2.1 Initialization
Flask All applications must create an application instance .
Web server using the Web server called Gateway Interface (WSGI, Web server gateway interface, pronounced "wiz-ghee") protocol, all the requests received from the client are transferred to the target process.
Flask application example is the object class, code that is created by the following
from flask import Flask
app = Flask(__name__) #Flask为一个类
Flask name class constructor must specify only one parameter, i.e. the application of the main module or package. In most cases, Python's __name__ variable is the value you want. Flask __name__ use to determine the location of the application, and then find the application location of other files, such as images and templates
2.2 Routing and view function
The client (e.g., a Web browser) sends a request to the Web server, Web server sends the request to the application instance Flask. Application examples need to know to run that code for each request url, it contains mappings url to Python function. Program dealing with the relationship between the URL and the function is called routing
@app.route('/') # 使用app.route装饰器来定义路由
def index(): # 把index()函数注册为应用根地址的处理程序
return '<h1>Hello World!</h1>'
def index(): # 将index函数注册为应用根地址的处理程序
return'<h1>Hello World!</h1>'
app.add_url_rule('/','index',index) # app.add_url_rule()接收3个参数:URL,端点名,视图函数
index () function thus processed is referred to as inbound requests view function . If the application www.bilibili.com server deployments in the domain name, after visiting http://www.bilibili.com in the browser, the server will trigger the execution index () function. The return value of this function is called in response, the content is received by the client. If the client is a Web browser, the response is displayed to the user to view the document. View response may be a simple function returns a string containing the HTML.
@app.route('/user/<name>') # 定义的路由有一部分是可变的
def user(name):
return '<h1>Hello,{}!</h1>'.format(name) # 路由URL在{}的内容就是动态部分
When you call the view function, Flask will be part of a dynamic parameter as an argument. The name parameter is used to generate a personalized welcome message.
Dynamic routing section default string, but also for others. The routing / User / int: id will match the dynamic URL fragment id is an integer, such as / user / 123. Flask support routing using string, int, float, and the type of path (path of a particular string, may comprise special is that forward slash)
Decorator is a standard feature of the Python language. Common usage is to be registered as a function of time handler, used when a particular event occurs
2.4 Web development server
Flask own Web application development server, start by flask run command. This command FLASK_APP environment variables specified Python script to find application examples
Flask Web server provides only for development and testing.
Flask Web development server can also be started programmatically: Call app.run () method
2.5 Dynamic Routing
Adding a dynamic routing. Visit China dynamic URL in your browser, you'll see a personalized message
hello.py : Flask application containing dynamic routing
from flask import Flask
app = Flask(__name__)
@app.route('/')
def index():
return '<h1>Hello World!</h1>'
@app.route('/user/<name>')
def user(name):
return '<h1>Hello,{}!</h1>'.format(name)
2.6 debug mode
Flask application can run in debug mode, in this mode, the default development server will load two tools: heavy-duty and debugger.
When enabled device reload, all the source files Flask will monitor the project and found that changes automatically restart the server. Running start overloading the server on especially convenient during development, because every change and save the source file after the server will automatically restart, let the changes to take effect
The debugger is a Web-based tool, when applied unhandled exception is thrown, it will appear in the browser. At this time, Web browser into an interactive stack trace, you can, evaluate expressions anywhere in the call stack inside the source code review
Debug mode is disabled by default, set FLASK_DEBUG before performing the flask run = 1 to enable the debugger
2.7 Command Line Options
flask --help or flask can see those options that can be used
flask shell open a Python shell session in the application context, you can run maintenance tasks or testing session, you can also debug the problem
flask run running applications in Web development server
flask run - host tells the server to listen for client connections sent on the network interface
connection Flask Web server listens on localhost, so the server will only accept connect the computer running the server to send the
flask run --host 0.0.0.0 make public Web server listens connected to the network interface
2.8 a request - response cycle
2.8.1 Application Context Request
flask receives a request from a client, let the view function can access some objects, in order to process the request. Http request object encapsulates a request sent by the client
In order to avoid the large number of parameters to view function mess, Flask temporary context using the globally accessible object becomes certain. There are such a context can write a view function:
from flask import Flask
@app.route('/')
def index():
user_agent = request.headers.get('User-Agent')
return '<p>Your browser is {}</p>'.format(user_agent)
Function in this view, the request to the global variable while (in fact, not be a global variable, in multi-threaded servers, multiple threads process different requests sent by different clients, each thread sees the request object must be different. Flask make use of context-specific variables in a globally accessible thread, at the same time it does not interfere with other threads)
the Flask activation (or push) the application and request context, after the completion of the request processing to remove them before the allocation request. After the application context is pushed, and g can be used current_app variables in the current thread.
After the request context is pushed, you can request and the session variables. If the variable is not activated or the application context request context, an error.
run
from hello import app
from flask import current_app
current_app.name # 未激活应用上下文调用会失败
Export
Traceback (most recent call last):
...
-
Run
app_ctx = app.app_context() # 获取上下文,推送完上下文就可以调用
app_ctx.push()
current_app.name
Export
'hello'
-
Run
app_ctx.pop()
2.8.2 allocation request
When the application receives a request sent by the client to find the view function to process the request, flask looks url requested in the application url map. url corresponding mapping relation between the url and view function. flask or a decorative application app.route app.add_url_rule () method for constructing a mapping
from hello import app
app.url_map # 查看flask应用中的url映射
Map([<Rule '/' (OPTIONS, GET, HEAD) -> index>,
<Rule '/static/<filename>' (OPTIONS, GET, HEAD) -> static>,
<Rule '/user/<name>' (OPTIONS, GET, HEAD) -> user>])
/ And / user / routing uses app.route defined in the application
/ static / special routing flask is added for accessing static files
url map (HEAD, OPTIONS, GET) request method is processed by a routing
flask for each route request specifies the method, so that even when the method of transmitting various requests to the same url, will use different views function processing. OPTIONS HEAD and processed by the flask.
