Article directory
Preface
指针数组是由指针组成的数组。它的每个元素都是一个指针,可以指向任何数据类型。在C语言中,我们可以定义一个指针数组如下:
int *arr[10]; // 声明一个大小为10的指针数组,每个元素都是一个int类型的指针
Each element in this array is a pointer of type int. We can use subscripts to access elements in an array and assign values to pointers.
Pointer arrays have a wide range of application scenarios, such as:
- Can be used to store multiple strings, each pointer points to a string;
- Can be used to implement polymorphism and store pointers of different types in the same pointer array;
- It can be used to implement dynamic memory allocation, store pointers in pointer arrays, and then dynamically allocate memory as needed.
It should be noted that when using a pointer array, you must pay attention to whether the memory pointed to by the pointer is valid to avoid problems such as pointer hanging.
提示:以下是本篇文章正文内容,下面案例可供参考
1. Pointer array
An array of pointers is an array containing pointer elements. Each array element is a pointer that points to the memory address of another data type. Pointer arrays are often used to store and process multiple address information.
In C language, you can define an array of pointers to store multiple pointers. For example, the following code defines a pointer array containing three integer pointers:
int *ptr_array[3];
This array can store three pointers to integer variables. These pointers can be initialized by assignment:
int a = 10;
int b = 20;
int c = 30;
ptr_array[0] = &a;
ptr_array[1] = &b;
ptr_array[2] = &c;
In this way, the ptr_array array contains three pointers to integer variables. These pointers can be accessed through subscripts,
for example *ptr_array[0] accesses the value of variable a.
In addition to integer pointers, other types of pointer arrays can also be defined, such as character pointer arrays, floating point pointer arrays, etc. Pointer arrays have many applications in programming, such as string arrays, function pointer arrays, etc.
int* arr1[10]; //整形指针的数组
char* arr2[4]; // 字符指针的数组
char** arr3[5]; // 二级字符指针的数组
1.1 Use pointer array to simulate two-dimensional array
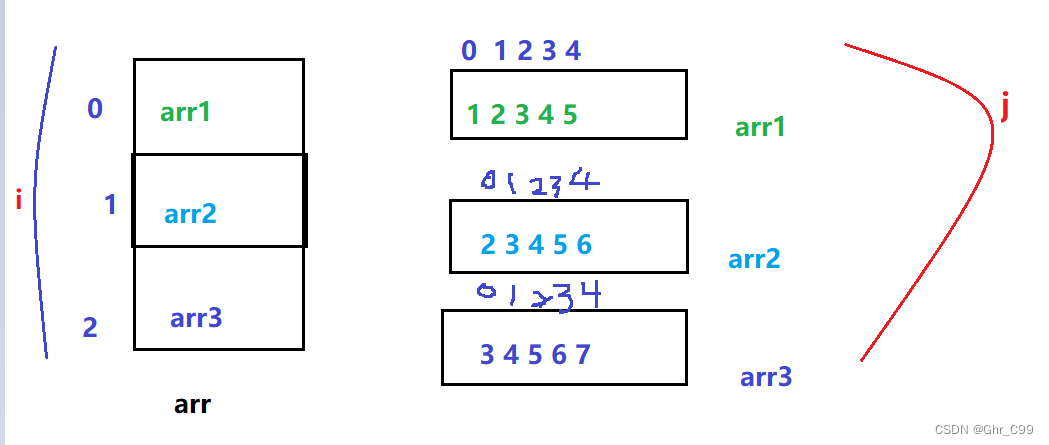
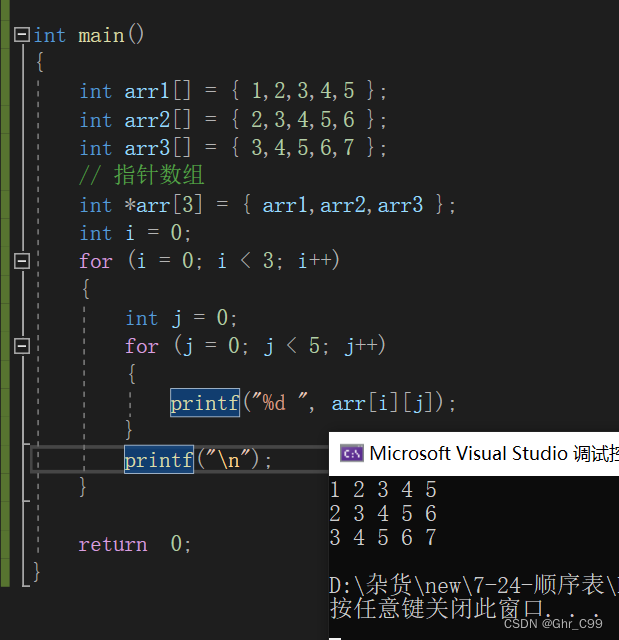
2. Array pointer
Are array pointers pointers? Or an array?
