课程大纲
第二章 Python10分钟入门
【2.1】:PyCharm社区版配置Anaconda开发环境
【2.2】:Python基础知识及正则表达式入门
第三章 Python操作Excel
【3.1】:xlrd 使用教程 读取 操作Excel
【3.2】:xlwt 使用教程 写入 操作Excel
【3.3】:xlutils 使用教程 修改 操作Excel
【3.4】:xlwings 使用教程 读取 写入 修改 操【作Excel
【3.5】:openpyxl 使用教程 读取 写入 修改 操作Excel
【3.6】:xlswriter 使用教程 读取 写入 修改 操作Excel
【3.7】:win32com 使用教程 读取 写入 修改 操作Excel
【3.8】:pandas 使用教程 读取 写入 修改 操作Excel
第四章 Python操作word
【4.1】:win32com 使用教程 操作word
【4.2】:python-docx 使用教程 操作word
第五章 Python操作ppt
【5.1】:win32com 使用教程 操作复制ppt PowerPoint
【5.2】:python-pptx 使用教程 操作ppt PowerPoint 添加文字 形状图表
文章目录
官方文档:https://python-pptx.readthedocs.io/en/latest/
5.2.1 pip安装python-pptx
pip install python-pptx
5.2.2 python-pptx 复制页面
使用python-pptx进行复制没有找到合适的方法,有以下两种解决办法:
- 使用win32com对ppt模板进行复制
- 增加模板ppt数量,然后使用python-pptx对不需要的模板页进行删减操作
5.2.3 python-pptx 删除页面
from pptx import Presentation
# 删除某一页ppt
def del_slide(prs,index):
slides = list(prs.slides._sldIdLst)
prs.slides._sldIdLst.remove(slides[index])
# 5.2.3 python-pptx 删除页面
def fun5_2_3():
# 打开ppt
ppt = Presentation('5.2 python-pptx测试.pptx')
# 获取所有页
slides = ppt.slides
number_pages = len(slides)
print("删除前ppt一共",number_pages,"页面")
# 删除第1页
del_slide(ppt,0)
# 再次获取所有页
slides = ppt.slides
number_pages = len(slides)
print("删除后ppt一共",number_pages,"页面")
ppt.save('5_2_3.pptx')
print('生成完毕')
if __name__ == '__main__':
fun5_2_3()
5.2.4 python-pptx 添加文字并设置样式
from pptx import Presentation
from pptx.util import Pt,Cm
# 5.2.4 python-pptx 添加文字
def fun5_2_4():
# 设置添加到当前ppt哪一页
n_page = 0
content = "我是添加的内容"
# 打开已存在ppt
ppt = Presentation('5.2 python-pptx测试.pptx')
# 获取需要添加文字的页面对象
slide = ppt.slides[n_page]
# 设置添加文字框的位置以及大小
left, top, width, height = Cm(16.9), Cm(1), Cm(12), Cm(1.2)
# 添加文字段落
new_paragraph = slide.shapes.add_textbox(left=left, top=top, width=width, height=height).text_frame
# 设置段落内容
new_paragraph.paragraphs[0].text = content
# 设置文字大小
new_paragraph.paragraphs[0].font.size = Pt(15)
# 保存ppt
ppt.save('5_2_4.pptx')
5.2.5 python-pptx 添加表格并设置样式
from pptx import Presentation
from pptx.util import Pt,Cm
from pptx.dml.color import RGBColor
from pptx.enum.text import MSO_ANCHOR
from pptx.enum.text import PP_ALIGN
# 5.2.5 python-pptx 添加表格并设置样式
def fun5_2_5():
# 设置需要添加到哪一页
n_page = 0
# 打开已存在ppt
ppt = Presentation('5.2 python-pptx测试.pptx')
# 获取slide对象
slide = ppt.slides[n_page]
# 设置表格位置和大小
left, top, width, height = Cm(6), Cm(12), Cm(13.6), Cm(5)
# 表格行列数,和大小
shape = slide.shapes.add_table(6, 7, left, top, width, height)
# 获取table对象
table = shape.table
# 设置列宽
table.columns[0].width = Cm(3)
table.columns[1].width = Cm(2.3)
table.columns[2].width = Cm(2.3)
table.columns[3].width = Cm(1.3)
table.columns[4].width = Cm(1.3)
table.columns[5].width = Cm(1.3)
table.columns[6].width = Cm(2.1)
# 设置行高
table.rows[0].height = Cm(1)
# 合并首行
table.cell(0, 0).merge(table.cell(0, 6))
# 填写标题
table.cell(1, 0).text = "时间"
table.cell(1, 1).text = "阶段"
table.cell(1, 2).text = "执行用例"
table.cell(1, 3).text = "新增问题"
table.cell(1, 4).text = "问题总数"
table.cell(1, 5).text = "遗留问题"
table.cell(1, 6).text = "遗留致命/" \
"严重问题"
# 填写变量内容
table.cell(0, 0).text = "产品1"
content_arr = [["4/30-5/14", "DVT1", "20", "12", "22", "25", "5"],
["5/15-5/21", "DVT1", "25", "32", "42", "30", "8"],
["5/22-6/28", "DVT1", "1", "27", "37", "56", "12"],
