XKC , the captain of the basketball team , is directing a train of nn team members. He makes all members stand in a row , and numbers them 1 \cdots n1⋯n from left to right.
The ability of the ii-th person is w_iwi , and if there is a guy whose ability is not less than w_i+mwi+m stands on his right , he will become angry. It means that the jj-th person will make the ii-th person angry if j>ij>i and w_j \ge w_i+mwj≥wi+m.
We define the anger of the ii-th person as the number of people between him and the person , who makes him angry and the distance from him is the longest in those people. If there is no one who makes him angry , his anger is -1−1 .
Please calculate the anger of every team member .
Input
The first line contains two integers nn and m(2\leq n\leq 5*10^5, 0\leq m \leq 10^9)m(2≤n≤5∗105,0≤m≤109) .
The following line contain nn integers w_1..w_n(0\leq w_i \leq 10^9)w1..wn(0≤wi≤109) .
Output
A row of nn integers separated by spaces , representing the anger of every member .
样例输入
6 1 3 4 5 6 2 10
样例输出
4 3 2 1 0 -1
这道题目就是区间查找大于等于某个数的最靠右的位置。
线段树查询,大区间里找小区间。
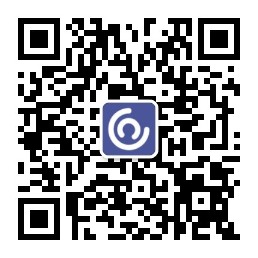
两个版本的板子, 比赛的时候,l和r写反了,一直没过。。。
参考模板来源:
关于如何用线段树实现查找区间内第一个小于(大于)某一值x的方法
代码1:
1 #include<iostream> 2 #include<cstdio> 3 #include<cstring> 4 #include<algorithm> 5 #include<bitset> 6 #include<cassert> 7 #include<cctype> 8 #include<cmath> 9 #include<cstdlib> 10 #include<ctime> 11 #include<deque> 12 #include<iomanip> 13 #include<list> 14 #include<map> 15 #include<queue> 16 #include<set> 17 #include<stack> 18 #include<vector> 19 using namespace std; 20 typedef long long ll; 21 22 const double PI=acos(-1.0); 23 const double eps=1e-6; 24 const ll mod=1e9+7; 25 const int inf=0x3f3f3f3f; 26 const int maxn=5e5+10; 27 const int maxm=100+10; 28 #define ios ios::sync_with_stdio(false);cin.tie(0);cout.tie(0); 29 #define lson l,m,rt<<1 30 #define rson m+1,r,rt<<1|1 31 32 ll tree[maxn<<2]; 33 34 void pushup(int rt) 35 { 36 tree[rt]=max(tree[rt<<1],tree[rt<<1|1]); 37 } 38 39 void update(int p,ll val,int l,int r,int rt) 40 { 41 if(l==r){ 42 tree[rt]=val; 43 return ; 44 } 45 46 int m=(l+r)>>1; 47 if(p<=m) update(p,val,lson); 48 else update(p,val,rson); 49 pushup(rt); 50 } 51 52 /* 53 int get(ll val,int l,int r,int rt) 54 { 55 if(l==r){ 56 return l; 57 } 58 59 int m=(l+r)>>1; 60 if(tree[rt<<1|1]>=val) return get(val,rson); 61 if(tree[rt<<1] > val) return get(val,lson); 62 } 63 */ 64 65 /* 66 int query(int L,int R,ll val,int l,int r,int rt) 67 { 68 if(L>r||R<l){ 69 return -1; 70 } 71 // if(l==r){ 72 // if(tree[rt]>=val){ 73 // return l; 74 // } 75 // else{ 76 // return -1; 77 // } 78 // } 79 if(L<=l&&r<=R){ 80 if(tree[rt]<val){ 81 return -1; 82 } 83 else{ 84 return get(val,l,r,rt); 85 } 86 } 87 88 int m=(l+r)>>1; 89 int ret=query(L,R,val,rson); 90 if(ret!