Article directory
1. Preface to the article
The main functions of this article include: login and registration of the sports and health platform, understand health knowledge, view articles and details about sports management, daily login and check-in, system notifications, message management, and submit sports functions. Using Java as the back-end language for support, the interface is friendly and development is simple.
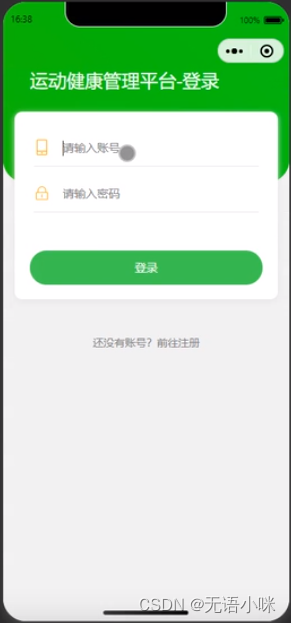
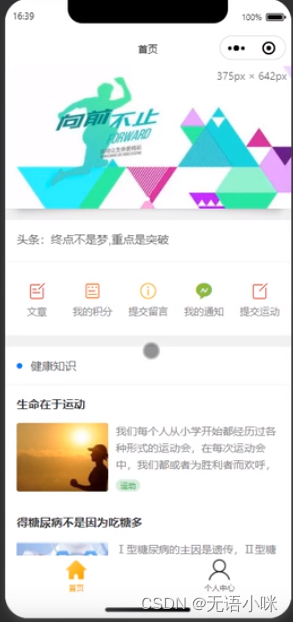
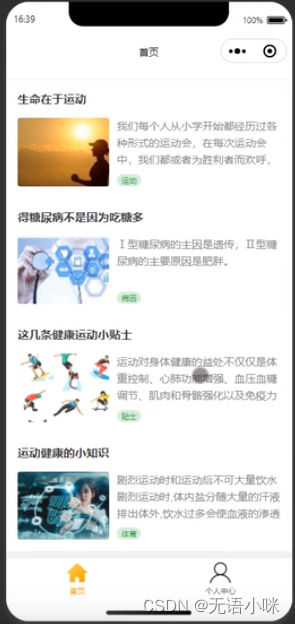
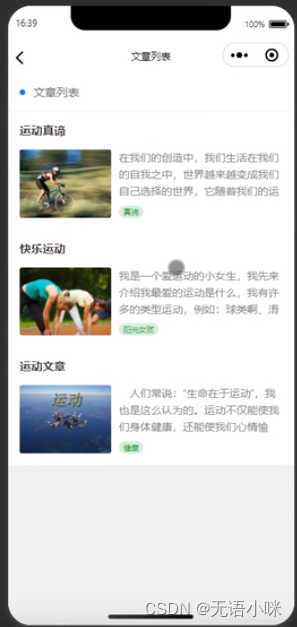
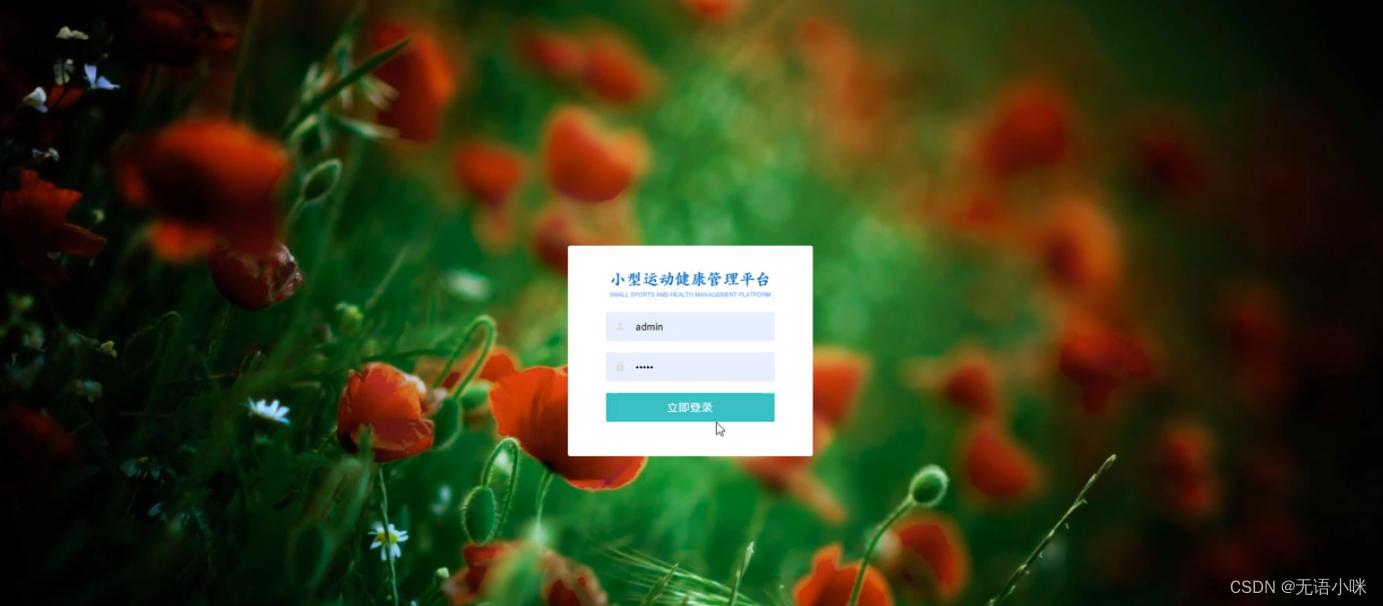
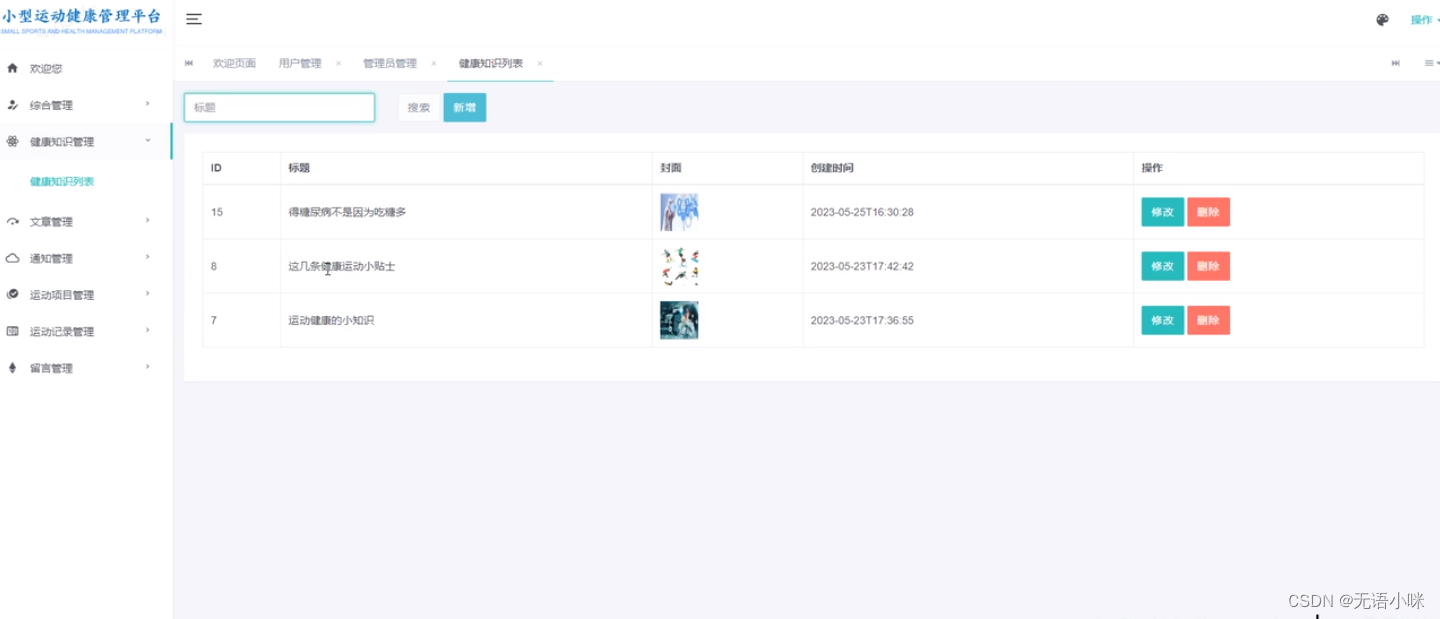
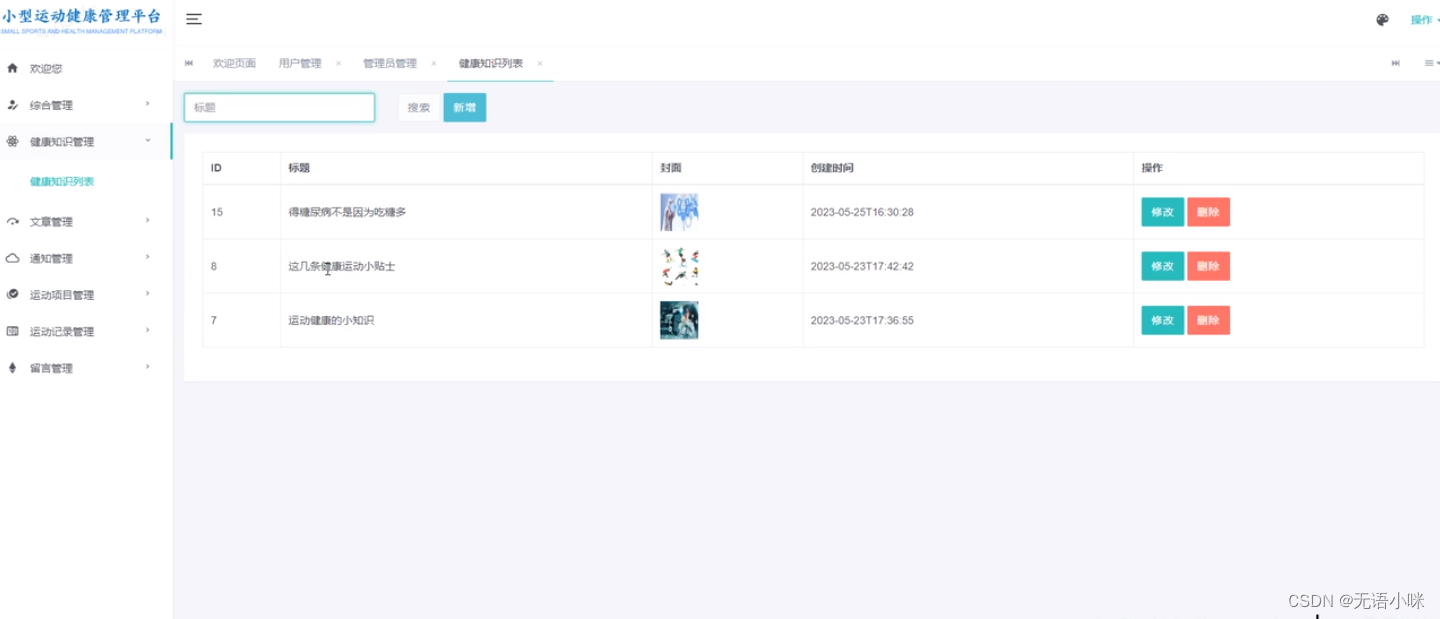
2. Development process and tool preparation
2.1. Download and install IntelliJ IDEA (back-end language development tool), Mysql database, and WeChat Web developer tools.
