This article records in detail the process of MySQL creating a database, not just the construction steps, but also the knowledge points involved in each step. Generally, there are two ways to create a database, one is commands, and the other is through database management tools. This article mainly records the creation through commands;
Subsequent studies are also based on this database;
Scenes
Creation of a university student achievement management database;
Contains four tables: student table, curriculum table, grade table, and teacher table:
At the same time, enter test data for each table;
The tables need to be associated through foreign keys;
Created by command
Learning to use commands to create a MySQL database has the following advantages:
- Gain a better understanding of how the MySQL database works and internals. By using the command line to operate the database, you can have a clearer understanding of the underlying implementation and interaction of the database, which is very helpful for in-depth learning of the MySQL database.
- It can improve efficiency in some scenarios. In the case of creating, modifying or managing MySQL databases in batches, using the command line can complete tasks more quickly and flexibly than desktop applications, and improve work efficiency.
- Can better grasp the security policy of the MySQL database. When using the command line to create a MySQL database, you need to set the database user name and password and other information, which can help us better grasp the database access control strategy and ensure data security.
- The command line method is more convenient and applicable. In different operating systems and environments, the command line method is common, and has certain applicability and convenience, which greatly increases the portability and ease of use of the MySQL database.
The following is a complete process: the structure is command + explanation + operation effect
Connect to the database
mysql -u root -p
mysql
It is a client command line tool for MySQL database;-u root
Specifies the user name used to log in to the MySQL databaseroot
.root
The user is the super administrator account of the MySQL database and has the highest authority;-p
Indicates that a password is required to log in to the database. After entering this command, you will be prompted for a password.
C:\Users\minch>mysql -u root -p
Enter password: ****
Welcome to the MySQL monitor. Commands end with ; or \g.
Your MySQL connection id is 24
Server version: 8.0.32 MySQL Community Server - GPL
Copyright (c) 2000, 2023, Oracle and/or its affiliates.
Oracle is a registered trademark of Oracle Corporation and/or its
affiliates. Other names may be trademarks of their respective
owners.
Type 'help;' or '\h' for help. Type '\c' to clear the current input statement.
create database
CREATE DATABASE student_score_db;
student_score_db
Create a database in the MySQL database called
mysql> CREATE DATABASE student_score_db;
Query OK, 1 row affected (0.01 sec)
Switch to the newly created database
USE student_score_db;
This command is used to select and enter
student_score_db
the database named . After executing this command, the MySQL database will direct the current session's operations to this database, and all subsequent SQL commands will be executed on this database.
mysql> USE student_score_db;
Database changed
Create a new student table
CREATE TABLE stu_info (
id INT PRIMARY KEY AUTO_INCREMENT, -- 学生ID,主键自增长
name VARCHAR(50) NOT NULL, -- 学生姓名,不允许为空
gender ENUM('男', '女') DEFAULT '男', -- 学生性别,枚举类型,默认为男
age INT DEFAULT 18, -- 学生年龄,默认为18岁
major VARCHAR(50) NOT NULL, -- 学生所在专业,不允许为空
class VARCHAR(50) NOT NULL, -- 学生所在班级,不允许为空
admission_date DATE NOT NULL -- 学生入学日期,不允许为空
);
This command is used to create a table named in the MySQL database
stu_info
, which contains the basic information of students, where:
id
It is the primary key in the table, usingINT
the type to represent the student ID, andPRIMARY KEY AUTO_INCREMENT
the field can be self-increased by setting ;name
is the student’s name, represented byVARCHAR(50)
, settingNOT NULL
means that the field is not allowed to be empty;gender
is the student’s gender,ENUM('男', '女')
represented by an enumeration type, where'男'
and'女'
are enumeration values, and use toDEFAULT '男'
set the default value'男'
;age
is the age of the student,INT
represented by the type, andDEFAULT 18
the default value is set by18
;major
It is the major of the student, use toVARCHAR(50)
indicate, set toNOT NULL
indicate that the field is not allowed to be empty;class
It is the class of the student, use toVARCHAR(50)
indicate, settingNOT NULL
means that the field is not allowed to be empty;admission_date
It is the student’s enrollment date,DATE
represented by the type, and settingNOT NULL
means that the field is not allowed to be empty.
