Software: A collection of calculations and data and instructions organized in a specific order
System software Application software
Application = algorithm + data structure
machine language assembly language high level language
1996 SUN released JDK1.0
C/S (c client) B/S
class object inheritance encapsulation polymorphism
The bottom layer of the computer stores data in complement code
Chapter 1: Getting to know java
oop (object-oriented
)
1. Java language features
Robustness: The pointer in C language is removed, and the automatic garbage collection mechanism (memory overflow and memory leak still occurs)
Cross-platform: compile once and run everywhere
Half compiled, half interpreted: just-in-time compilation
Identifier: alphanumeric underscore dollar sign
The number cannot start, cannot contain spaces, and is case sensitive
Key words can be concatenated with underscores
package name: lowercase
Classes, interfaces: Big CamelCase
Variable name, method name: small hump
Always bright name: XXX_YYY_ZZZ
Keywords: already defined words
Reserved words: not yet defined, words reserved by the system
Chapter 2: Variables
1. Basic data types:
1.1 Integer types
byte-----(1 byte=8bit -128~127)
short----(2 bytes)
int-------(4 bytes, default type)
long-----(8 bytes. Declaration ends with L/l without default int)
1.2 Decimal type
float-----(4 bytes, accurate to 7 digits, add f/F single precision at the end)
double--(8 bytes, double precision, default type)
1.3 Character type
char-----(a character 2 bytes)
char a = 97 A = 65
1.4 Boolean type
boolean-(true,false)
Note: char byte short will be converted to int by default during operation
Reduce the precision of double type conversion
2. Reference data type:
String
3. Hexadecimal
Binary: 0~1
Octal: 0~7
Decimal: 0~9
Hexadecimal: 0~9 10~a 11~b 12~c 13~d 14~e 15~f
4. Type conversion:
Automatic conversion: small to large
Mandatory type conversion: large to small, prone to loss of data precision
int x = 100;
byte y= (byte)x;
5. Operators:
Remainder: % The result of taking the remainder depends on the sign before %
Auto-increment: ++ , auto-increment before ++x and auto-increment after x++
Decrement: -- , -- decrement x before x -- decrement after --
In r1: x1=3 (x1++ +)=4
*Stitching: (1+2+"1+2"+1+2) = "31+212"
6. Assignment operator:
x+=10 is equivalent to x=x+10
-= *= /= %= != ==
7. And (and) or (or) not (not) :
true && true == true true || false == true not true == false (!)
Short circuit phenomenon:
&& : the first expression is false and the result is false The second is short-circuited
|| : the first expression is true and the result is true ~
bitwise % bitwise | does not short circuit
8. Ternary operator: A>B?a:b
9. Priority:
Chapter 3: Flow Control Statements
1. Conditional statement
1.1: if statement
1. if
2. if.....else
3. if.....else if..... Stop if the first if condition is met
1.2: switch statement
2. Loop statement:
2.1 : while first judges and recycles the infinite loop while (true)
2.2: do...while loop first and then judge
2.3: for infinite loop: delete condition
2.4:for...each
Syntax: for(1 2 : 3){code block}
1 is the type of data obtained after traversal
2 is the name of the data traversed
,
3 is the data to be traversed
for(Integer i : a) {
System.out.print(i);
}
Note: You can use debug mode to enter the loop
3. Loop interruption
break: Terminate the execution of the statement block and exit the loop
continu: Only jump out of the loop and continue to execute the next loop
return : end the entire method
4. Data type:
1. Basic data types
data is stored
2. Reference data type
memory address is stored
2.1, array
2.2, class
2.3. Interface
Chapter 4, Arrays
1. Array: It can be regarded as a combination of multiple same data types to achieve unified management of these data
Note: After the array length is confirmed, it cannot be changed
reference data type
Array exception: storage exceeded (array out of bounds exception)
java.lang.ArrayIndexOutOfBoundsException
Runtime exception:
Compile-time exception: Error reported in java file---compile---class file
Null pointer exception:
Bubble Sort:
Tools:
Arrays: sort
copyof copy
Random number: 1~10
Random rd = new Random();
int x = rd.nextInt(10)+1;
【10,99】random two digits
Formula: (b-a+1)+a
(int)(Math.random( )*(99 - 10 +1) + 10
Chapter 5, Methods
main: the entry point of the program, the main method
Methods cannot be nested
static static can be called directly
void method return value no return value
The overall structure of the method:
[access control character][modifier] return value type method name (parameter){
method body
}
Category: with parameters Method: say (String name)
No-argument method: say()
Has a return value: int say(){ return 10}
no return value: void say()
Method overloading: The phenomenon of allowing more than one method with the same name to exist in a class is called overloading
Conditions: the parameter list is different, and the return value can be different
1. The number of parameters of the method can be different
say(int x)say (int x,int y)
2. The number is the same, but the type is different
say (int x) say (Sring name)
注、say (String name1,int name2)
say (int name1, Strng name2) also works
The method will only be executed when it is called
return: can only return once, but return can write multiple, end method
Must match the return value type in the declaration
Ordinary methods are called by objects
Static methods are called by classes and objects
Chapter 6: Object Orientation
Object-Oriented: Result-Oriented
1. Give all things/business to different objects to complete
2. Everything is an object
Process-oriented: focus on process/step
2. Pay attention to every step of the process to operate step by step
Main: The definition of classes and the use of objects are the core of Java language programming
Objects are created through classes
Concept: Classifying similar objects into a class defines common properties and methods
Summary: A class is a template for an object
Member variables: defined in the class (global variables)
Local variables: defined in the method
Object: an individual of a certain type of thing that actually exists, also known as an actual instance
Summary: The creation of objects is also known as instantiating classes
Construction method: used for attribute assignment, no return value, the name is consistent with the class name
Create objects with
Note: 1. If it is not written, the system will add a null parameter constructor by default in the class class
2. If the constructor is defined, the system will not append
3. The constructor is called when the class is instantiated
4. Generally, an empty structure will be appended by default
5. Multiple constructors can be created in a class
Features: 1. No return value type
2. The name is consistent with the class name
3. You cannot use return to return a value in a method
4. General access rights are public
Ordinary methods and constructors:
1. Ordinary method: complete the business Construction method: instantiate the class
2. With or without return type, value
Anonymous objects: single-use objects that become garbage after use
Garbage collection mechanism:
Scope: only applicable to heap memory, stack does not need to be cleaned up first in last out
When does it become trash?