The answer is: pointers.
We are already familiar with:
integer pointer: int pint; a pointer that can point to integer data.
Floating point pointer: float pf; a pointer that can point to floating point data.
The array pointer should be: a pointer that can point to the array.

2.1 The array name is the address of the first element of the array
There are two exceptions:1. sizeof (array name), the array name here is not the address of the first element of the array, the array name represents the entire array, sizeof (array name) calculates the size of the entire array, the unit is bytes.
2. & array name. The array name here represents the entire array. & array name retrieves the address of the entire array. In addition, the array name in all other places is the address of the first element of the array.
Array pointers are generally convenient for two-dimensional arrays.
3. One-dimensional array parameters are passed. The formal parameter part can be an array or a pointer.
4. Two-dimensional array parameters are passed. The formal parameter part can be an array or a pointer.
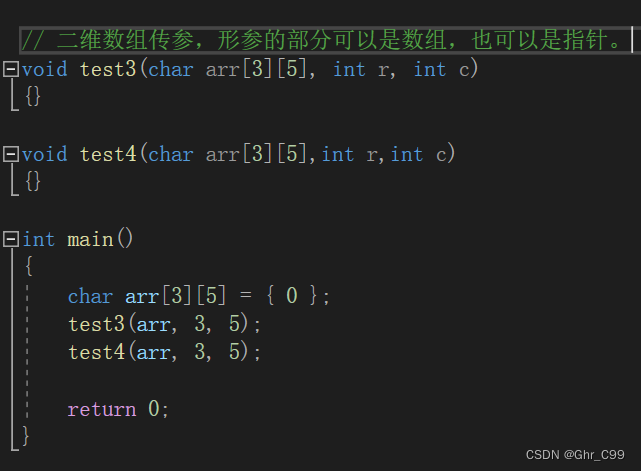
2.2 Two-dimensional array parameter transfer
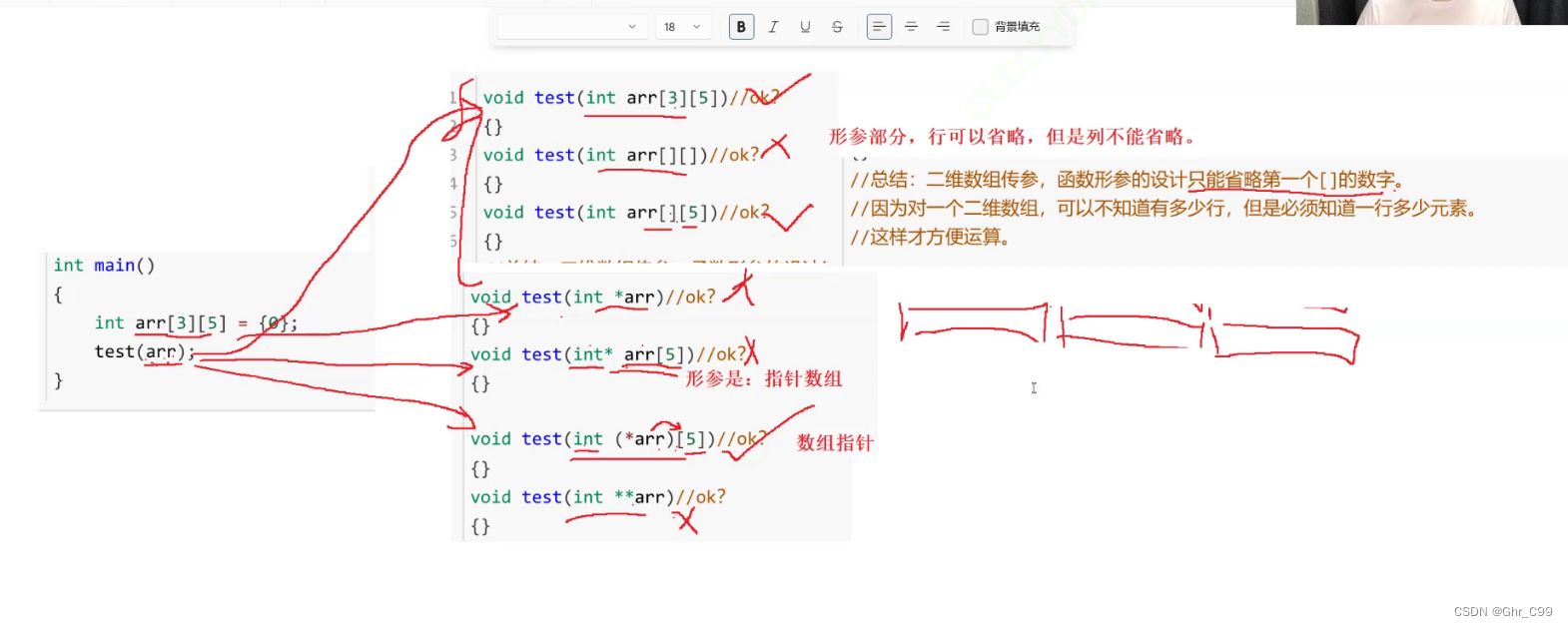
2.3 First-level pointer parameter passing
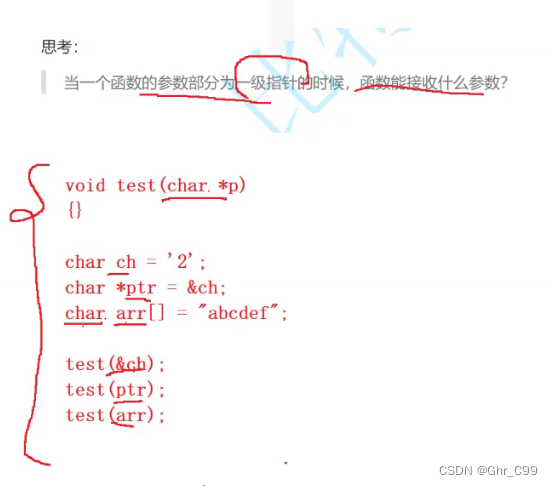
2.4 Second-level pointer parameter passing
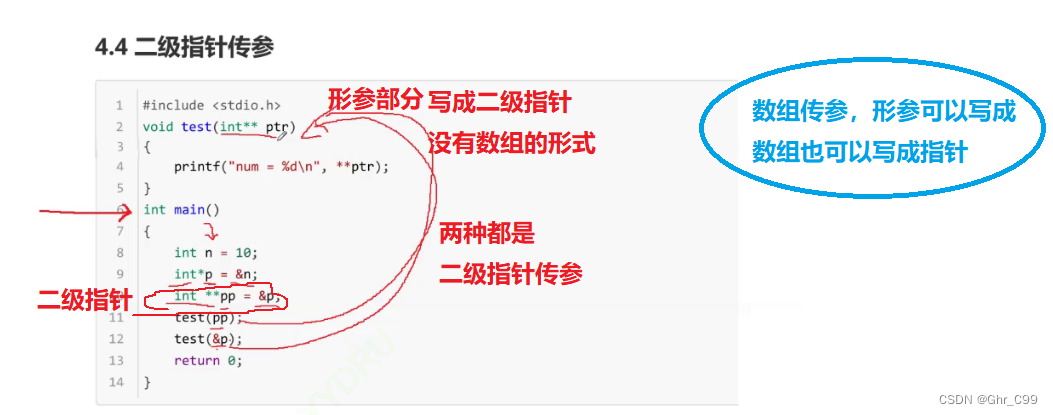
Thinking: When our formal parameter part is a secondary pointer, what kind of parameters can be received?
