异常
异常是指在程序的运行过程中所发生的不正常的事件,它会中断正在运行的程序
JAVA中的异常处理
异常处理的五个关键字
- try
- catch
- finally
- throw
- throws
异常对象常用的方法
常见的异常类型
多重catch块
排列catch 语句的顺序:先子类后父类
发生异常时按顺序逐个匹配
只执行第一个与异常类型匹配的catch语句
catch (InputMismatchException e) {//子类写在前面 System.out.println("输入整数"); e.printStackTrace(); }catch (Exception e) {//父类写在后面 System.out.println("输入错误"); e.printStackTrace(); } finally{ System.out.println("欢迎提出建议");//无论程序有没有异常都输出 }
throw与throws
异常处理原则
异常处理与性能
异常只能用于非正常情况
不要将过于庞大的代码块放在try中
在catch中指定具体的异常类型
需要对捕获的异常做处理
异常结构图
异常链
//新建两个自定义Exception类 public class FkException extends Exception{//第一个自定义付款类 //重写Exception里的两个方法 public FkException(String message, Throwable cause) { super(message, cause); // TODO Auto-generated constructor stub } public FkException(String message) { super(message); // TODO Auto-generated constructor stub } } //第二个自定义购物车类 class GwException extends Exception{ public GwException(String message, Throwable cause) { super(message, cause); // TODO Auto-generated constructor stub } public GwException(String message) { super(message); // TODO Auto-generated constructor stub } }
//新建一个购物车的类方便传参数 public class Gwc { private String shangpin; private int money; public String getShangpin() { return shangpin; } public void setShangpin(String shangpin) { this.shangpin = shangpin; } public int getMoney() { return money; } public void setMoney(int money) { this.money = money; } }
//新建一个购物车的方法把建好的Gwc类当参数放进去 public static void gwc(Gwc gwcs) throws GwException { if(gwcs==null){ throw new GwException("购物车不能为空");//抛出异常 }else{ System.out.println("添加成功"); } }
//在新建一个付款类继续抛出一个异常
public static void fukuan(Gwc gwcs) throws FkException{
try {
gwc(gwcs);
} catch (GwException e) {
e.printStackTrace();
throw new FkException("付款不能为0");
}
}
//我们去main方法里去调用写的方法 public static void main(String[] args) { Gwxt s=new Gwxt(); try { s.fukuan(null); } catch (Exception e) { e.printStackTrace(); }
}
//下图则是控制台打印出来的结果,可以看到并没有关联起来,我们可以修改一下
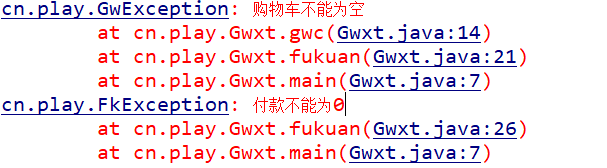
public static void fukuan(Gwc gwcs) throws FkException{ try { gwc(gwcs); } catch (GwException e) { e.printStackTrace(); throw new FkException("付款不能为0",e); //修改之后的代码,把e添加到抛出的异常里 } }
//如下就是我们想要的结果了
小提示
printStackTrace();打印异常堆栈信息
exit(1) 退出虚拟机
try-catch块中存在return语句,是否还执行finally块? 如果执行,说出执行顺序?
先执行finally然后再回去执行return
try-catch- finally块中, finally块唯一不执行的情况是什么?
exit(1)
try-catch-finally结构中try语句块是必须的,catch、finally语句块均可选,但两者至少出现之一