1. Create a Canvas component
First, we create a Canvas, and then we create an empty object under the Canvas, named Resgister. Set the anchor point of the empty object to expand the full screen.
2. We create an Image component under the Resgister empty object and rename it to bg. We also set its anchor point to the full screen expanded state. Next, we import our picture UI material, modify its type to 2D and UI, and click Apply. Then we can use it in the Unity engine.
Next, we drag the corresponding bg picture to the corresponding position.
3. Create the InputFiled component
(1). We create the InputFiled component under bg, and rename the created InputFiled component to userName. Next, we click Placehoder to adjust its size and adjust the corresponding component style.
(2). We also need to adjust the font size, style and alignment of the Text inside, etc. This Text modification is the size of the characters when we input, and the Placehoder above is the font style displayed by the InputFiled component.
(3) Click the userNamer component and press the shortcut key Ctrl+D to copy one side out, we renamed it password, we only need to modify the words in the Placehoder.
We continue to copy a copy and change its name to confirmPassword, which is exactly the same as the above operation, only need to modify the words inside. Change to: Please confirm the password...
Finally, we need to adjust the positions of these three objects appropriately.
Here we have reached such an effect, and then we drag the corresponding picture into it.
(4). We create a Button component under bg and rename it to registerButton. We set its anchor point so that it is in the corresponding position and adjust its size. Modify the words inside, and modify the color on the button to make it more obvious to click or move.
Next, we ctrl+D to copy an identical copy, name it loginButton, adjust its position and only need to modify the words in the text, no other operations are required.
Next we create a text to display at the top, telling us that we have entered the registration page. We set the anchor point of this text to the top full screen.
We adjust the style of the text. We need to make it bigger because it's on top. The specific operation is shown in the figure.
(5). Let's create another Text component, which we use to receive registration feedback. We renamed it TipsText, modified the text inside, font size, alignment, color we set to red.
After setting up, our UI is basically built. The renderings are as follows:
It still looks and feels good. Let's run it next
As can be seen from the above picture, the UI has been completed, and then we need to write code to achieve the corresponding effect.
code:
using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.UI; using System; using UnityEngine.SceneManagement; public class RegisterManager : MonoBehaviour { public InputField usernameInput; public InputField passwordInput; public InputField confirmPasswordInput; public Text TipsText; public void RegisterInformation() { if (PlayerPrefs.GetString(usernameInput.text) == "") { if (passwordInput.text == confirmPasswordInput.text) { PlayerPrefs.SetString(usernameInput.text, usernameInput.text); PlayerPrefs.SetString(usernameInput.text + "password", passwordInput.text); //绿色 TipsText.color = Color.green; TipsText.text = "注册成功!"; } else { //红色 TipsText.color = Color.red; TipsText.text = "两次密码输入不一致"; } } else { //红色 TipsText.color = Color.red; TipsText.text = "用户已存在"; } } public void Login() { if (PlayerPrefs.GetString(usernameInput.text) != "") { if (PlayerPrefs.GetString(usernameInput.text + "password") == passwordInput.text) { //绿色 TipsText.color = Color.green; TipsText.text = "登录成功,请稍等..."; StartCoroutine(success()); } else { //红色 TipsText.color = Color.red; TipsText.text = "密码错误"; } } else { //红色 TipsText.color = Color.red; TipsText.text = "账号不存在"; } } //登入成功 IEnumerator success() { //等待2秒 yield return new WaitForSeconds(2); //加载场景 SceneManager.LoadScene(1); } }
4. Return to the Unity engine to mount the corresponding components
(1). The method corresponding to the button button mount
5. Finally, let's run it to see the effect.
Register page
This is a simple Register registration page production. If there is no more advanced method, you can use the simple method I made to make the whole project more perfect.
at last
The above steps are the whole process of making a simple and complete registration page system. Hope to help you! ! !
Friends who have seen it, click three times with one button. Your support makes me more motivated to create and share. I hope it will always bring you surprises and gains.
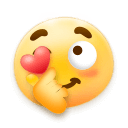