Table of contents
Basic understanding of serial port
About electrical standards and protocols
About the level of the serial port
Configuration of related registers and working mode of serial port
Introduction to common functions of stm32HAL library
Serial port sending and receiving functions
Serial port interrupt callback function
Status tag variable: USART_RX_STA
Serial port reception interrupt process
Serial port experiment (non-interruption)
Serial port experiment (interruption)
Basic understanding of serial port
Features:It is a method of wired communication between devices.Data is transmitted sequentially bit by bitTwo-way communication, full duplexTransfer speed is relatively slow
About electrical standards and protocols
RS-232
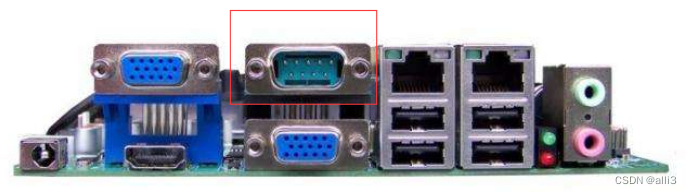
RS-422
Since the receiver uses high input impedance and the transmit driver has stronger driving capability than RS232, it allows multiple receiving nodes to be connected on the same transmission line, up to 10 nodes. That is, there is one master device (Master) and the rest are slave devices (Slave). The slave devices cannot communicate with each other, so RS-422 supports point-to-many two-way communication. The maximum transmission distance of RS-422 is 1219 meters, and the maximum transmission rate is 10Mb/s. The length of a balanced twisted pair is inversely proportional to the transmission rate.
RS-485
It is developed on the basis of RS-422. No matter the four-wire or two-wire connection method, up to 32 devices can be connected to the bus.
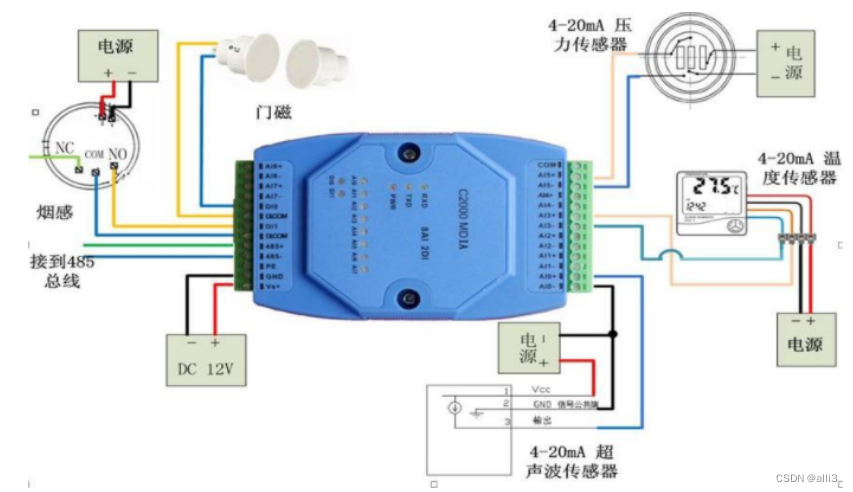
About the level of the serial port
Asynchronous serial refers to UART (Universal Asynchronous Receiver/Transmitter), universal asynchronous reception/transmission.UART includes TTL level serial port and RS232 level serial port
RS232 level
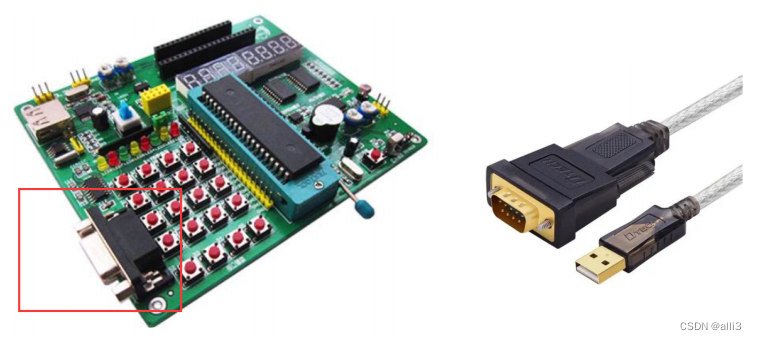
TTL level
Serial port wiring method
RXD: data input pin, data acceptance
TXD: data transmission pin, data transmission
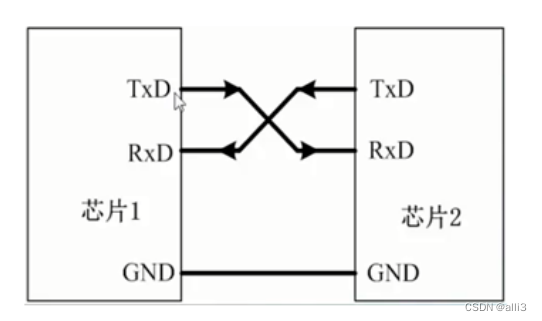
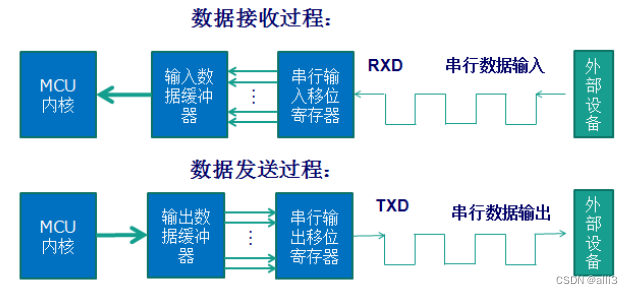
Configuration of related registers and working mode of serial port
How is the character 'a' uploaded from the microcontroller to the PC ?