1. After the anonymous object is used
2.Student s1 = new Student(1001, Zhang San, male, Shenyang Institute of Science and Technology);
s1 = null;
Student s1 = new Student(1001, Zhang San, male, Shenyang Institute of Science and Technology);
s1 = new Student(1001, Li Si, male, Shenyang Institute of Science and Technology);
Zhang San becomes trash
3. The first 99 times beyond the life cycle become
for(int i = 0;i<100;i++){
person p = new person( );
}
this keyword: currently represents the object of this class
1. Reference instance (this class) variable:
When the parameter in the method has the same name as the member variable of the current class, the local variable takes precedence, and the member variable is hidden.
Application situation:
1. When the variable and attribute in the method have the same name
2. Call other overloaded constructors in a constructor
this( ) can only be used in the constructor and must be placed on the first line
3. It can also be used in ordinary methods: return the reference of the current object
Chapter 7: Advanced Object-Oriented Features
1. Packaging
2. Inheritance
3. Polymorphism
Encapsulation: Encapsulation is the hiding of information, hiding the implementation details of objects from users to see, packaging things together, and then presenting them in a new and complete form.
1. Privatization attributes
2. Provide public methods to access attributes
3. Use the toString method to convert the data in the address stored in the class into a String type
advantage:
1. Allow users to access data only through pre-customized methods, and can easily add control logic to limit unreasonable operations on attributes
2. It is easy to modify and enhance the maintainability of the code
3. Data inspection is possible
Inheritance: (extension of class)
Definition: Inheritance is to derive a new subclass from the parent class (existing class), the new subclass can have the properties and methods of the parent class, and can extend its own new properties and methods
person human (parent class)
Student student class (subclass) worker worker class (subclass) Dog (dog class) cannot inherit
Conditions for inheritance to occur:
Under what circumstances can two classes have an inheritance relationship?
Subclasses can replace parent classes at any time
Subclass inheritance keyword extends word extension
The inheritance of classes in java is actually the extension of classes
Features:
1. The subclass inherits all the properties and methods of the parent class, and can also add its own properties and methods.
2. Java can only have single inheritance, that is to say, each class can only have one direct parent class
3. A parent class can have multiple subclasses
Subclass instantiation process:
1. When a subclass is instantiated, its parent class is instantiated first, and then the subclass is instantiated.
2. The construction method of the parent class must be called first, and then the construction method of the subclass is called after the construction method of the parent class is completed.
3. If an error occurs because the parent class does not have an empty structure
3.1 You can add an empty structure to the parent class
3.2 Use super
Method rewriting: @override
There must be an inheritance or implementation relationship
The difference between method rewriting and overloading:
1. There is an inheritance or implementation relationship in rewriting, and overloading does not need
2. Rewrite and change the method body, and overload to change the formal parameter list
The same point: but the method name does not change
make coding more efficient
Easy to maintain
code reuse
super keyword: call the parent class) constructor
Note: It can only appear in the construction method of the subclass, and must be the parameter in the first line of super(), which determines which construction method of the parent class to call
If super does not appear in the subclass construction method, the compiler will add super() by default, that is, call the empty construction method of the parent class. If the parent class does not have an empty construction method, the compiler will prompt an error.
The role of the java package:
Keyword: package
at the top of the class file
Only one package can be declared per source file
If there is no package statement, it defaults to an unnamed package (default package)
1. Packages allow classes to be combined into smaller units,
2. Helps avoid naming conflicts
3. Packages allow classes, data and methods to be protected on a wider scale
4. A package can be a collection of classes, interfaces and subpackages
Commonly used jar packages in java:
1. Basic core package:
java.lang: The base class library for the Java programming language
java.sql: Provides classes for accessing and processing data from a Java standard data source (usually a relational database)
java.util: includes various common toolkits such as collection classes, time processing modes, and date and time tools
java.io: Provides system input, output, file copy, and generation of word, ppt, and pdf through data streams, object sequences, and file systems
java.net provides all classes for implementing network communication applications
javax.swing: Provides a series of lightweight user interface components, which are currently commonly used packages for Java user interfaces
Import package keyword: import
Example: import java.util.Arrays;
import java.util.*; Import all packages *
The java.lang package does not need to be imported, the system automatically imports it
Note:
1. Only public classes in other packages can be imported;
2. A class can use multiple import statements to introduce other packages;
3. The import statement is the first non-comment statement after the package statement (if any).
Access modification:
protected When the inheritance condition occurs, it is consistent with the public scope
Does not inherit from the default scope
Method overriding (method rewriting):
The characteristics of the overridden method (subclass) and the overridden method (parent class):
1. same method name
2. The same parameter list (parameter number, parameter type, parameter order must be the same)
3. the same return type
4. The access rights of the overridden method of the subclass should be no less than the access rights of the overridden method in the parent class
frequent errors
1. Bracket problem occurs in pairs { } scope { } variables cannot have the same name
2. No other methods can be defined in the main method, only methods can be called
3. Careless
4 Run the program to find the master method
5 Clarify the idea of answering the question before writing *After reading the question
Upcasting: used in polymorphic features
That is, upward transformation: refers to the conversion of subclasses to parent classes, which is an automatic conversion
Animal an = new Cat ( ) ;
The upward transformation loses the properties and methods newly extended by the subclass, and only the properties and methods inherited from the parent class can be used
Downcasting:
That is, downcasting (Downcasting) is called mandatory conversion, which is to explicitly convert the parent class object into the subclass type.
