目录
扫描二维码关注公众号,回复:
16506701 查看本文章
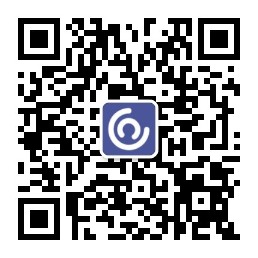
1.链表静态增加和动态遍历
#include <stdio.h>
struct Test
{
int data;
struct Test *next;
};
void printflink(struct Test *head)
{
while(head!=NULL){
printf("%d\n",head->data);
head = head->next;
}
}
int main()
{
struct Test *head = NULL;
struct Test p1 = {11,NULL};
struct Test p2 = {22,NULL};
struct Test p3 = {33,NULL};
struct Test p4 = {44,NULL};
head = &p1;
p1.next = &p2;
p2.next = &p3;
p3.next = &p4;
printflink(&p1);
return 0;
}
2.统计链表节点各数及链表查找
#include <stdio.h>
struct Test
{
int data;
struct Test *next;
};
void printflink(struct Test *head)
{
while(head!=NULL){
printf("%d\n",head->data);
head = head->next;
}
}
int getlinksum(struct Test *head)
{
int cnt;
while(head!=NULL){
cnt++;
head = head->next;
}
return cnt;
}
int findlink(struct Test *head,int newdata)
{
while(head!=NULL){
if(head->data == newdata){
return 1;
}
head = head->next;
} return 0;
}
int main()
{
struct Test *head = NULL;
struct Test p1 = {11,NULL};
struct Test p2 = {22,NULL};
struct Test p3 = {33,NULL};
struct Test p4 = {44,NULL};
head = &p1;
p1.next = &p2;
p2.next = &p3;
p3.next = &p4;
printflink(&p1);
int ret1 = getlinksum(head);
printf("the link sum = %d\n",ret1);
int ret2 = findlink(&p1,111);
if(ret2 == 1){
printf("hava newdata\n");
}
else{
printf("no newdata\n");
}
return 0;
}
3.链表从指定节点后方插入新节点
#include <stdio.h>
struct Test
{
int data;
struct Test *next;
};
void printflink(struct Test *head)
{
while(head!=NULL){
printf("%d\n",head->data);
head = head->next;
}
}
int getlinksum(struct Test *head)
{
int cnt;
while(head!=NULL){
cnt++;
head = head->next;
}
return cnt;
}
void insertfrombehind(struct Test *head,int data,struct Test *new)
{
while(head!=NULL){
if(head->data == data){
new->next = head->next;
head->next = new;
}
head = head->next;
}
}
int main()
{
struct Test *head = NULL;
struct Test p1 = {11,NULL};
struct Test p2 = {22,NULL};
struct Test p3 = {33,NULL};
struct Test p4 = {44,NULL};
struct Test new = {520,NULL};
head = &p1;
p1.next = &p2;
p2.next = &p3;
p3.next = &p4;
printflink(head);
int ret1 = getlinksum(head);
printf("the link sum = %d\n",ret1);
insertfrombehind(head,44,&new);
printflink(head);
int ret2 = getlinksum(head);
printf("the newlink sum = %d\n",ret2);
return 0;
}
4.链表从指定节点前方插入新节点
#include <stdio.h>
struct Test
{
int data;
struct Test *next;
};
void printflink(struct Test *head)
{
while(head!=NULL){
printf("%d\n",head->data);
head = head->next;
}
}
int getlinksum(struct Test *head)
{
int cnt;
while(head!=NULL){
cnt++;
head = head->next;
}
return cnt;
}
struct Test* insertfromfront(struct Test *head,int data,struct Test *new)
{
if(head->data == data){
new->next = head;
head = new;
printf("insert success\n");
return head;
}
while(head->next!=NULL){
if(head->next->data == data){
new->next = head->next;
head->next = new;
printf("insert ok\n");
return head;
}
head = head->next;
}
}
int main()
{
struct Test *head = NULL;
struct Test p1 = {11,NULL};
struct Test p2 = {22,NULL};
struct Test p3 = {33,NULL};
struct Test p4 = {44,NULL};
struct Test new = {520,NULL};
head = &p1;
p1.next = &p2;
p2.next = &p3;
p3.next = &p4;
printflink(head);
int ret1 = getlinksum(head);
printf("the link sum = %d\n",ret1);
head = insertfromfront(head,11,&new);
printflink(head);
int ret2 = getlinksum(head);
printf("the newlink sum = %d\n",ret2);
return 0;
}
5.链表删除指定节点
include <stdio.h>
struct Test
{
int data;
struct Test *next;