The ASSII code of a is 97 , which is 0x61 in hexadecimal and 01010001 in binary . These 8 bits are the data bits.Serial port working mode 1, one frame of data has 10 bits, start bit 0 + data bit + stop bit 1Then one frame of data of a is 0 1000 1010 1

There are 8 bits in a byte. For example, the ASSII code of the letter 'a' is 97 in decimal and 0110 0001 in binary. It is sent from the bit to the high bit at a time and received as well.
Introduction to common functions of stm32HAL library
Serial port sending and receiving functions
HAL_UART_Transmit(); // Serial port sends data, using timeout management mechanism
HAL_UART_Receive();//The serial port receives data and uses the timeout management mechanism
HAL_UART_Transmit_IT();//Send data in serial port interrupt mode
HAL_UART_Receive_IT(); //Serial port interrupt mode receives data
HAL_StatusTypeDef HAL_UART_Transmit(UART_HandleTypeDef *huart,
uint8_t *pData, uint16_t Size, uint32_t Timeout)
HAL_StatusTypeDef HAL_UART_Receive_IT(UART_HandleTypeDef *huart,
uint8_t *pData, uint16_t Size)
Serial port interrupt callback function
Status tag variable: USART_RX_STA

Serial port reception interrupt process
Serial port experiment (non-interruption)
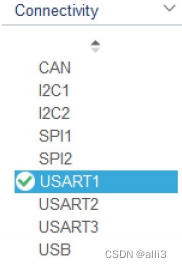
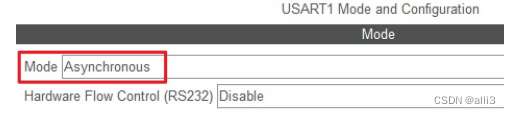
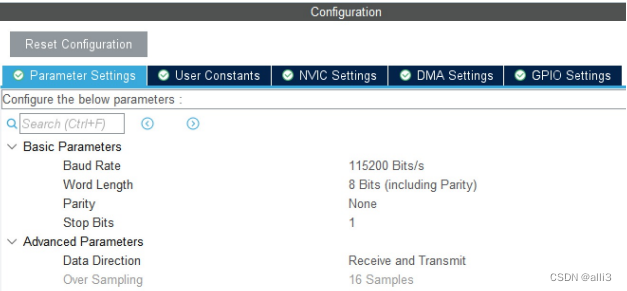
4. Use MicroLIB library
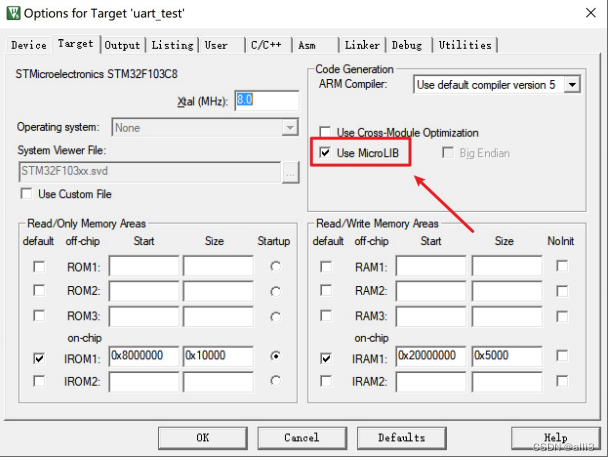
Programming implementation
# include <stdio.h># include <string.h>unsigned char ch [ 20 ] = { 0 };int fputc ( int ch , FILE * f ){unsigned char temp [ 1 ] = { ch };HAL_UART_Transmit ( & huart1 , temp , 1 , 0xffff );return ch ;}In the main function:unsigned char ch [ 20 ] = { 0 };HAL_UART_Transmit ( & huart1 , "hello world\n" , strlen ( "hello world\n" ), 100 );while ( 1 ){HAL_UART_Receive ( & huart1 , ch , 19 , 100 );//HAL_UART_Transmit(&huart1, ch, strlen(ch), 100);printf ( ch );memset ( ch , 0 , strlen ( ch ));}
Serial port experiment (interruption)
Same as above for the first 4 steps
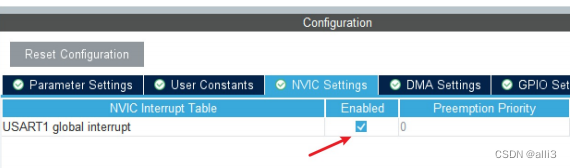
Programming implementation