* Objects that have been up-converted can only be down-converted. Objects do not allow direct downcasting without upcasting. Objects cannot be directly downcast
Person p parent class student s subclass p----->s
False: No upcasting, no direct downcasting
turn up first
Animal a = new Cat ( ) ;
further down
Cat a = (Cat) a ;
Instanceof (instance): Determine whether this class object belongs to a certain class
Cats cannot represent animals, animals include cats
Vehicle v = new Vehicle();
system.out.println(v instanceof Vehicle);
//Determine whether the v object belongs to an instance of the vehicle class
Instanceof class object has nothing to do with the class ---- error
Class object and parent class -------True
Class object and this class -------True
Parent class object and subclass ------- False
Both the cat object and the dog class inherit from animal ---- error
Polymorphism: different objects respond differently to the same behavior
Case 1: There is no inheritance to implement overloading of relational methods (compile-time polymorphism)
sort(array1)
sort(Number 1,Number 2,Number 3)
sort(object array Student[] stu)
(cover)
Case 2: There is inheritance to achieve relational shaping (polymorphism at runtime)
Polymorphic conditions:
There must be inheritance, or implementation.
have to rewrite
Parent class references point to subclass objects (upcasting)
Effect: Once the above three conditions are met, when the overridden method in the parent class is called, which subclass object is created at runtime, the overridden method in the subclass will be called.
Advantages of polymorphism:
Simplify the code
Improve code organization and readability
easy to expand
Static properties:
Elements that can be modified by static include: attributes, methods, and code blocks. The problem to be noted is that static can only modify class members, not local variables and constructors
Attribute: static variable public static String name;
Features: Member variables decorated with static, they are created when the class is loaded, as long as the class exists, the static variable exists.
Accessing static properties does not require class instantiation and does not require creating objects to access directly ---- class name. attribute
If it is a static property, there is only one in the space shared by all objects
public static string city="Shenyang City";
public static String city2="Shenyang City";
Only one "Shenyang City" is shared
Cannot refer to non-static members (including methods and variables) in static methods
The reason why it will report Cannot make a static reference to the non-static field email email---attribute
This error is because in static methods, non-static members (including methods and variables) cannot be directly accessed.
Because non-static variables depend on the existence of the object, the object must be instantiated before its variables will exist in memory.
Therefore, this thing is very simple to solve. First, you can change the variable to static. The second is to instantiate the object first, and then use the object name. Variable name to call
Method: add before the return value type public static void Test(){ }
Static code block: static { }
Note:
1. Static methods can only directly access static members, but not non-static members in the class. The attributes and local variables in the static method are all static, no need to be static
2. This and super keywords cannot be used in static methods
3. Static methods cannot be overridden by non-static methods (only the method body can be changed), and static cannot modify the
constructor
static application
Singleton mode: Singleton mode (singleton) is to ensure that there is only one instance of a class and provide a global access point to access it
Singleton mode:
1. Private constructor
2. Provide a static private member variable of itself
3. Provide public methods to instantiate objects
Effect:
1. A class can only have one instance and only one memory address without wasting resources
2. It must create the instance itself
final keyword: (modified keyword) appears in the same position as static
Modified attributes: Variables (properties and local variables) modified with final cannot be reassigned, assigned at the time of declaration, or assigned in the construction method, and will not assign initial values by default to final attributes.
Modified method: A method modified with final cannot be overridden (cannot be overridden) in a subclass, that is, it cannot be modified.
Modified class: final modified class cannot be inherited, but he can inherit other classes
Final variables are all capital letters, with underscores between words.
Abstract class:
1. Abstract method: The method modified by abstract is called abstract method
2. If a class has an abstract method, it must be an abstract class
3. There are two kinds of modifiers for modifying abstract classes: public and default modifiers;
4. Abstract classes can have abstract methods or non-abstract methods;
5. An abstract method is a method without a body. must be rewritten
6. Abstract methods only make sense if they are overridden
7. Abstract classes can inherit abstract classes without having to rewrite
Summary: abstract classes cannot be instantiated, they must be inherited, there is no constructor by default, you can add
After the subclass inherits the abstract class, it is either still an abstract class, or all the abstract methods of the parent class are rewritten
abstract Modifier class/method
Abstract method A method modified with abstract is called an abstract method
If a class has abstract methods, it must be an abstract class
/* This class is used as an abstract class constructor test */
/* Does the abstract class have a constructor? have
* Since abstract classes cannot be instantiated,
Why should there be a constructor?
* Not for personal use,
Instead, use super() when creating objects for subclasses; */
Abstract methods must be rewritten after subclass inheritance.
So, which keywords cannot be used with the abstract keyword? The following keywords, in abstract classes. It is possible to use it, but it is meaningless.
1.private: After being privatized, subclasses cannot rewrite it, which is contrary to abstract.
2. static: Static priority exists over objects, and there is a loading order problem.
3. final: After being modified by final, it cannot be rewritten, which is contrary to abstract.
interface (interface): not a class (class)
1. There are two kinds of modifiers for modifying interfaces: public and default modifiers;
Generally public
2. Interfaces can be multiple inheritance, interfaces can only inherit interfaces, not classes;
3. The attribute must be constant (with initial value), and the method must be abstract (no method body).
The default public abstract in the interface can not be written
Interface----class relationship: implement
implements
implements tool, execute
A class can implement an interface A class can implement multiple interfaces
Classes can inherit classes Classes can only inherit single
The interface solves the disadvantage that classes cannot inherit more, and classes can implement more
Interfaces can inherit multiple
Note: The class that implements the interface is called the implementation class of this interface, and the interface can accept all its implementation class objects
Summary: Four Questions
What is the interface?
An interface is a special abstract class that is used to describe a class that provides functional specifications to the outside world, capable of multiple inheritance
What can the interface do?
It can replace the class to achieve the role of multiple inheritance, and the interface can be implemented more
How to use the interface?
To implement by class, use class demo implements + interface name
Why use an interface?
To address the limitations of class inheritance
interface ------- class
1. Interface and interface --------- can inherit (multiple inheritance) extends
2. Class and class --------- can inherit (single inheritance) extends
3. Classes and interfaces --- ----- can implement (multiple implementations) implements
4. A class can either implement the interface or inherit the parent class extends implements can appear at the same time
abnormal:
Exceptions are some errors in the program, but not all errors are exceptions and some exceptions can be handled.
