The first step is to configure maven first.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
<version>2.2.2.RELEASE</version>
</dependency>
<!-- apache commons-text-->
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-text</artifactId>
<version>1.1</version>
</dependency>
<dependency>
<groupId>cn.hutool</groupId>
<artifactId>hutool-http</artifactId>
<version>5.8.6</version>
</dependency>
<dependency>
<groupId>cn.hutool</groupId>
<artifactId>hutool-json</artifactId>
<version>5.8.6</version>
</dependency>
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>fastjson</artifactId>
<version>1.2.28</version>
</dependency>
The second step is to directly upload the code! hey-hey
public static final String APPID = "你的公众号的APPID";
public static final String SECRET = "你的公众号的SECRET";
Obtaining the AccessToken credential is very important and needs to be updated every two hours.
public static String getAccessToken() throws Exception {
String accessTokenUrl = "https://api.weixin.qq.com/cgi-bin/token?grant_type=client_credential&appid="
+ APPID + "&secret=" + SECRET;
System.out.println("URL for getting accessToken accessTokenUrl=" + accessTokenUrl);
URL url = new URL(accessTokenUrl);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("GET");
connection.setDoOutput(true);
connection.setDoInput(true);
connection.connect();
//获取返回的字符
InputStream inputStream = connection.getInputStream();
int size = inputStream.available();
byte[] bs = new byte[size];
inputStream.read(bs);
String message = new String(bs, "UTF-8");
//获取access_token
JSONObject jsonObject = JSONObject.parseObject(message);
String accessToken = jsonObject.getString("access_token");
String expires_in = jsonObject.getString("expires_in");
System.out.println("accessToken=" + accessToken);
System.out.println("expires_in=" + expires_in);
return accessToken;
}
public static String httpRequest(String requestUrl,String requestMethod,String output){
try{
URL url = new URL(requestUrl);
HttpsURLConnection connection = (HttpsURLConnection) url.openConnection();
connection.setRequestMethod(requestMethod);
connection.setDoOutput(true);
connection.setDoInput(true);
connection.setUseCaches(false);
if(null != output){
OutputStream outputStream = connection.getOutputStream();
outputStream.write(output.getBytes("utf-8"));
outputStream.close();
}
// 从输入流读取返回内容
InputStream inputStream = connection.getInputStream();
InputStreamReader inputStreamReader = new InputStreamReader(inputStream, "utf-8");
BufferedReader bufferedReader = new BufferedReader(inputStreamReader);
String str = null;
StringBuffer buffer = new StringBuffer();
while ((str = bufferedReader.readLine()) != null){
buffer.append(str);
}
bufferedReader.close();
inputStreamReader.close();
inputStream.close();
inputStream = null;
connection.disconnect();
return buffer.toString();
}catch(Exception e){
e.printStackTrace();
}
return "";
}
private String sendMsg() throws Exception {
String result = null;
//通过上述方法获取accessToken
String accessToken = getAccessToken();
String requestUrl = "https://api.weixin.qq.com/cgi-bin/message/template/send?access_token="+accessToken;
JSONObject requestBody = new JSONObject();
requestBody.put("touser", "指定用户的openId");
requestBody.put("template_id", "消息模板id");
JSONObject data = new JSONObject();
JSONObject thing3 = new JSONObject();
thing3.put("value", "你的数据");
JSONObject time7 = new JSONObject();
time7.put("value", "你的数据");
JSONObject thing6 = new JSONObject();
thing6.put("value", "你的数据");
JSONObject date3 = new JSONObject();
date3.put("value", "你的数据");
data.put("first", thing3);
data.put("keyword1", time7);
data.put("keyword2", thing6);
data.put("remark", date3);
requestBody.put("data", data);
System.out.println("数据为:" + requestBody);
//将数据通过流的方式进行解析返回
result = httpRequest(requestUrl, "POST", requestBody.toString());
return result;
}
Finally, let’s introduce the message template. This is also a trap, and 70% of the time is spent in this place alone! ! ! !
First, enter the official account management platform, and then find the message template as shown in the figure below. If you don’t have it at the beginning, go to the new function to activate it. Don’t look for subscription notifications. Note that although the two are very similar, the requests are different. , the template is also different.
