + 1;
}
}