1. Syntax error: This error will be found when compiling the program
2. Logic error: This kind of error is rarely found easily when writing a program, because this kind of error is not a syntax error, so the compiler cannot prompt the corresponding error message.
3. Runtime error: Although some programs are compiled successfully, there are problems during the running process that cause the program to terminate abnormally, and a runtime error occurs
1. java.lang.ArithmeticException arithmetic exception
exception tree
Object class: a class without a parent class in java, the total class of all objects
Throwable class: is the general class of all errors and exceptions in the Java language
Eror class: specifically refers to serious errors that occur during the running of the application. Such as: virtual machine memory exhaustion, stack overflow and so on. In general, this kind of error is catastrophic, so there is no need to use exception handling mechanism to handle Error.
Exception: The general class of exceptions in the program, there are many different subclasses
Every subcategory is a mistake
RuntimeExcption: runtime exception
IOException : compile-time exception (red line under code)
Object general class (| inherited)
|
Throwable error class
| |
Error Error Exception Exception
| |
RuntimeExcption IOException
try{
}catch{
}
try: capture
catch: processing
Ways to handle exceptions:
1. Self-handling: Statements that may cause exceptions are enclosed in try blocks, and corresponding statements that handle exceptions are enclosed in catch blocks.
2. Avoid exceptions: Include a throws clause in the method declaration to notify potential callers that exceptions may occur in the method, but such exceptions are not handled and must be handled by the caller.
finally clause:
The finally statement is placed after the try ..catch statement, and the code block in the finlly statement is always executed regardless of whether the exception is caught or not
Note: When System.exit(0) is executed in the try or catch code block, the content in the finally code block will not be executed
System.exit(0): Force exit the virtual machine
Custom exception:
throw
throw
new
Exception();
1. The capture scope of the catch clause is limited to the matching try clause, and cannot catch exceptions in other try clauses;
2. The try clause cannot be used alone, it must be used in combination with the catch clause or the finally clause. Multiple catch clauses can be set, and the finally clause can only
there is one;
3. When there are multiple catch clauses, only the clause that catches the exception can be executed, and the remaining catch clauses cannot be executed;
4. The scope of variables in the three clauses of try/catch/finally is independent and cannot be accessed each other. If you want to be accessible in all three clauses, you need to define the variable outside these clauses;
5. Do not write too large a try clause, try not to have too many exceptions in a try clause;
6. If there is a possibility of an exception in a method, it can be caught and processed, or it can be declared to be handled by the caller;
7. No other statements are allowed after the throw statement, because these statements have no chance to execute;
8. Exception handling cannot be used to branch the program, it is just a mechanism to deal with abnormal situations.
Chapter 9: Tools
java API: Application Programming Interface
It is the java class of various functions provided by JDK
Object class: the superclass (parent class) of all classes
object is the only class in the Java language that has no parent class.
public boolean equals (Object obj):
Compares the values of two object references for equality (compare addresses).
Indicates whether the object calling this method is equal to the obj object. That is, whether the addresses are equal.
It will be rewritten (covered) when equals is used
For example, the Sring class will be rewritten, and then the values of the two strings will be compared.
equals is not rewritten and can only compare reference data types
== Reference data types can be compared with primitive data types
"=" operator:
〉Compare basic data types: equivalent to arithmetic equal sign
〉Compare reference data type: compare the referenced value and cannot be overwritten.
Usually, subclasses override equals() to change its meaning. Therefore, in some classes, equals( ) compares addresses, and in some classes, this method does not compare addresses. Specifically, it depends on the provisions of this method newly defined by the subclass. Look at the equals() method in the wrapper class. There is a rule in java: if equals() returns two objects that are equal, then the integers returned by calling hashCode() on these two objects must be equal. Otherwise, an error will occur when using the Hash type collection.
public int hashCode() :
This method returns the hash code of the object. The hash code is a hexadecimal integer representing the object, which is like the ID number of the object. During the running of the program, each time the same object is called
The hash code returned by hashCode() must be the same, but if the same program is executed multiple times, the hash code of the same object during one execution of the program and the next execution of the program may not be the same. In fact, the default hash code is obtained by converting the memory address of the object, so different objects will have different hash codes.
public String toString():
Returns the class name @hashcode; in fact returning such a string has no practical significance
Generally, subclasses will override this method to return meaningful text.
Wrapper class:
What is the difference between primitive data types and reference data types?
In java: Everything is object. Everything is an object
Wrap primitive data types into objects
Advantages: After becoming a wrapper class, the basic data type has its own properties and methods
Basic data type: -----> wrapper class
The information entered by the user is all data in the form of a string
Phone number: "86779312"
Age: "23"
id : "1001”
string <--------> wrapper class <-------> number
Integer
i = 10;
String
s1 = Integer.
toString(
i);
System.
out.println(
s1+1);
String
str =
"10";
int
x
= Integer.
parseInt
(
str
);
System.
out.println(
x+1);
Integer i = 10;
Integer and int can be converted directly
String class: The string class is a final type of class. cannot be inherited
String
str1 =
"hello";
//Constant pool (in the method area) 1.7 heap 1.8 element space
String
str2 =
new String(
"hello");
//in the heap
StringBuffer
sb =
new StringBuffer(
"good");
1.String: fixed-length string
Any modification of the String object to it actually produces a new string
Once the content of the String class object is initialized, it cannot be changed.
char[]
c = {
'h',
'e',
'l',
'l',
'o'};
String
s =
new String(
c,0,3);
//hel
byte[]
b = {76,111,118,101};
String
ss =
new String(
b);
System.
out.println(
ss);
//Love
string s1 ="hello";
String ss1 = "hello" ;
string ss2 = "hello" ;
String ss3 = "hello";
1. Only one hello in the constant pool is created and pointed to by four objects
2. Anywhere in the program, the same string literal constant is the same object
3. The strirg class resets the equals method, which is used to compare whether the contents of two strings are equal
2.StringBuffer: variable string
StringBuffer: You can use stringBuffer to dynamically manipulate the contents of the string without generating additional objects
append added at the end
insert Selected position to join
System.
out
.println(Math.
round
(-10.5));//-10 <=0.5 舍
>0.5 is -11
Date class:
java.util.Date date class: represents the specified time information, which can be accurate to milliseconds.
