Eye-catching before watching (dog head)
Table of contents
1. Data type
There are eight basic data types :
1. Four categories:
Integer, floating point, character and boolean
2. Eight types:
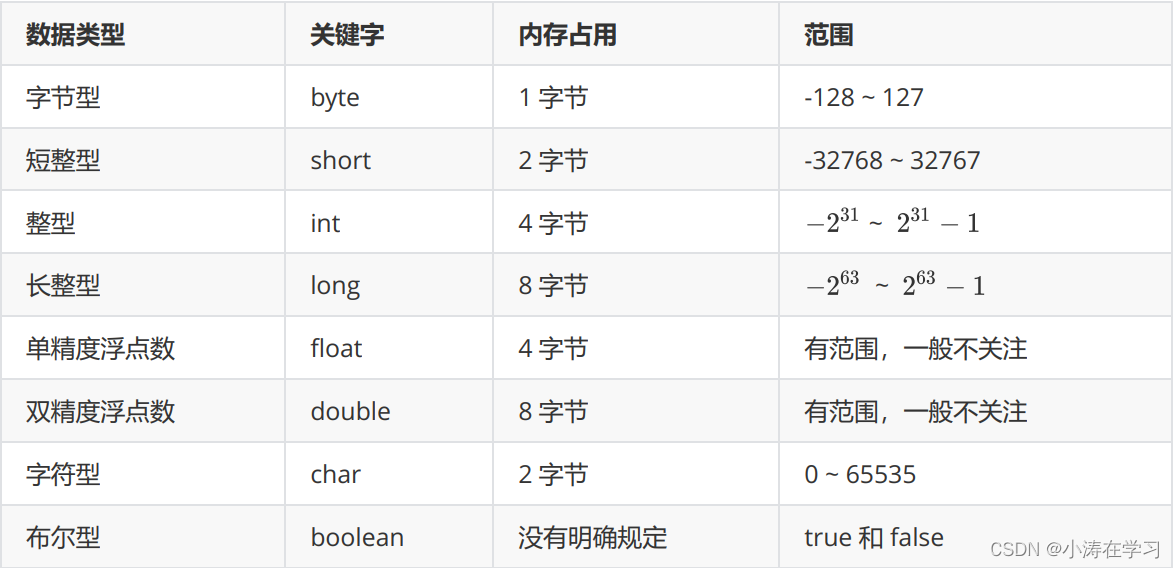
Note:
Whether in a 16-bit system or a 32-bit system, int occupies 4 bytes, and long occupies 8 bytes. Both integer
and floating point types are signed. The integer type
defaults to int type, and the floating point type defaults to The double
string is a reference type
2. Variables
(1) Variable concept
In a program, in addition to constants that remain unchanged, some contents may change frequently, such as a person's age, height, score, calculation results of mathematical functions, etc. For these frequently changing contents, in a Java program , called variables . Data types are used to define different types of variables .
(2) Grammar format
The syntax format for defining variables is:
data type variable name = initial value;
for example:
1. Integer variable
Notes:
1. Int is 4 bytes no matter what system it is in.
2. It is recommended to use the first definition. If there is no suitable initial value, it can be set to 0.
3. When setting the initial value of a variable, the value cannot exceed The representation range of int, otherwise it will cause overflow
4. Variables must be assigned an initial value before use, otherwise a compilation error will be reported
5. The packaging type of int is Integer
2. Long integer variable
Notes:
1. Add L or l after the initial value of a long integer variable. It is recommended to add L.
2. The long integer type occupies 8 bytes regardless of the system.
3. The representation range of the long integer type is:
4. long The packaging type is Long
3. Short integer variable
Notes:
1. short occupies 2 bytes in any system
2. The representation range of short is: -32768 ~ 32767
3. Be careful not to exceed the range when using it (generally less used)
4. The packaging type of short is Short
4. Byte variables
Notes:
1. byte occupies 1 byte in any system
2. The range of byte is: -128 ~ 127
3. The packaging type of byte is Byte
5. Floating point variables
(1) Double precision floating point type
Notes:
1. Double occupies 8 bytes in any system
2. Floating point numbers and integers are stored differently in memory and cannot be calculated simply in the form of
3. The packaging type of double is Double4. The memory layout of the double type complies with the IEEE 754 standard (the same as the C language). If you try to use limited memory space to represent potentially infinite decimals, there will inevitably be a certain precision error. Therefore, the floating point number is an approximation, not an exact value.