# include <stdio.h>// Serial port receiving buffer ( 1 byte)uint8_t buf = 0 ;// Define the maximum number of received bytes 200 ( can be adjusted according to needs)# define UART1_REC_LEN 200// Receive buffer , the data received by the serial port is placed in this array, the maximum is UART1_REC_LEN bytesuint8_t UART1_RX_Buffer [ UART1_REC_LEN ];// Receive status// bit15 , reception completion flag// bit14 , received 0x0d// bit13~0 , the number of valid bytes receiveduint16_t UART1_RX_STA = 0 ;// Reception completion callback function, after receiving a data, process it herevoid HAL_UART_RxCpltCallback ( UART_HandleTypeDef * huart ){// This function is called for all triggers to determine which serial port triggered the interrupt.if ( huart -> Instance == USART1 ){// Determine whether the reception is completed (UART1_RX_STA bit15 is 1 )if (( UART1_RX_STA & 0x8000 ) == 0 ){// If 0x0d (carriage return) has been received,if ( UART1_RX_STA & 0x4000 ){//Then determine whether 0x0a (line feed) is receivedif ( buf == 0x0a )//If both 0x0a and 0x0d are received, set bit15 to 1UART1_RX_STA |= 0x8000 ;else// Otherwise, consider receiving error and start againUART1_RX_STA = 0 ;}else // If 0x0d (carriage return) is not received{//Then first determine whether the received character is 0x0d (carriage return)if ( buf == 0x0d ){//If yes, set bit14 to 1UART1_RX_STA |= 0x4000 ;}else{// Otherwise, save the received data in the cache arrayUART1_RX_Buffer [ UART1_RX_STA & 0X3FFF ] = buf ;UART1_RX_STA ++ ; // If the received data is larger than UART1_REC_LEN ( 200 bytes), restart receptionif ( UART1_RX_STA > UART1_REC_LEN - 1 )UART1_RX_STA = 0 ;}}}// Re-enable interruptsHAL_UART_Receive_IT ( & huart1 , & buf , 1 );}}int fputc ( int ch , FILE * f ){unsigned char temp [ 1 ] = { ch };HAL_UART_Transmit ( & huart1 , temp , 1 , 0xffff );return ch ;}main function partHAL_UART_Receive_IT ( & huart1 , & buf , 1 );while ( 1 ){/* USER CODE END WHILE *//* USER CODE BEGIN 3 *///Judge whether the serial port reception is completedif ( UART1_RX_STA & 0x8000 ){printf ( " Received data: " );//Send the received data to the serial portHAL_UART_Transmit ( & huart1 , UART1_RX_Buffer , UART1_RX_STA & 0x3fff , 0xffff );// Wait for sending to completewhile ( huart1 . gState != HAL_UART_STATE_READY );printf ( "\r\n" );//Restart next receptionUART1_RX_STA = 0 ;}printf ( "hello liangxu\r\n" );HAL_Delay ( 1000 );}