Table of contents
1.1 Understanding of character pointers
1.2 The character pointer stores the string
1.3 The use of character pointers
2.1 Understanding of pointer arrays
3.1 Understanding of array pointers
3.2 The difference between array name and & array name
3.4 Array parameters, pointer parameters
3.5 One-dimensional array parameter passing
3.6 Two-dimensional array parameter passing
3.7 Level 1 Pointer Parameter Passing
3.8 Second-level pointer parameter passing
1. Character pointer
1.1 Understanding of character pointers
Understanding of character pointers: In fact, it is very simple, just compare it with other types, please see the code below.
#define _CRT_SECURE_NO_WARNINGS 1
#include<stdio.h>
#include<stdlib.h>
int main()
{
int a = 10; //定义一个整型变量
printf("%d\n", a);
int* p = &a; //将变量a的地址存放的整型指针变量p中
*p = 15; //对p解引用改变a地址的内容,即把15换成10
printf("%d\n", a);
printf("-------------\n");
char c = 'A'; //定义一个字符变量
printf("%c\n", c); //将变量c的地址存放的字符指针变量pc中
char* pc = &c; 对pc解引用改变a地址的内容,即把'A'换成'C'
*pc = 'C';
printf("%c\n", c);
return 0;
}
Same as the usage of integer pointer type, the pointer is used to store the address of the variable , dereference changes the content of the address, but the type is different, the integer pointer stores the address of the integer type variable, and the character pointer stores the character Address of type variable.
1.2 The character pointer stores the string
We know that the character pointer can store the address of a character variable, so what if it is a stored string?
#define _CRT_SECURE_NO_WARNINGS 1
#include<stdio.h>
int main()
{
char arr[] = "hello classmate!";
printf("%s\n", arr);
printf("%c\n",*arr);
printf("----------------\n");
const char* pstr = "hello classmate!";
printf("%s\n", pstr);
printf("%c\n", *pstr);
return 0;
}
Analyzing the above code, you will also be surprised to find that the way the character pointer stores the string variable is similar to the array name of the array indicating the address of the first element. According to the above code, we specifically divide it into upper and lower parts.
The first part : A character array of arr array name is defined, the array name is the address of the first element of the array, so the array name arr is the address of the character 'h', and when we print strings, we generally only need to enter the array name to print characters String, and this also shows that the string printing can be printed as long as the address of the first element is given. Since arr is the address of the first element, dereferencing arr is the first element.
The second part : very similar to the first part, we define a pstr character pointer to store the address of the string, the result is printed and found that pstr does not store the address of the entire "hello classmate" string , but the first character The address of 'h' .
Printing strings and pstr dereferences are the same as the above arrays, so I won't go into details. As for why const is added, it is because pstr stores a constant string. Since it is a constant, const is added to prevent it from being modified.
1.3 The use of character pointers
Take a look at the code first, and do a little research yourself.
#include <stdio.h>
int main()
{
char str1[] = "hello friend.";
char str2[] = "hello friend.";
const char *str3 = "hello friend.";
const char *str4 = "hello friend.";
if(str1 ==str2)
printf("str1 and str2 are same\n");
else
printf("str1 and str2 are not same\n");
if(str3 ==str4)
printf("str3 and str4 are same\n");
else
printf("str3 and str4 are not same\n");
return 0;
}
finish watching? OK First of all, we all know that str1 and str2 are the address of the first element of the array, and then you find that they are both the address of the first character 'h', you may think that str1 == str2, don't be blinded by such an illusion. str1 and str2 are two arrays, which open up two different spaces in the memory. Even if the contents are the same, the addresses cannot be the same. The addresses of the first elements of the two are naturally different, so str1 is not equal to str2.
Looking at the character pointers str3 and str4, they store the address of the character 'h', but because str3 and str4 are pointers . When pointing to the same string, they will actually point to the same block of memory, which is actually the same block address. To put it simply, str3 and str4 use the same block address.
2. Array of pointers
2.1 Understanding of pointer arrays
What is an array of pointers? Is it an array or a pointer? In the future, we will judge in this way, whoever is placed in the back, the pointer array, and the array is placed in the back, so the pointer array is an array, an array used to store pointers.
#include<stdio.h>
int main()
{
int arr1[10]; //arr1存放了10个元素,类型是整型
char arr2[10];//arr2存放了10个元素,类型是字符型
int* arr3[10];//arr3存放了10个元素,类型是整型指针
char* arr4[10];//arr4存放了10个元素,类型是字符指针
return 0;
}
Because the content of the pointer array is very shallow, this content should be understandable, and we focus on the array pointer.