mysql> CREATE TABLE stu_info (
-> id INT PRIMARY KEY AUTO_INCREMENT, -- 学生ID,主键自增长
-> name VARCHAR(50) NOT NULL, -- 学生姓名,不允许为空
-> gender ENUM('男', '女') DEFAULT '男', -- 学生性别,枚举类型,默认为男
-> age INT DEFAULT 18, -- 学生年龄,默认为18岁
-> major VARCHAR(50) NOT NULL, -- 学生所在专业,不允许为空
-> class VARCHAR(50) NOT NULL, -- 学生所在班级,不允许为空
-> admission_date DATE NOT NULL -- 学生入学日期,不允许为空
-> );
Query OK, 0 rows affected (0.02 sec)
Enter student data
INSERT INTO stu_info (name, gender, age, major, class, admission_date)
VALUES
('张三', '男', 19, '计算机科学与技术', '2022计算机1班', '2022-09-01'),
('李四', '男', 20, '软件工程', '2021软件1班', '2021-09-01'),
('王五', '女', 18, '信息管理与信息系统', '2023信息1班', '2023-09-01'),
('赵六', '男', 19, '计算机科学与技术', '2022计算机2班', '2022-09-01'),
('刘七', '女', 20, '数据科学与大数据技术', '2021数据1班', '2021-09-01'),
('钱八', '男', 18, '网络工程', '2023网络1班', '2023-09-01');
This command is
stu_info
a statement used to insert multiple pieces of student information data into the table. Specifically, this command will insert 6 records into the table, each of which isVALUES
specified by a clause, each clause represents the value of a record, and multipleVALUES
clauses are separated by commas.
mysql> INSERT INTO stu_info (name, gender, age, major, class, admission_date)
-> VALUES
-> ('张三', '男', 19, '计算机科学与技术', '2022计算机1班', '2022-09-01'),
-> ('李四', '男', 20, '软件工程', '2021软件1班', '2021-09-01'),
-> ('王五', '女', 18, '信息管理与信息系统', '2023信息1班', '2023-09-01'),
-> ('赵六', '男', 19, '计算机科学与技术', '2022计算机2班', '2022-09-01'),
-> ('刘七', '女', 20, '数据科学与大数据技术', '2021数据1班', '2021-09-01'),
-> ('钱八', '男', 18, '网络工程', '2023网络1班', '2023-09-01');
Query OK, 6 rows affected (0.01 sec)
Records: 6 Duplicates: 0 Warnings: 0
Create curriculum
CREATE TABLE course (
id INT PRIMARY KEY AUTO_INCREMENT, -- 课程ID,主键自增长
name VARCHAR(50) NOT NULL, -- 课程名称,不允许为空
teacher_id INT NOT NULL, -- 教师ID,外键,参考教师表中的ID字段
credit DOUBLE NOT NULL -- 课程学分,不允许为空
);
This command is
course
the statement used to create a table named . Specifically, the table contains the following columns:
id
: course ID, the type isINT
, is the primary key column, and usesAUTO_INCREMENT
the attribute to indicate self-growth;name
: course name, the type isVARCHAR(50)
, not allowed to be empty;teacher_id
: Teacher ID, the type isINT
, not allowed to be empty, it is a foreign key column, referring to the column in the teacher tableID
;credit
: course credit, the type isDOUBLE
, not allowed to be empty.Among them, the primary key column specifies
PRIMARY KEY
a constraint, which forces the value of this column to be unique in the table, and usesAUTO_INCREMENT
the keyword to indicate that the value of this column will be automatically incremented.
