For more articles, please follow my personal blog: https://seven777777.github.io/myblog/
I saw a blog post that listed the blogger’s online interview questions about ByteDance: https://blog.csdn.net/handsomezhanghui/article/details/108691314 . In response to these questions, I tried to make the following answers. for reference. (For some answers, please refer to other information on the Internet)
Question 1
question:
把下面的纯文整理为3行4列
aaaa "dd" f g;fd分
1 "2dd" 113 512
q 'w' er,-/ g
The compiled results are as follows:
answer
const str =` aaaa "dd" f g;fd分
1 "2dd" 113 512
q 'w' er,-/ g`
let result = str.split(/[(\r\n)\r\n]+/).map(item=>{
return item.trim().split(/\s+/)
})
- First convert the string into an array based on carriage return or line feed, and match the regular rules based on carriage return or line feed:
/[(\r\n)\r\n]+/
- Remove null characters before and after each line of string:
string.trim()
- A regular string that removes null characters from each item, matches one or more spaces, converts it into an array, and matches one or more spaces:
/\s+/
Question 2
question:
阐述一下对原码/反码/补码的理解,相互之间的转换方法
answer
Original code, complement code and complement code are the three representation methods of fixed-point numbers in the computer.
Original code: The sign bit plus the absolute value of the true value, that is, the first bit represents the sign, "0" represents positive, "1" represents negative, and the remaining bits represent the value.
[+1]原 = 0000 0001
[-1]原 = 1000 0001
The complement code and the complement code are both converted on the basis of the original code. The conversion rules are as follows:
-
reverse code
- The complement of a positive number is itself
- The one's complement code of a negative number is based on its original code, the sign bit remains unchanged, and the remaining bits are inverted.
[+1] = [00000001]原 = [00000001]反 [-1] = [10000001]原 = [11111110]反
-
complement
- The complement of a positive number is itself
- The complement of a negative number is based on its original code, the sign bit remains unchanged, the remaining bits are inverted, and finally +1. (That is, +1 on the basis of the complement code)
[+1] = [00000001]原 = [00000001]反 = [00000001]补 [-1] = [10000001]原 = [11111110]反 = [11111111]补
For a detailed explanation, you can refer to the following article: https://www.cnblogs.com/zhangziqiu/archive/2011/03/30/ComputerCode.html , the explanation is very detailed
Question 3
question:
日常信息流开发过程中,经常需要显示文章发表的时间与当前时间的关系状态。
比如:刚刚、1分钟之前、一个小时之前等。要求实现-一个函数,输入为一个日期时间戳日期,
输出为输入日期与当前时间的关系:1分钟(59秒)以内显示刚刚
其他显示XXX前,比如: 1分钟前、2小时前、!一天前
能够实现该函数后,让其稍加改造,如:输入日期与当前时间相差超过2天就显示该日期等
answer
Simply follow the requirements and write a function
// time为时间戳
function timeAgo(time){
var dateStart = new Date(time);//获取开始时间
var dateEnd = new Date();//获取当前时间
var dateDiff = dateEnd.getTime() - dateStart.getTime()//计算时间差 毫秒
var dayDiff = Math.floor(dateDiff / (24 * 3600 * 1000));//计算出相差天数
// 计算小时差
var leave1=dateDiff%(24*3600*1000) //计算天数后剩余的毫秒数
var hours=Math.floor(leave1/(3600*1000))//计算出小时数
//计算相差分钟数
var leave2=leave1%(3600*1000) //计算小时数后剩余的毫秒数
var minutes=Math.floor(leave2/(60*1000))//计算相差分钟数
//计算相差秒数
var leave3=leave2%(60*1000) //计算分钟数后剩余的毫秒数
var seconds=Math.round(leave3/1000)
console.log(" 相差 "+dayDiff+"天 "+hours+"小时 "+minutes+" 分钟"+seconds+" 秒")
let strMap = {
0:'天前',
1:'小时前',
2:'分钟前',
3:'刚刚'
}
let timeDiffArray = [dayDiff,hours,minutes,seconds]
let result = '刚刚'
for(var i = 0; i < timeDiffArray.length; i++){
let val = timeDiffArray[i]
if(val > 0){
result = (i < 3 ? val : '') + strMap[i]
break;
}
}
// 输入日期与当前时间相差超过2天就显示该日期
if(dayDiff && dayDiff>2){
result = dateStart.toLocaleDateString()
}
return result
}
timeAgo(1600933140000)
Strictly speaking, this function is not rigorous. In actual situations, there are many factors to consider, such as the format of the incoming time, timestamp? Time string? Is it the correct time format? etc. All need to be judged and processed. If the above is correct, you may also need to judge whether the incoming time is before the current time, if it is in the future, what should be done, and other details, in fact, in the actual development process, Need to consider.