3. Array pointer
3.1 Understanding of array pointers
The array is a pointer according to the principle of whoever puts it behind, so the array pointer is a pointer. what is that for ? we know
int main()
{
int *p1[10];
int (*p2)[10];
//p1, p2分别是什么?
return 0;
}
We must remember that the priority of [ ] is higher than that of * , so p1 is combined with [10] first, and the type is int*, which means that p1 is an array of pointers.
p2 is first added () to ensure that p2 is first combined with *. p is first combined with *, indicating that p2 is a pointer variable, and then it points to an array of 10 integers. So p2 is a pointer pointing to an array, called an array pointer.
3.2 The difference between array name and & array name
Because before understanding the array pointer, it is still necessary to be able to distinguish between the array name and the & array name.
int arr[10];
#include <stdio.h>
int main()
{
int arr[10] = {0};
printf("%p\n", arr);
printf("%p\n", &arr);
return 0;
}
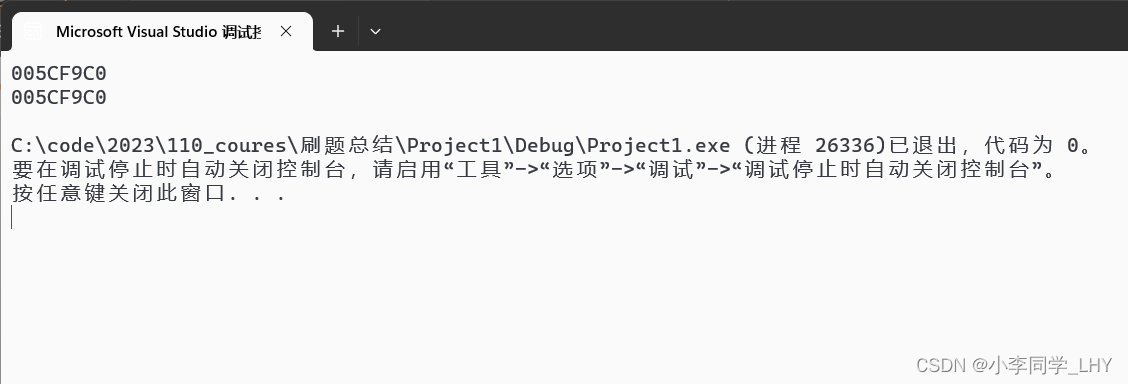
It can be seen that the address printed by the array name and & array name is the same. Are the two the same?
Let's look at the following code again:
#include <stdio.h>
int main()
{
int arr[10] = { 0 };
printf("arr = %p\n", arr);
printf("&arr= %p\n", &arr);
printf("arr+1 = %p\n", arr+1);
printf("&arr+1= %p\n", &arr+1);
return 0;
}
The result of the operation is as follows:
First of all, we need to understand the array name:
the array name is the address of the first element of the array,
there are two exceptions:
1. sizeof(array name) , the array name here is not the address of the first element of the array, the array name represents the entire array, sizeof(array name) calculates the size of the entire array in bytes.
2. &Array name , where the array name represents the entire array, and &array name retrieves the address of the entire array.
In addition, the array name in all places is the address of the first element of the array.
3.3 Use of array pointers
#include <stdio.h>
int main()
{
int arr[10] = {1,2,3,4,5,6,7,8,9,0};
int (*p)[10] = &arr;//把数组arr的地址赋值给数组指针变量p
return 0;
}
Generally speaking, array pointers are more used in passing parameters in two-dimensional arrays.
#include <stdio.h>
void print_arr1(int arr[3][5], int row, int col)
{
int i = 0;
int j = 0;
for (i = 0; i < row; i++)
{
for (j = 0; j < col; j++)
{
printf("%d ", arr[i][j]);
}
printf("\n");
}
}
void print_arr2(int(*arr)[5], int row, int col)
{
int i = 0;
int j = 0;
for (i = 0; i < row; i++)
{
for (j = 0; j < col; j++)
{
printf("%d ", arr[i][j]);
}
printf("\n");
}
}
int main()
{
int arr[3][5] = { 1,2,3,4,5,6,7,8,9,10 };
print_arr1(arr, 3, 5);
printf("-----------------\n");
//数组名arr,表示首元素的地址
//但是二维数组的首元素是二维数组的第一行
//所以这里传递的arr,其实相当于第一行的地址,是一维数组的地址
//可以数组指针来接收
print_arr2(arr, 3, 5);
return 0;
}
The running code is as follows:
When a two-dimensional array is passed as a parameter, the function can receive both a two-dimensional array and an array pointer , because the array name of a two-dimensional array is the address of the first element, and the first element is not a variable, but a one-dimensional array, so you can use Array pointer to receive.