mysql> CREATE TABLE course (
-> id INT PRIMARY KEY AUTO_INCREMENT, -- 课程ID,主键自增长
-> name VARCHAR(50) NOT NULL, -- 课程名称,不允许为空
-> teacher_id INT NOT NULL, -- 教师ID,外键,参考教师表中的ID字段
-> credit DOUBLE NOT NULL -- 课程学分,不允许为空
-> );
Query OK, 0 rows affected (0.02 sec)
Enter curriculum data
INSERT INTO course (name, teacher_id, credit)
VALUES
('高等数学I', 1, 4.0),
('Java程序设计', 2, 3.0),
('计算机组成原理', 3, 4.0),
('数据库原理与应用', 4, 3.5),
('操作系统原理', 5, 3.5),
('数据结构与算法', 6, 4.5);
This is to insert data into the curriculum, the specific syntax has also been mentioned above, refer to the student data entry module;
mysql> INSERT INTO course (name, teacher_id, credit)
-> VALUES
-> ('高等数学I', 1, 4.0),
-> ('Java程序设计', 2, 3.0),
-> ('计算机组成原理', 3, 4.0),
-> ('数据库原理与应用', 4, 3.5),
-> ('操作系统原理', 5, 3.5),
-> ('数据结构与算法', 6, 4.5);
Query OK, 6 rows affected (0.00 sec)
Records: 6 Duplicates: 0 Warnings: 0
Create grade sheet
CREATE TABLE score (
id INT PRIMARY KEY AUTO_INCREMENT, -- 成绩ID,主键自增长
student_id INT NOT NULL, -- 学生ID,外键,参考学生表中的ID字段
course_id INT NOT NULL, -- 课程ID,外键,参考课程表中的ID字段
score DOUBLE NOT NULL -- 学生得分,不允许为空
);
Create a table named in the MySQL database
score
with the following columns:
id
: Achievement ID, the type isINT
, is the primary key column, and usesAUTO_INCREMENT
the attribute to indicate self-growth;student_id
: Student ID, the type isINT
, not allowed to be empty, it is a foreign key column, referring to the column in the student tableID
;course_id
: course ID, the type isINT
, not allowed to be empty, it is a foreign key column, referring to the column in the course tableID
;score
: Student score, the type isDOUBLE
, not allowed to be empty.Among them, the primary key column specifies
PRIMARY KEY
a constraint, which forces the value of this column to be unique in the table, and usesAUTO_INCREMENT
the keyword to indicate that the value of this column will be automatically incremented.
mysql> CREATE TABLE score (
-> id INT PRIMARY KEY AUTO_INCREMENT, -- 成绩ID,主键自增长
-> student_id INT NOT NULL, -- 学生ID,外键,参考学生表中的ID字段
-> course_id INT NOT NULL, -- 课程ID,外键,参考课程表中的ID字段
-> score DOUBLE NOT NULL -- 学生得分,不允许为空
-> );
Query OK, 0 rows affected (0.01 sec)
Enter grade data
INSERT INTO score (student_id, course_id, score)
VALUES
(1, 1, 85.0),
(1, 2, 90.5),
(2, 3, 78.0),
(2, 4, 92.0),
(3, 5, 88.5),
(3, 6, 95.0);
Ditto!
mysql> INSERT INTO score (student_id, course_id, score)
-> VALUES
-> (1, 1, 85.0),
-> (1, 2, 90.5),
-> (2, 3, 78.0),
-> (2, 4, 92.0),
-> (3, 5, 88.5),
-> (3, 6, 95.0);
Query OK, 6 rows affected (0.01 sec)
Records: 6 Duplicates: 0 Warnings: 0
create teacher table
CREATE TABLE teacher (
id INT PRIMARY KEY AUTO_INCREMENT, -- 教师ID,主键自增长
name VARCHAR(50) NOT NULL, -- 教师姓名,不允许为空
gender ENUM('男', '女') DEFAULT '男', -- 教师性别,枚举类型,默认为男
age INT DEFAULT 35, -- 教师年龄,默认为35岁
title VARCHAR(50) NOT NULL -- 教师职称,不允许为空
);
Create a table named in the MySQL database
teacher
with the following columns:
id
: Teacher ID, the type isINT
, is the primary key column, and usesAUTO_INCREMENT
the attribute to indicate self-growth;name
: teacher name, the type isVARCHAR(50)
, not allowed to be empty;gender
: Teacher gender, the type isENUM('男', '女')
, and the default value is'男'
. Because there are only two possibilities for gender, useENUM
the enumeration type to limit its value range;age
: teacher age, type isINT
, default value is35
. Because age can have many possibilities, and the default value is35
, so directly useINT
the type and set the default value;title
: Teacher title, the type isVARCHAR(50)
, not allowed to be empty.
