代码:
#include<stdio.h>
#include<stdlib.h>
typedef struct LNode {
int data;
struct LNode *next;
}LNode,*LinkList;
LNode *GetElem(LinkList L, int i) {
int j = 1;
LNode *p = L->next;
if (i == 0)
return L;
if (i < 1)
return NULL;
while (p != NULL && j < i) {
p = p->next;
j++;
}
return p;
}
bool InitList(LinkList &L) {
L = (LNode *)malloc(sizeof(LNode));
if (L == NULL) {
return false;
}
L->next = NULL;
return true;
}
bool Empty(LinkList L) {
if (L->next == NULL) {
return true;
}
else {
return false;
}
}
bool InsertNextNode(LNode *p, int e) {
if (p == NULL) {
return false;
}
LNode *s = (LNode *)malloc(sizeof(LNode));
if (s == NULL) {
return false;
}
s->data = e;
s->next = p->next;
p->next = s;
return true;
}
bool ListInsert(LinkList &L, int i, int e) {
if (i < 1) {
return false;
}
LNode *p;
p = L;
int j = 0;
while (p!=NULL && j < i-1) {
p = p->next;
j++;
}
return InsertNextNode(p, e);
}
bool InsertPriorNode(LNode *p,int e) {
if (p == NULL) {
return false;
}
LNode *s = (LNode *)malloc(sizeof(LNode));
if (s == NULL) {
return false;
}
s->next = p->next;
p->next = s;
s->data = p->data;
p->data = e;
return true;
}
void DisplayList(LinkList L) {
LNode *p;
p = L->next;
int i = 0;
while (p!= NULL) {
printf("%d ", p->data);
p = p->next;
i++;
}
printf("一共有%d个元素\n",i);
}
bool NodeDelete(LinkList &L, int i,int &e) {
if (i < 1) {
return false;
}
LNode *p;
LNode *q;
int j = 0;
p = L;
while (p != NULL && j < i - 1) {
p = p->next;
j++;
}
if (p == NULL) {
return false;
}
if (p->next == NULL) {
return false;
}
q = p->next;
e = q->data;
p->next = q->next;
free(q);
return true;
}
bool DeleteNode(LNode *p) {
if (p == NULL) {
return false;
}
LNode *q = p->next;
if (q == NULL) {
return false;
}
p->data = q->data;
p->next = q->next;
free(q);
return true;
}
void main() {
LinkList L;
InitList(L);
DisplayList(L);
ListInsert(L, 1, 1);
ListInsert(L, 2, 2);
ListInsert(L, 3, 3);
ListInsert(L, 4, 4);
DisplayList(L);
ListInsert(L, 3, 7);
DisplayList(L);
LNode *p;
p = L;
int i = 0;
while (p != NULL && i < 5) {
p = p->next;
i++;
}
InsertPriorNode(p, 9);
DisplayList(L);
int e = -1;
NodeDelete(L, 2 ,e);
DisplayList(L);
printf("删除的元素值为%d\n", e);
p = p->next;
if (!DeleteNode(p)) {
printf("删除失败!\n");
}
DisplayList(L);
}
结果:
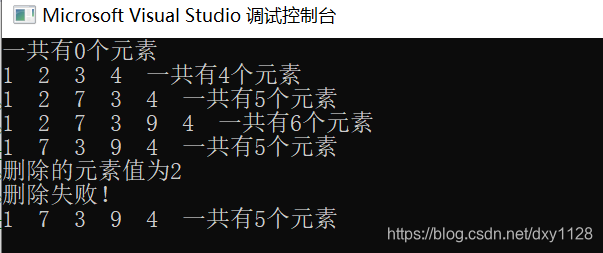