Lambda expressions
1. Prerequisites
Must be an interface. There can only be one abstract method in an interface
2. Use the format
(data type parameter name 1, data type parameter name 2,...) -> {method body}
( ): It means to execute the corresponding abstract method in the interface. If there are parameters in the method, then you need to pass the parameters
->: fixed format. The function is to pass the parameters in parentheses to the method body in curly brackets
{ }: represents the method body after implementing the abstract method in the interface
Use a Lambda expression to call a method with parameters and return type
Omission rules for Lambda expressions:
If there is only one parameter in the method, both the data type and parentheses can be omitted If there are multiple parameters in the method, the data type can be omitted
If there is only one line of code in the Collection interface in the braces, then the braces, return, and semicolon can all be omitted (the three must be omitted together)
Chapter 10: Assembly
Features: If you want to operate on variable-length data, you can use collections. (The number is uncertain)
Collection overview:
1. Collection classes in Java are used to store objects (wrapper classes)
2. The collection is equivalent to a container, which contains a group of objects - container class
3. Each object in it appears as an element of the collection
4. The collection class provided by the Java API is located in the java.util package
Collections and arrays:
1. The array is also a container, it has a fixed length, and the access is faster, but the array will not automatically expand
2. Arrays can contain objects of basic data types or reference types, while collections can only contain objects of reference types
Collection inheritance tree:
1、
List interface:
1. Orderly storage, allowing duplication
retainAll() retains only
Arraylist class
1. The bottom layer uses an array to realize the index value and the set does not
2. The initial value of the capacity is 10
3. Linear sequential storage is a linear table
Its properties are very close to arrays
Each expansion: current capacity + current capacity>>1 (about 1.5 times)
1. Expansion mechanism of ArrayList 1.
The calculation method of expansion is to shift to the right, namely: newSize = this.size +(this.size>>1). Shifting to the right, only when the current value is even, it is divided by 2; when it is odd, the last digit is erased, that is, first subtract 1, and then divide by 2;
2. The upper limit of expansion: the length of ArrayList is not unlimited, its default maximum length value is Integer...
ArrayList list = newArrayList<>(20);//Capacity 20
Unique methods in ArrayList (just understand):
ensureCapacity(int minCapacity)
If necessary, increase the capacity of this ArrayList instance to ensure that it can at least
Holds the number of elements specified by the minimum capacity parameter
We generally use the ArrayList constructor to specify the initial capacity trimToSize()
Resizes the capacity of this ArrayList instance to the current size of the list.
LinkedList class
Orderly and repeatable
1. It is the java implementation of the linked list in the data structure
Features: fast insertion and deletion, slow addition and deletion
of ArrayList
, but
high query efficiency
of ArrayList
Unique methods in LinkedList (just understand):
addFirst(E e)
Inserts the specified element at the beginning of this list.
addLast(E e)
Adds the specified element to the end of this list.
getFirst()
Returns the first element of this list.
getLast()
Returns the last element of this list.
removeFirst()
Removes and returns the first element of this list.
removeLast()
Removes and returns the last element of this list.
Stack: first in last out
push()
add the element to the beginning of this collection
pop()
Remove the first element of the collection and return
Queue: first in first out
offer adds the element to the end of this element poll
Remove the first element of the collection and return
descendingIterator() returns the iterator object in reverse order
Vector
Set interface (with sorting algorithm):
1. Unordered deposit, unique
HashSet class: Hash algorithm
comparetor :
int compare( T o1, T o2)
Compares the order of its two arguments. Returns a negative integer, zero, or a positive integer because the first argument is less than, equal to, or greater than the second argument.
TreeSet class: Binary Tree
2. Map interface
HashMap class:
Allow null values and keys
The default capacity is 16, and the capacity is doubled each time
newHashMap< >(32);//capacity 32
newHashMap<>(32,0.75f);// 0.75f load factor
new HashMap<>(hm3);//can add other map objects
Note: 1. An instance of HashMap has two parameters that affect its performance: initial capacity and load factor.
2. Load factor: The load factor is a measure that allows the hash table to be satisfied before the capacity is automatically increased. default 0.75
0.75: As a general rule, the default load factor (0.75 > provides a good compromise between time and space costs.
The expansion of Hashmap needs to meet two conditions: the number of current data storage (namely size()) must be greater than or equal to the threshold; whether the currently added data has a hash conflict. (1) When the hashmap is storing values (the default size is 16, the load factor is 0.75, and the threshold is 12), it is possible that when the last 16 values are stored, the expansion phenomenon will occur when the 17th value is stored, because each of the first 16 values occupies a position in the underlying array, and there is no hash collision.
High time complexity: sacrifice time to save memory
High space complexity: sacrifice memory to save time
1. The map stores key/value pairs such as paired object groups (a group of objects can be regarded as an element), and the "value" object is queried through the "key" object
key: key value: value where key is unique
2. Map is another collection interface different from collection
3. In Map, the key value is unique (cannot be repeated), and the key object is associated with the value object
traverse:
//Convert the map collection to a SET collection of Entry type
Set<Entry<String, String>>
entrySet =
hm.entrySet();
for (Entry<String, String>
entry :
entrySet) {
System.
out.println(
entry.getKey()+
"="+
entry.getValue());
}
isEmpty();
As long as the put() method is called, it is not empty
values() get all values
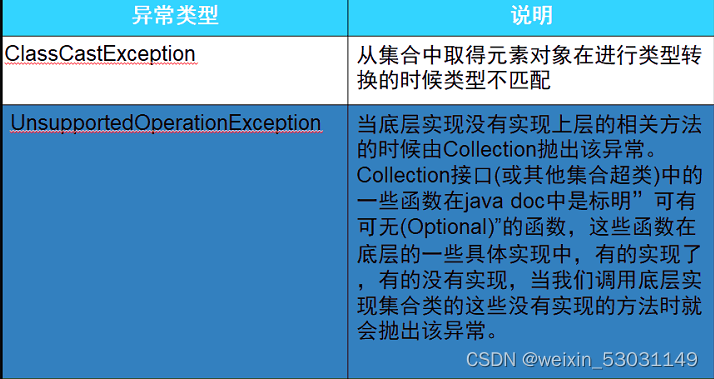
HashTable 类 :
Comparison between HashMap and Hashtable
Hashtable is based on the old Dictionary class, and HashMap is an implementation of the Map interface introduced in Java 1.2.