["5/22-6/28", "DVT1", "1", "27", "37", "56", "12"]]
# 修改表格样式
for rows in range(6):
for cols in range(7):
# Write column titles
if rows == 0:
# 设置文字大小
table.cell(rows, cols).text_frame.paragraphs[0].font.size = Pt(15)
# 设置文字颜色
table.cell(rows, cols).text_frame.paragraphs[0].font.color.rgb = RGBColor(255, 255, 255)
# 设置文字左右对齐
table.cell(rows, cols).text_frame.paragraphs[0].alignment = PP_ALIGN.CENTER
# 设置文字上下对齐
table.cell(rows, cols).vertical_anchor = MSO_ANCHOR.MIDDLE
# 设置背景为填充
table.cell(rows, cols).fill.solid()
# 设置背景颜色
table.cell(rows, cols).fill.fore_color.rgb = RGBColor(34, 134, 165)
elif rows == 1:
table.cell(rows, cols).text_frame.paragraphs[0].font.size = Pt(10)
table.cell(rows, cols).text_frame.paragraphs[0].font.color.rgb = RGBColor(0, 0, 0)
table.cell(rows, cols).text_frame.paragraphs[0].alignment = PP_ALIGN.CENTER
table.cell(rows, cols).vertical_anchor = MSO_ANCHOR.MIDDLE
table.cell(rows, cols).fill.solid()
table.cell(rows, cols).fill.fore_color.rgb = RGBColor(204, 217, 225)
else:
table.cell(rows, cols).text = content_arr[rows - 2][cols]
table.cell(rows, cols).text_frame.paragraphs[0].font.size = Pt(10)
table.cell(rows, cols).text_frame.paragraphs[0].font.color.rgb = RGBColor(0, 0, 0)
table.cell(rows, cols).text_frame.paragraphs[0].alignment = PP_ALIGN.CENTER
table.cell(rows, cols).vertical_anchor = MSO_ANCHOR.MIDDLE
table.cell(rows, cols).fill.solid()
table.cell(rows, cols).fill.fore_color.rgb = RGBColor(204, 217, 225)
ppt.save('5_2_5.pptx')
效果如下:
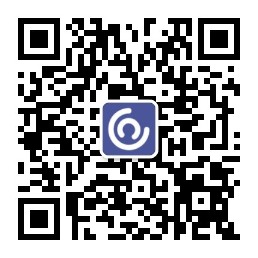
5.2.6 python-pptx 添加图表并设置样式
from pptx import Presentation
from pptx.util import Pt,Cm
from pptx.dml.color import RGBColor
from pptx.enum.text import MSO_ANCHOR
from pptx.enum.text import PP_ALIGN
from pptx.chart.data import ChartData
from pptx.enum.chart import XL_CHART_TYPE
# 5.2.6 python-pptx 添加图表并设置样式
def fun5_2_6():
# 设置需要添加到哪一页
n_page = 0
# 打开已存在ppt
ppt = Presentation('5.2 python-pptx测试.pptx')
# 获取slide对象
slide = ppt.slides[n_page]
# 初始化图表
chart_data = ChartData()
# 填充需要添加的内容
content_arr = [["4/30-5/14", "DVT1", "20", "12", "22", "25", "5"],
["5/15-5/21", "DVT1", "25", "32", "42", "30", "8"],
["5/22-6/28", "DVT1", "1", "27", "37", "56", "12"],
["5/22-6/28", "DVT1", "1", "27", "37", "56", "12"]]
# 填充图表
chart_data.categories = [content_arr[0][0], content_arr[1][0], content_arr[2][0], content_arr[3][0]]
chart_data.add_series("问题总数", (content_arr[0][4], content_arr[1][4], content_arr[2][4], content_arr[3][4]))
chart_data.add_series("遗留问题总数", (content_arr[0][5], content_arr[1][5], content_arr[2][5], content_arr[3][5]))
chart_data.add_series("遗留致命严重\n问题总数", (content_arr[0][6], content_arr[1][6], content_arr[2][6], content_arr[3][6]))
# 设置位置
left, top, width, height = Cm(6), Cm(10), Cm(16.1), Cm(7.5)
# 添加图表
chart = slide.shapes.add_chart(
XL_CHART_TYPE.LINE, left, top, width, height, chart_data
).chart
chart.has_legend = True
chart.legend.include_in_layout = False
# chart.series[0].smooth = True # 是否平滑
# chart.series[1].smooth = True
# chart.series[2].smooth = True
chart.font.size = Pt(10) # 文字大小
ppt.save('5_2_6.pptx')
print('折线图添加完成')
效果如下:
其它图标可参考:https://www.cnblogs.com/adam012019/p/11348938.html
5.2.7 python-pptx 添加任意形状并设置样式
