实验 模型和代码的转换
一、实验内容:
【任务4-1】图4-1中是一个“学生自动注册系统”中类模型的一个局部概念模型。请将模型转换为正确的Java代码。所有的类都实现数据封装。每个类的详细说明如下
图4-1 学生自动注册系统局部模型图
- Person类说明
图4-2 Person类的实现类图
属性name(姓名)和ssn(社保号)
display()用于将Person对象的属性值打印。格式如下:
System.out.println("Person Information:");
System.out.println("\tName: " + this.getName());
System.out.println("\tSoc. Security No.: " + this.getSsn());
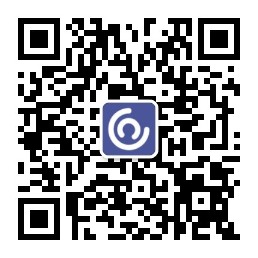
- Professor类说明(双向关联)继承Person类,与Section类的一对多关联”讲授”。因此必须为Professor对象提供维护读到个Section对象的方法。通过创建类型为ArrayList的属性teaches实现这一点。
图4-3 Professor类的实现类图
- displayTeachingAssignments()方法:在控制台输出教师讲授的每一门课程的安排。格式如下:
System.out.println("Teaching Assignments for " + getName() + ":");
System.out.println("\tCourse No.: " +讲授的课程的编号);
System.out.println("\tSection No.: " + 讲授的班级的编号);
System.out.println("\tCourse Name: " +讲授的课程的名字);
System.out.println("\tDay and Time: " +讲授的班级的时间 + " - " + 讲授的班级
的时间段);
System.out.println("\t-----");
例如:
Teaching Assignments for Snidely Whiplash:
Course No.: CMP101
Section No.: 1
Course Name: Beginning Computer Technology
Day and Time: M - 8:10 - 10:00 PM
- display()方法:先输出教师的个人信息,再输出老师的教学安排。显示实例如下:
有教学任务的教师的输出:
Person Information:
Name: Snidely Whiplash
Soc. Security No.: 987-65-4321
Professor-Specific Information:
Title: Full Professor
Teaches for Dept.: Physical Education
Teaching Assignments for Snidely Whiplash:
Course No.: CMP101
Section No.: 1
Course Name: Beginning Computer Technology
Day and Time: M - 8:10 - 10:00 PM
没有教学任务的老师的输出结果
Person Information:
Name: John Smith
Soc. Security No.: 567-81-2345
Professor-Specific Information:
Title: Full Professor
Teaches for Dept.: Chemistry
Teaching Assignments for John Smith:
(none)
- agreeToTeacher(Section s)方法:方法接受一个Seciton对象的引用作为参数,并且首先将其存储在teaches ArrayList中。这样可以建立Professor和Section的单向关联。同时考虑到Section对象也同样需要知道该班级授课的是哪一个Professor(例如,在学生需要打印课程表时)。此方法还需要建立另一方的关联。
- Course类(自反关联、单向关联、双向关联)
- Section类的一对多关联(双向关联):offeredAsSection属性
- 和自己的自反关联,对于给定Course对象X指导其他Course对象A B C等是它的先修课程,但它并不知道哪些课程对象把它作为了先修课程。可以理解成为这是单向关联:prerequisites属性
- scheduleSection()方法:为课程对象创建的班次。该方法调用Section类的构造方法实例化一个新的Seciton对象,并且在将这个Section对象的一个句柄返回给客户代码之前,将这个Section对象的另一个句柄存储在offeredAsSection ArrayList中。另外该方法将offeredAsSection ArrayList 的大小+1的数字作为Section类构造方法的第一个参数(表示Seciton的编号)
图4-4 Course类的实现类图
- Section类
- 与Course的关联由属性representedCourse建立
- 与Professor的关联由属性professor建立
- toString方法的打印格式如下:
getRepresentedCourse().getCourseNo() + " - " + getSectionNo() + " - " +getDayOfWeek() + " - " +getTimeOfDay();
- display方法的打印格式如下:
Section Information:
Course No.: CMP101
Section No: 1
Offered: M at 8:10 - 10:00 PM
In Room: GOVT101
Professor: Snidely Whiplash
- getFullSectionNo()方法返回的字符串
return getRepresentedCourse().getCourseNo() + " - " + getSectionNo();
例如: CMP101 - 1
图4-5 Section类的实现类图
- SRS类:测试类。通过创建一个main方法的将作为测试”驱动器”。源代码见附件。对应的对象图如图4-6所示。
图4-6 局部模型的对象图
//代码:
//Person.java
package demo3;
public abstract class Person {
private String name;
private String ssn;
public Person() {
super();
}
public Person(String name, String ssn) {
super();
this.name = name;
this.ssn = ssn;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getSsn() {
return ssn;
}
public void setSsn(String ssn) {
this.ssn = ssn;
}
@Override
public abstract String toString();
public void display()
{
System.out.println("Person Information:");
System.out.println("\tName: " + this.getName());
System.out.println("\tSoc. Security No.: " + this.getSsn());
}
}
//Professor.java
package demo3;
import java.util.*;
public class Professor extends Person{
private String title;
private String department;
private ArrayList<Section> teaches=new ArrayList<>();
public Professor() {
super();
}
public Professor(String name,String ssn,String title, String department) {
super(name,ssn);
this.title = title;
this.department = department;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getDepartment() {
return department;
}
public void setDepartment(String department) {
this.department = department;
}
@Override
public String toString() {
return "Professor [title=" + title + ", department=" + department
+ ", teaches=" + teaches + "]";
}
public void display()
{
super.display();
System.out.println("Professor-Specific Information:");
System.out.println("\tTitle: "+this.getTitle());
System.out.println("\tTeaches for Department: "+this.getDepartment());
displayTeachingAssignments();
}
public void displayTeachingAssignments()
{
System.out.println("Teaching Assignments for " + getName() + ":");
if(teaches.size()!=0)
{
for(int i=0;i<teaches.size();i++)
{