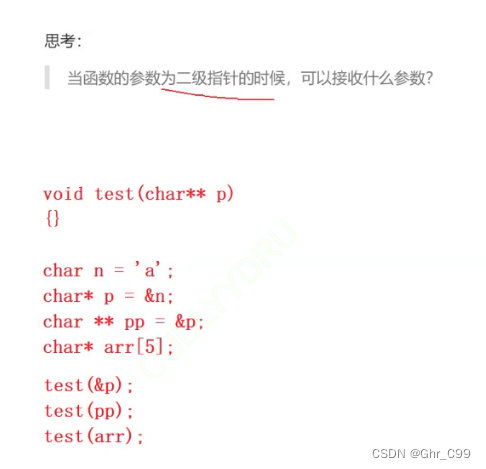
3. Function pointer
function pointer - pointer to a function
-
Function:
The function name is the address of the function
& the function name is still the address of the function -
Array:
The array name is the address of the first element of the array
& the array name is the address of the entire array
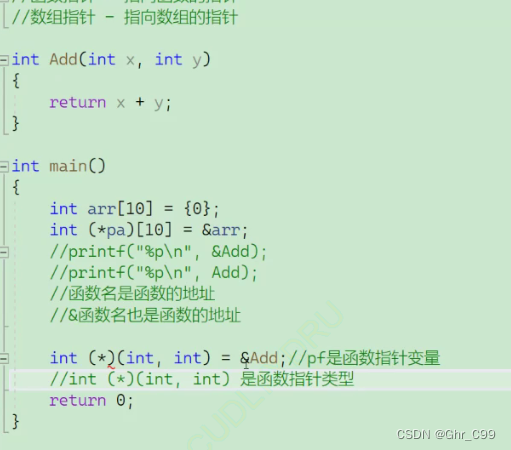
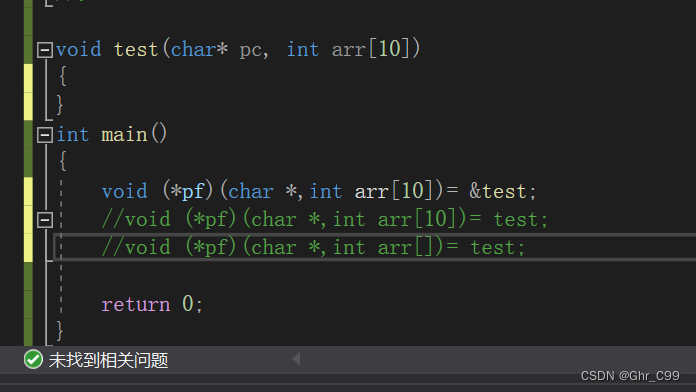
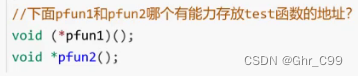
First of all, to be able to store addresses, pfun1 or pfun2 is required to be a pointer. So which one is the pointer? ?
pfun1 can be stored. pfun1 is first combined with *, indicating that pfun1 is a pointer. The pointer points to a function. The pointed function has no parameters and the return value type is void.