mysql> CREATE TABLE teacher (
-> id INT PRIMARY KEY AUTO_INCREMENT, -- 教师ID,主键自增长
-> name VARCHAR(50) NOT NULL, -- 教师姓名,不允许为空
-> gender ENUM('男', '女') DEFAULT '男', -- 教师性别,枚举类型,默认为男
-> age INT DEFAULT 35, -- 教师年龄,默认为35岁
-> title VARCHAR(50) NOT NULL -- 教师职称,不允许为空
-> );
Query OK, 0 rows affected (0.02 sec)
Enter teacher data
INSERT INTO teacher (name, gender, age, title)
VALUES
('张老师', '男', 38, '教授'),
('李老师', '女', 39, '副教授'),
('王老师', '男', 35, '讲师'),
('赵老师', '女', 36, '教授'),
('刘老师', '男', 40, '副教授'),
('钱老师', '女', 37, '讲师');
Ditto!
mysql> INSERT INTO teacher (name, gender, age, title)
-> VALUES
-> ('张老师', '男', 38, '教授'),
-> ('李老师', '女', 39, '副教授'),
-> ('王老师', '男', 35, '讲师'),
-> ('赵老师', '女', 36, '教授'),
-> ('刘老师', '男', 40, '副教授'),
-> ('钱老师', '女', 37, '讲师');
Query OK, 6 rows affected (0.01 sec)
Records: 6 Duplicates: 0 Warnings: 0
add foreign key
-- 在课程表中添加外键,参考教师表的ID字段
ALTER TABLE course ADD CONSTRAINT fk_course_teacher FOREIGN KEY (teacher_id) REFERENCES teacher(id);
-- 在成绩表中添加外键,参考学生表和课程表的ID字段
ALTER TABLE score ADD CONSTRAINT fk_score_student FOREIGN KEY (student_id) REFERENCES stu_info(id);
ALTER TABLE score ADD CONSTRAINT fk_score_course FOREIGN KEY (course_id) REFERENCES course(id);
Take the first command as an example;
This SQL statement
course
adds a foreign key constraint on the table, which contains the following keywords (for specific explanation, refer to the Q&A section):
ALTER TABLE
: used to modify the existing table structure;course
: the name of the table to be modified;ADD CONSTRAINT
: Add a constraint;fk_course_teacher
: the name of the new constraint;FOREIGN KEY
: Specify the constraint as a foreign key constraint;(teacher_id)
: The name of the foreign key column,teacher
which column in the reference table. The content in brackets indicates the column name;REFERENCES teacher(id)
: The table and column referenced by the foreign key column.REFERENCES
The keyword specifies the name of the referenced table, and the content in the brackets indicates a column name of the table, which is used as the reference value of the foreign key column.To sum up, the function of this SQL statement is to set the column
course
in the tableteacher_id
as a foreign key column, referring to the columnteacher
in the tableid
. In this way, when inserting, updating, or deleting data, the MySQL database will automatically check whether the foreign key constraints are met, thereby ensuring data consistency between tables.It should be noted that in order to use foreign key constraints, the column
teacher
in the referenced table must first be createdid
and set as the primary key. Because the role of foreign key constraints is to ensure that a column value in the reference table must exist in a column in the current table, the column in the reference table must be set to be unique and non-null.
mysql> ALTER TABLE course ADD CONSTRAINT fk_course_teacher FOREIGN KEY (teacher_id) REFERENCES teacher(id);
Query OK, 6 rows affected (0.05 sec)
Records: 6 Duplicates: 0 Warnings: 0
mysql> ALTER TABLE score ADD CONSTRAINT fk_score_student FOREIGN KEY (student_id) REFERENCES stu_info(id);
Query OK, 6 rows affected (0.05 sec)
Records: 6 Duplicates: 0 Warnings: 0
mysql> ALTER TABLE score ADD CONSTRAINT fk_score_course FOREIGN KEY (course_id) REFERENCES course(id);
Query OK, 6 rows affected (0.05 sec)
Records: 6 Duplicates: 0 Warnings: 0
Verify creation
show tables;
This command is to display all tables in the current database, and the results are as follows:
The specific query table data situation will be introduced in the following blog;
mysql> show tables;
+----------------------------+
| Tables_in_student_score_db |
+----------------------------+
| course |
| score |
| stu_info |
| teacher |
+----------------------------+
4 rows in set (0.00 sec)
You can also view it through the database management tool. The following is the data table viewed with Navicat, and the foreign key relationship is also displayed clearly;
Navicat installation: https://blog.jiumoz.com/archives/navicatpremium16-de-po-jie-yu-an-zhuang
Navicat connection: https://blog.jiumoz.com/archives/mysql-an-zhuang-pei-zhi-yu-navicat-lian-jie
Q&A
ALTER TABLE keyword
ALTER TABLE
It is an SQL statement, which is used to modify the structure of a table in an existing relational database, including operations such as adding, modifying, and deleting columns. ThroughALTER TABLE
the command, we can expand or shrink the created table, which improves the flexibility and maintainability of the data.For example, we can use to
ALTER TABLE
add new columns, update existing column data types, add constraints to tables, etc. This command is an essential function in a relational database management system (RDBMS).It should be noted that
ALTER TABLE
the command needs to be used with caution, because this command may cause the loss of table data or destroy the integrity of the table.
