Catch That Cow
Time Limit: 2000 ms Memory Limit: 65536 KiB
Problem Description
Farmer John has been informed of the location of a fugitive cow and wants to catch her immediately. He starts at a point N (0 ≤ N ≤ 100,000) on a number line and the cow is at a point K (0 ≤ K ≤ 100,000) on the same number line. Farmer John has two modes of transportation: walking and teleporting. * Walking: FJ can move from any point X to the points X - 1 or X + 1 in a single minute * Teleporting: FJ can move from any point X to the point 2 × X in a single minute. If the cow, unaware of its pursuit, does not move at all, how long does it take for Farmer John to retrieve it?
Input
Line 1: Two space-separated integers: N and K
Output
Line 1: The least amount of time, in minutes, it takes for Farmer John to catch the fugitive cow.
Sample Input
5 17
Sample Output
4
Hint
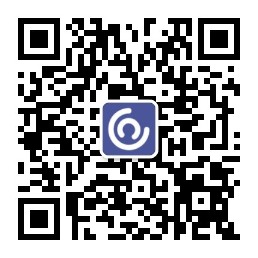
题目大意
在一条笔直的道路上,农民要捉牛,给出农民和牛的位置,牛不动,农民有三种方式可以走,例如农民在5,牛在17. 农民可以倍数级走,5*2。可以向前走一步5+1, 向后走一步5-1,每一种方式都花费一分钟,问最少花费的时间可以捉到牛。
思路
这是一道典型的广搜题,核心是使用一个一维数组,用它的下标记录农民所在位置,用它下标所对应的值来记录它所花费的时间,将每次的下标入队这里不用循环是因为农民三次的移动判断条件互不相同,每到一个位置,就将上个位置所有的时间加1赋值给它。
#include <iostream>
#include <queue>
#include <stdlib.h>
#include <string.h>
#include <stdio.h>
using namespace std;
int step[100005], visit[100005];
/// 到某点的步数 标记数组
void Init_gra(int start, int end) ///初始化
{
memset(step,0,sizeof(step));
memset(visit,0,sizeof(visit));
}
int BFS(int start, int end) ///BFS遍历
{
queue<int>q; ///借助队列
int h, e; ///h为暂存记录变量,存每次循环队列头需要处理的元素
int i; ///循环控制变量
q.push(start); ///把起始点压入队列
visit[start] = 1; ///起始点标为已访问
while(!q.empty()) ///BFS核心循环
{
h = q.front(); ///暂存
q.pop(); ///出队列
for(i = 0; i < 3; i++) ///三种操作,每种都试一下
{
if(i == 0)
{
e = h-1;
}
else if(i == 1)
{
e = h+1;
}
else
{
e = h * 2;
}
if(e > 100000 || e < 0 || visit[e] != 0) ///若越界或者已经访问则此点不可能是路径上的一个点
continue;
if(visit[e] == 0) ///若此点未被访问且未越界
{
q.push(e); ///将此点压入
step[e] = step[h] + 1; ///到达此点的步数是上一个节点+1
visit[e] = 1; ///标记为已访问
}
if(e == end) ///若当前访问的节点恰好为目标点,则返回到达此节点的步数
{
return step[e];
}
}
}
}
int main()
{
int start, pos;
scanf("%d %d", &start, &pos);
if(start >= pos)
{
printf("%d\n", start - pos); ///因为是有向图,每个节点只能指向x-1,x+1和2x处,若起点在目标点之后,则只需一个一个往后走
}
else
{
printf("%d\n", BFS(start,pos)); ///一般情况,跑一下BFS
}
return 0;
}