Hashtable is thread-safe, which means it is synchronized, while HashMap is thread-safe and not synchronized.
HashMap. Allows null as an entry key or value, while Hashtable does not allow null.
TreeMap class
Comparison of HashMap and TreeMap
HashMap is implemented based on a hash table.
TreeMap is implemented based on trees.
HashMap can optimize the use of HashMap space by tuning the initial capacity and load factor. TreeMap has no tuning options, because the tree is always in a balanced state
HashMap performance is better than TreeMap.
iterators:
Concept: The Iterator object is called an iterator, which is used to conveniently implement the traversal operation on the elements in the container.
Features: Iterator is designed for traversal, it can take out elements from the collection and delete elements (remove()), but there is no function of adding elements add()
The function of the element.
Iterator method:
next() : Return the element to the right of the cursor and move the cursor to the next position Get the data pointed to by the iterator and continue to move down
hasnext(): Determine whether there is an element to the right of the cursor
Process: first judge whether there is an element on the right through hasnext, if there is an element next() to get the data, if not, stop the next() method
Collections tool class:
copy(list l,list s):
1. The default number of elements when ArrayList is declared is 0, and the size of size() changes only when add() and remove() are performed.
2. When copying a collection, one must declare the number of elements in the destination collection, which must be equal to or greater than the number of elements in the source collection.
3. If it is not declared or is less than the number of elements in the source collection, an error will be reported, and an exception of the subscript boundary (java.lang.IndexOutOfBoundsException) will be reported.
// Declare a destination collection without specifying the number of elements in the collection
ArrayList<String> arrayList3 = new ArrayList<>();
//Add the null value of String type to the destination collection
Collections.addAll(
arrayList3,new String[arrayList1.size()]);
Generics:
Problems with using collections:
The add() method in the collection accepts a parameter of an Object object. When obtaining an object in the collection, it must perform a casting (forced type conversion) operation. There may be problems in the modeling operation, which usually only happen when the program is running, and it is difficult to find the problems.
The use of generics in collections:
1. Before the object is put into the collection, set a limit for it
2. When obtaining the objects in the collection, no modeling operation is required
3. When different types of objects are added to the collection, errors can be checked at compile time
Files and streams:
1. Documents:
1. The management of files in Java is realized through the File class in the java.io package
Mainly for the management of file or directory path names
>Property information of the file
> Document inspection
>Deletion of files, etc.
>does not include the access king of the file
Stream: the circulation of data in a program (data flow)
1. The data stream is a series of continuous data collection, kb, Mb, GB
2. In the Java program, the input/output operation of data is performed in the form of Stream.
Analyzing conditions:
input stream: the incoming program's
Output stream: Outgoing program's
In a java program, data is read from the input stream (read into memory), and data is output from the output stream (stored from memory to a file or displayed on the screen)
Classification of streams:
Depending on the direction of flow
> input stream, output stream
According to the unit of processing data
> byte stream, character stream
by function
> Node flow (low-level flow), processing flow (high-level flow)
In the Java language, classes that control data flow are placed in the java.io package
Byte input stream: inputStream
Byte output stream: outputStream
Character input stream: Reader read: input
byte input stream: writer write out: output
fis1
.read(
b
)
b: array
byte
[]
b
=
new
byte
[1024];
When the storage is full, return 1024, and when the storage is complete, return -1
Advanced byte input stream BufferedInputStream
Advanced byte output stream BufferedOutputStream
object serialization
The mechanism for saving and reading objects by using the objectInputStream and objectOutputStream classes is called the serialization mechanism
1. ObjectInputStream
2. ObjectoutputStream
What is object serialization:
Serializable
serialization interface,
identification interface
The Serializable interface does not define any properties or methods. It is only used to indicate that a class can be serialized. If a class is serializable, all its subclasses are serializable
Object serialization refers to the process of converting an object into a sequence of bytes
Deserialization: ObjectInputStream stream readObject
Byte output stream: FileoutputStream
Features:
The output stream writes data, and when the file is associated, if the file does not exist, it will be created automatically
The output stream writes data. If the associated file already exists, the original content will be cleared and written again.
If you don't want to clear the original content, you can add a true switch at the position of the construction method
The role of the character stream:
When operating plain text files, it can solve the problem of Chinese garbled characters
Question: how to solve it?
Answer: The bottom layer of the character stream is actually read in the form of (byte stream + encoding table)
GBK -> a Chinese takes up 2 bytes
UTF-8->One Chinese occupies 3 bytes
According to Pinshe's default encoding table, it will decide to read several bytes at a time and convert them into characters in memory
Question: If there are non-Chinese characters in the text, why there are no garbled characters during the reading process?
Answer: There is a judgment at the bottom of the character stream. If the read is a Chinese character, read 2 bytes at a time (GBK)
If non-Chinese characters are read, then read one byte at a time.
Question: How does the system judge whether the currently read character is Chinese?
Answer: Chinese characters are generally negative bytes, and non-Chinese characters are positive numbers. Generally, they are negative bytes, some Chinese -> the first byte is negative, and the following bytes may be positive
Filewriter -> character output stream
Note:
1. If the character output stream does not call the close method\flush method, the data will not be written out to the file
2. The main function of the close method is to close the stream to release resources, and it also has the effect of brushing out data
After the close method is called, the write method cannot be called to write data,
character buffer stream
BufferedInputStream BufferedOutputStream
BufferedReader
readLine() -> Read a whole line of string at a time End mark: null will not read carriage return and line feed
Bufferedwriter
newLine()->write a carriage return and line feed, but this method has a cross-platform effect
Question: What is the role of the transformation stream?