Use ALTER TABLE to add new columns
When we need to add a new column to an existing database table, we can use
ALTER TABLE
the command to achieve it. Here is anALTER TABLE
example SQL statement using add a new column:ALTER TABLE 表名 ADD 列名 数据类型;
Among them,
表名
indicates the name of the target table to be modified,列名
indicates the name of the new column to be added, and数据类型
indicates the data type of the column.For example, suppose we have a
students
table named and now want to add a new column in the tableage
, its data type is integer. You can use the following SQL statement to achieve:ALTER TABLE students ADD age INT;
After the above statement is executed,
students
a new column will be added to the tableage
, and its data type is integer.It should be noted that when we add a new column to an existing table, the default value of the new column is NULL. If you need to assign a default value to the new column, you can use the keyword
DEFAULT
. For example:ALTER TABLE students ADD address VARCHAR(50) DEFAULT 'N/A';
The above SQL statement will
students
add a new column in the tableaddress
, its data type is string type, and the default value is assigned'N/A'
.
ADD CONSTRAINT keyword
ADD CONSTRAINT
It is an SQL statement used to add constraints (constraint) to a relational database table, and is usuallyALTER TABLE
used in conjunction with the command. Many types of constraints can beADD CONSTRAINT
added using , such as primary key constraints, unique constraints, foreign key constraints, and more.The following is a sample SQL statement to add a primary key constraint:
ALTER TABLE table_name ADD CONSTRAINT pk_column PRIMARY KEY (column1, column2);
where
table_name
is the target table name,pk_column
is the name of the primary key constraint to be added,column1
andcolumn2
is the column name to be included in the primary key constraint.It should be noted that before executing this command, you should ensure that the target table already contains the required columns and data, and these columns should be defined as non-nullable (ie NOT NULL). Otherwise, adding constraints will fail because the constraints cannot be satisfied.
What does constraint mean
Constraint (Constraint) is a rule used to define the data rules and integrity in the table to ensure the correctness, consistency and validity of the data. Constraints can limit the value range, necessity, and uniqueness of certain columns in a table, and can also define relationships between tables, such as primary keys and foreign keys.
Common MySQL constraints include:
- PRIMARY KEY: The primary key constraint is used to uniquely identify each row of data in the table.
- UNIQUE: Unique constraint, used to limit the data in the column cannot be repeated.
- NOT NULL: Not-null constraint, used to restrict the data in the column cannot be empty.
- CHECK: Check constraints, used to limit the data in the column must meet the specified conditions.
- FOREIGN KEY: Foreign key constraints are used to define the relationship between two tables to ensure that the data in the child table always matches the data in the parent table.
It should be noted that in MySQL, constraints can be defined together when the table is created, or can be added and modified through the ALTER TABLE statement after the table is created.
Difference between primary key and primary key constraint
Primary Key and Primary Key Constraint are closely related concepts, but not identical.
A primary key is a column or columns used to uniquely identify each record in a relational database table (ensure that their combined values are unique in the entire table) . The columns contained in the primary key must meet the conditions that each row of data is unique and non-null. The primary key is usually used to query, update, and delete data on the table. In a table, the primary key is defined by specific column values, rather than an independent constraint defined on the table.
A primary key constraint is a constraint applied to the primary key column in order to ensure the integrity of the primary key. It is a special constraint that is mainly used to specify that the primary key in the table must meet the conditions of uniqueness and non-nullness. Primary key constraints are usually implemented by a relational database management system (RDBMS), which prevents users from performing operations on primary key columns that will destroy data integrity, such as modifying or deleting certain data in primary key columns.
