【LeetCode】188. Best Time to Buy and Sell Stock IV 买卖股票的最佳时机 IV(Hard)(JAVA)
题目地址: https://leetcode.com/problems/best-time-to-buy-and-sell-stock-iv/
题目描述:
You are given an integer array prices where prices[i] is the price of a given stock on the i-th day.
Design an algorithm to find the maximum profit. You may complete at most k transactions.
Notice that you may not engage in multiple transactions simultaneously (i.e., you must sell the stock before you buy again).
Example 1:
Input: k = 2, prices = [2,4,1]
Output: 2
Explanation: Buy on day 1 (price = 2) and sell on day 2 (price = 4), profit = 4-2 = 2.
Example 2:
Input: k = 2, prices = [3,2,6,5,0,3]
Output: 7
Explanation: Buy on day 2 (price = 2) and sell on day 3 (price = 6), profit = 6-2 = 4. Then buy on day 5 (price = 0) and sell on day 6 (price = 3), profit = 3-0 = 3.
Constraints:
- 0 <= k <= 10^9
- 0 <= prices.length <= 1000
- 0 <= prices[i] <= 1000
题目大意
给定一个整数数组 prices ,它的第 i 个元素 prices[i] 是一支给定的股票在第 i 天的价格。
设计一个算法来计算你所能获取的最大利润。你最多可以完成 k 笔交易。
注意:你不能同时参与多笔交易(你必须在再次购买前出售掉之前的股票)。
解题方法
- 采用动态规划,找出动态规划函数:股票的题目都可以用动态规划,用分状态表示即可
- 一个三维数组表示交易的情况 dp[i][j]: 表示前面 i 个股票,做多交易 j 次;dp[i][j][0]: 表示当前持有股票; dp[i][j][1]: 表示当前不持有股票
- 加入 dp[i][j] 之前的状态都已经计算出来了。 dp[i][j][0] , 要么这一次不交易:dp[i - 1][j][0]; 要么这一次交易:dp[i][j - 1][1] + prices[i],求出最大值即可。同理也可以求出 dp[i][j][1]
- note: 因为只用到了 dp[i - 1][j][1] 和 dp[i - 1][j][0] 的状态,可以优化用二维数组即可
class Solution {
public int maxProfit(int k, int[] prices) {
if (prices.length <= 1 || k == 0) return 0;
// dp[i][j][0]: has stock; dp[i][j][1]: no stock
int[][][] dp = new int[prices.length][k + 1][2];
for (int i = 0; i < prices.length; i++) {
for (int j = 0; j <= k; j++) {
if (i == 0) {
dp[i][j][0] = -prices[i];
dp[i][j][1] = 0;
} else if (j == 0) {
dp[i][j][0] = Math.max(-prices[i], dp[i - 1][j][0]);
dp[i][j][1] = 0;
} else {
dp[i][j][0] = Math.max(dp[i - 1][j][1] - prices[i], dp[i - 1][j][0]);
dp[i][j][1] = Math.max(dp[i - 1][j - 1][0] + prices[i], dp[i - 1][j][1]);
}
}
}
return dp[prices.length - 1][k][1];
}
}
执行耗时:5 ms,击败了79.78% 的Java用户
内存消耗:41.3 MB,击败了15.51% 的Java用户
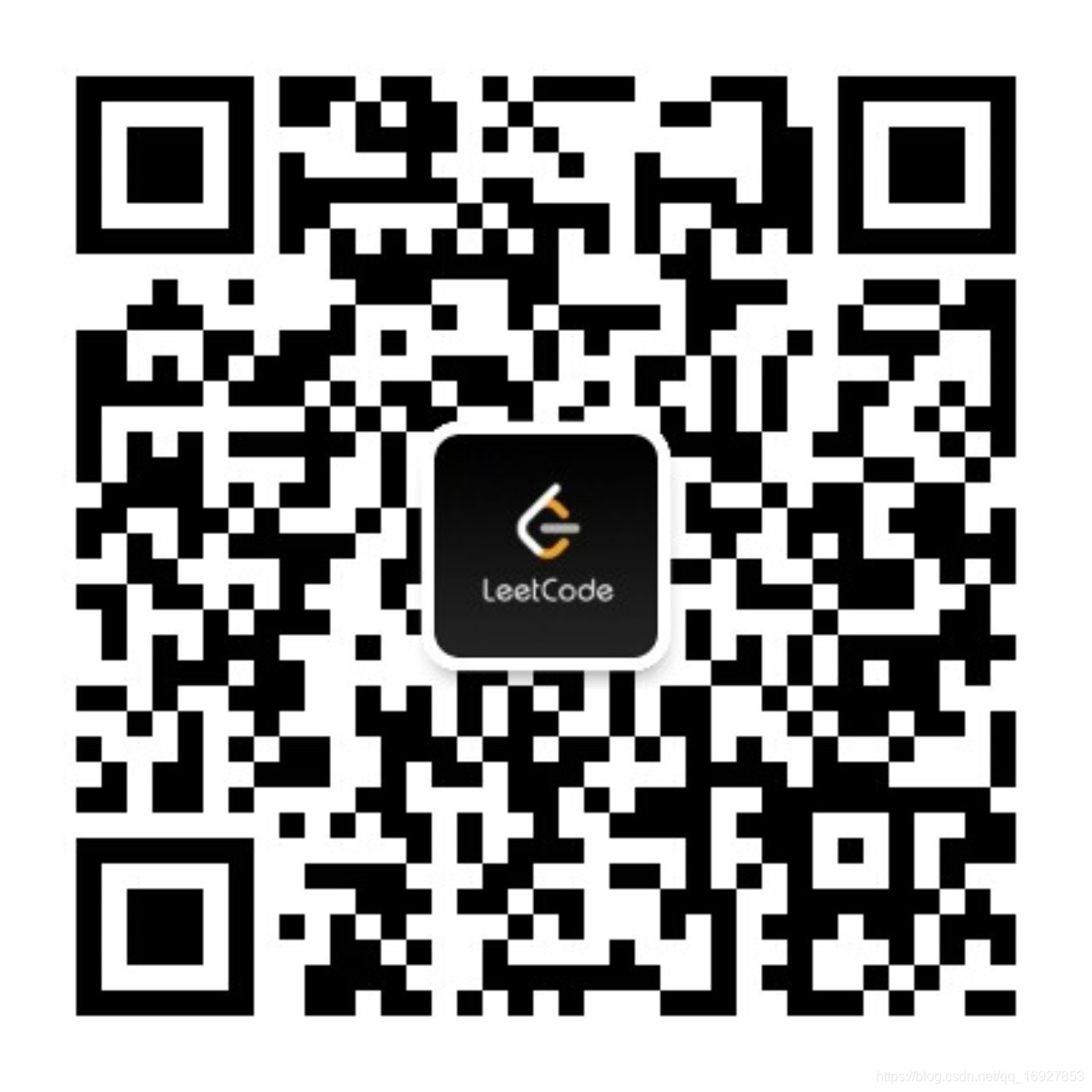