System.out.println("\tCourse No.: " +teaches.get(i).getRepresentedCourse().getCourseNo());
System.out.println("\tSection No.: " +teaches.get(i).getSectionNo());
System.out.println("\tCourse Name: " +teaches.get(i).getRepresentedCourse().getCourseName());
System.out.println("\tDay and Time: " +teaches.get(i).getDayOfWeek() + " - " + teaches.get(i).getTimeOfDay());
System.out.println("\t-----");
}
}
else
System.out.println("none");
}
public void agreeToTeach(Section s)
{
teaches.add(s);
s.setInstructor(this);
}
}
//Course.java
package demo3;
import java.util.*;
public class Course{
private String courseNo;
private String courseName;
private double credits;
private ArrayList<Section> offeredAsSection=new ArrayList<>();
public ArrayList<Course> prerequisites=new ArrayList<>();
public Course()
{
}
public Course(String courseNo, String courseName, double credits) {
this.courseNo = courseNo;
this.courseName = courseName;
this.credits = credits;
}
public String getCourseNo() {
return courseNo;
}
public void setCourseNo(String courseNo) {
this.courseNo = courseNo;
}
public String getCourseName() {
return courseName;
}
public void setCourseName(String courseName) {
this.courseName = courseName;
}
public double getCredits() {
return credits;
}
public void setCredits(double credits) {
this.credits = credits;
}
public void display()
{
System.out.println("Course Information:");
System.out.println("\tCourseNo: "+getCourseNo());
System.out.println("\tCourseName: "+getCourseName());
System.out.println("\tCredits: "+getCredits());
}
@Override
public String toString() {
return "Course [courseNo=" + courseNo + ", courseName=" + courseName
+ ", credits=" + credits + ", offeredAsSection="
+ offeredAsSection + ", prerequisites=" + prerequisites + "]";
}
public void addPrerequisite(Course c)
{
prerequisites.add(c);
}
public Collection<Course> getPrerequisites()
{
return prerequisites;
}
public Section scheduleSection(char day,String time,String room,int capacity)
{
Section section=new Section(String.valueOf(offeredAsSection.size()+1),day,time,room,capacity);
offeredAsSection.add(section);
section.setRepresentedCourse(this);
return section;
}
}
//Section.java
package demo3;
public class Section{
private String sectionNo;
private char dayOfWeek;
private String room;
private int seatingCapacity;
private String timeOfDay;
private Course representedCourse=new Course();
private Professor instructor;
public Section(String sectionNo, char dayOfWeek, String timeOfDay,String room,
int seatingCapacity) {
this.sectionNo = sectionNo;
this.dayOfWeek = dayOfWeek;
this.room = room;
this.seatingCapacity = seatingCapacity;
this.timeOfDay = timeOfDay;
}
public String getSectionNo() {
return sectionNo;
}
public void setSectionNo(String sectionNo) {
this.sectionNo = sectionNo;
}
public char getDayOfWeek() {
return dayOfWeek;
}
public void setDayOfWeek(char dayOfWeek) {
this.dayOfWeek = dayOfWeek;
}
public String getRoom() {
return room;
}
public void setRoom(String room) {
this.room = room;
}
public int getSeatingCapacity() {
return seatingCapacity;
}
public void setSeatingCapacity(int seatingCapacity) {
this.seatingCapacity = seatingCapacity;
}
public String getTimeOfDay() {
return timeOfDay;
}
public void setTimeOfDay(String timeOfDay) {
this.timeOfDay = timeOfDay;
}
public Course getRepresentedCourse() {
return representedCourse;
}
public void setRepresentedCourse(Course representedCourse) {
this.representedCourse = representedCourse;
}
public Professor getInstructor() {
return instructor;
}
public void setInstructor(Professor instructor) {
this.instructor = instructor;
}
@Override
public String toString() {
return getRepresentedCourse().getCourseNo() + " - " + getSectionNo() + " - " +getDayOfWeek() + " - " +getTimeOfDay();
}
public String getFullSectionNo()
{
return getRepresentedCourse().getCourseNo() + " - " + getSectionNo();
}
public void display()
{
System.out.println("Section Information:");
System.out.println("\tCourse No: "+representedCourse.getCourseNo());
System.out.println("\tSection No: "+getSectionNo());
System.out.println("\tOffered: "+getTimeOfDay());
System.out.println("\tIn Room: "+getRoom());
System.out.println("\tProfessor: "+getInstructor().getName());
}
}
//SRS.java
package demo3;
import java.util.ArrayList;
public class SRS {
// We can effectively create "global" data by declaring
// collections of objects as public static attributes in
// the main class; these can then be accessed throughout the
// SRS application as: SRS.collectionName; e.g., SRS.faculty.
