Table of contents
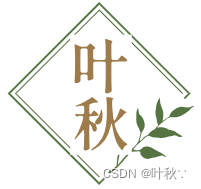
introduction
In modern web development, SpringMVC is a widely used framework that provides rich functionality and flexible configuration options. This article will delve into two important topics: JSON data return and exception handling mechanism in SpringMVC. We will gradually introduce the relevant configuration and usage methods, and deepen understanding through cases and comprehensive examples.
1. JSON data return in SpringMVC
1.1 Import dependencies
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.9.3</version>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-core</artifactId>
<version>2.9.3</version>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-annotations</artifactId>
<version>2.9.3</version>
</dependency>
1.2 Configure spring-MVC.xml
In order for SpringMVC to correctly handle JSON data return, we need to configure it accordingly in the configuration file. In order to correctly handle requests returned by JSON data.
<bean class="org.springframework.web.servlet.mvc.method.annotation.RequestMappingHandlerAdapter">
<property name="messageConverters">
<list>
<ref bean="mappingJackson2HttpMessageConverter"/>
</list>
</property>
</bean>
<bean id="mappingJackson2HttpMessageConverter"
class="org.springframework.http.converter.json.MappingJackson2HttpMessageConverter">
<!--处理中文乱码以及避免IE执行AJAX时,返回JSON出现下载文件-->
<property name="supportedMediaTypes">
<list>
<value>text/html;charset=UTF-8</value>
<value>text/json;charset=UTF-8</value>
<value>application/json;charset=UTF-8</value>
</list>
</property>
</bean>
1.3 Use of @ResponseBody annotation
@ResponseBody
The function of the annotation is to convert the object returned by the Controller method into the specified format through an appropriate converter, and then write it into the body area of the response object. It is usually used to return data JSON
or XML data.
Note: After using this annotation, the view parser will not be used , but the data will be written directly to the input stream. Its effect is equivalent to outputting data in the specified format through the response object.
<select id="selBySnamePager" resultType="com.yuan.model.Student" parameterType="com.yuan.model.Student" >
select
<include refid="Base_Column_List" />
from t_mysql_student
<where>
<if test="sname!=null">
and sname like concat('%',#{sname},'%')
</if>
</where>
</select>
<select id="mapListPager" resultType="java.util.Map" parameterType="com.yuan.model.Student" >
select
<include refid="Base_Column_List" />
from t_mysql_student
<where>
<if test="sname != null">
and sname like concat('%',#{sname},'%')
</if>
</where>
</select>
/**
* 返回List<T>
* @param req
* @param student
* @return
*/
@ResponseBody
@RequestMapping("/list")
public List<Student> list(HttpServletRequest req, Student student){
PageBean pageBean = new PageBean();
pageBean.setRequest(req);
List<Student> lst = this.studentBiz.selBySnamePager(student, pageBean);
return lst;
}
/**
* 返回T
* @param req
* @param Student
* @return
*/
@ResponseBody
@RequestMapping("/load")
public Student load(HttpServletRequest req, Student Student){
if(Student.getSname() != null){
List<Student> lst = this.studentBiz.selBySnamePager(Student, null);
return lst.get(0);
}
return null;
}
/**
* 返回List<Map>
* @param req
* @param Student
* @return
*/
@ResponseBody
@RequestMapping("/mapList")
public List<Map> mapList(HttpServletRequest req, Student Student){
PageBean pageBean = new PageBean();
pageBean.setRequest(req);
List<Map> lst = this.studentBiz.mapListPager(Student, pageBean);
return lst;
}
/**
* 返回Map
* @param req
* @param Student
* @return
*/
@ResponseBody
@RequestMapping("/mapLoad")
public Map mapLoad(HttpServletRequest req, Student Student){
if(Student.getSname() != null){
List<Map> lst = this.studentBiz.mapListPager(Student, null);
return lst.get(0);
}
return null;
}
@ResponseBody
@RequestMapping("/all")
public Map all(HttpServletRequest req, Student Student){
PageBean pageBean = new PageBean();
