get text width
Method 1: First draw the rectangular area where the text is located, and then get the width of the rectangular area
Rect rect = new Rect();
mPaint.getTextBounds(text,0,text.length(), rect);
canvas.drawRect(rect, mPaint);
float textWidth1 = rect.width();
Log.d("111","textWidth:"+textWidth1);
In the above method, since the rectangle frame is close to the text, there is no extra space.
Method 2: Measure the text width directly through the measureText method of Paint
float textWidth2 = mPaint.measureText(text);
Log.d("111", "textWidth:"+textWidth2);
canvas.drawLine(0, rect.bottom + 20, textWidth2, rect.bottom + 20, mPaint);
The width calculated by this method will add the space at the beginning and the end, which is the space between text and text, and exists for aesthetics. It would be more intuitive if underlined.
Method 3: Calculate the width of each text, and finally calculate the sum
float[] textWidths =new float[text.length()];
mPaint.getTextWidths(text, textWidths);
float textWidth1 =0;
for(int i=0;i<textWidths.length;i++){
textWidth1 = textWidth1 + textWidths[i];
}
Log.d("111","textWidth:"+textWidth1);
The width calculated by this method is the same as that of Method 2.
TextPaint textPaint =new TextPaint();
textPaint.setTextSize(280);
textPaint.setColor(Color.BLUE);
textPaint.setStrokeWidth(8);
textPaint.setAntiAlias(true);
textPaint.setStyle(Paint.Style.FILL);
float textWidth1 = Layout.getDesiredWidth(text,textPaint);
Log.d("111","textWidth:"+textWidth1);
The width calculated by the method is consistent with the previous two.
get text height
When drawing text on the control, it is often necessary to consider the center position of the text, which requires calculating and obtaining the width and height of the entire text.
Look at the picture to understand the font baseline
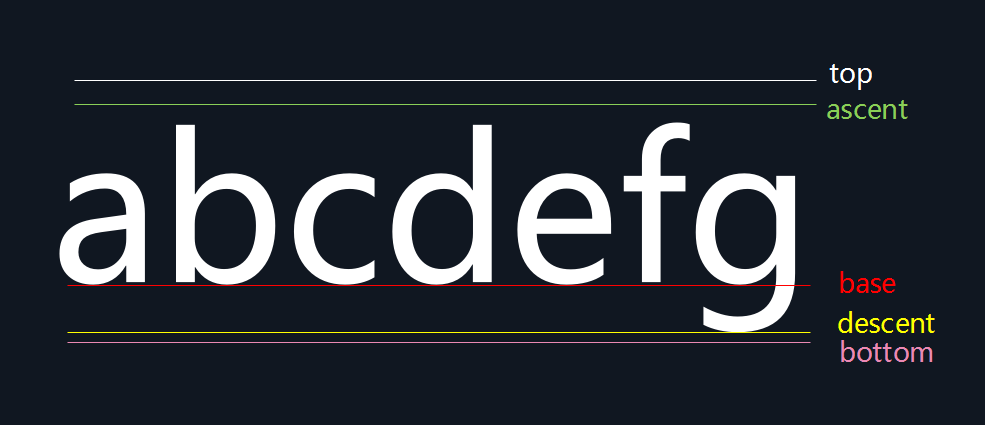
All the above properties are encapsulated in the FontMetrics class, through which the width and height of the text can be obtained and calculated
top: The maximum distance above the baseline (base) that is considered the highest glyph in a font of a certain size.
ascent: The recommended distance above the baseline (base) in a single line of text.
descent: The recommended distance below the baseline in a single line of text.
bottom: In a font of a certain size, it is regarded as the lowest glyph, the maximum distance below the baseline (base).
If we want to calculate the height of this text, just use (descent-ascent)
Through the above picture, I have a general understanding of the baseline. The specific code is
private void init(){
mPaint = new Paint();
mPaint.setColor(Color.BLUE);
mPaint.setAntiAlias(true);
mPaint.setStrokeWidth(8);
mPaint.setTextSize(280);
mPaint.setStyle(Paint.Style.FILL);
}
@Override
protected void onDraw(Canvas canvas){
super.onDraw(canvas);
canvas.translate(200,400);
mPaint.setColor(Color.parseColor("#65A21F"));
canvas.drawLine(-200,0, canvas.getWidth()-200,0, mPaint);
canvas.drawLine(0,-400,0, canvas.getHeight()-400, mPaint);
mPaint.setColor(Color.BLACK);
mPaint.setStrokeWidth(2);
mPaint.setStyle(Paint.Style.STROKE);
Rect rect = new Rect();
mPaint.getTextBounds(text,0,text.length(), rect);
canvas.drawRect(rect, mPaint);
float textHeight1 = rect.height();
Log.d("111","textHeight:"+textHeight1);
float textWidth = mPaint.measureText(text);//获取文本的宽度
Paint.FontMetrics fontMetrics = mPaint.getFontMetrics();
mPaint.setStyle(Paint.Style.FILL);
mPaint.setStrokeWidth(2);
mPaint.setColor(Color.RED);
canvas.drawLine(0, fontMetrics.ascent, mPaint.measureText(text), fontMetrics.ascent, mPaint);
mPaint.setTextSize(50);
canvas.drawText("ascent", textWidth, fontMetrics.ascent, mPaint);
mPaint.setColor(Color.parseColor("#6EAD24"));
canvas.drawLine(0, fontMetrics.bottom, textWidth, fontMetrics.bottom, mPaint);
mPaint.setTextSize(50);
canvas.drawText("bottom", textWidth, fontMetrics.bottom +30, mPaint);
mPaint.setColor(Color.parseColor("#13AA9C"));
canvas.drawLine(0, fontMetrics.descent, textWidth, fontMetrics.descent, mPaint);
mPaint.setTextSize(50);
canvas.drawText("descent", textWidth, fontMetrics.descent, mPaint);
mPaint.setColor(Color.parseColor("#9C17B3"));
canvas.drawLine(0,0, textWidth,0, mPaint);
mPaint.setTextSize(50);
canvas.drawText("baseline", textWidth, fontMetrics.leading, mPaint);
mPaint.setColor(Color.parseColor("#DF3A72"));
canvas.drawLine(0, fontMetrics.top, textWidth, fontMetrics.top, mPaint);
mPaint.setTextSize(50);
canvas.drawText("top", textWidth, fontMetrics.top, mPaint);
mPaint.setTextSize(280);
mPaint.setColor(Color.BLUE);
mPaint.setStrokeWidth(8);
mPaint.setStyle(Paint.Style.FILL);
canvas.drawText(text,0,0, mPaint);}
Method 1: First draw the rectangular area where the text is located, and then get the height of the rectangular area
Rect rect = new Rect();
mPaint.getTextBounds(text,0,text.length(), rect);
canvas.drawRect(rect, mPaint);
float textHeight1 = rect.height();
Log.d("111","textHeight:"+textHeight1);
Method 2: Obtain measurement data through getFontMetrics() or getFontMetricsInt()
To calculate the height only need descent-ascent
Paint.FontMetrics fontMetrics = mPaint.getFontMetrics();
float height1 = fontMetrics.descent - fontMetrics.ascent;
Log.d("111", "文本的推荐高度:"+height1);
float height2 = fontMetrics.bottom - fontMetrics.top;
Log.d("111", "文本的最大高度:"+height2);
Method 3: Realized by getFontSpacing
float height3 = mPaint.getFontSpacing();
Log.d("aaa", "文本的高度:"+height3);