In practical applications, we usually use the primary key as a basic element, and use the primary key constraint to ensure the integrity and uniqueness of the column data contained in the primary key, thereby improving the stability and security of the database.
Can primary key constraints constrain non-primary key columns?
In a relational database, a primary key constraint is a constraint applied to the primary key column in order to ensure the uniqueness and non-nullness of the primary key column in the table. Therefore, the primary key constraint can only be applied to the primary key column in the table, but not to other columns.
In addition to primary key constraints, in relational databases, there are also unique constraints (UNIQUE Constraint) that can be applied to different columns in a table to ensure that the values of these columns are unique. In addition, the check constraint (CHECK Constraint) can restrict the non-primary key columns in the table in some cases to prevent unexpected or wrong data.
Application of MySQL Constraints in Development
A MySQL constraint is a rule that restricts the values of certain columns in a database table or the relationship between them. It ensures data integrity and consistency, avoiding invalid or wrong data being stored in the database. Here's how the MySQL constraints apply in development:
- Primary key constraint: It can ensure that each row of data in the table has a unique identifier, and this identifier cannot be empty. Primary key constraints also improve performance when querying and sorting.
- Foreign key constraints: Can ensure that fields in a table that point to other tables contain only values that exist in that table. Foreign key constraints also prevent accidental deletion of data (such as deleting data referenced by other tables).
- Unique constraint: It can ensure that the value of a certain column in the table is unique, and can also prevent null values from appearing in a specific column.
- Not-null constraint: It can ensure that a column in the table is not empty.
- Check Constraints: Additional rules can be defined to ensure that the data values of a column or columns conform to the specification. For example, you can use a check constraint to ensure that a date field is always a date after the current date.
- Default value constraint: You can specify a default value for a field, and if the field is not written when inserting data, the default value will be automatically filled.
- Composite Constraints: Rules can be imposed on multiple columns simultaneously to ensure that the information in a table is structured correctly.
Application example of MySQL constraints in fastapi
When using the MySQL database in FastAPI, you can define and manage constraints through SQLAlchemy and name them. Here is an example showing how to create and name constraints in FastAPI and MySQL:
from sqlalchemy import Column, Integer, String, ForeignKey from sqlalchemy.orm import relationship from sqlalchemy.ext.declarative import declarative_base Base = declarative_base() class User(Base): __tablename__ = 'users' id = Column(Integer, primary_key=True) name = Column(String(50), nullable=False, unique=True, name="unique_user_name") age = Column(Integer, nullable=False) class Item(Base): __tablename__ = 'items' id = Column(Integer, primary_key=True) name = Column(String(50), nullable=False, name="item_name_not_null") price = Column(Integer, nullable=False) user_id = Column(Integer, ForeignKey('users.id')) user = relationship("User", backref="items")
In the above example, we added constraint names for the name column in the users table and the name column in the items table: unique_user_name and item_name_not_null. The names of these constraints make it easier for other developers to find and understand the table structure as well as for code maintenance and debugging.
To use the model defined above, and create its corresponding MySQL table, you need to use SQLAlchemy and the MySQL database to perform the following steps:
from sqlalchemy import create_engine from sqlalchemy.orm import sessionmaker SQLALCHEMY_DATABASE_URL = "mysql://user:password@localhost/db_name" engine = create_engine( SQLALCHEMY_DATABASE_URL ) SessionLocal = sessionmaker(autocommit=False, autoflush=False, bind=engine) Base.metadata.create_all(bind=engine)
In the above example, we first create a SQLAlchemy engine and define a SessionLocal class for database access. Then, we use the create_all() method to create all the table structures and associate them with the connected MySQL database.
Whether the constraint name should correspond to the constraint name in the database
If a MySQL constraint name is defined in code, its corresponding constraint name should match it in the database. This is because the MySQL constraint name is a kind of metadata defined when the table is created and will be stored in the database system tables. When modifying or dropping existing constraints, MySQL needs to know exactly which constraints must be changed.
If the MySQL constraint names defined in the code do not match those stored in the actual database, an error will result. For example, if you define a unique constraint called "unique_user_name", but MySQL names it "uq_users_name_5588" when the table is actually created, errors will occur when querying and updating related data.