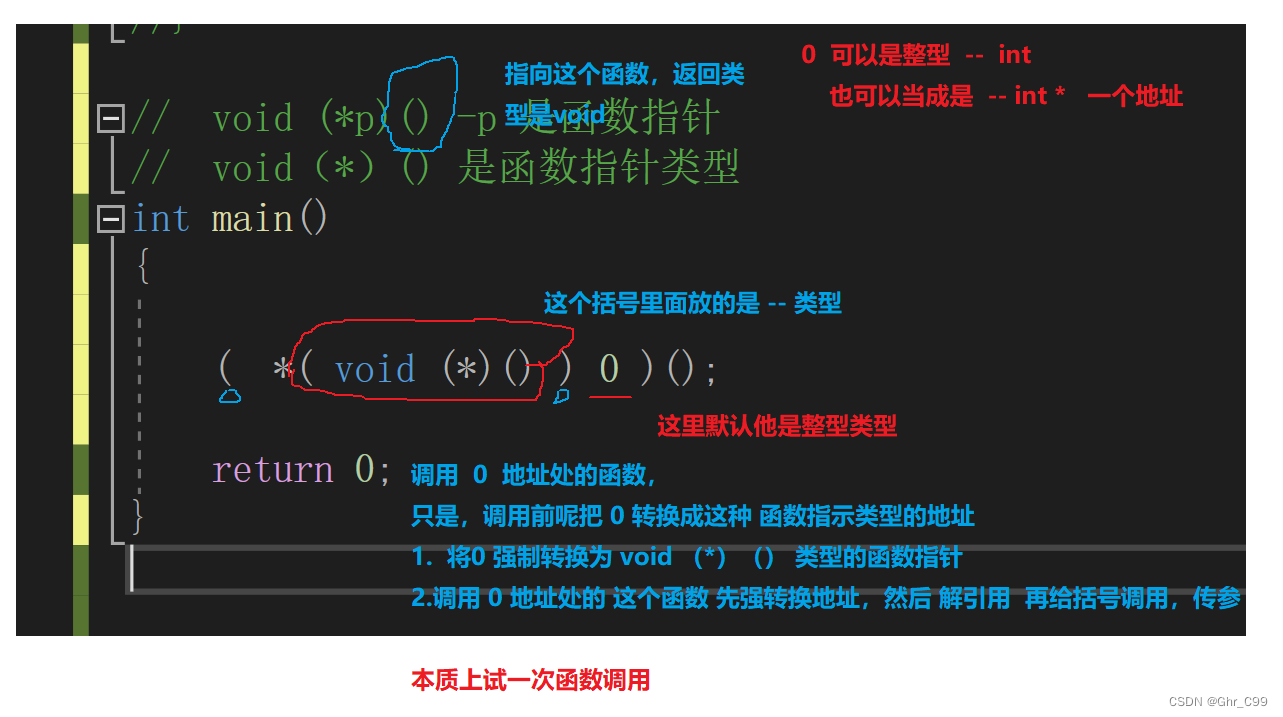
4. typedef renaming
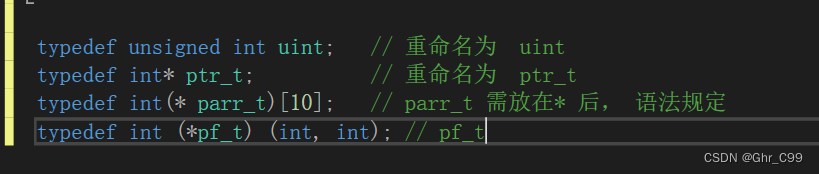
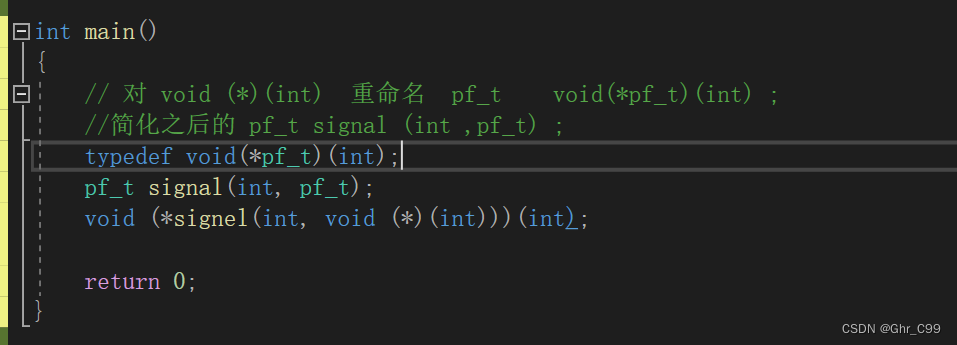