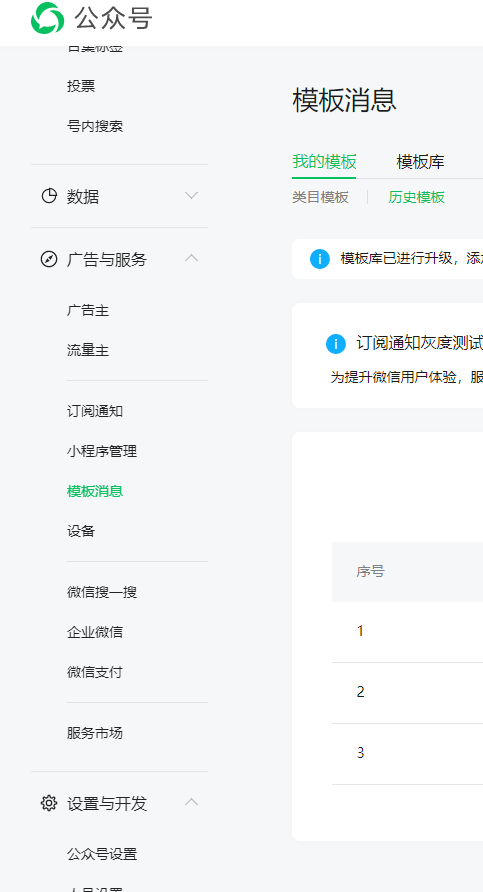
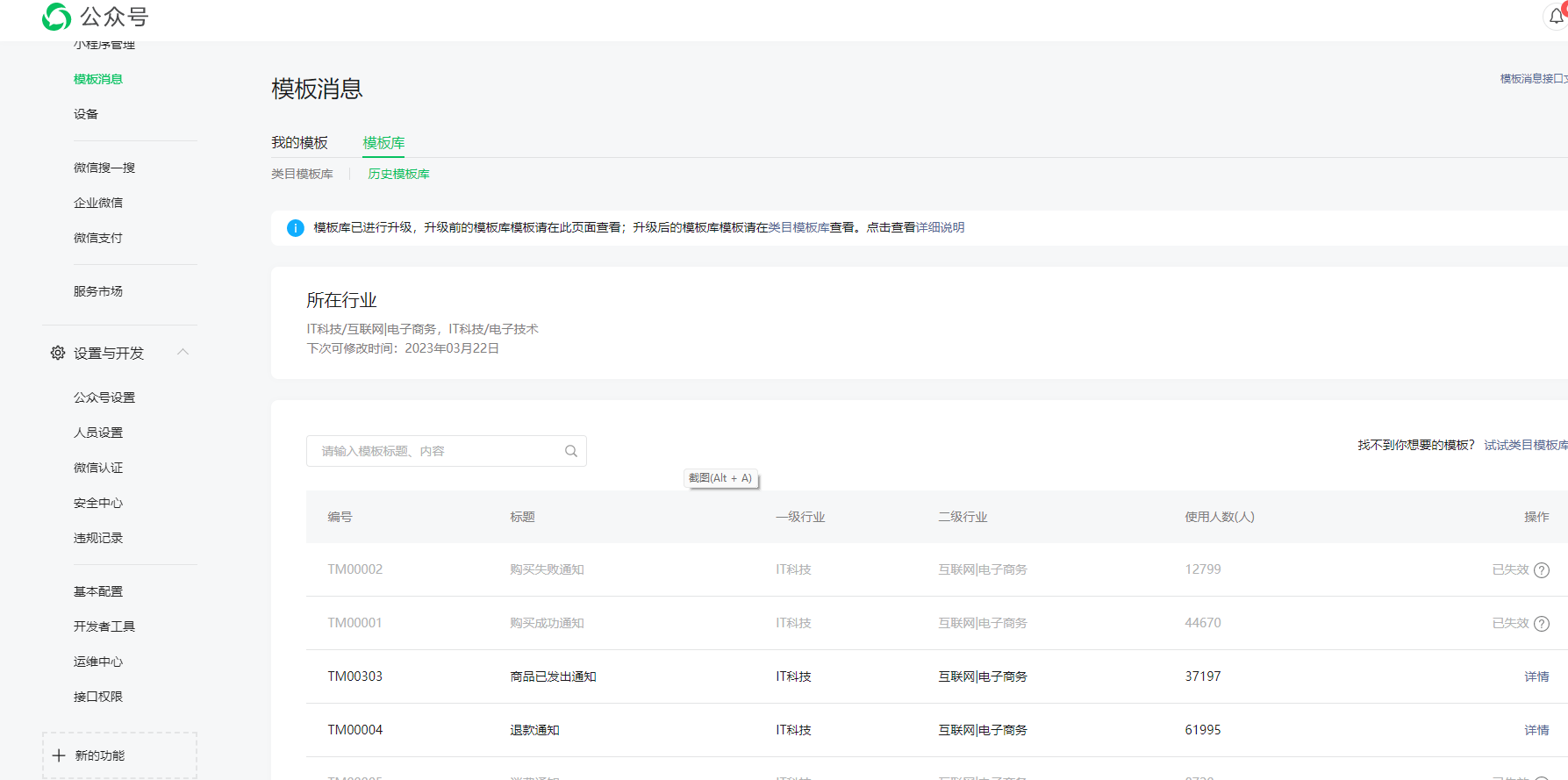