Therefore, it still takes some time to implement such a tool function very rigorously.
However, in the actual development process, if you encounter similar needs, there are actually many open source tools on the Internet that can be used directly. You don’t have to think about making your own wheels all the time.
Question 4
question:
请实现一个观察者模式,拥有四个方法on, of, once和trigger
const Event = {
on() {} //绑定
off() {} //解绑
once() {} //绑定一次
trigger() {} //触发事件
}
function echo(msg) { console.log(msg);
var. event= new Event();
event.on('test', echo);
event.trigger('test', 123); //输出123
event.off('test', echo); //删除某个事件回调
event.off('test'); //删除所有事件回调
event.once('test', echo);//只触发一次
answer
class Event {
constructor() {
this.events = {};
}
on(event,callback){
if(!this.events.hasOwnProperty(event)){
this.events[event] = []
}
if(typeof callback == 'function'){
this.events[event].push(callback)
}else{
throw new Error('缺少回调函数');
}
return this
}
trigger(event,...args){
if(this.events.hasOwnProperty(event)){
this.events[event].forEach((item,index,arr)=>{
item.apply(this,args)
})
}else {
throw new Error(`"${event}"事件未注册`);
}
return this
}
off(event,callback){
if(!this.events.hasOwnProperty(event)){
throw new Error(`"${event}"事件未注册`);
}else if(typeof callback != 'function'){
<!--如果callback不传,则表示移除事件中的所有回调函数-->
this.events[event] = []
}else{
this.events[event].forEach((item,index,arr)=>{
if(item == callback){
arr.splice(index,1)
}
})
}
return this
}
once(event,callback){
var onceFunc = (...args) => {
callback.apply(this,args)
this.off(event,onceFunc)
}
this.on(event,onceFunc)
return this
}
}
function echo(msg) { console.log(msg)};
function echo2(msg) { console.log(msg + 'sss')};
function echo3(msg) { console.log(msg + 'ddd')};
have a test
var event = new Event();
event.on('testOn',echo)
event.on('testOn',echo2)
event.on('testOn',echo3)
event.trigger('testOn','11111')
event.once('test', echo);
event.trigger('test','222')
event.trigger('test','333')
event.trigger('testOn','44444')
event.off('testOn',echo)
event.trigger('testOn','offff')
event.off('testOn')
event.trigger('testOn','offffall')
The print result is as follows:
Question 5
question:
分别使用正则和非正则实现1234567890.12格式化为:1,234,567,890.12。
answer:
var num = 1234567890.12
// 正则
var resultNum = (num + '').replace(/(\d)(?=(\d{3})+\.)/g, function ($0, $1) {
return $1 + ',';
})
console.log(resultNum) //1,234,567,890.12
// 非正则
var resultNum2 = num.toLocaleString()
console.log(resultNum2); //1,234,567,890.12
Question 6
question:
请写出程序运行结果
var x = 1;
var bar = function(param) {
console.log(this.x)
};
var obj1 = {
x: 1
};
var obj2 = {
x: 2
};
var obj3 = {
x: 3
};
var func = bar.bind(obj1);
func();//1
func = bar.bind(obj1).bind(obj2);
func();//1
func = bar.bind(obj1).bind(obj2).bind(obj3);
func();//1
answer:
The output results are all x values of the first bound obj object. The reason is that in Javascript, the foreign binding function object returned by the bind() method only remembers the context when it is created (if parameters are provided), and multiple bind() calls are invalid.
The deeper reason is that the implementation of bind() is equivalent to using a function to wrap a call/apply internally. The second bind() is equivalent to wrapping the first bind() again, so the bind after the second time is cannot take effect.
Regarding this issue, you can study the article https://segmentfault.com/a/1190000021944575 in detail , which is quite comprehensive. The above answer is excerpted from this article.
Question 7
question:
页面中有个table,然后里面有很多项,这个table的数据是异步加载进来,然后渲染出来的,想要实现点击td就输出td标签内的内容。
answer:
According to my personal understanding, this question is not that complicated. Let me extract the key points: 1. Asynchronous loading of data 2. Many items
Assuming it is implemented based on native or jquery
First of all, consider the performance optimization of 2, which is to use event delegation, so the click event cannot be bound to the td itself. As for where to bind it, the first thing that comes to mind is its parent, but since the data is loaded asynchronously, it table
may This will cause the DOM to not be rendered when the event is bound, causing the binding to fail.
So the event bound on table
or body
depends on whether the table element exists when the data is not loaded.
$('table').on('click','td',function(e){
alert(e.target.innerHTML)
})
$('body').on('click','table td',function(e){
alert(e.target.innerHTML)
})
There may be a misunderstanding, please leave a message to correct me!