pageBean.setRequest(req);
List<Student> lst = this.studentBiz.selBySnamePager(Student, pageBean);
Map map = new HashMap();
map.put("lst",lst);
map.put("pageBean",pageBean);
return map;
}
@ResponseBody
@RequestMapping("/jsonStr")
public String jsonStr(HttpServletRequest req, Student Student){
return "clzEdit";
}
- @JsonIgnore hides an attribute in json data
@ToString
public class Student {
@JsonIgnore
@NotBlank(message = "编号不能为空")
private String sid;
@NotBlank(message = "名字不能为空")
private String sname;
@NotBlank(message = "年龄不能为空")
private String sage;
@NotBlank(message = "性别不能为空")
private String ssex;
1.4.Jackson
- 1.4.1. Introduction
Jackson is a simple Java-based application library. Jackson can easily convert Java objects into json objects and xml documents. It can also convert json and xml into Java objects. Jackson relies on fewer jar packages, is easy to use and has relatively high performance. The Jackson community is relatively active and the update speed is relatively fast.
Features
- Easy to use, it provides a high-level look and feel that simplifies common use cases.
- There is no need to create a mapping, the API provides default mappings for most object serialization.
- High performance, fast, low memory usage
- Create clean json
- Does not depend on other libraries
- Code open source
1.4.2. Commonly used annotations
annotation | illustrate |
---|---|
@JsonIgnore | Acts on fields or methods to completely ignore the attributes corresponding to the annotated fields and methods. |
@JsonProperty | Acts on fields or methods and is used to serialize/deserialize attributes. It can be used to avoid missing attributes and provide renaming of attribute names. |
@JsonIgnoreProperties | Acts on classes to indicate that some attributes need to be ignored during serialization/deserialization. |
@JsonUnwrapped | Acts on property fields or methods, used to add properties of child JSON objects to the enclosing JSON object |
@JsonFormat | Specifies the format when serializing date/time values |
2. Exception handling mechanism
2.1 Why global exception handling is needed
Exception handling is an important development task that can help us better handle errors and exceptions in our programs. This section will introduce why we need a global exception handling mechanism and discuss its importance and advantages.
2.2 Exception handling ideas
Exceptions in the system's dao, service, and controller are all thrown upward through throws Exception. Finally, the springmvc front-end controller hands it over to the exception handler for exception handling. Springmvc provides a global exception handler (a system has only one exception handler) for unified exception handling.
2.3 SpringMVC exception classification
- Use the simple exception handler SimpleMappingExceptionResolver provided by Spring MVC;
- Implement Spring's exception handling interface HandlerExceptionResolver to customize your own exception handler;
- 使用@ControllerAdvice + @ExceptionHandler
2.4 Comprehensive case
Through a comprehensive case, we will gain an in-depth understanding of how to handle exceptions in SpringMVC. This section will introduce a practical case and demonstrate how to use the global exception handling mechanism to handle exceptions.
- Exception handling method one
SpringMVC comes with an exception handler called SimpleMappingExceptionResolver, which implements the HandlerExceptionResolver interface. All global exception handlers need to implement this interface.
<!-- springmvc提供的简单异常处理器 -->
<bean class="org.springframework.web.servlet.handler.SimpleMappingExceptionResolver">
<!-- 定义默认的异常处理页面 -->
<property name="defaultErrorView" value="error"/>
<!-- 定义异常处理页面用来获取异常信息的变量名,也可不定义,默认名为exception -->
<property name="exceptionAttribute" value="ex"/>
<!-- 定义需要特殊处理的异常,这是重要点 -->
<property name="exceptionMappings">
<props>
<prop key="java.lang.RuntimeException">error</prop>
</props>
<!-- 还可以定义其他的自定义异常 -->
</property>
</bean>
- error.jsp
<%--
Created by IntelliJ IDEA.
User: yuanh
Date: 2023/9/13
Time: 16:07
To change this template use File | Settings | File Templates.
--%>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>Title</title>
</head>
<body>
异常
${ex}
</body>
</html>
- Exception code
-
Abnormal operation effects
-
Exception handling method two
GlobalException
package com.yuan.exception;
public class GlobalException extends RuntimeException {
public GlobalException() {
}
public GlobalException(String message) {
super(message);
}
public GlobalException(String message, Throwable cause) {
super(message, cause);
}
public GlobalException(Throwable cause) {
super(cause);
}
public GlobalException(String message, Throwable cause, boolean enableSuppression, boolean writableStackTrace) {
super(message, cause, enableSuppression, writableStackTrace);
}
}
GlobalExceptionHandler
package com.yuan.Component;
import com.yuan.exception.GlobalException;
import org.springframework.stereotype.Component;
import org.springframework.web.servlet.HandlerExceptionResolver;
import org.springframework.web.servlet.ModelAndView;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
@Component
public class GlobalExceptionHandler implements HandlerExceptionResolver {
@Override
public ModelAndView resolveException(HttpServletRequest httpServletRequest,
HttpServletResponse httpServletResponse,
Object o, Exception e) {
ModelAndView mv = new ModelAndView();
mv.setViewName("error");
if (e instanceof GlobalException){
GlobalException globalException = (GlobalException) e;
mv.addObject("ex",globalException.getMessage());
mv.addObject("msg","全局异常....");
}else if (e instanceof RuntimeException){
RuntimeException runtimeException = (RuntimeException) e;
mv.addObject("ex",runtimeException.getMessage());
mv.addObject("msg","运行时异常....");
}else {
mv.addObject("ex",e.getMessage());
mv.addObject("msg","其他异常....");
}
return mv;
}
}
Exception method
@ResponseBody
@RequestMapping("/jsonStr")
public String jsonStr(HttpServletRequest req, Student Student){
if(true)
throw new GlobalException("异常123");
return "clzEdit";
}
- Handling exception three @ControllerAdvice
package com.yuan.Component;
import com.yuan.exception.GlobalException;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.ResponseBody;
import java.util.HashMap;
import java.util.Map;
@ControllerAdvice
public class GlobalExceptionResolver {
// 跳转错误页面
// @ExceptionHandler
// public ModelAndView handler(Exception e){
// ModelAndView mv = new ModelAndView();
// mv.setViewName("error");
// if (e instanceof GlobalException){
// GlobalException globalException = (GlobalException) e;
// mv.addObject("ex",globalException.getMessage());
// mv.addObject("msg","全局异常....");
// }else if (e instanceof RuntimeException){
// RuntimeException runtimeException = (RuntimeException) e;
// mv.addObject("ex",runtimeException.getMessage());
// mv.addObject("msg","运行时异常....");
// }
// return mv;
// }
// 返回错误json数据
@ResponseBody
@ExceptionHandler
public Map handler(Exception e){
Map map = new HashMap();
if (e instanceof GlobalException){
GlobalException globalException = (GlobalException) e;
map.put("ex",globalException.getMessage());
map.put("msg","全局异常....");
}else if (e instanceof RuntimeException){
RuntimeException runtimeException = (RuntimeException) e;
map.put("ex",runtimeException.getMessage());
map.put("msg","运行时异常....");
}else {
map.put("ex",e.getMessage());
map.put("msg","其它异常....");
}
return map;
}
}
Summarize
This article takes an in-depth look at the JSON data return and exception handling mechanisms in SpringMVC. We started with configuration and usage methods, gradually introduced relevant knowledge points, and deepened our understanding through cases and comprehensive examples. I hope this article can help readers better understand and apply the JSON data return and exception handling mechanism in SpringMVC.