This article mainly introduces the programming code for logging in, registering, and exiting the python login system. It has certain reference value and friends in need can refer to it. I hope you will gain a lot after reading this article. Let the editor take you to understand it together.
Design tasks
Preliminarily design the program login interface and analyze the design steps in detail.
Detailed analysis of the program
Basic frame design
```
import tkinter as tk
import tkinter.messagebox
root = tk.Tk() # Create application window
root.title("User login interface design")
root.geometry("230x100")
# --------Function block code starts-------
--------End of function block code------
root.mainloop()
Design labels to prompt users
labelName = tk.Label(root, text='User name:', justify=tk.RIGHT, width=80)
labelPwd = tk.Label(root, text='User password:', justify=tk.RIGHT, width=80)
Design input box
entryName = tk.Entry(root, width=80, textvariable=varName)
entryPwd = tk.Entry(root, show='*', width=80, textvariable=varPwd)
Design button
buttonOk = tk.Button(root, text='登录', relief=tk.RAISED, command=login)
buttonCancel = tk.Button(root, text='重置', relief=tk.RAISED, command=cancel)
buttonquit = tk.Button(root, text='退出', relief=tk.RAISED, command=_quit)
Design function
**Associated variables**
varName = tk.StringVar()
varName.set('')
varPwd = tk.StringVar()
varPwd.set('')
**Login button processing function**
def login():
# Get username and password
name = entryName.get()
pwd = entryPwd.get()
if name == 'admin' and pwd == '123456':
tk.messagebox.showinfo(title='Python tkinter', message='OK')
else:
tk.messagebox.showerror('Python tkinter', message='Error')
**Re-enter button processing function**
def cancel():
# Clear the username and password entered by the user
varName.set('')
varPwd.set('')
**Exit button processing function**
def _quit():
root.quit()
root.destroy()
Arrangement of various components
labelName.place(x=10, y=5, width=80, height=20)
labelPwd.place(x=10, y=30, width=80, height=20)
entryName.place(x=100, y=5, width=80, height=20)
entryPwd.place(x=100, y=30, width=80, height=20)
buttonOk.place(x=30, y=70, width=50, height=20)
buttonCancel.place(x=90, y=70, width=50, height=20)
buttonquit.place(x=150, y=70, width=50, height=20)
Complete program assembly
import tkinter as tk
import tkinter.messagebox
root = tk.Tk() # Create application window
root.title("User login interface design")
root.geometry("230x100")
--------Function block code starts-------
functional function design
varName = tk.StringVar()
varName.set('')
varPwd = tk.StringVar()
varPwd.set('')
def login():
# Get username and password
name = entryName.get()
pwd = entryPwd.get()
if name == 'admin' and pwd == '123456':
tk.messagebox.showinfo(title='Python tkinter', message='OK')
else:
tk.messagebox.showerror('Python tkinter', message='Error')
def cancel():
# Clear the username and password entered by the user
varName.set('')
varPwd.set('')
def _quit():
root.quit()
root.destroy()
Design of various components in the main window
labelName = tk.Label(root, text='User name:', justify=tk.RIGHT, width=80)
labelPwd = tk.Label(root, text='User password:', justify=tk.RIGHT, width=80)
entryName = tk.Entry(root, width=80, textvariable=varName)
entryPwd = tk.Entry(root, show='*', width=80, textvariable=varPwd)
buttonOk = tk.Button(root, text='登录', relief=tk.RAISED, command=login)
buttonCancel = tk.Button(root, text='重置', relief=tk.RAISED, command=cancel)
buttonquit = tk.Button(root, text='退出', relief=tk.RAISED, command=_quit)
The arrangement position of each component in the main window = formation
labelName.place(x=10, y=5, width=80, height=20)
labelPwd.place(x=10, y=30, width=80, height=20)
entryName.place(x=100, y=5, width=80, height=20)
entryPwd.place(x=100, y=30, width=80, height=20)
buttonOk.place(x=30, y=70, width=50, height=20)
buttonCancel.place(x=90, y=70, width=50, height=20)
buttonquit.place(x=150, y=70, width=50, height=20)
--------End of function block code------
root.mainloop() # Window run loop
final effect
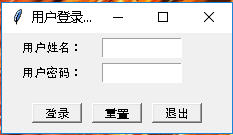
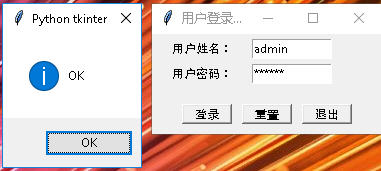
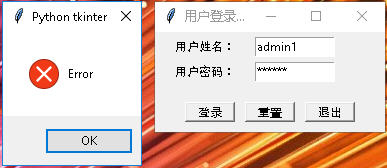