It can be said, in this application, url mapping three routes using GET method (that the client would like to request a message such as a page)
2.8.3 request object
flask request by a context variable opening request object, this object contains all the information of the HTTP request sent by the client.
flask common attributes and methods
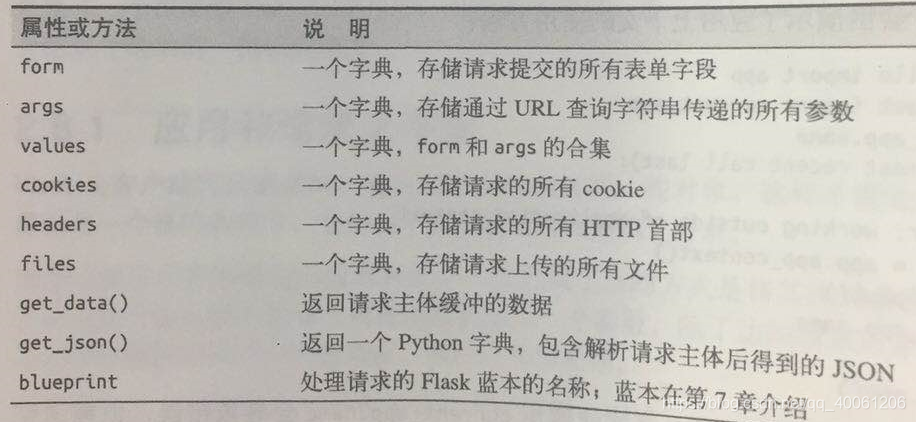
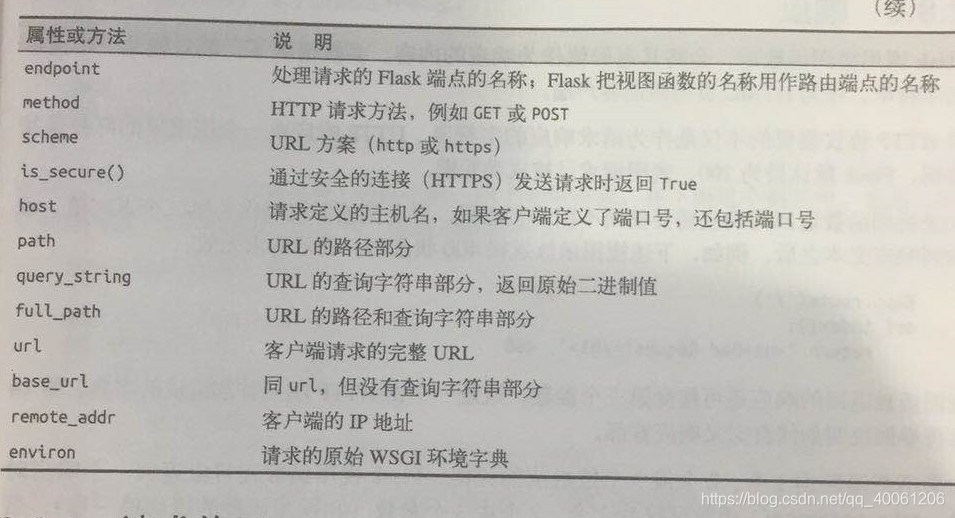
2.8.4 request hook
In order to avoid writing code are repeated in each view function, the Flask provides a registration function generic function, the function call request may be registered before or after the function assigned to the view.
Request hooks achieved by decorators.
flask supports four hooks:
- before_request
register a function that runs before every request - before_first_request
register a function, only the first operation before processing a request. You can add a server initialization tasks - after_request
registration function, if not an unhandled exception occurs, run after each request - teardown_request
registration function, even if there is an unhandled exception is thrown, also run after each request
Shared data between the request and view function hook function using general context of the global variable g
2.8.5 response
Flask rear view function call, returns it to the value of the content as a response. In most cases, the response is a simple string, as a html page loopback client.
However, the HTTP protocol requires more than just a response request string, it is important that the HTTP status code, Flask default is 200, indicating that the request has been successfully processed
If the view function returns require different response status code, a numeric code can be returned as the second value, the response text to be added after.
make_response () is acceptable, two, or three parameters (return values and as a function of the view), and then returns a response object equivalent. Sometimes you need to generate a response in view of the function object, and then calling various methods on the response object, set the response further
redirect page document did not respond, just tell the browser to a new url, to load a new page.
Redirection status code 302 is typically provided in the header portion url target location, the redirect response may be used in the form of three values of return value generator, you may be set in the response object.
flask provided redirect () function may generate a redirect response:
from flask import redirect
@app.route('/')
def index():
return redirect('http://www.bilibili.com')
abort () function is used to handle errors
from flask import abort
@app.route('/user/<id>')
def get_user(id):
user = load_user(id)
if not user:
abort(404) # 若url中动态参数id对应的用户不存在,则返回状态码404,abort()不会把控制权交给调用函数,而是抛出异常
return '<h1>Hello,{}</h1>'.format(user.name)