类与对象
在C语言中,关注的是过程,故C语言是面向过程分析出求解问题的步骤。
而在C++中,关注的是对象,故C++是面向对象的,关注的是对象之间的交互。
#define _CRT_SECURE_NO_WARNINGS
#include <iostream>
using namespace std;
struct Student
{
void SetStudentInfo(const char* name, const char* gender, int age)
{
strcpy(_name, name);
strcpy(_gender, gender);
_age = age;
}
void PrintStudentInfo()
{
cout << _name << " " << _gender << " " << _age << " " << endl;
}
char _name[20];
char _gender[20];
int _age;
};
int main()
{
Student stu;
stu.SetStudentInfo("zhangsan", "mail", 18);
stu.PrintStudentInfo();
system("pause");
return 0;
}
1、类的定义
用class关键字来定义一个类,ClassName为类的名称,{}为类体,由成员函数和成员变量构成。
类中数据称为类的属性或者成员变量;
类中的函数称为类的方法或者成员函数
#include <iostream>
using namespace std;
class ClassName
{
//类体:由成员函数和成员变量组成
};//大括号的结尾必须加分号
//例如:
class Person
{
public:
void ShowInfo()
{
cout << _name << " " << _sex << " " << _age << endl;
}
public:
char* _name;
char* _sex;
int _age;
};
int main()
{
system("pause");
return 0;
}
类定义的两种方式:
1、声明和定义全部放在类体中,需要注意的是:成员函数如果在类中定义,编译器可能会将其当成内联函数来处理
2、声明在.h文件中,类的定义放在.cpp文件中
2、类的作用域
类定义了一个新的作用域,类的所有成员都在类的作用域中。在类体外定义成员需要用::作用域解析符指明成员属于哪个类域。
#include <iostream>
using namespace std;
class Person
{
public:
void PrintPersonInfo();
private:
char* _name;
char* _sex;
int _age;
};
void Person::PrintPersonInfo()
{
cout << _name << " " << _sex << " " << _age << endl;
}
int main()
{
system("pause");
return 0;
}
3、类的实例化
类的实例化
1、定义了一个类并没有给这个类分配实际的内存空间来存储它
2、一个类可以实例化多个对象,实例化出对象,占用实际的物理空间,存储类成员变量
3、类是对象的抽象,对象是类的具体表现
#include <iostream>
using namespace std;
class Student
{
public:
void PrintStudentInfo()
{
cout << _name << " " << _gender << " " << _age << " " << endl;
}
public:
char* _name;
char* _gender;
int _age;
};
int main()
{
Student stu;
stu._name = "zhangsan";
stu._gender = "mail";
stu._age = 10;
stu.PrintStudentInfo();
system("pause");
return 0;
}
4、类的访问限定符及封装
4.1、类的访问限定符
类的访问限定符
public 公有的
protected 受保护的
private 私有的
注:
1、public修饰额成员在类外可以直接被访问
2、protected和private修饰的成员不能直接被访问
3、访问权限从当前作用域到下一个访问限定符截止
4、class的默认访问权限为private struct为了兼容C 默认访问限定符为public。
4.2、封装
封装是指将数据和方法有机结合,隐藏对象的属性和实现细节,仅对外公开接口来和对象进行交互
封装的本质是一种管理
5、类的对象大小计算
5.1、类的大小
一个类的大小就是该类的成员变量的大小之和,要计算成员变量的大小,就要采用内存对齐的规则来计算。
5.2、内存对齐规则
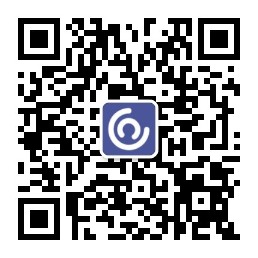
1、第一个成员在结构体偏移量为0的位置
2、其他成员变量要对齐到某个数字(对齐数)的整数倍的地址处
3、对齐数 = min(默认对齐数, 该成员大小)VS默认对齐数为8,gcc默认对齐数为4
4、结构体总的大小 = min(所有变量最大者, 默认对齐数)的整数倍
5、如果嵌套了结构体,则嵌套的结构体对齐到自己的最大对齐数的整数倍处,结构体整体的大小就是所有最大对齐数的整数倍
#include <iostream>
using namespace std;
class A1
{
public:
void FUN()
{
}
private:
int _a;
};
class A2
{
public:
void FUN()
{
}
};
class A3
{
};
struct A4
{
public:
void FUN()
{
}
private:
int _a;
};
struct A5
{
public:
void FUN()
{
}
};
struct A6
{
};
int main()
{
cout << sizeof(A1) << endl;
cout << sizeof(A2) << endl;
cout << sizeof(A3) << endl;
cout << sizeof(A4) << endl;
cout << sizeof(A5) << endl;
cout << sizeof(A6) << endl;
system("pause");
return 0;
}
6、类的成员函数的this指针
6.1、this指针的定义
C++编译器给每个成员函数增加了一个隐藏的指针参数,让该指针指向当前对象(函数运行时调用该函数的对象),在函数体中所有成员变量的操作都是通过该指针去访问,操作对用户透明,编译器自动完成
#include <iostream>
using namespace std;
class Date
{
public:
void Display()
{
cout << _year << " " << _month << " " << _day << endl;
}
void SetDate(int yeay, int month, int day)
{
_year = yeay;
_month = month;
_day = day;
}
private:
int _year;
int _month;
int _day;
};
int main()
{
Date d1, d2;
d1.SetDate(2020, 1, 24);
d2.SetDate(2020, 3, 26);
d1.Display();
d2.Display();
system("pause");
return 0;
}
6.2、this指针的特性
1、this指针是:类类型* const
2、只能在成员函数内部使用
3、this指针的本质是一个成员函数的形参,是对象调用成员函数的时候,将对象地址作为实参传递给this形参,多以对象中不存储this指针。
4、this指针是成员函数第一个隐含的指针形参,一般情况有编译器通过ecx寄存器自动传递,不需要用户传递。
#include <iostream>
using namespace std;
class A
{
public:
void PrintA()
{
cout << _a << endl;
}
void Show()
{
cout << "Show()" << endl;
}
private:
int _a;
};
int main()
{
A* a = nullptr;
a->PrintA();
a->Show();
system("pause");
return 0;
}