=-1){ 91 return ret; 92 } 93 return query(L,R,val,lson); 94 } 95 */ 96 97 int query(int L,int R,ll val,int l,int r,int rt) 98 { 99 if(L>r||R<l){ 100 return -1; 101 } 102 if(l==r){ 103 if(tree[rt]>=val){ 104 return l; 105 } 106 else{ 107 return -1; 108 } 109 } 110 if(L<=l&&r<=R){ 111 if(tree[rt]<val){ 112 return -1; 113 } 114 // else{ 115 // return get(val,l,r,rt); 116 // } 117 } 118 119 int m=(l+r)>>1; 120 int ret=query(L,R,val,rson); 121 if(ret!=-1){ 122 return ret; 123 } 124 return query(L,R,val,lson); 125 } 126 127 ll a[maxn]; 128 int ans[maxn]; 129 130 int main() 131 { 132 int n; 133 ll m; 134 scanf("%d%lld",&n,&m); 135 for(int i=1;i<=n;i++){ 136 scanf("%lld",&a[i]); 137 update(i,a[i],1,n,1); 138 } 139 for(int i=1;i<=n;i++){ 140 int pos=query(i,n,a[i]+m,1,n,1); 141 // cout<<pos<<endl; 142 if(pos!=-1) ans[i]=pos-i-1; 143 else ans[i]=-1; 144 } 145 for(int i=1;i<n;i++){ 146 printf("%d ",ans[i]); 147 } 148 printf("%d\n",ans[n]); 149 }
代码2:
1 #include<iostream> 2 #include<cstdio> 3 #include<cstring> 4 #include<algorithm> 5 #include<bitset> 6 #include<cassert> 7 #include<cctype> 8 #include<cmath> 9 #include<cstdlib> 10 #include<ctime> 11 #include<deque> 12 #include<iomanip> 13 #include<list> 14 #include<map> 15 #include<queue> 16 #include<set> 17 #include<stack> 18 #include<vector> 19 using namespace std; 20 typedef long long ll; 21 22 const double PI=acos(-1.0); 23 const double eps=1e-6; 24 const ll mod=1e9+7; 25 const int inf=0x3f3f3f3f; 26 const int maxn=5e5+10; 27 const int maxm=100+10; 28 #define ios ios::sync_with_stdio(false);cin.tie(0);cout.tie(0); 29 #define lson l,m,rt<<1 30 #define rson m+1,r,rt<<1|1 31 32 ll tree[maxn<<2]; 33 34 void pushup(int rt) 35 { 36 tree[rt]=max(tree[rt<<1],tree[rt<<1|1]); 37 } 38 39 void update(int p,ll val,int l,int r,int rt) 40 { 41 if(l==r){ 42 tree[rt]=val; 43 return ; 44 } 45 46 int m=(l+r)>>1; 47 if(p<=m) update(p,val,lson); 48 else update(p,val,rson); 49 pushup(rt); 50 } 51 52 int get(ll val,int l,int r,int rt) 53 { 54 if(l==r){ 55 return l; 56 } 57 58 int m=(l+r)>>1; 59 if(tree[rt<<1|1]>=val) return get(val,rson); 60 if(tree[rt<<1] > val) return get(val,lson); 61 } 62 63 int query(int L,int R,ll val,int l,int r,int rt) 64 { 65 if(L>r||R<l){ 66 return -1; 67 } 68 // if(l==r){ 69 // if(tree[rt]>=val){ 70 // return l; 71 // } 72 // else{ 73 // return -1; 74 // } 75 // } 76 if(L<=l&&r<=R){ 77 if(tree[rt]<val){ 78 return -1; 79 } 80 else{ 81 return get(val,l,r,rt); 82 } 83 } 84 85 int m=(l+r)>>1; 86 int ret=query(L,R,val,rson); 87 if(ret!=-1){ 88 return ret; 89 } 90 return query(L,R,val,lson); 91 } 92 93 ll a[maxn]; 94 int ans[maxn]; 95 96 int main() 97 { 98 int n; 99 ll m; 100 scanf("%d%lld",&n,&m); 101 for(int i=1;i<=n;i++){ 102 scanf("%lld",&a[i]); 103 update(i,a[i],1,n,1); 104 } 105 for(int i=1;i<=n;i++){ 106 int pos=query(i,n,a[i]+m,1,n,1); 107 // cout<<pos<<endl; 108 if(pos!=-1) ans[i]=pos-i-1; 109 else ans[i]=-1; 110 } 111 for(int i=1;i<n;i++){ 112 printf("%d ",ans[i]); 113 } 114 printf("%d\n",ans[n]); 115 }