};
void printflink(struct Test *head)
{
while(head!=NULL){
printf("%d\n",head->data);
head = head->next;
}
}
int getlinksum(struct Test *head)
{
int cnt;
while(head!=NULL){
cnt++;
head = head->next;
}
return cnt;
}
int findlink(struct Test *head,int newdata)
{
while(head!=NULL){
if(head->data == newdata){
return 1;
}
head = head->next;
}
return 0;
}
struct Test* deleteNode(struct Test *head,int data)
{
if(head->data == data){
head = head->next;
return head;
}
while(head->next!=NULL){
if(head->next->data == data){
head->next = head->next->next;
return head;
}
head = head->next;
}
return head;
}
int main()
{
struct Test *head = NULL;
struct Test p1 = {11,NULL};
struct Test p2 = {22,NULL};
struct Test p3 = {33,NULL};
struct Test p4 = {44,NULL};
struct Test new = {520,NULL};
head = &p1;
p1.next = &p2;
p2.next = &p3;
p3.next = &p4;
printflink(head);
int ret1 = getlinksum(head);
printf("the link sum = %d\n",ret1);
head = deleteNode(head,11);
printflink(head);
int ret2 = getlinksum(head);
printf("the newlink sum = %d\n",ret2);
return 0;
}
6.链表修改指定节点
#include <stdio.h>
struct Test
{
int data;
struct Test *next;
};
void printflink(struct Test *head)
{
while(head!=NULL){
printf("%d\n",head->data);
head = head->next;
}
}
int getlinksum(struct Test *head)
{
int cnt;
while(head!=NULL){
cnt++;
head = head->next;
}
return cnt;
}
int changeNode(struct Test *head,int data,int newdata)
{
while(head!=NULL){
if(head->data == data){
head->data = newdata;
return 1;
}
head = head->next;
}
return 0;
}
int main()
{
struct Test *head = NULL;
struct Test p1 = {11,NULL};
struct Test p2 = {22,NULL};
struct Test p3 = {33,NULL};
struct Test p4 = {44,NULL};
struct Test new = {520,NULL};
head = &p1;
p1.next = &p2;
p2.next = &p3;
p3.next = &p4;
printflink(head);
int ret1 = getlinksum(head);
printf("the link sum = %d\n",ret1);
changeNode(head,11,111);
printflink(head);
int ret2 = getlinksum(head);
printf("the newlink sum = %d\n",ret2);
return 0;
}
7.头插法动态创建链表
#include <stdio.h>
#include <stdlib.h>
struct Test
{
int data;
struct Test *next;
};
void printflink(struct Test *head)
{
while(head!=NULL){
printf("%d\n",head->data);
head = head->next;
}
}
struct Test* insertfromhead(struct Test *head,struct Test *new)
{
if(head == NULL){
head = new;
}
else{
new->next = head;
head = new;
}
return head;
}
struct Test* headinsert(struct Test *head)
{
struct Test *new;
while(1){
new = (struct Test*)malloc(sizeof(struct Test));
scanf("%d",&(new->data));
if(new->data == 0){
printf("end\n");
free(new);
return head;
}
head = insertfromhead(head,new);
}
}
int main()
{
struct Test *head = NULL;
head = headinsert(head);
printflink(head);
return 0;
}
8.尾插法动态创建链表
#include <stdio.h>
#include <stdlib.h>
struct Test
{
int data;
struct Test *next;
};
void printflink(struct Test *head)
{
while(head!=NULL){
printf("%d\n",head->data);
head = head->next;
}
}
struct Test* insertfromend(struct Test *head,struct Test *new)
{
struct Test *p = head;
if(p == NULL){
head = new;
return head;
}
while(p!=NULL){
if(p->next == NULL){
p->next = new;
return head;
}
p = p->next;
}
}
struct Test* endinsert(struct Test *head)
{
struct Test *new;
while(1){
new = (struct Test*)malloc(sizeof(struct Test));
scanf("%d",&(new->data));
if(new->data == 0){
printf("end\n");
free(new);
return head;
}
head = insertfromend(head,new);
}
}
int main()
{
struct Test *head = NULL;
head = endinsert(head);
printflink(head);
return 0;
}