public static ArrayList<Professor> faculty;
public static ArrayList<Course> courseCatalog;
public static void main(String[] args) {
Professor p1, p2, p3;
Course c1, c2, c3, c4, c5;
Section sec1, sec2, sec3, sec4, sec5, sec6, sec7;
// -----------
// Professors.创建教师
// -----------
p1 = new Professor("Jacquie Barker", "123-45-6789",
"Adjunct Professor", "Information Technology");
p2 = new Professor("John Smith", "567-81-2345",
"Full Professor", "Chemistry");
p3 = new Professor("Snidely Whiplash", "987-65-4321",
"Full Professor", "Physical Education");
// Add these to the appropriate ArrayList.
//将创建的教师保存到集合类中
faculty = new ArrayList<Professor>();
faculty.add(p1);
faculty.add(p2);
faculty.add(p3);
// --------
// Courses.创建课程
// --------
c1 = new Course("CMP101", "Beginning Computer Technology", 3.0);
c2 = new Course("OBJ101", "Object Methods for Software Development", 3.0);
c3 = new Course("CMP283", "Higher Level Languages (Java)", 3.0);
c4 = new Course("CMP999", "Living Brain Computers", 3.0);
c5 = new Course("ART101","Beginning Basketweaving", 3.0);
// Add these to the appropriate ArrayList. 将创建的课程保存到对应的集合类中
courseCatalog = new ArrayList<Course>();
courseCatalog.add(c1);
courseCatalog.add(c2);
courseCatalog.add(c3);
courseCatalog.add(c4);
courseCatalog.add(c5);
// Establish some prerequisites (c1 => c2 => c3 => c4).
//设置课程之间的关系 c1是c2的先修课程,c2是c3的先修课程,c3是c4的先修课程
c2.addPrerequisite(c1);
c3.addPrerequisite(c2);
c4.addPrerequisite(c3);
// ---------
// Sections.创建教学班级
// ---------
sec1 = c1.scheduleSection('M', "8:10 - 10:00 PM", "GOVT101", 30);
sec2 = c1.scheduleSection('W', "6:10 - 8:00 PM", "GOVT202", 30);
sec3 = c2.scheduleSection('R', "4:10 - 6:00 PM", "GOVT105", 25);
sec4 = c2.scheduleSection('T', "6:10 - 8:00 PM", "SCI330", 25);
sec5 = c3.scheduleSection('M', "6:10 - 8:00 PM", "GOVT101", 20);
sec6 = c4.scheduleSection('R', "4:10 - 6:00 PM", "SCI241", 15);
sec7 = c5.scheduleSection('M', "4:10 - 6:00 PM", "ARTS25", 40);
// Recruit a professor to teach each of the sections.
//为老师排课
p3.agreeToTeach(sec1);
p2.agreeToTeach(sec2);
p1.agreeToTeach(sec3);
p3.agreeToTeach(sec4);
p1.agreeToTeach(sec5);
p2.agreeToTeach(sec6);
p3.agreeToTeach(sec7);
System.out.println("======================");
System.out.println("Professor Information:");
System.out.println("======================");
System.out.println();
p1.display();
p2.display();
p3.display();
System.out.println("====================");
System.out.println("Course Information:");
System.out.println("====================");
System.out.println();
c1.display();
c2.display();
c3.display();
System.out.println("====================");
System.out.println("Section Information:");
System.out.println("====================");
System.out.println();
sec1.display();
sec2.display();
sec3.display();
sec4.display();
sec5.display();
sec6.display();
sec7.display();
}
}
//运行结果: