Class starts this week: Big news | The latest 2023 full-stack test development skills practical guide V2.0 (Issue 4)
1 Introduction
Web automated testing plays a key role in ensuring quality, improving efficiency, and accelerating software development iterations. It has become an indispensable part of modern software testing. Today I will introduce and recommend several commonly used Web automated testing tools.
2. Commonly used testing tools
Commonly used web automation testing tools include:
Selenium: Selenium is one of the most famous web automation testing tools, supporting multiple programming languages, such as Java, Python, C#, etc. It can simulate user operations in the browser and implement automated testing.
Cypress: Cypress is a modern web automation testing tool focused on end-to-end testing. It provides a simple API and rich functions, supports real-time reloading and automatic waiting, making development and debugging more efficient.
Playwright: Playwright is an automated testing tool developed by Microsoft and supports multiple browsers, including Chrome, Firefox and Safari. It provides a powerful API and rich functions, supporting multi-window and multi-tab testing.
Puppeteer: Puppeteer is a Chrome-based Node.js library that can implement automated testing by controlling the Chrome browser. It provides a rich API that can simulate user operations in the browser.
TestCafe: TestCafe is a cross-browser automated testing tool that can run test cases in various browsers. It provides a simple API and rich functionality, supporting parallel testing and remote testing.
3. Three key points:
3.1 Selenium automated testing
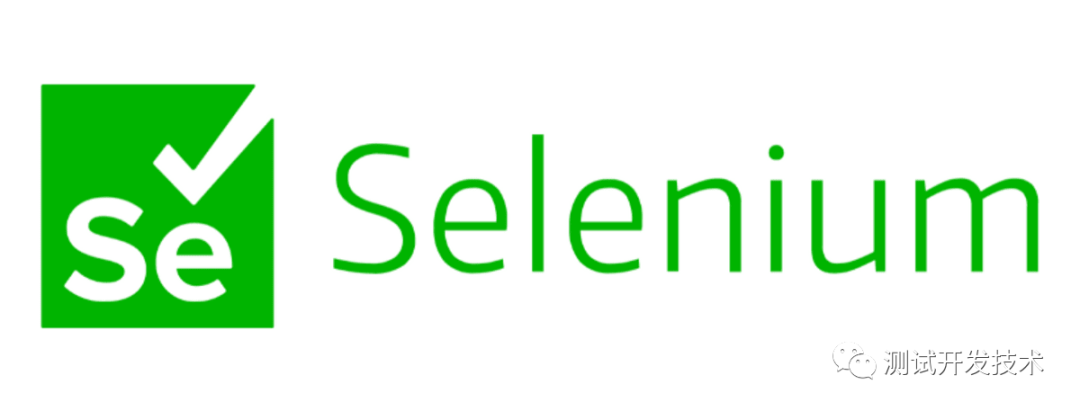
1. First, how to quickly learn Selenium from the overall perspective? You can follow the following steps:
Official website: Visit the Selenium official website (
https://www.selenium.dev/
) to learn about the latest version, documentation, API reference, sample code, etc.Learning materials: Reading official documentation is the best way to learn Selenium. The official documentation provides detailed tutorials and examples, covering all aspects of Selenium, including positioning elements, operating browsers, handling pop-ups, etc.
Programming language: Choose a programming language to learn and practice. Selenium supports multiple programming languages, such as Java, Python, C#, etc. Choose a familiar language to get started faster.
Environment setup: According to the selected programming language, install the corresponding development environment and Selenium library. For example, using Python you can install the selenium library via pip.
Write test scripts: Write test scripts based on learning materials and needs. You can start with simple tests and gradually expand to complex scenarios. Focus on learning element positioning, operating browsers, assertions and reports, etc.
2. When learning a tool, you need to know what it can be used for. Selenium’s applicable scenarios include:
Automated testing: The most common scenario for Selenium is web automated testing, which can simulate user operations in the browser and verify whether system functions and interactions are normal.
Web crawler: Selenium can also be used to build a web crawler, simulate user login, click and fill in forms and other operations, and capture web page data.
Data collection: Selenium can collect data on web pages, including text, pictures, links, etc.
3. Selenium advantages and disadvantages: Advantages :
Multi-browser support: Selenium supports multiple browsers, including Chrome, Firefox, Safari, etc., and can be tested in different browsers.
Powerful API: Selenium provides a rich API that can complete various operations, such as element positioning, page navigation, form filling, etc.
Community support: Selenium has huge community support, and you can get a lot of learning resources and problem-solving help.
shortcoming:
Steep learning curve: Selenium's learning curve is relatively steep, and you need to master concepts and skills such as element positioning and operating APIs.
Dependence on the browser: Selenium needs to rely on the browser for testing, needs to install the browser driver, and may be restricted by the browser version.
4. Complete example of automated testing (Python):
from selenium import webdriver
# 创建浏览器驱动
driver = webdriver.Chrome()
# 打开网页
driver.get("https://www.example.com")
# 定位元素并操作
element = driver.find_element_by_id("element_id")
element.send_keys("example")
# 断言
assert "Example" in driver.title
# 关闭浏览器
The above example uses the Python language and Chrome browser driver to open a web page, locate an element, and enter text in the input box. Finally, an assertion is made to determine whether the page title contains "Example". Finally closed the browser driver.
3.2 Cypress automated testing
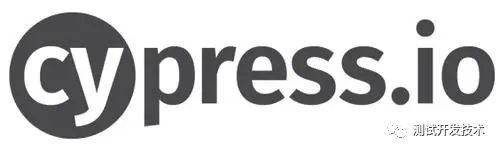
Using the same routine, you can follow the following steps to learn Cypress:
Official website : Visit the Cypress official website (
https://www.cypress.io/
) to learn about the latest version, documentation, API reference, sample code, etc.Learning materials : Reading official documentation is the best way to learn Cypress. The official documentation provides detailed tutorials and examples covering all aspects of Cypress, including installation, usage, assertions, and positioning elements.
Environment setup : Install Node.js and npm (Node Package Manager), and then use npm to install Cypress.
Write test scripts : Write test scripts based on learning materials and needs. Cypress uses JavaScript to write test scripts, and you can use the API provided by Cypress for element positioning, operations, assertions, etc.
Applicable scene:
Web application testing: The most common scenario for Cypress is automated testing of web applications, which can simulate user operations in the browser and verify whether system functions and interactions are normal.
End-to-end testing: Cypress can perform end-to-end testing, a complete testing process from the user interface to the back-end database, to ensure the normal operation of the entire application system.
Fast feedback testing: Cypress features fast feedback, allowing you to view test results and assertion errors in real time to improve testing efficiency.
advantage:
Easy to use: Cypress’s API and commands are simple and easy to understand, with a low learning curve and quick to get started.
Real-time feedback: Cypress provides real-time test feedback. You can view page operations and assertion results in real time during the test process, making it easy to debug and locate problems.
Automatic waiting: Cypress has the feature of automatic waiting, which can intelligently wait for page elements to be loaded, reducing manual waiting time.
shortcoming:
Only supports browsers: Cypress only supports testing in browsers and does not support automated testing of other client applications.
Only supports JavaScript: Cypress only supports writing test scripts in JavaScript. For testers who are not familiar with JavaScript, additional learning and adaptation may be required.
Complete example of Cypress automated testing:
// 在Cypress测试脚本中,可以使用describe和it来组织测试用例
describe('Example Test Suite', () => {
// 在每个测试用例之前执行的操作可以放在beforeEach中
beforeEach(() => {
// 打开网页
cy.visit('https://www.example.com')
})
// 编写测试用例
it('should display correct title', () => {
// 断言页面标题是否正确
cy.title().should('include', 'Example')
})
it('should fill in form', () => {
// 定位并填写表单
cy.get('#name').type('John Doe')
cy.get('#email').type('[email protected]')
cy.get('#submit').click()
// 断言表单提交后的结果
cy.get('#result').should('contain', 'Thank you')
})
})
The above example uses JavaScript to write two test cases. The first test case verifies whether the page title contains "Example", the second test case simulates filling in the form and submitting it, and then asserts whether the result contains "Thank you". Before each test case, a web page is opened.
3.3 Playwright automated testing
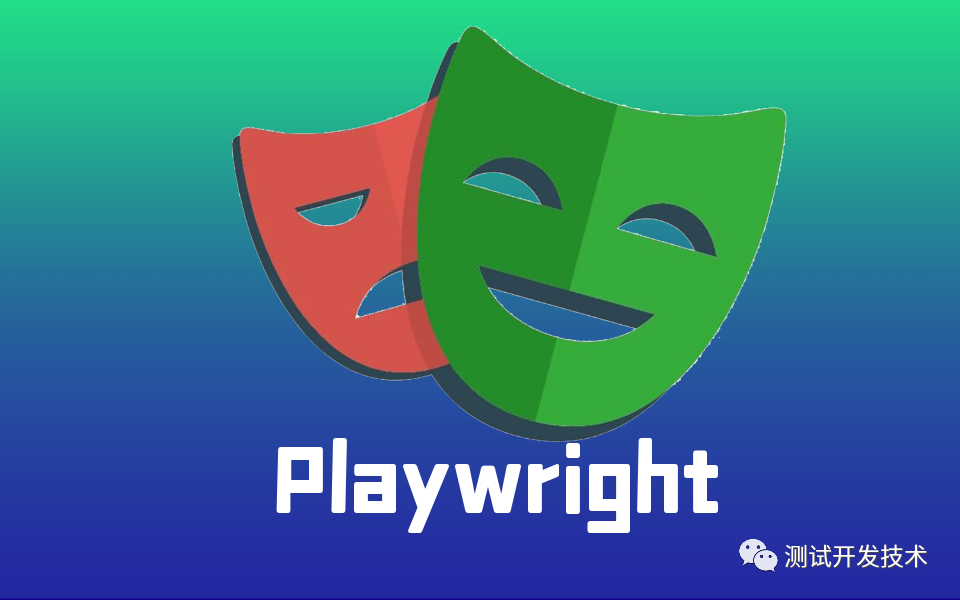
To learn Playwright you can follow these steps:
1. Official website : Visit the Playwright official website ( https://playwright.dev/
) to learn about the latest version, documentation, API reference, sample code, etc.
2. Learning materials : Reading official documentation is the best way to learn Playwright. The official documentation provides detailed tutorials and examples covering all aspects of Playwright, including installation, usage, positioned elements, assertions, etc.
3. Environment setup : Install Node.js and npm (Node Package Manager), and then use npm to install Playwright.
4. Write test scripts : Write test scripts based on learning materials and needs. Playwright uses JavaScript or TypeScript to write test scripts, and you can use the API provided by Playwright for browser operations, element positioning, assertions, etc.
Applicable scene:
Web application testing: Playwright's most common scenario is automated testing of web applications, which can simulate user operations in the browser and verify whether system functions and interactions are normal.
Cross-browser testing: Playwright supports multiple browsers, including Chrome, Firefox and WebKit, and can conduct cross-browser automated testing.
Fast feedback testing: Playwright features fast feedback, allowing you to view test results and assertion errors in real time, making it easy to debug and locate problems.
advantage:
Cross-browser support: Playwright supports multiple browsers and can conduct automated cross-browser testing to ensure application compatibility on different browsers.
Powerful API: Playwright provides a rich API that can perform browser operations, element positioning and assertions, etc. to meet various testing needs.
Multi-language support: Playwright supports multiple programming languages such as JavaScript, TypeScript, Python and .NET, making it easy for developers to choose the language that suits them for test script writing.
shortcoming:
Steep learning curve: Compared with other automated testing tools, Playwright has a steep learning curve and requires a certain foundation in JavaScript or TypeScript.
Relatively new: Playwright is relatively new and may not be as mature as other mature automation testing tools in some aspects.
Complete example of automated testing:
// 导入Playwright库
const { chromium } = require('playwright');
(async () => {
// 启动浏览器
const browser = await chromium.launch();
// 创建新页面
const page = await browser.newPage();
// 打开网页
await page.goto('https://www.example.com');
// 断言页面标题是否正确
const title = await page.title();
expect(title).toContain('Example');
// 定位并填写表单
await page.fill('#name', 'John Doe');
await page.fill('#email', '[email protected]');
await page.click('#submit');
// 断言表单提交后的结果
const result = await page.textContent('#result');
expect(result).toContain('Thank you');
// 关闭浏览器
await browser.close();
})();
The above example writes a complete Playwright test script using JavaScript. The script starts the browser, creates a new page, opens the web page, and then performs assertions and form operations. Finally closed the browser. The expect syntax is used in the assertion part, and test frameworks such as Jest can be used to make assertions.
4. Summary
Tools are just means. Different testing tools have their own characteristics and applicable scenarios. There is no so-called best tool. The most important thing is to choose the tool that suits your project needs for automated testing!
If you find it useful, please follow, like, watch, and share it in your circle of friends.
Recommended reading:
Breaking News | 2023 Latest Full-stack Test Development Skills Practical Guide V2.0 (Issue 4)
The most comprehensive software testing engineer development knowledge system map in 2022!
END
All original articles
Publish to this public account "Test Development Technology" for the first time
Long press the QR code/scan the code on WeChat to add the author