版权声明:版权声明:本文为博主原创文章,转载请附上博文链接! https://blog.csdn.net/weixin_43851310/article/details/87796116
JSON处理
JSON(JavaScript Object Notation) 是一种轻量级的数据交换格式
Json在线解析及格式化网站:https://www.json.cn/
encoding/json包
func Marshal(v interface{}) ([]byte, error)
Marshal函数返回v的json编码。func MarshalIndent(v interface{}, prefix, indent string) ([]byte, error)
MarshalIndent类似Marshal但会使用缩进将输出格式化。
通过结构体生成json:
import (
"encoding/json"
"fmt"
)
//成员变量必须首字母大写
type Student struct {
Name string `json:"-"` //此字段不会输出到屏幕
Subjects []string `json:"subject"` //二次编码
IsOk bool `json:",string"` //输出为字符串
Price float64
}
func main() {
s := Student{"tom", []string{"Go", "C++", "Python"}, true, 3.14}
//编码,根据内容生成json文件
//buf, err := json.Marshal(s)
buf, err := json.MarshalIndent(s, " ", " ")
//格式化编码,第二个参数为空格,第三个参数为tab
if err != nil {
fmt.Println("err =", err)
return
}
fmt.Println("buf =", string(buf))
}
Output :
buf = {
"subject": [
"Go",
"C++",
"Python"
],
"IsOk": "true",
"Price": 3.14
}
通过map生成json:
import (
"encoding/json"
"fmt"
)
func main() {
m := make(map[string]interface{},4)
m["name"] = "tom"
m["subjects"] = []string{"Go","C++","Python"}
m["isok"] = true
m["price"] = 3.14
//编码成json
buf, err := json.MarshalIndent(m, "", " ")
if err != nil {
fmt.Println("err =", err)
return
}
fmt.Println("buf =", string(buf))
}
Output :
buf = {
"isok": true,
"name": "tom",
"price": 3.14,
"subjects": [
"Go",
"C++",
"Python"
]
}
json解析到结构体:
func Unmarshal(data []byte, v interface{}) error
Unmarshal函数解析json编码的数据并将结果存入v指向的值。
import (
"encoding/json"
"fmt"
)
//成员变量必须首字母大写
type Student struct {
Name string `json:"name"`
Subjects []string `json:"subject"` //二次编码
IsOk bool `json:"isok"`
Price float64 `json:"price"`
}
func main() {
buf := `
{
"Name": "tom",
"subject": [
"Go",
"C++",
"Python"
],
"IsOk": true,
"Price": 3.14
}`
var tmp Student //定义一个结构体变量
err := json.Unmarshal([]byte(buf), &tmp) //第二个参数要地址传递
if err != nil {
fmt.Println("err =", err)
return
}
fmt.Println("tmp =", tmp)K
fmt.Printf("tmp = %+v\n", tmp) //%+v可以详细显示
//如果只解析其中的某些成员变量,可以重新定义一个结构体
var tmp2 struct {
Subjects []string `json:"subject"` //二次编码
}
err = json.Unmarshal([]byte(buf), &tmp2) //第二个参数要地址传递
if err != nil {
fmt.Println("err =", err)
return
}
fmt.Printf("tmp2 = %+v\n", tmp2) //%+v可以详细显示
}
Output :
tmp = {tom [Go C++ Python] true 3.14}
tmp = {Name:tom Subjects:[Go C++ Python] IsOk:true Price:3.14}
tmp2 = {Subjects:[Go C++ Python]}
json解析到map:
import (
"encoding/json"
"fmt"
)
func main() {
buf := `
{
"Name": "tom",
"subject": [
"Go",
"C++",
"Python"
],
"IsOk": true,
"Price": 3.14
}`
m := make(map[string]interface{},4)
err := json.Unmarshal([]byte(buf),&m)
if err != nil {
fmt.Println("err =", err)
return
}
fmt.Printf("m = %+v\n", m)
//m = map[IsOk:true Price:3.14 Name:tom subject:[Go C++ Python]]
//由于数据中有各个类型的数据,需要进行提取
//利用类型断言
for key,value := range m{
switch data := value.(type) {
case string:
fmt.Printf("map[%s]的值类型为string, value = %s\n",key,data)
case bool:
fmt.Printf("map[%s]的值类型为bool, value = %v\n",key,data)
case float64:
fmt.Printf("map[%s]的值类型为float64, value = %f\n",key,data)
case []string:
fmt.Printf("map[%s]的值类型为[]string, value = %v\n",key,data)
case []interface{}:
fmt.Printf("map[%s]的值类型为[]interface, value = %v\n",key,data)
}
}
}
Output:
注意: subject类型为[]interface{}类型
扫描二维码关注公众号,回复:
5291404 查看本文章
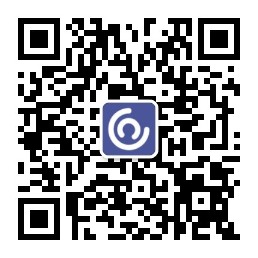
m = map[Name:tom subject:[Go C++ Python] IsOk:true Price:3.14]
map[Price]的值类型为float64, value = 3.140000
map[Name]的值类型为string, value = tom
map[subject]的值类型为[]interface, value = [Go C++ Python]
map[IsOk]的值类型为bool, value = true
json解析结构体较为简单,可以直接看到类型
json解析map方便,但是确定某一种类型要通过类型断言一步一步算回来