触摸屏幕动作捕捉
触摸屏幕动作的捕捉基于一个载体,可以是某一张图片,某一个控件,某一个布局。
在Activity中通过findViewById或其他途径获取一个View,通过setOnTouchListener对其进行动作捕捉。捕捉动作就三个,按下动作,移动动作和抬起动作。在onTouch中可以使用even.getAction()获取当前动作的类型,对不同类型进行不同的处理。
View.setOnTouchListener(new View.OnTouchListener() {
@Override
public boolean onTouch(View v, MotionEvent event) {
switch (event.getAction()) {
case MotionEvent.ACTION_DOWN:
//do something
case MotionEvent.ACTION_MOVE:
//do something
case MotionEvent.ACTION_UP:
//do something
}
}
反射获取资源及动态调整图片尺寸
在Activity中获取资源、使用资源时,如getResource(),ContextCompat.getDrawable(),setBackgroundResource()等等方法,他们所需要的都是资源的ID。
这时候我们通常会知道资源的名称,可以通过名称去获得其ID,再使用ID进行接下来的操作。
getResources().getIdentifier(name,type,package);
name为资源的名称,即文件名。type为资源类型,如drawable,raw,mipmap等等,package 在大多数情况下就使用getPackageName()进行获取当前应用的包就可以。
获取到的资源在不同机器上运行时,需要根据不同的屏幕大小进行尺寸调整。下面方法可以获得当前运行设备的屏幕尺寸,根据尺寸和图片的固定尺寸,便可以确定我们需要将图片拉伸或缩小多少比例,以此来确保显示在各设备上均可正常显示。
widthPixels = this.getResources().getDisplayMetrics().widthPixels;
heightPixels = this.getResources().getDisplayMetrics().heightPixels;
scaleWidth = (float) widthPixels / 720;
scaleHeight = (float) heightPixels / 1280;
动态调整尺寸在通过屏幕动作捕获时非常关键,捕获屏幕往往获取的是当前的坐标,坐标是以本设备为基准的,所以我们在处理时以比例来进行数据的处理判断,才可以保证程序在不同机型上具有通用性,否则就可能导致程序的异常。
简单的动画演示
简单的动画演示就是连续切换显示的图片,切换的间隔时间较短,达到动画的效果。
创建演示动画可以在Layout中进行定义也可以在Activity中动态定义。
①layout中定义动画
在RES目录下新建目录 anim (名称不可变,固定名称)。然后在anim 下新建animation resource file
使用<item>标签在<set>标签中添加动画的每一帧,android:drawable属性指定每一帧展示的图片,android:duration展示每一帧间隔的时间。
<?xml version="1.0" encoding="utf-8"?>
<set>
android:oneshot="false"
xmlns:android="http://schemas.android.com/apk/res/android">
<item android:drawable="@drawable/pic1" android:duration="150"/>
</set>
在Activity中使用AnimationDrawable类进行动画的播放,播放载体依赖主布局中的一个ImageView。需要将动画设置为ImageView的背景资源。
ImageView.setBackgroundResource(R.anim.XXX);
AnimationDrawable animation = (AnimationDrawable) ImageView.getBackground();
ImageView.post(new Runnable(){
@Override
public void run(){
animation.start();
}
})
②Activity中定义动画
实例化一个AnimationDrawable类,使用animation.addFrame(drawable,duration);方法为动画动态添加帧。
使用animation.setOnshot(boolean)设置是否循环播放。
之后就和上面相同,将其设置为ImageView的背景,在ImageView.poset()方法中设置动画开始播放。
和音乐播放器一样,动画也可以获取当前播放位置(帧位置),播放总时长,使用stop(),start()进行播放,停止等操作。
提示信息
在开发软件,经常要给用户反馈一些信息,如提示用户的错误操作,程序出现了异常等等。
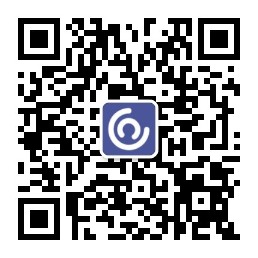
提示方法1:
Toast.makeText(context, message, duration).show();
toast提示非常好用,会在手机上出现一个不影响程序的小提示框,用户无法对其操作,类似于一个提示、通知信息,仅具有告知作用。
context为上下文,使用当前Activity获取应用的context都可以,message是提示信息内容,duration是提示信息存在的时间,长时间Toast.LONG和短时间 Toast.SHORT。
提示方法2:
Dialog类
官方给出的有
AlertDialog,CharacterPickerDialog,Presentation三种直接继承了Dialog的类,另有继承了AlertDialog三个类 DatePickerDiaglog,ProggressDialog,TimePickerDialog.
最简单的AlertDialog弹框提示,类似于JAVAWEB中的ALERT。
AlertDialog.Builder builder = new AlertDialog.Builder(上下文);
builder.setMessage("提示信息");
builder.setTitle("弹框标题");
builder.setPositiveButton("按钮名称1", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
dialog.dismiss();
//点击后的处理时间
}
});
builder.setNegativeButton("按钮名称2", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
dialog.dismiss();
//点击后的处理时间
}
});
builder.create().show();
参考:https://www.cnblogs.com/gzdaijie/p/5222191.html
其中还列举了一些常用的dialog
然而dialog的功能不止于此,包括但不限于带图片,带动画,带音乐、进度条等等等各种你能想到的自定义方式。
基于AlertDialog类,我们可以自定义各种我们想要的弹出框。
以本需求中的演示按钮,弹出动画为例。可以看出弹出框就是一个界面,在界面上我们可以像设计其他任何页面一样去设计这个弹出页面,实现各种功能。
比较常用的进度条,就用相同的布局就可以,开启一个子线程不断请求当前处理状态,每次请求到状态后去动态更新布局中的控件参数。
文字控件中设置最新的处理状态组成提示信息
图片控件设置底图不变,覆盖一张根据当前处理进度百分比调整宽度的图片,就能实现进度条向前移动的效果。
弹框演示动画源码如下:
----------------弹出框类----------------------------
public class AnimationDialog extends AlertDialog {
private AnimationDrawable animation;
private String loadingTip;
//首先定义构造方法,实例化弹出框需要传入上下文,弹出框的提示信息和弹出框内嵌入的动画效果
public AnimationDialog(Context context, String loadingTip, AnimationDrawable animation) {
super(context);
this.loadingTip = loadingTip;
this.animation = animation;
//AlertDialog自带的方法,设置点击边缘时取消弹出框。
setCanceledOnTouchOutside(true);
}
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
//设置弹出框内的显示布局文件
setContentView(R.layout.progress_dialog);
//获取布局文件中的文字框并设置传入的提示文字
TextView loadingTv = (TextView) findViewById(R.id.loadingTv);
loadingTv.setText(loadingTip);
//获取布局文件中的图片框,与传入的动画绑定并开始播放
ImageView loadingIv = (ImageView) findViewById(R.id.loadingIv);
loadingIv.setBackground(animation);
loadingIv.post(new Runnable() {
@Override
public void run() {
animation.start();
}
});
}
}
------------弹出框布局文件----------------------------
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#333333">
<ImageView
android:id="@+id/loadingIv"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerHorizontal="true" />
<TextView
android:id="@+id/loadingTv"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/loadingIv"
android:layout_centerHorizontal="true"
android:textColor="#FFFFFF"
android:textSize="10sp" />
</RelativeLayout>