Introduction to TextView
Writing is a common way for us to convey information. To display text on Android applications, we usually use TextView. We already know how to get the TextView in the layout before, and we also know setText()
how to modify the displayed text.
Combined with our actual life and learning experience, what aspects can we control when writing? Text content; text color; size; background, etc.
The simplest TextView :
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
Thanks to the powerful prompt function of as, when we enter <Te in the layout, as may prompt a pop-up.
Just press Enter or double-click the TextView.
Here we focus on two basic properties layout_width
and layout_height
. Represents the width and height settings of TextView respectively. In fact, these two properties are properties of View. TextView inherits from View. The width and height attributes are basic attributes and must be set.
width and height attributes
layout_width/layout_height can be filled with wrap_content, match_parent or specific values.
- wrap_content : Indicates that the width/height of the control can be determined by the content. For TextView, the longer the text, the wider its width, up to the maximum width/height allowed by the parent view (upper container ).
- match_parent : Indicates that the width/height of the control reaches the maximum value allowed by the parent view. In layman's terms, it means filling up the space.
- We can also enter specific values . For example, 80dp. dp is a unit in Android, usually used to specify the width, height, spacing distance, etc. of controls. Similarly, the unit used to represent text size is sp in Android.
Show text
Displaying text may be the most important use of TextView. To set text in the layout, use the text attribute.
<TextView
android:id="@+id/tv1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="新手教程" />
<TextView
android:id="@+id/sample_tv"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/app_name" />
This involves a coding style issue. The IDs are set for TextView respectively above. Some people like camel case style, such as sampleTv.
We can see that there are many ways to set text. You can write the content directly (hard code), or you can use string resources. If you write the content directly, as will give a yellow warning, and it is recommended that the user switch to the @string resource method. You can see the warning of as by moving the mouse over as.
To use the @string resource, we first look at another xml file, strings.xml. It's inside res/values.
<string name="app_name">2021</string>
The resource naming style is also lowercase letters and underlined.
We can use R to find many resources in res.
As we mentioned earlier, you can use the setText method of TextView to set text content, such as setText(“123”). You can also pass in the name (number) of the text resource, similar to setText(R.string.app_name). It should be noted that R.string.app_name itself is an int number, and TextView will find the corresponding resource based on this number. If you call setText(123) like this, the following error will most likely be reported.
android.content.res.Resources$NotFoundException: String resource ID #0x0
at android.content.res.Resources.getText(Resources.java:360)
Text settings
Generally speaking, we will set the color, background, etc. of the TextView text.
- textColor sets the font color
- textSize sets the font size
- textStyle sets the font style
textStyle sets the font style
- normal no special effects, default value
- italic italic
- bold bold
Set in xml:
Example 1: Set italics
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Fisher"
android:textColor="#000000"
android:textStyle="italic" />
Example 2: Set italics and bold
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="bold|italic"
android:textColor="#000000"
android:textStyle="bold|italic" />
Set in code
Use TextView's setTypeface method to set the font effect.
tv1.setTypeface(null, Typeface.NORMAL); // 普通
tv1.setTypeface(null, Typeface.BOLD); // 加粗
tv2.setTypeface(null, Typeface.ITALIC); // 斜体
tv3.setTypeface(null, Typeface.BOLD_ITALIC); // 加粗和斜体
setTypeface(@Nullable Typeface tf, @Typeface.Style int style) has 2 parameters. The first one is the font, which can be ignored here. The second is the effect, which includes normal, bold, italic, bold and italic.
Font (font library)
By default, the typeface attribute of TextView supports three fonts: sans, serif and monospace. The system defaults to sans as the font for text display. But these three fonts only support English. If Chinese is displayed, no matter which one of these three fonts is selected, the display effect will be the same.
Set font in layout : Use android:typeface
to set font.
<!-- sans字体 -->
<TextView
android:text="Hello,World"
android:typeface="sans" />
<!-- serifs字体 -->
<TextView
android:text="Hello,World"
android:typeface="serif" />
<!-- monospace字体 -->
<TextView
android:text="Hello,World"
android:typeface="monospace" />
Fonts used in code :
tv.setTypeface(Typeface.SERIF);
tv.setTypeface(Typeface.SANS_SERIF);
tv.setTypeface(Typeface.MONOSPACE);
Introducing the font library requires introducing ttf font files. Place the font files in the assets/font directory. AssetManager is used in the code to obtain fonts.
For example: Set the font in Activity.
TextView tv1 = findViewById(R.id.tv1);
Typeface tf = Typeface.createFromAsset(getAssets(), "fonts/otherFont.ttf");
tv1.setTypeface(tf); // 使用字体
Share one last time
[Produced by Tencent Technical Team] Getting started with Android from scratch to mastering it, Android Studio installation tutorial + full set of Android basic tutorials
Android programming introductory tutorial
Java language basics from entry to familiarity
Kotlin language basics from entry to familiarity
Android technology stack from entry to familiarity
Comprehensive learning on Android Jetpack
For novices, it may be difficult to install Android Studio. You can watch the following video to learn how to install and run it step by step.
Android Studio installation tutorial
With the Java stage of learning, it is recommended to focus on video learning at this stage and supplement it with book checking and filling in gaps. If you mainly focus on books, you can type the code based on the book's explanations, supplemented by teaching videos to check for omissions and fill in the gaps. If you encounter problems, you can go to Baidu. Generally, many people will encounter entry-level problems and give better answers.
You need to master basic knowledge points, such as how to use the four major components, how to create Service, how to layout, simple custom View, animation, network communication and other common technologies.
A complete set of zero-based tutorials has been prepared for you. If you need it, you can add the QR code below to get it for free.
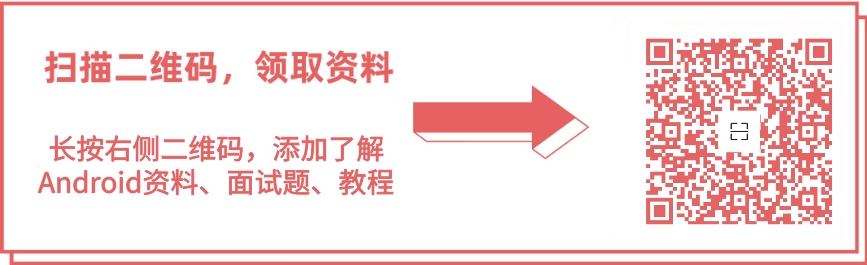
A complete set of basic Android tutorials