用于线程实现的Python模块Python线程有时称为轻量级进程,因为线程比进程占用的内存少得多。 线程允许一次执行多个任务。
在Python中,以下模块在一个程序中实现线程
_thread 模块
threading 模块
concurrent.futures 模块
主要区别在于_thread模块将线程视为一个函数,而threading模块将每个线程视为一个对象并以面向对象的方式实现它。 此外,_thread模块在低级线程中有效并且比threading模块具有更少的功能。
Python3.2带来了 concurrent.futures 模块,这个模块具有线程池和进程池、管理并行编程任务、处理非确定性的执行流程、进程/线程同步等功能。
_thread 模块
在Python的早期版本中,拥有thread模块,但在相当长的一段时间里它已被视为“已弃用”。 鼓励用户改用threading模块。 因此,在Python 3中,thread模块不再可用。 它已被重命名为_thread,用于Python3中的向后不兼容。
为了在_thread模块的帮助下生成新的线程,我们需要调用它的start_new_thread方法。 这种方法的工作可以通过以下语法来理解 -
_thread.start_new_thread ( function, args[, kwargs] )
args是一个参数的元组kwargs是关键字参数的可选字典
如果想在不传递参数的情况下调用函数,那么需要在args中使用一个空的参数元组。
此方法调用立即返回,子线程启动,并调用与传递的列表(如果有的话)args的函数。 线程在函数返回时终止。
import _thread
import time
def print_time( threadName, delay):
count = 0
while count < 5:
time.sleep(delay)
count += 1
print ("%s: %s" % ( threadName, time.ctime(time.time()) ))
try:
_thread.start_new_thread( print_time, ("Thread-1", 2, ) )
_thread.start_new_thread( print_time, ("Thread-2", 4, ) )
except:
print ("Error: unable to start thread")
while 1:
pass
threading模块
threading模块以面向对象的方式实现,并将每个线程视为一个对象。 因此,它为线程提供了比_thread模块更强大,更高层次的支持。
threading 模块中的其他方法
threading模块包含_thread模块的所有方法,但它也提供了其他方法。 其他方法如下
- threading.activeCount() - 此方法返回处于活动状态的线程对象的数量
- threading.currentThread() - 此方法返回调用者线程控制中的线程对象数。
- threading.enumerate() - 此方法返回当前活动的所有线程对象的列表。
为了实现线程,threading模块具有提供以下方法的Thread类 -
- run() - run()方法是线程的入口点。
- start() - start()方法通过调用run方法来启动线程。
- join([time]) - join()等待线程终止。
- isAlive() - isAlive()方法检查线程是否仍在执行。
- getName() - getName()方法返回线程的名称。
- setName() - setName()方法设置线程的名称。
使用threading模块
import threading
import time
exitFlag = 0
class myThread (threading.Thread):
def __init__(self, threadID, name, counter):
threading.Thread.__init__(self)
self.threadID = threadID
self.name = name
self.counter = counter
def run(self):
print ("Starting " + self.name)
print_time(self.name, self.counter, 5)
print ("Exiting " + self.name)
def print_time(threadName, delay, counter):
while counter:
if exitFlag:
threadName.exit()
time.sleep(delay)
print ("%s: %s" % (threadName, time.ctime(time.time())))
counter -= 1
thread1 = myThread(1, "Thread-1", 1)
thread2 = myThread(2, "Thread-2", 2)
thread1.start()
thread2.start()
thread1.join()
thread2.join()
print ("Exiting Main Thread")
带有线程状态的Python程序有五种线程状态 - 新的,可运行,运行,等待和死亡。 在这五个中,我们将主要关注三个状态 - 运行,等待和死亡。 一个线程获取处于运行状态的资源,等待处于等待状态的资源; 如果执行和获取的资源的最终版本处于死亡状态。下面的Python程序在start(),sleep()和join()方法的帮助下将分别显示线程是如何进入运行,等待和死亡状态的。
第1步 - 导入必要的模块,threading和timeimport threading
import time
Python第2步 - 定义一个函数,它将在创建线程时调用。
def thread_states():
print("Thread entered in running state")
Python第3步 - 使用time模块的sleep()方法让线程等待2秒钟。
time.sleep(2)
Python第4步 - 现在,创建一个名为T1的线程,它接受上面定义的函数的参数。
T1 = threading.Thread(target=thread_states)
Python第5步 - 现在,使用start()函数,可以开始启动线程。 它会产生这个信息,这个信息是在定义函数时设定的。
T1.start()
# Thread entered in running state
Python第6步 - 现在,最后可以在完成执行后使用join()方法终止线程。
T1.join()
在Python中启动一个线程
import threading
import time
import random
def Thread_execution(i):
print("Execution of Thread {} started\n".format(i))
sleepTime = random.randint(1,4)
time.sleep(sleepTime)
print("Execution of Thread {} finished".format(i))
for i in range(4):
thread = threading.Thread(target=Thread_execution, args=(i,))
thread.start()
print("Active Threads:" , threading.enumerate())
设置守护线程
import threading
import time
def nondaemonThread():
print("starting my thread")
print("ending my thread")
def daemonThread():
while True:
print("Hello")
if __name__ == '__main__':
nondaemonThread = threading.Thread(target = nondaemonThread)
daemonThread = threading.Thread(target = daemonThread)
daemonThread.setDaemon(True)
daemonThread.start()
nondaemonThread.start()
守护线程必须在主进程存活的状态下运行,主进程关闭所有守护线程全部退出
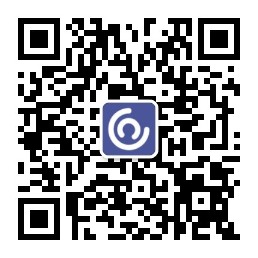