The conversion stream can read and write data according to the specified encoding table
InputStreamRreader :
A bridge from byte streams to character streams
InputStreamReader(InputStream in)
InputStreamReader( InputStream in, String charsetName)
Multithreading:
As long as accessing resources is multi-threaded
Process: It is a running application. A process can contain multiple threads, but at least one thread must be executing.
After a Java program runs, at least one main thread will be opened
Thread: An execution path of the program, multi-threading means that multiple execution paths are executing at the same time (illusion)
CPU switching is fast
There are two ways to implement multithreading:
1. Inherit the Thread class
2. Implement the Runnable interface
3. Both of the above need to complete multi-threading through the Thread class construction
4. Call the start() method to activate the thread object
1. Inherit the thread class / implement the Runnable interface
2. Rewrite the run method
3. Write the task to be executed in the run method
4. Create a thread object
5. Call the start method to start the thread. Automatically call the run method/
Pass the Runnable implementation class object as a resource to the parameterized structure of the Thread class, and call the start method to start the thread
new Thread(
thread object
).start()
Summary: Only when the start method is called, can the thread be truly opened
If only the run method is called, it is only a call to the member method, not to start the thread.
How to get the thread name?
Thread class:
getName( );
Thread.currentThread().
getName()
: Get the name of the currently executing thread object
How to set a name for a thread?
Thread类 :
setName(String name);
Through the constructor of the Thread class, directly specify
new Thread(b,"Thread B-->").start();
Callable: It can not only open the thread, but also get the return value result of the thread
1. Implement the Callable interface and rewrite the call method
2. Create a FutureTask object and pass the callable implementation class object into the constructor
3. Create a Thread class object, pass FutureTask into the constructor, and call the start method to start the thread
Call the get method to get the return value of the call method
Implementing the Runnable interface (or Callable interface) has the following significant benefits compared to inheriting the Thread class to implement multi-threading:
It is suitable for multiple threads to process the same shared resource. The limitations brought about by Java single inheritance can be avoided.
Inherit the Thread class: you can easily use any method in the parent class
Note: In fact, most multi-threaded applications in actual development will use the Runnable interface or Callable interface to implement multi-threading.
t1
.setDaemon(
true
); set to background thread
isDaemon() judgment
Timed wait:
Thread.sleep(1000...); thread sleep
Thread.yield(); Thread yield
t1.join(); thread insertion can set the parameter ti insertion time
In the application program, the most direct way to schedule threads is to set the priority of threads. A thread with a higher priority has a greater chance of getting CPU execution, while a thread with a lower priority has a smaller chance of getting CPU execution.
The priority of a thread is represented by an integer between 1 and 10, and the larger the number, the higher the priority.
In addition to directly using numbers to represent the priority of the thread, you can also use the three provided in the Thread class
A static constant representing the thread's priority.
t1.
setPriority(10);
t1.
setPriority
(Thread.Max_priority);
Application scenarios of synchronization technology:
When the code of a thread is executing, we hope that the CPU will not switch to other threads for work. At this time, synchronization technology can be used
Synchronized code block:
synchronized (lock object) {
// any type of object
}
Any type of object: lock objects of multiple threads, must be the same lock
It is recommended to use a universal lock: class name.class -> bytecode object
Synchronization method:
(not recommended to be cumbersome)
@Override
public void run() {
while (true) {
method();
}
}
public synchronized void method() {
}
Starting from JDK 5, Java has added a more powerful Lock lock. Lock lock with
The synchronized implicit lock is basically the same in function. Its biggest advantage is that the Lock lock can allow a thread to return after failing to continuously acquire the synchronization lock without continuing to wait. In addition, the Lgck lock is more flexible in use.
Synchronization lock:
Lock lock = new Lock();
lock.lock(); lock
lock.unlock(); release the lock
Deadlock: Definition: Two threads are waiting for each other's lock when they are running, which causes the program to stagnate. This phenomenon is called deadlock.
Synchronization nested synchronization causes
Ways to avoid deadlock:
1. Locking order (threads are locked in a certain order)
2. Locking time limit (add a certain time limit when the thread tries to acquire the lock, and give up the lock if it exceeds the time limit
request, and release the lock it owns)
3. Deadlock detection
Ticket grabbing system:
Errors will occur in concurrent access by multiple threads, and resources need to be locked
synchronized
Design Patterns:
1. Producer and consumer model
Case: coffee refill
Producer: The clerk who produces coffee, refills the coffee, wakes up and waits after the refill is complete
Consumers: grab coffee, whoever grabs it will drink, wake up the clerk to refill, grab coffee
Thread:
wait( ) wait release lock while waiting
notifyyAll( ) wake up
sleep( ) Sleep does not release the lock when sleeping
join( )
annotation
Annotation is a new technology introduced from JDK5.0.
The role of Annotation:
It is not the program itself, it can explain the program. (This is no different from a comment)
Can be read by other programs (such as: compiler, etc.).
Annotation format:
Annotations exist in the code as "@ annotation name", and some parameter values can also be added, for example: @SuppressWarnings(value="unchecked")
Where is Annotation used?
It can be attached to package, class, method, field, etc., which is equivalent to adding additional auxiliary information to them. We can program to access these metadata through the reflection mechanism
The role of meta-annotation is to annotate other annotations. Java defines four standard meta-annotation types, which are used to provide explanations for other annotation types.