这里的形状可以是这些:
形状别名可以再这里查看:
https://docs.microsoft.com/zh-cn/office/vba/api/Office.MsoAutoShapeType
并对应这里,找到正确的枚举名:
https://python-pptx.readthedocs.io/en/latest/api/enum/MsoAutoShapeType.html#msoautoshapetype
程序示例:
from pptx import Presentation
from pptx.util import Pt,Cm
from pptx.dml.color import RGBColor
from pptx.enum.text import MSO_ANCHOR
from pptx.enum.text import PP_ALIGN
from pptx.chart.data import CategoryChartData, ChartData
from pptx.enum.chart import XL_CHART_TYPE
from pptx.enum.shapes import MSO_SHAPE
# 5.2.7 python-pptx 添加形状并设置样式
def fun5_2_7():
# 设置需要添加到哪一页
n_page = 0
# 打开已存在ppt
ppt = Presentation('5.2 python-pptx测试.pptx')
# 获取slide对象
slide = ppt.slides[n_page]
# 添加矩形
# 设置位置以及大小
left, top, width, height = Cm(2.5), Cm(4.5), Cm(30), Cm(0.5)
# 添加形状
rectangle = slide.shapes.add_shape(MSO_SHAPE.RECTANGLE, left, top, width, height)
# 设置背景填充
rectangle.fill.solid()
# 设置背景颜色
rectangle.fill.fore_color.rgb = RGBColor(34, 134, 165)
# 设置边框颜色
rectangle.line.color.rgb = RGBColor(34, 134, 165)
# 添加正三角+文字(正常)
left, top, width, height = Cm(3), Cm(5.1), Cm(0.5), Cm(0.4)
slide.shapes.add_shape(MSO_SHAPE.FLOWCHART_EXTRACT, left, top, width, height)
new_paragraph = slide.shapes.add_textbox(left=left - Cm(0.95), top=top + Cm(0.4), width=Cm(2.4),
height=Cm(1.1)).text_frame
content = """2020/01/05
内容1"""
new_paragraph.paragraphs[0].text = content
new_paragraph.paragraphs[0].font.size = Pt(10) # 文字大小
new_paragraph.paragraphs[0].alignment = PP_ALIGN.CENTER
# 添加正三角+文字(延期)
left, top, width, height = Cm(9), Cm(5.1), Cm(0.5), Cm(0.4)
extract = slide.shapes.add_shape(MSO_SHAPE.FLOWCHART_EXTRACT, left, top, width, height)
extract.fill.solid()
extract.fill.fore_color.rgb = RGBColor(255, 0, 0)
extract.line.color.rgb = RGBColor(255, 0, 0)
new_paragraph = slide.shapes.add_textbox(left=left - Cm(0.95), top=top + Cm(0.4), width=Cm(2.4),
height=Cm(1.1)).text_frame
content = """2020/01/05
内容2"""
new_paragraph.paragraphs[0].text = content # 文字内容
new_paragraph.paragraphs[0].font.size = Pt(10) # 文字大小
new_paragraph.paragraphs[0].font.color.rgb = RGBColor(255, 0, 0) # 文字颜色
new_paragraph.paragraphs[0].alignment = PP_ALIGN.CENTER # 文字水平对齐方式
# 添加倒三角+间隔条+文字
left, top, width, height = Cm(5), Cm(4), Cm(0.5), Cm(0.4)
slide.shapes.add_shape(MSO_SHAPE.FLOWCHART_MERGE, left, top, width, height)
gap = slide.shapes.add_shape(MSO_SHAPE.RECTANGLE, left + Cm(0.2), top + Cm(0.5), Cm(0.05), Cm(0.5))
gap.fill.solid()
gap.fill.fore_color.rgb = RGBColor(255, 255, 255)
gap.line.color.rgb = RGBColor(255, 255, 255)
new_paragraph = slide.shapes.add_textbox(left=left - Cm(0.95), top=top - Cm(1), width=Cm(2.4),
height=Cm(1.1)).text_frame
content = """2020/01/05
内容3"""
new_paragraph.paragraphs[0].text = content
new_paragraph.paragraphs[0].font.size = Pt(10) # 文字大小
new_paragraph.paragraphs[0].alignment = PP_ALIGN.CENTER
# 添加当前时间图形
left, top, width, height = Cm(7), Cm(4), Cm(0.5), Cm(0.4)
now = slide.shapes.add_shape(MSO_SHAPE.DOWN_ARROW, left, top, width, height)
now.fill.solid()
now.fill.fore_color.rgb = RGBColor(254, 152, 47)
now.line.color.rgb = RGBColor(254, 152, 47)
ppt.save('5_2_7.pptx')
print('进度条添加完成')
效果如下:
以上模块功能可能没列举全,大家有什么希望的操作可以直接留言,我收到留言后会增加相关操作示例(若有),并对文章进行更新,谢谢大家!