Therefore, when adding MySQL constraint names in development, it is important to ensure that each constraint name matches the name actually stored in the database. Additionally, names that are too long or unclear should be avoided to quickly locate constraint issues in the future.
Original address: https://blog.jiumoz.com/archives/sql-bi-ji-1mysql-chuang-jian-shu-ju-ku
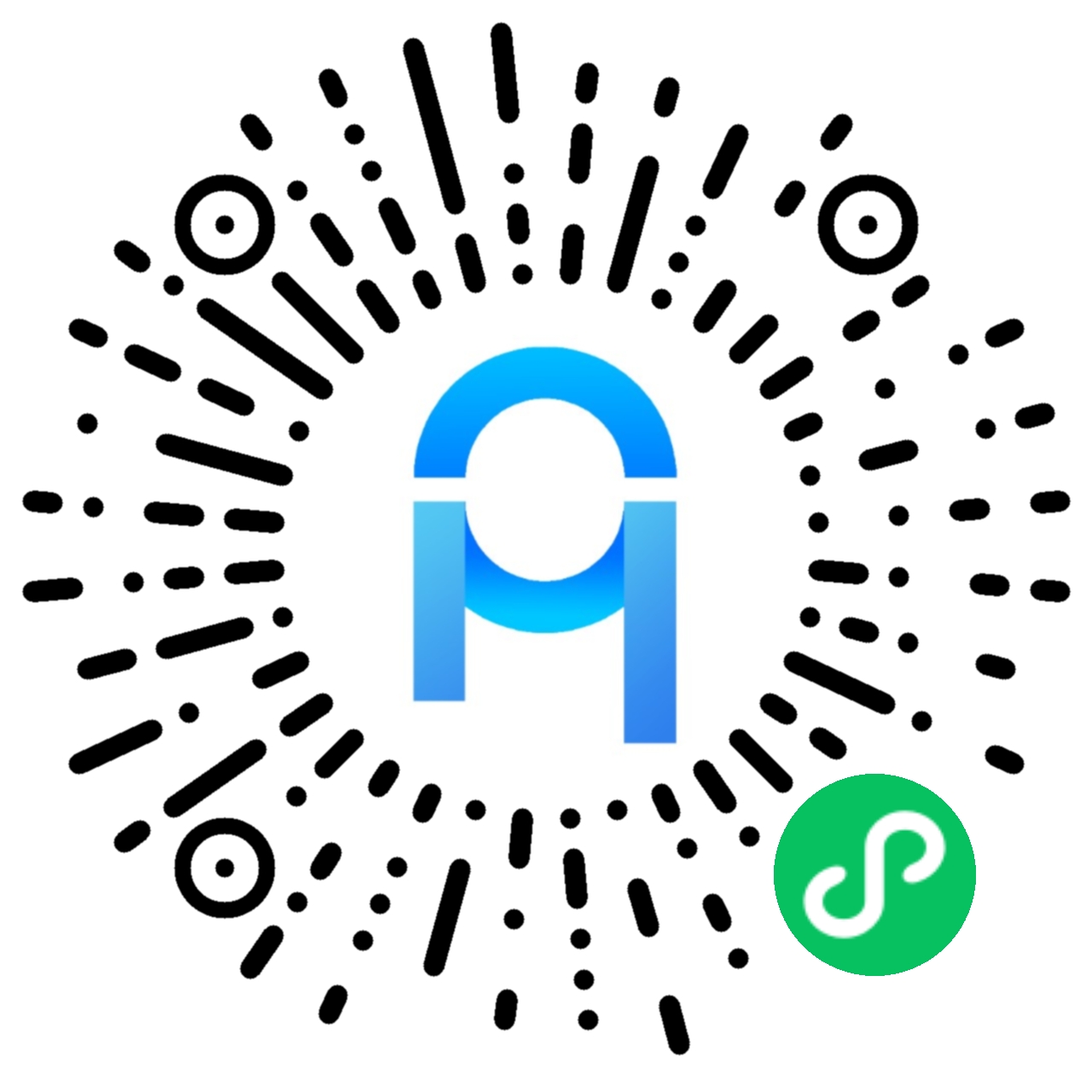