These types and their supporting classes are found in the java.lang.annotation package. (Target , @Retention , @Documented , @Inherited )
Target: Used to describe the scope of use of annotations (ie: where the described annotations can be used)
@Retention: indicates at what level the annotation information needs to be saved, and is used to describe the life cycle of the annotation
(SOURCE<CLASS< RUNTIME)
>@Document: Indicates that the annotation will be included in javadoc
@Inherited: Indicates that subclasses can inherit the annotation in the parent class
reflection
Reflection (reflection) is the key to Java being regarded as a dynamic language. The reflection mechanism allows the program to obtain internal information of any class by means of Reflection APl during execution, and can directly manipulate the internal properties and methods of any object.
Class c= Class.forName(java.lang.String")
After the class is loaded, an object of Class type is generated in the method area of the heap memory (a class has only one Class object), and this object contains the complete structural information of the class. We can see the class structure through this object. This object is like a mirror, through which we can see the structure of the class, so we call it vividly: reflection
advantage:
Dynamic object creation and compilation can be realized, showing great flexibility
shortcoming:
have an impact on performance. Using reflection is basically an interpreted operation, we can tell the JVM, what we want to do and it does what we want. Such operations are always slower than performing the same operations directly.
java.lang.Class: represents a class
java.lang.reflect.Method: represents the method of the class
java.lang.reflect.Field: represents the member variable of the class
java.lang.reflect.Constructor: represents the constructor of the class
If the specific class is known, it can be obtained through the class attribute of the class. This method is the most safe and reliable, and the program performance is the highest.
Class clazz= Person.class;
Knowing an instance of a certain class, call the getClass() method of the instance to obtain the Class object
Class clazz = person.getClass();
The full class name of a class is known, and the class is in the class path, which can be obtained through the static method
forName()
of the Class class , and ClassNotFoundException may be thrown
Class clazz= Class.forName("demo01.Student");
The built-in basic data type can directly use the class name.Type
You can also use ClassLoader, which we will explain later
Which types can have Class objects?
class: outer class, member (member inner class, static inner class), local inner class, anonymous inner class
interface: interface
[]: array one-dimensional and two-dimensional
enum: enumeration
annotation: annotation @interface
primitive type: basic data type
void:
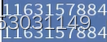
private
static
<
E
>
void
print
(
E
[
]
e
)
{
for
(
E
d
:
e
)
{
System
.
out
.
println
(
d
)
;
}
}
java中对象和对象引用的区别
1.何谓对象?
在Java中有一句比较流行的话,叫做“万物皆对象”,这是Java语言设计之初的理念之一。要理解什么是对象,需要跟类一起结合起来理解。下面这段话引自《Java编程思想》中的一段原话:
“按照通俗的说法,每个对象都是某个类(class)的一个实例(instance),这里,‘类’就是‘类型’的同义词。”
从这一句话就可以理解到对象的本质,简而言之,它就是类的实例,比如所有
的人统称为“人类”,这里的“人类”就是一个类(物种的一种类型),而具体到每个人,比如张三这个人,它就是对象,就是“人类”的实例。
2.何谓对象引用?
我们先看一段话:
“每种编程语言都有自己的数据处
理方式。有些时候,程序员必须注意将要处理的数据是什么类型。你是直接操纵元素,还是用某种基于特殊语法的间接表示(例如C/C++里的指针)来操作对象。所有这些在 Ja
va 里都得到了简化,一切都被视为对象。因此,我们可采用一种统一的语法。尽管将一切都“看作”对象,但操纵的标识符实际是指向一个对象的“引用”(reference)。”
这段话来自于《Java编程思想》,很显然,从这段话可以看出对象和对象引用不是一回事,是两个完全不同的概念。举个例子,我们通常会用下面这一行代码来创建一个对象:
Person person = new Person("张三");
有人会说,这里的person是一个对象,是Person类的一个实例。
Some people will also say that the person here is not a real object, but a reference to the created object.
Which statement is correct? Let's not worry about which statement is right, and then look at the two lines of code:
Person person;
person = new Person("Zhang San");
The functions implemented by these two lines of code are exactly the same as the above one line of code. Everyone knows that in Java, new is used to create objects on the heap. If person is an
object , why does the second line
need to use new to create objects?
It can be seen that person is not the created
object, what is it? The above paragraph is very clear, "The manipulated identifier is actually a reference to an object", that is to say, a person is a reference, which is a reference to an object that can point to the Person class
. The statement that actually creates the object is new Person("Zhang San");
Annotation is a new technology introduced from JDK5.0.
The role of Annotation
:
It is not the program itself, it can explain the program. (This is no different from a comment)
Can be read by other programs (such as: compiler, etc.).
Annotation format:
Annotations exist in the code with "@ annotation name", and some parameter values can also be added, for example: @SuppressWarnings(value="unchecked")
Where is Annotation used?
>It can be attached to package, class, method, field, etc., which is equivalent to adding additional auxiliary information to them. We can program to access these metadata through the reflection mechanism
@Override:定义在java.lang.Override 中,此注释只适用于修辞方法﹐表示一个方法声明打算b
重写超类中的另一个方法声明.
@Deprecated:定义在java.lang.Deprecated中,此注释可以用于修辞方法﹐属性﹐类﹐表示
不鼓励程序员使用这样的元素﹐通常是因为它很危险或者存在更好的选择.
@suppressWarnings:
(镇压警告)定义在java.lang.SuppressWarnings中,用来抑制编译时的警告信息.与前两个注释有所不同,你需要添加一个参数才能正确使用,这些参数都是已经定义好了的,我们选择性的使用就好了﹒
@SuppressWarnings("all")
@SuppressWarnings("unchecked")
@SuppressWarnings(value={"unchecked" , "deprecation"})
元注解的作用就是负责
注解其他注解,Java定义了4个标准的meta-annotation类型,他们被用来提供对其他annotation类型作说明.
These types and their supporting classes are found in the java.lang.annotation package. ( @Target , @Retention , Documented , @lnherited )
Target: Used to describe the scope of use of annotations (ie: where the described annotations can be used)
@Retention: indicates at what level the annotation information needs to be saved, and is used to describe the life cycle of the annotation
(SOURCE< CLASS< RUNTIME)
@Document: Indicates that the annotation will be included in javadoc
@Inherited: Indicates that subclasses can inherit the annotation in the parent class