3. Development steps
1.Create maven project
First create a project named SpringBootDemo and select [New Project]
Then in the pop-up window below, select [New Project] on the left menu (Note: Unlike the idea version before 2022, there is no [Maven] option on the left here, do not select [Maven Archetype]!!!), enter Name (project name): SpringBootDemo, select [java] for language, select [maven] for build system, and then select jdk. I chose jdk18 here.
Then click [Create]
2. Create module under project
Right-click and select [new] - [Module...]
on the left side and select [Spring initializr] to quickly create a spring boot project through the Spring initializr tool integrated in idea. On the right side of the window: name can be set according to your own preferences, group and artifact have the same rules as above, other options can keep their default values, [next]
Check [Spring Boot DevTools] for the Developer Tools module, and check [Spring Web] for the web module.
At this point, a Springboot project has been built and subsequent functions can be developed.
3. Write a motion entity class, Mapper, and service (three-tier architecture)
@Data
public class Motion {
//运动记录id
@TableId(type = IdType.AUTO)
private Long id;
//运动类型id
private Integer typeId;
//类型
private Integer type;
//用户id
private Long userId;
//运动分数
private int num;
//创建使劲
private LocalDateTime createTime;
//运动内容
private String content;
}
Since we use mybatis-plus, we don’t need to write simple additions, deletions, modifications and queries ourselves. The framework comes with it. We only need to implement or inherit its Mapper and Service.