(2) Single precision floating point type
The float type occupies four bytes in Java and also complies with the IEEE 754 standard. Due to the small precision range of the data represented, double is generally preferred when floating point numbers are used in engineering, and float is not recommended. The packaging type of float is: Float.
6. Character variables
Notes:
1. Java uses single quotes + a single letter to represent character literals.
2. A character in a computer is essentially an integer. ASCII is used to represent characters in C language, while Unicode is used to represent characters in Java. Therefore
One character occupies two bytes and represents more types of characters, including Chinese.3.The packaging type of char is Character
7. Boolean variables
Precautions:
1. Variables of type boolean have only two values, true means true and false means false.
2. Java's boolean type and int cannot be converted to each other. There is no such usage as 1 means true and 0 means false.
3. Java virtual machine specification , there is no clear stipulation on how many bytes boolean occupies, and there are no bytecode instructions specifically used to process boolean. In Oracle's virtual machine implementation, boolean occupies 1 byte.
4. The packaging type of boolean is Boolean
8. Type conversion
In Java, when the data types involved in the operation are inconsistent, type conversion will be performed. Type conversion in Java is mainly divided into two categories: automatic type conversion (implicit
) and forced type conversion (explicit).
(1), automatic type conversion (implicit)
Automatic type conversion means that the code does not need to undergo any processing. The compiler will automatically process it when the code is compiled. Features: When the data range is small, it will be converted to a large data range automatically.
(2) Forced type conversion (explicit)
Forced type conversion: When operating, the code needs to go through a certain format and cannot be completed automatically. Features: Large data range to small data range.
int a = 10; long b = 100L; b = a; // int-->long,数据范围由小到大,隐式转换 a = (int)b; // long-->int, 数据范围由大到小,需要强转,否则编译失败 float f = 3.14F; double d = 5.12; d = f; // float-->double,数据范围由小到大,隐式转换 f = (float)d; // double-->float, 数据范围由大到小,需要强转,否则编译失败 a = d; // 报错,类型不兼容 a = (int)d; // int没有double表示的数据范围大,需要强转,小数点之后全部丢弃 byte b1 = 100; // 100默认为int,没有超过byte范围,隐式转换 byte b2 = (byte)257; // 257默认为int,超过byte范围,需要显示转换,否则报错 boolean flag = true; a = flag; // 编译失败:类型不兼容 flag = a; // 编译失败:类型不兼容
Notes:
1. Assignment between variables of different numeric types means that a type with a smaller range can be implicitly converted to a type with a larger range 2.
If you need to assign a value from a type with a large range to a type with a small range, forced type conversion is required. However, the precision may be lost.
3. When assigning a literal constant, Java will automatically check the numeric range.
4. Forced type conversion may not be successful, and irrelevant types cannot be converted to each other.
9. Type improvement
When different types of data are operated on each other, the smaller data type will be promoted to the larger data type .
(1) 1. Between int and long: int will be promoted to long
(2), byte and byte operations
(3) Conclusion
Both byte and byte are of the same type, but a compilation error occurs. The reason is that although a and b are both bytes, calculating a + b will first promote a and b to int, and then perform the calculation, and the result obtained is also int. This is assigned to c, and the above error will occur.
Since the computer's CPU usually reads and writes data from the memory in units of 4 bytes. For the convenience of hardware implementation, such as byte and short, which are less than 4 bytes The type will be promoted to int first and then participate in the calculation.
Correct way to write:Summary of type promotion:
1. Different types of data mixed operations, small range will be promoted to large range.
2. For short, byte, which is smaller than 4 bytes, will be promoted to 4 bytes int first. , and then calculate.
10. String type
Use the String class to define string types in Java, such as:
In some cases, it is necessary to convert between strings and integer numbers:
(1), convert int to String
(2) Convert String to int