Question 8
question:
完成以下布局,尽可能列出你能想到的方案,页面结构包括页头,主体内容,页脚
当主体内容高度超过浏览器窗口时,页脚隐藏,
当主体内容高度不能填充浏览器窗口时 页脚需停留在页面底部
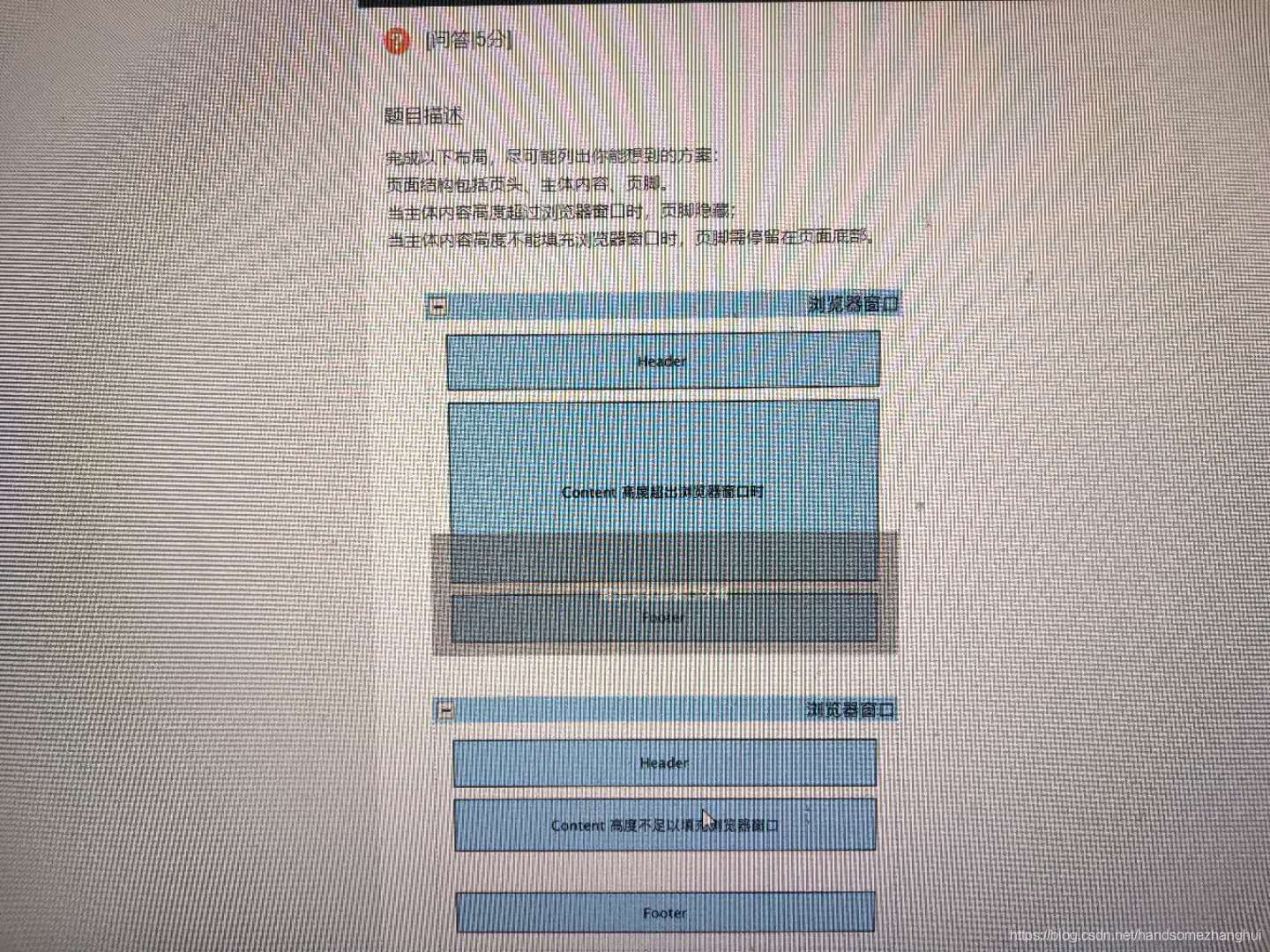
answer:
This question is relatively simple. Generally speaking, there are two common implementation directions, so I won’t go into details.
Option 1: flex layout
Option 2: Use vh or percentage, combined with css to calculate the function calc
All the above demo codes can be viewed by clicking the link below:
https://github.com/seven777777/blog-demo/blob/master/tiktok-answer.html
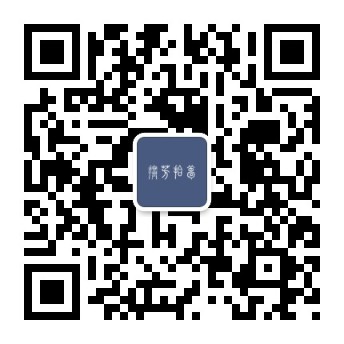