Such as code:
#include <stdio.h>
int main()
{
int arr[3][5] = { 1,2,3,4,5, 2,3,4,5,6, 3,4,5,6,7 };
Print(arr, 3, 5);
return 0;
}
3.4 Array parameters, pointer parameters
3.5 One-dimensional array parameter passing
An array and a function are defined, and the array name is put in when calling the function, and the function can be received by means of an array and a pointer when receiving. Because the array is stored continuously in the memory, if you pass in the array name, you can know the address of the first element, and then use the pointer to receive the address of the first element, you can know the entire array. Receiving the array name with an array is essentially receiving a pointer .
Using a simple example:
void test(int arr[])//ok?
{}
void test(int arr[10])//ok?
{}
void test(int* arr)//ok?
{}
void test2(int* arr[20])//ok?
{}
void test2(int** arr)//ok?
{}
int main()
{
int arr[10] = { 0 };
int *arr2[10] = { 0 };
test(arr);
test2(arr2);
}
Answer in order:
The main function calls the test function and passes the name of the arr array.
void test(int arr[])//ok? arr is an array and can be received by an array. ok
{}
void test(int arr[10])//ok? Similarly, arr is an array and can be received by an array. ok
{}
void test(int* arr)//ok? The address of the first element can be received by pointer. ok{}
The main function calls the test2 function and passes the arr2 array name.
void test2(int* arr[20])//ok? arr2 is an array of pointers, which can be received by an array of pointers. ok
{}
void test2(int** arr)//ok? The first element of arr2 is a first-level pointer, which must be received by a second-level pointer. ok
{}
3.6 Two-dimensional array parameter passing
When passing parameters in a two-dimensional array, the address of the first element is actually passed, and the first element is a one-dimensional array. Use an array pointer to receive a one-dimensional array, or use a two-dimensional array to receive it (remember that it is essentially received by a pointer)
void test(int arr[3][5])//ok?
{}
void test(int arr[][])//ok?
{}
void test(int arr[][5])//ok?
{}
void test(int *arr)//ok?
{}
void test(int* arr[5])//ok?
{}
void test(int (*arr)[5])//ok?
{}
void test(int **arr)//ok?
{}
int main()
{
int arr[3][5] = {0};
test(arr);
}
void test ( int arr [ 3 ][ 5 ]) // The one-dimensional array address passed in the past can also be received by two-dimensional array. ok{}void test ( int arr [][]) // two-dimensional array can omit rows, but cannot omit columns. not ok{}void test ( int arr [][ 5 ]) // same as above, ok{}void test ( int * arr ) // The passed one-dimensional array address, so use the array pointer to receive, not ok{}void test ( int* arr [ 5 ]) //Don't be confused, brothers, this is an array of pointers, not an array pointer, not ok{}void test ( int ( * arr )[ 5 ]) // confirm that it is an array pointer, ok{}void test ( int ** arr ) // what the second-level pointer can receive is the first-level pointer, and cannot directly receive the address/not ok{}
3.7 Level 1 Pointer Parameter Passing
When the parameter of the function is a first-level pointer, what kind of parameters can be passed in when calling?
1. For a single variable address , there is no problem with receiving the address of the first-level pointer.
void my_printf(char* str) { printf("%c\n", *str); } int main() { char pc = 'K'; my_printf(&pc); return 0; }
2. Array name. Because the array name is the address of the first element;
void my_printf(char* str) { printf("%s\n", str); } int main() { char arr[] = "CSDN"; my_printf(arr); return 0; }
3. Level 1 pointer. Use a variable to receive the address of the array name. At this time, the variable is a first-level pointer, so the first-level pointer passes parameters and the first-level pointer receives.
void my_printf(char* str) { printf("%c\n", *str); } int main() { char cp = 'J'; char* cpp = &cp; my_printf(cpp); return 0; }
3.8 Second-level pointer parameter passing
1. The address of the first-level pointer.
void my_printf(char** str) { printf("%c\n", **str); } int main() { char pc = 'A'; char* pcc = &pc; my_printf(&pcc); return 0; }
2. Secondary pointer.
void my_printf(char** str) { printf("%c\n", **str); } int main() { char pc = 'B'; char* pcc = &pc; char** pccc = &pcc; my_printf(pccc); return 0; }
3. Array of pointers.
void my_printf(char** str) { printf("%s\n", *str); } int main() { char* arr[] = {"abcdef"}; my_printf(arr); return 0; }
Alright, that's all for today's content. If you can help the judge, I hope the judge will give you three consecutive support! ! ! ,