4. Write the motion management Controller class
@RestController
@RequestMapping("motion")
public class MotionController {
@Autowired
private MotionMapper motionMapper;
@Autowired
private MotionTypeMapper motionTypeMapper;
@Autowired
private UserMapper userMapper;
//查询列表
@PostMapping("selectPage")
public Map selectPage(@RequestBody Motion motion, Integer pageSize, Integer pageNum) {
ReturnMap returnMap = new ReturnMap();
//分页需要的Page
Page<Motion> page = new Page<>();
page.setCurrent(pageNum + 1);
page.setSize(pageSize);
QueryWrapper<Motion> queryWrapper = new QueryWrapper<>();
//可根据条件模糊查询
Page<Motion> selectPage = motionMapper.selectPage(page, queryWrapper.lambda()
.eq(motion.getTypeId() != null, Motion::getTypeId, motion.getTypeId())
.orderByDesc(Motion::getCreateTime));
List<Motion> list = selectPage.getRecords();
for (Motion data : list) {
MotionType motionType = motionTypeMapper.selectById(data.getTypeId());
data.setTypeName(motionType != null ? motionType.getTitle() : "");
User user = userMapper.selectById(data.getUserId());
data.setUserName(user != null ? user.getNickname() : "");
}
selectPage.setRecords(list);
returnMap.setData("page", selectPage);
return returnMap.getreturnMap();
}
//查询用于运动积分查询列表
@PostMapping("list")
public Map selectPage(Long userId) {
ReturnMap returnMap = new ReturnMap();
QueryWrapper<Motion> queryWrapper = new QueryWrapper<>();
List<Motion> list = motionMapper.selectList
(queryWrapper.lambda().eq(Motion::getUserId, userId).orderByDesc(Motion::getCreateTime));
int integralSum = 0;
for (Motion motion1 : list) {
MotionType motionType = motionTypeMapper.selectById(motion1.getTypeId());
if (motion1.getType() == 1) {
motion1.setTitle("签到");
} else {
motion1.setTitle(motionType != null ? motionType.getTitle() : "");
}
motion1.setTypeName(motionType != null ? motionType.getTitle() : "");
SimpleDateFormat simpleDateFormat = new SimpleDateFormat("yyyy-MM-dd");
motion1.setTimeCreate(simpleDateFormat.format(Date.from(motion1.getCreateTime().atZone(ZoneId.systemDefault()).toInstant())));
integralSum += motion1.getNum();
}
returnMap.setData("integralSum", integralSum);
returnMap.setData("list", list);
return returnMap.getreturnMap();
}
Because you want to write a Rest-style API, you need to mark the @RestController annotation on the Controller.
5. Write code related to small programs
Article list:
<view class="cu-bar bg-white solid-bottom">
<view class="action">
<text class="cuIcon-title text-blue"></text>文章列表
</view>
</view>
<view class="cu-card article no-card " wx:for="{
{articleList}}" wx:key="{
{index}}" bindtap="showModal" data-target="Modal" data-index="{
{index}}">
<view class="cu-item shadow">
<view class="title">
<view class="text-cut">{
{
item.title}}</view>
</view>
<view class="content">
<image src="{
{item.image}}" mode="aspectFill"></image>
<view class="desc">
<view class="text-content">{
{
item.content}}</view>
<view>
<view class="cu-tag bg-green light sm round">{
{
item.icon}}</view>
</view>
</view>
</view>
</view>
</view>
Sign-in function:
<view class="cu-bar bg-white solid-bottom">
<view class="action">
<text class="cuIcon-title text-green"></text>当前积分:{
{
integralSum}}
</view>
<view class="action" bindtap="signIn" >
<text class="cuIcon-roundadd text-green">签到</text>
</view>
</view>
<view class="cu-list menu {
{menuBorder?'sm-border':''}} {
{menuCard?'card-menu margin-top':''}}">
<view class="cu-item" wx:for="{
{integralRecord}}" wx:key="{
{index}}">
<view class="content padding-tb-sm">
<view>
<text class="cuIcon-footprint text-green margin-right-xs"></text> {
{
item.title}}</view>
<view class="text-gray text-sm">
<text class="cuIcon-time margin-right-xs"></text> {
{
item.timeCreate}}</view>
</view>
<view class="action">
+{
{
item.num}}
</view>
</view>
</view>
This project is friendly to beginners who are new to the Springboot framework, and also friendly to those who are new to small programs. Since the project resources are too large, you can privately message the blogger to obtain the project.