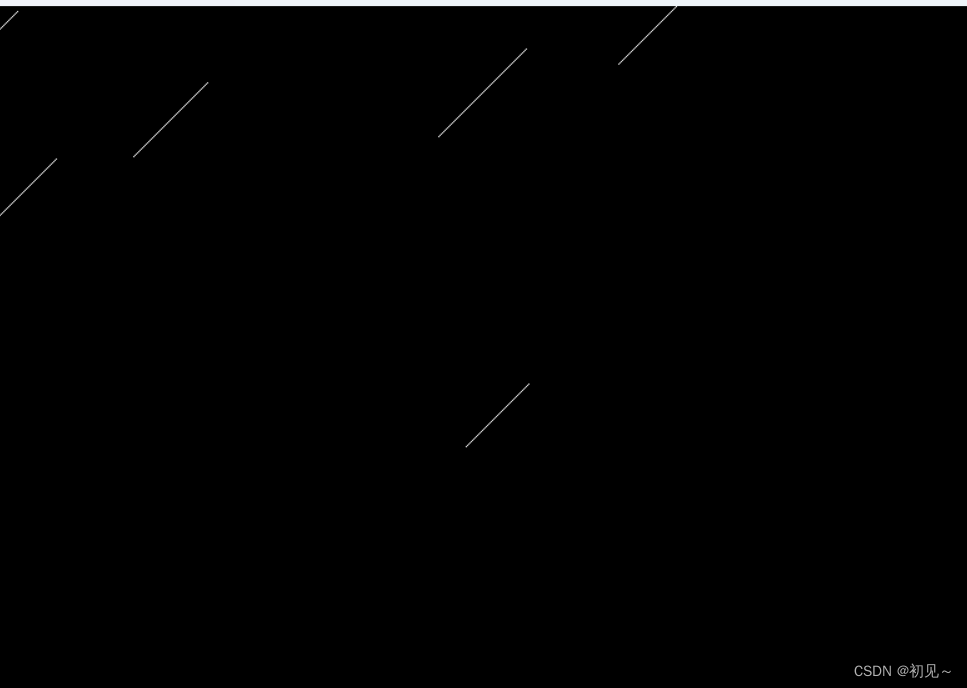
Importe las bibliotecas necesarias, incluidas pygame, random y sys;
Respecto a pygame, si estás interesado puedes leer este artículo: Python - Una breve charla sobre Pygame
import pygame
import random
import sys
Inicialice la biblioteca pygame;
pygame.init()
Definir constantes, incluido el tamaño de la pantalla, la cantidad de meteoros, la velocidad máxima de los meteoros, la longitud máxima de los meteoros, la longitud mínima de los meteoros, etc.;
Aquí definimos el tamaño de la pantalla como 800x600, el número de meteoros como 10, la velocidad máxima como 10, la longitud máxima como 80 y la longitud mínima como 40.
SCREEN_WIDTH = 800
SCREEN_HEIGHT = 600
NUM_METEORS = 10
MAX_SPEED = 10
MAX_LENGTH = 80
MIN_LENGTH = 40
Definir la clase de meteoro, incluidos atributos como posición, velocidad, longitud y métodos para dibujar y actualizar meteoros;
class Meteor:
def __init__(self):
self.x = random.randint(0, SCREEN_WIDTH)
self.y = random.randint(-SCREEN_HEIGHT, 0)
self.speed = random.randint(1, MAX_SPEED)
self.length = random.randint(MIN_LENGTH, MAX_LENGTH)
def draw(self, surface):
pygame.draw.line(surface, (255, 255, 255), (self.x, self.y), (self.x - self.length, self.y + self.length))
def update(self):
self.x -= self.speed
self.y += self.speed
if self.x < -self.length or self.y > SCREEN_HEIGHT + self.length:
self.__init__() # 如果流星超出屏幕,重新初始化流星属性
En el código anterior, definimos una clase Meteor, que incluye la posición, velocidad, longitud y otros atributos del meteoro, así como métodos para dibujar y actualizar meteoros. En el método de inicialización __init__, generamos aleatoriamente la posición, velocidad y longitud del meteoro. En el método de dibujo, utilizamos el método draw.line de la biblioteca pygame para dibujar el meteoro. En el método de actualización, actualizamos la posición del meteoro en función de su velocidad y posición. Si el meteoro sale de la pantalla, reiniciamos las propiedades del meteoro.
Defina la función principal, incluidas funciones como crear meteoros, actualizar meteoros y dibujar meteoros;
# 创建流星
meteors = []
for i in range(NUM_METEORS):
meteors.append(Meteor())
# 创建屏幕
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
# 主函数
def main():
# 循环调用更新和绘制流星的方法
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
screen.fill((0, 0, 0)) # 填充屏幕颜色
for meteor in meteors:
meteor.update()
meteor.draw(screen)
pygame.display.update() # 更新屏幕显示
# 调用主函数
if __name__ == '__main__':
main()
En el código anterior, primero creamos NUM_METEORS meteoros y los agregamos a la lista de meteoros. Luego creamos un objeto de pantalla y recorrimos la función principal para llamar a los métodos para actualizar y dibujar el meteoro. En cada bucle, primero llenamos la pantalla de negro, luego actualizamos la posición de cada meteoro y dibujamos el meteoro. Finalmente, llame al método pygame.display.update() para actualizar la visualización de la pantalla.
Salga de la biblioteca de pygame.
pygame.quit()
sys.exit()
Finalmente, debemos salir de la biblioteca pygame cuando finalice el programa.
El código completo es el siguiente:
import pygame
import random
import sys
# 初始化pygame
pygame.init()
# 定义常量
SCREEN_WIDTH = 800
SCREEN_HEIGHT = 600
NUM_METEORS = 10
MAX_SPEED = 10
MAX_LENGTH = 80
MIN_LENGTH = 40
# 定义流星类
class Meteor:
def __init__(self):
self.x = random.randint(0, SCREEN_WIDTH)
self.y = random.randint(-SCREEN_HEIGHT, 0)
self.speed = random.randint(1, MAX_SPEED)
self.length = random.randint(MIN_LENGTH, MAX_LENGTH)
def draw(self, surface):
pygame.draw.line(surface, (255, 255, 255), (self.x, self.y), (self.x - self.length, self.y + self.length))
def update(self):
self.x -= self.speed
self.y += self.speed
if self.x < -self.length or self.y > SCREEN_HEIGHT + self.length:
self.__init__()
# 创建流星
meteors = []
for i in range(NUM_METEORS):
meteors.append(Meteor())
# 创建屏幕
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
# 主函数
def main():
# 循环调用更新和绘制流星的方法
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
screen.fill((0, 0, 0))
for meteor in meteors:
meteor.update()
meteor.draw(screen)
pygame.display.update()
# 调用主函数
if __name__ == '__main__':
main()
# 退出pygame
pygame.quit()
sys.exit()