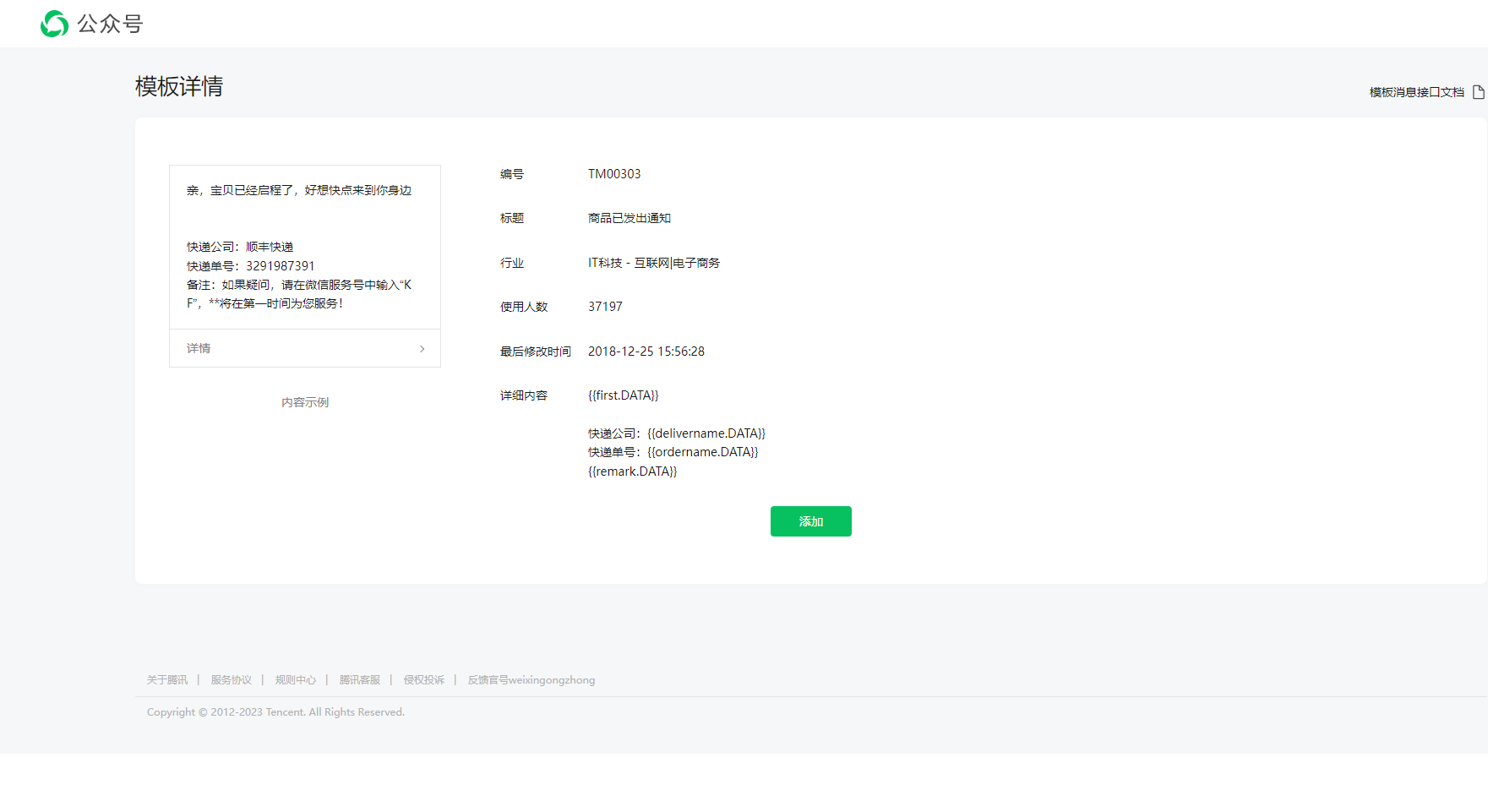
In this way, you can add it step by step, specify the line! ! !