单向链表的获取元素,删除元素,插入元素看似很难,但是当抓住要点后,一切问题都迎刃而解。我来发表一下我学习链表时的一些思路与见解,仅供学习交流之用,因水平问题,如有错误短视之处,恳请谅解。
struct note{
char name[20];
int heartlevel;
struct note *next;
};
void get(struct note *head,int n){
......
}
定义while(step<n){
step++;
p=p->next;
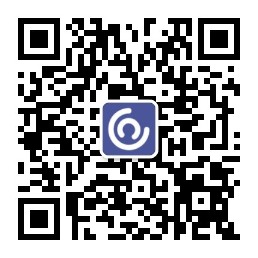
if(p==NULL){
flag=1;
printf("There are no data.\n");
break;
}
}
当n在链表内时printf("n=%d,creature=%s,hrearlevel=%d\n",n,p->name,p->heartlevel);
当flag为1时,数据不存在。void get(struct note *head,int n){//输入一个n,返回其对应的元素,如果没有,则输出There are no creatures.
struct note *p=head;
int flag=0;
int step=1;
while(step<n){
step++;
p=p->next;
if(p==NULL){
flag=1;
printf("There are no data.\n");
break;
}
}
if(flag==0){
printf("n=%d,creature=%s,hrearlevel=%d\n",n,p->name,p->heartlevel);
}
}
struct note *p=head;
struct note *q;
int step=1;
int flag=0;
if(head==NULL){
printf("There are no data.\n");
}
if(n==1){
struct note *q=head;
head=head->next;
free(q);
printf("delete OK\n");
}
while(step<n-1){
p=p->next;
step++;
}
while(p->next&&step<n-1){
p=p->next;
step++;
}
if((p->next==NULL)&&step<=n-1){
flag=1;
printf("There are no data.\n");
}
flag=1标明n超过链表长度;p->next=NULL;
free(q);
printf("delete OK\n");
p->next=NULL;
free(q);
struct note *delete(struct note *head,int n){
struct note *p=head;
struct note *q;
int step=1;
int flag=0;
if(head==NULL){
printf("There are no data.\n");
}else{
if(n==1){
struct note *q=head;
head=head->next;
free(q);
printf("delete OK\n");
}else{
while(p->next&&step<n-1){
p=p->next;
step++;
}
if((p->next==NULL)&&step<=n-1){
flag=1;
printf("There are no data.\n");
}
if(flag==0){
q=p->next;
if(q->next==NULL){
p->next=NULL;
free(q);
printf("delete OK\n");
}else{
p->next=q->next;
free(q);
printf("delete OK\n");
}
}
}
}
return head;
}
struct note *inster(struct note *head,int n){
struct note *p,*q,*temp;
if(head==NULL){
printf("There are no data.\n");
把p指向n-1的位置(先不考虑在头节点前添加的情况):int step=1;
int flag=0;
p=head;
while(p->next&&step<n-1){
p=p->next;
step++;
}
if(p->next==NULL&&step<=n-1){
flag=1;
printf("There are no data.\n");
}
temp=(struct note*)malloc(sizeof(struct note));
temp=(struct note*)malloc(sizeof(struct note));
head=temp;
temp->next=p;
setbuf(stdin, NULL);
scanf("%s %d",temp->name,&temp->heartlevel);
temp=(struct note*)malloc(sizeof(struct note));
head=temp;
temp->next=p;
setbuf(stdin, NULL);
scanf("%s %d",temp->name,&temp->heartlevel);
struct note *inster(struct note *head,int n){
struct note *p,*q,*temp;
if(head==NULL){
printf("There are no data.\n");
}else{
int step=1;
int flag=0;
p=head;
while(p->next&&step<n-1){
p=p->next;
step++;
}
if(p->next==NULL&&step<=n-1){
flag=1;
printf("There are no data.\n");
}
if(flag==0){
if(n!=1){
temp=(struct note*)malloc(sizeof(struct note));
q=p->next;
p->next=temp;
temp->next=q;
setbuf(stdin, NULL);
scanf("%s %d",temp->name,&temp->heartlevel);
}else{
temp=(struct note*)malloc(sizeof(struct note));
head=temp;
temp->next=p;
setbuf(stdin, NULL);
scanf("%s %d",temp->name,&temp->heartlevel);
}
}
}
return head;
}
fflush(stdin); 或rewind(stdin);